Spread Rest Js
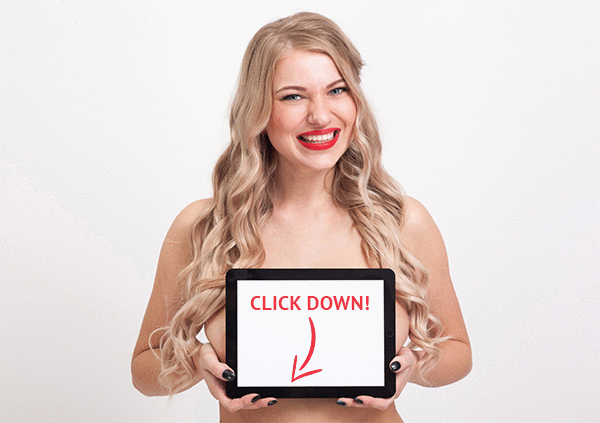
🛑 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Spread Rest Js عربي English Español Français Indonesia Italiano 日本語 한국어 Русский Türkçe Українська 简体中文 function sum ( a , b ) { return a + b ; } alert ( sum ( 1 , 2 , 3 , 4 , 5 ) ) ; function sumAll ( ... args ) { // args is the name for the array let sum = 0 ; for ( let arg of args ) sum += arg ; return sum ; } alert ( sumAll ( 1 ) ) ; // 1 alert ( sumAll ( 1 , 2 ) ) ; // 3 alert ( sumAll ( 1 , 2 , 3 ) ) ; // 6 function showName ( firstName , lastName , ... titles ) { alert ( firstName + ' ' + lastName ) ; // Julius Caesar // the rest go into titles array // i.e. titles = ["Consul", "Imperator"] alert ( titles [ 0 ] ) ; // Consul alert ( titles [ 1 ] ) ; // Imperator alert ( titles . length ) ; // 2 } showName ( "Julius" , "Caesar" , "Consul" , "Imperator" ) ; The rest parameters must be at the end function f ( arg1 , ... rest , arg2 ) { // arg2 after ...rest ?! // error } function showName ( ) { alert ( arguments . length ) ; alert ( arguments [ 0 ] ) ; alert ( arguments [ 1 ] ) ; // it's iterable // for(let arg of arguments) alert(arg); } // shows: 2, Julius, Caesar showName ( "Julius" , "Caesar" ) ; // shows: 1, Ilya, undefined (no second argument) showName ( "Ilya" ) ; Arrow functions do not have "arguments" function f ( ) { let showArg = ( ) => alert ( arguments [ 0 ] ) ; showArg ( ) ; } f ( 1 ) ; // 1 alert ( Math . max ( 3 , 5 , 1 ) ) ; // 5 let arr = [ 3 , 5 , 1 ] ; alert ( Math . max ( arr ) ) ; // NaN let arr = [ 3 , 5 , 1 ] ; alert ( Math . max ( ... arr ) ) ; // 5 (spread turns array into a list of arguments) let arr1 = [ 1 , - 2 , 3 , 4 ] ; let arr2 = [ 8 , 3 , - 8 , 1 ] ; alert ( Math . max ( ... arr1 , ... arr2 ) ) ; // 8 let arr1 = [ 1 , - 2 , 3 , 4 ] ; let arr2 = [ 8 , 3 , - 8 , 1 ] ; alert ( Math . max ( 1 , ... arr1 , 2 , ... arr2 , 25 ) ) ; // 25 let arr = [ 3 , 5 , 1 ] ; let arr2 = [ 8 , 9 , 15 ] ; let merged = [ 0 , ... arr , 2 , ... arr2 ] ; alert ( merged ) ; // 0,3,5,1,2,8,9,15 (0, then arr, then 2, then arr2) let str = "Hello" ; alert ( [ ... str ] ) ; // H,e,l,l,o let str = "Hello" ; // Array.from converts an iterable into an array alert ( Array . from ( str ) ) ; // H,e,l,l,o let arr = [ 1 , 2 , 3 ] ; let arrCopy = [ ... arr ] ; // spread the array into a list of parameters // then put the result into a new array // do the arrays have the same contents? alert ( JSON . stringify ( arr ) === JSON . stringify ( arrCopy ) ) ; // true // are the arrays equal? alert ( arr === arrCopy ) ; // false (not same reference) // modifying our initial array does not modify the copy: arr . push ( 4 ) ; alert ( arr ) ; // 1, 2, 3, 4 alert ( arrCopy ) ; // 1, 2, 3 let obj = { a : 1 , b : 2 , c : 3 } ; let objCopy = { ... obj } ; // spread the object into a list of parameters // then return the result in a new object // do the objects have the same contents? alert ( JSON . stringify ( obj ) === JSON . stringify ( objCopy ) ) ; // true // are the objects equal? alert ( obj === objCopy ) ; // false (not same reference) // modifying our initial object does not modify the copy: obj . d = 4 ; alert ( JSON . stringify ( obj ) ) ; // {"a":1,"b":2,"c":3,"d":4} alert ( JSON . stringify ( objCopy ) ) ; // {"a":1,"b":2,"c":3} If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting. If you can't understand something in the article – please elaborate. To insert few words of code, use the tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …) We want to make this open-source project available for people all around the world. Help to translate the content of this tutorial to your language! Many JavaScript built-in functions support an arbitrary number of arguments. In this chapter we’ll learn how to do the same. And also, how to pass arrays to such functions as parameters. A function can be called with any number of arguments, no matter how it is defined. There will be no error because of “excessive” arguments. But of course in the result only the first two will be counted. The rest of the parameters can be included in the function definition by using three dots ... followed by the name of the array that will contain them. The dots literally mean “gather the remaining parameters into an array”. For instance, to gather all arguments into array args : We can choose to get the first parameters as variables, and gather only the rest. Here the first two arguments go into variables and the rest go into titles array: The rest parameters gather all remaining arguments, so the following does not make sense and causes an error: There is also a special array-like object named arguments that contains all arguments by their index. In old times, rest parameters did not exist in the language, and using arguments was the only way to get all arguments of the function. And it still works, we can find it in the old code. But the downside is that although arguments is both array-like and iterable, it’s not an array. It does not support array methods, so we can’t call arguments.map(...) for example. Also, it always contains all arguments. We can’t capture them partially, like we did with rest parameters. So when we need these features, then rest parameters are preferred. If we access the arguments object from an arrow function, it takes them from the outer “normal” function. As we remember, arrow functions don’t have their own this . Now we know they don’t have the special arguments object either. We’ve just seen how to get an array from the list of parameters. But sometimes we need to do exactly the reverse. For instance, there’s a built-in function Math.max that returns the greatest number from a list: Now let’s say we have an array [3, 5, 1] . How do we call Math.max with it? Passing it “as is” won’t work, because Math.max expects a list of numeric arguments, not a single array: And surely we can’t manually list items in the code Math.max(arr[0], arr[1], arr[2]) , because we may be unsure how many there are. As our script executes, there could be a lot, or there could be none. And that would get ugly. Spread syntax to the rescue! It looks similar to rest parameters, also using ... , but does quite the opposite. When ...arr is used in the function call, it “expands” an iterable object arr into the list of arguments. We also can pass multiple iterables this way: We can even combine the spread syntax with normal values: Also, the spread syntax can be used to merge arrays: In the examples above we used an array to demonstrate the spread syntax, but any iterable will do. For instance, here we use the spread syntax to turn the string into array of characters: The spread syntax internally uses iterators to gather elements, the same way as for..of does. So, for a string, for..of returns characters and ...str becomes "H","e","l","l","o" . The list of characters is passed to array initializer [...str] . For this particular