Typescript Spread Operator
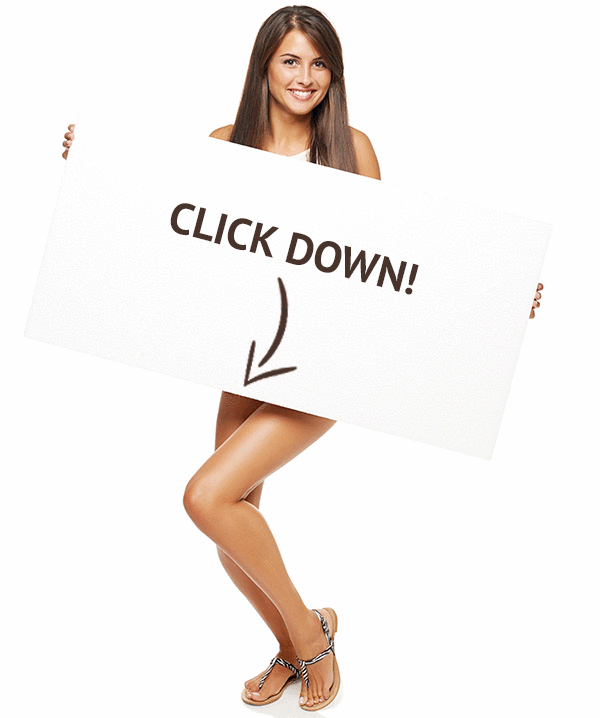
🔞 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Typescript Spread Operator
//iterate args array, add all numbers and return sum
let origArrayOne = [ 1, 2, 3]; //1,2,3
let origArrayTwo = [ 4, 5, 6]; //4,5,6
//Create new array from existing array
let copyArray = [...origArrayOne]; //1,2,3
//Create new array from existing array + more elements
let newArray = [...origArrayOne, 7, 8]; //1,2,3,7,8
//Create array by merging two arrays
let mergedArray = [...origArrayOne, ...origArrayTwo]; //1,2,3,4,5,6
let origObjectOne = {a: 1, b: 2, c: 3}; //{a: 1, b: 2, c: 3}
let origObjectTwo = {d: 4, e: 5, f: 6}; //{d: 4, e: 5, f: 6}
//Create new object from existing object
let copyObject = {...origObjectOne}; //{a: 1, b: 2, c: 3}
//Create new object from existing object + more elements
let newObject = {...origObjectOne, g: 7, h: 8}; //{a: 1, b: 2, c: 3, g: 7, h: 8}
//Create object by merging two objects
let mergedObject = {...origObjectOne, ...origObjectTwo}; //{a: 1, b: 2, c: 3, d: 4, e: 5, f: 6}
myFunction(...parametersArray); //0, 1, 2
console.log.apply(console, numbers); //1 2 3 4 5
console.log( "1" , "2" , "3" , "4" , "5" ); //1 2 3 4 5
console.log(...argsArray); //1 2 3 4 5
Let us know if you liked the post. That’s the only way we can improve.
Subscribe to the newsletter and updates!
Copyright © 2022 · Hosted on Cloudways · Sitemap
The spread operator is a new addition to the features available in the JavaScript ES6 version. The spread operator is used to expand or spread an iterable or an array.
The spread operator (in form of ellipsis ) can be used in two ways:
The spread operator is most widely used for method arguments in form of rest parameters where more than 1 value is expected. A typical example can be a custom sum(...args) method which can accept any number of method arguments and add them to produce the sum.
We can use the spread operator to create arrays from existing arrays in the given fashion.
We can also use the spread operator to create objects from the existing objects in the given fashion.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
The JavaScript’s apply() method calls a function with a given this value, and arguments provided as an array . For example, in the below example, both highlighted function calls are equivalent. They both print the same output.
Spread operator (part of ECMAScript 6) is a better version of the apply() method. Using the spread operator, we can write the above statement as given below.
This blog provides tutorials and how-to guides on Java and related technologies.
It also shares the best practices, algorithms & solutions, and frequently asked interview questions.
myFunction ( a , ... iterableObj , b )
[ 1 , ... iterableObj , '4' , 'five' , 6 ]
{ ... obj , key : 'value' }
const obj = { key1 : 'value1' } ;
const array = [ ... obj ] ; // TypeError: obj is not iterable
const array = [ 1 , 2 , 3 ] ;
const obj = { ... array } ; // { 0: 1, 1: 2, 2: 3 }
function myFunction ( x , y , z ) { }
const args = [ 0 , 1 , 2 ] ;
myFunction . apply ( null , args ) ;
function myFunction ( x , y , z ) { }
const args = [ 0 , 1 , 2 ] ;
myFunction ( ... args ) ;
function myFunction ( v , w , x , y , z ) { }
const args = [ 0 , 1 ] ;
myFunction ( - 1 , ... args , 2 , ... [ 3 ] ) ;
const dateFields = [ 1970 , 0 , 1 ] ; // 1 Jan 1970
const d = new Date ( ... dateFields ) ;
const parts = [ 'shoulders' , 'knees' ] ;
const lyrics = [ 'head' , ... parts , 'and' , 'toes' ] ;
// ["head", "shoulders", "knees", "and", "toes"]
const arr = [ 1 , 2 , 3 ] ;
const arr2 = [ ... arr ] ; // like arr.slice()
arr2 . push ( 4 ) ;
// arr2 becomes [1, 2, 3, 4]
// arr remains unaffected
const a = [ [ 1 ] , [ 2 ] , [ 3 ] ] ;
const b = [ ... a ] ;
b . shift ( ) . shift ( ) ;
// 1
// Oh no! Now array 'a' is affected as well:
a
// [[], [2], [3]]
let arr1 = [ 0 , 1 , 2 ] ;
const arr2 = [ 3 , 4 , 5 ] ;
// Append all items from arr2 onto arr1
arr1 = arr1 . concat ( arr2 ) ;
let arr1 = [ 0 , 1 , 2 ] ;
const arr2 = [ 3 , 4 , 5 ] ;
arr1 = [ ... arr1 , ... arr2 ] ;
// arr1 is now [0, 1, 2, 3, 4, 5]
const arr1 = [ 0 , 1 , 2 ] ;
const arr2 = [ 3 , 4 , 5 ] ;
// Prepend all items from arr2 onto arr1
Array . prototype . unshift . apply ( arr1 , arr2 ) ;
// arr1 is now [3, 4, 5, 0, 1, 2]
let arr1 = [ 0 , 1 , 2 ] ;
const arr2 = [ 3 , 4 , 5 ] ;
arr1 = [ ... arr2 , ... arr1 ] ;
// arr1 is now [3, 4, 5, 0, 1, 2]
const obj1 = { foo : 'bar' , x : 42 } ;
const obj2 = { foo : 'baz' , y : 13 } ;
const clonedObj = { ... obj1 } ;
// Object { foo: "bar", x: 42 }
const mergedObj = { ... obj1 , ... obj2 } ;
// Object { foo: "baz", x: 42, y: 13 }
const obj1 = { foo : 'bar' , x : 42 } ;
Object . assign ( obj1 , { x : 1337 } ) ;
console . log ( obj1 ) ; // Object { foo: "bar", x: 1337 }
const objectAssign = Object . assign ( { set foo ( val ) { console . log ( val ) ; } } , { foo : 1 } ) ;
// Logs "1"; objectAssign.foo is still the original setter
const spread = { set foo ( val ) { console . log ( val ) ; } , ... { foo : 1 } } ;
// Nothing is logged; spread.foo is 1
const obj1 = { foo : 'bar' , x : 42 } ;
const obj2 = { foo : 'baz' , y : 13 } ;
const merge = ( ... objects ) => ( { ... objects } ) ;
const mergedObj1 = merge ( obj1 , obj2 ) ;
// Object { 0: { foo: 'bar', x: 42 }, 1: { foo: 'baz', y: 13 } }
const mergedObj2 = merge ( { } , obj1 , obj2 ) ;
// Object { 0: {}, 1: { foo: 'bar', x: 42 }, 2: { foo: 'baz', y: 13 } }
const obj1 = { foo : 'bar' , x : 42 } ;
const obj2 = { foo : 'baz' , y : 13 } ;
const merge = ( ... objects ) => objects . reduce ( ( acc , cur ) => ( { ... acc , ... cur } ) ) ;
const mergedObj1 = merge ( obj1 , obj2 ) ;
// Object { foo: 'baz', x: 42, y: 13 }
Web technology reference for developers
Code used to describe document style
Protocol for transmitting web resources
Interfaces for building web applications
Developing extensions for web browsers
Web technology reference for developers
Learn to structure web content with HTML
Learn to run scripts in the browser
Learn to make the web accessible to all
Frequently asked questions about MDN Plus
Spread syntax ( ... ) allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created.
Spread syntax looks exactly like rest syntax. In a way, spread syntax is the opposite of rest syntax. Spread syntax "expands" an array into its elements, while rest syntax collects multiple elements and "condenses" them into a single element. See rest parameters and rest property .
Spread syntax can be used when all elements from an object or array need to be included in a new array or object, or should be applied one-by-one in a function call's arguments list. There are three distinct places that accept the spread syntax:
Although the syntax looks the same, they come with slightly different semantics.
Only iterable objects, like Array , can be spread in array and function parameters. Many objects are not iterable, including all plain objects that lack a Symbol.iterator method:
On the other hand, spreading in object literals enumerates the own properties of the object. For typical arrays, all indices are enumerable own properties, so arrays can be spread into objects.
When using spread syntax for function calls, be aware of the possibility of exceeding the JavaScript engine's argument length limit. See Function.prototype.apply() for more details.
It is common to use Function.prototype.apply() in cases where you want to
use the elements of an array as arguments to a function.
With spread syntax the above can be written as:
Any argument in the argument list can use spread syntax, and the spread syntax can be
used multiple times.
When calling a constructor with new , it's not possible to directly use an array and apply() , because apply() calls the target function instead of constructing it, which means, among other things, that new.target will be undefined . However, an array can be easily used with new thanks to spread syntax:
Without spread syntax, to create a new array using an existing array as one part of it,
the array literal syntax is no longer sufficient and imperative code must be used
instead using a combination of push() ,
splice() , concat() , etc. With spread syntax this becomes much more succinct:
Just like spread for argument lists, ... can be used anywhere in the array
literal, and may be used more than once.
Note: Spread syntax effectively goes one level deep while copying an array. Therefore, it may be unsuitable for copying multidimensional arrays. The same is true with Object.assign() — no native operation in JavaScript does a deep clone.
Array.prototype.concat() is often used to concatenate an array to the end
of an existing array. Without spread syntax, this is done as:
Array.prototype.unshift() is often used to insert an array of values at
the start of an existing array. Without spread syntax, this is done as:
Note: Unlike unshift() , this creates a new arr1 , instead of modifying the original arr1 array in-place.
Shallow-cloning (excluding prototype) or merging of objects is possible using a shorter syntax than Object.assign() .
Note that Object.assign() can be used to mutate an object, whereas spread syntax can't.
In addition, Object.assign() triggers setters on the target object, whereas spread syntax does not.
You cannot naively re-implement the Object.assign() function through a single spreading:
In the above example, the spread syntax does not work as one might expect: it spreads an array of arguments into the object literal, due to the rest parameter. Here is an implementation of merge using the spread syntax, whose behavior is similar to Object.assign() , except that it doesn't trigger setters, nor mutates any object:
BCD tables only load in the browser
Last modified: Aug 16, 2022 , by MDN contributors
Your blueprint for a better internet.
Visit Mozilla Corporation’s not-for-profit parent, the Mozilla Foundation . Portions of this content are ©1998– 2022 by individual mozilla.org contributors. Content available under a Creative Commons license .
Sign up or log in to customize your list.
more stack exchange communities
company blog
Stack Overflow for Teams
– Start collaborating and sharing organizational knowledge.
Create a free Team
Why Teams?
Asked
2 years, 2 months ago
5,432 13 13 gold badges 52 52 silver badges 103 103 bronze badges
Highest score (default)
Trending (recent votes count more)
Date modified (newest first)
Date created (oldest first)
60.4k 7 7 gold badges 71 71 silver badges 83 83 bronze badges
859 6 6 silver badges 14 14 bronze badges
2,509 3 3 gold badges 18 18 silver badges 15 15 bronze badges
133k 75 75 gold badges 622 622 silver badges 665 665 bronze badges
Stack Overflow
Questions
Help
Products
Teams
Advertising
Collectives
Talent
Company
About
Press
Work Here
Legal
Privacy Policy
Terms of Service
Contact Us
Cookie Settings
Cookie Policy
Stack Exchange Network
Technology
Culture & recreation
Life & arts
Science
Professional
Business
API
Data
Accept all cookies
Customize settings
Find centralized, trusted content and collaborate around the technologies you use most.
Connect and share knowledge within a single location that is structured and easy to search.
In the above ts is complaining about
Any idea how I can fix it? i.e how can I allow to overwrite
Trending sort is based off of the default sorting method — by highest score — but it boosts votes that have happened recently, helping to surface more up-to-date answers.
It falls back to sorting by highest score if no posts are trending.
Typescript is pointing out that these two lines are useless:
You're setting these properties manually, but then you immediately spread over them, wiping them out. This is probably not what you meant to do. If you want these two values to trump what's found in additionalProperties , then switch the order:
If the order is correct, but additionalProperties won't always have color and backgroundColor , then mark those as optional:
Or if it's behaving the way you want (ie, the properties are always getting overwritten, and that's intentional), then delete the useless lines of code.
I don't know if there is a configuration for this. However, you could destructure additionalProperties to exclude color and backgroundColor :
If you want those props to be just default values, you can make use of Object.assign.
Typescript's Partial utility type seems the best way to make this happen.
In your example, the constructor would be changed to:
I had some defaults set like this that weren't a problem until Typescript 4.4 (possibly an earlier version) :
I was now getting the error Error TS2783: 'stateOrProvince' is specified more than once, so this usage will be overwritten. which makes sense.
The destination for this value is an Angular form which requires empty fields to be explicitly set. While normally addressFields would contain a value for every field (as specified by its type) I had some older customers who may have a cached value in their local storage without all these fields hence these defaults.
Fortunately the following seems to get around the error, and I think looks nicer anyway as it groups things together.
Not 100% sure why the compiler lets this through but it does :-)
Thanks for contributing an answer to Stack Overflow!
By clicking “Post Your Answer”, you agree to our terms of service , privacy policy and cookie policy
To subscribe to this RSS feed, copy and paste this URL into your RSS reader.
Site design / logo © 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA . rev 2022.9.7.42963
By clicking “Accept all cookies”, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy .
Sign up or log in to customize your list.
more stack exchange communities
company blog
Stack Overflow for Teams
– Start collaborating and sharing organizational knowledge.
Create a free Team
Why Teams?
Asked
6 years, 10 months ago
Modified
1 year, 2 months ago
46.2k 8 8 gold badges 93 93 silver badges 140 140 bronze badges
3,912 7 7 gold badges 21 21 silver badges 38 38 bronze badges
Highest score (default)
Trending (recent votes count more)
Date modified (newest first)
Date created (oldest first)
var __read = (this && this.__read) || function (o, n) {
var m = typeof Symbol === "function" && o[Symbol.iterator];
if (!m) return o;
var i = m.call(o), r, ar = [], e;
try {
while ((n === void 0 || n-- > 0) && !(r = i.next()).done) ar.push(r.value);
}
catch (error) { e = { error: error }; }
finally {
try {
if (r && !r.done && (m = i["return"])) m.call(i);
}
finally { if (e) throw e.e
Lesbian Kiss Hd
Rocco S Intimate Castings 22 Evil Angel
Sexy Womens Lingerie