task we could also use Array.from , because it converts an iterable (like a string) into an array: The result is the same as [...str] . But there’s a subtle difference between Array.from(obj) and [...obj] : So, for the task of turning something into an array, Array.from tends to be more universal. Remember when we talked about Object.assign() in the past ? It is possible to do the same thing with the spread syntax. Note that it is possible to do the same thing to make a copy of an object: This way of copying an object is much shorter than let objCopy = Object.assign({}, obj) or for an array let arrCopy = Object.assign([], arr) so we prefer to use it whenever we can. When we see "..." in the code, it is either rest parameters or the spread syntax. There’s an easy way to distinguish between them: Together they help to travel between a list and an array of parameters with ease. All arguments of a function call are also available in “old-style” arguments : array-like iterable object. myFunction ( a , ... iterableObj , b ) [ 1 , ... iterableObj , '4' , 'five' , 6 ] { ... obj , key : 'value' } const obj = { key1 : 'value1' } ; const array = [ ... obj ] ; // TypeError: obj is not iterable const array = [ 1 , 2 , 3 ] ; const obj = { ... array } ; // { 0: 1, 1: 2, 2: 3 } function myFunction ( x , y , z ) { } const args = [ 0 , 1 , 2 ] ; myFunction . apply ( null , args ) ; function myFunction ( x , y , z ) { } const args = [ 0 , 1 , 2 ] ; myFunction ( ... args ) ; function myFunction ( v , w , x , y , z ) { } const args = [ 0 , 1 ] ; myFunction ( - 1 , ... args , 2 , ... [ 3 ] ) ; const dateFields = [ 1970 , 0 , 1 ] ; // 1 Jan 1970 const d = new Date ( ... dateFields ) ; const parts = [ 'shoulders' , 'knees' ] ; const lyrics = [ 'head' , ... parts , 'and' , 'toes' ] ; // ["head", "shoulders", "knees", "and", "toes"] const arr = [ 1 , 2 , 3 ] ; const arr2 = [ ... arr ] ; // like arr.slice() arr2 . push ( 4 ) ; // arr2 becomes [1, 2, 3, 4] // arr remains unaffected const a = [ [ 1 ] , [ 2 ] , [ 3 ] ] ; const b = [ ... a ] ; b . shift ( ) . shift ( ) ; // 1 // Oh no! Now array 'a' is affected as well: a // [[], [2], [3]] let arr1 = [ 0 , 1 , 2 ] ; const arr2 = [ 3 , 4 , 5 ] ; // Append all items from arr2 onto arr1 arr1 = arr1 . concat ( arr2 ) ; let arr1 = [ 0 , 1 , 2 ] ; const arr2 = [ 3 , 4 , 5 ] ; arr1 = [ ... arr1 , ... arr2 ] ; // arr1 is now [0, 1, 2, 3, 4, 5] const arr1 = [ 0 , 1 , 2 ] ; const arr2 = [ 3 , 4 , 5 ] ; // Prepend all items from arr2 onto arr1 Array . prototype . unshift . apply ( arr1 , arr2 ) ; // arr1 is now [3, 4, 5, 0, 1, 2] let arr1 = [ 0 , 1 , 2 ] ; const arr2 = [ 3 , 4 , 5 ] ; arr1 = [ ... arr2 , ... arr1 ] ; // arr1 is now [3, 4, 5, 0, 1, 2] const obj1 = { foo : 'bar' , x : 42 } ; const obj2 = { foo : 'baz' , y : 13 } ; const clonedObj = { ... obj1 } ; // Object { foo: "bar", x: 42 } const mergedObj = { ... obj1 , ... obj2 } ; // Object { foo: "baz", x: 42, y: 13 } const obj1 = { foo : 'bar' , x : 42 } ; Object . assign ( obj1 , { x : 1337 } ) ; console . log ( obj1 ) ; // Object { foo: "bar", x: 1337 } const objectAssign = Object . assign ( { set foo ( val ) { console . log ( val ) ; } } , { foo : 1 } ) ; // Logs "1"; objectAssign.foo is still the original setter const spread = { set foo ( val ) { console . log ( val ) ; } , ... { foo : 1 } } ; // Nothing is logged; spread.foo is 1 const obj1 = { foo : 'bar' , x : 42 } ; const obj2 = { foo : 'baz' , y : 13 } ; const merge = ( ... objects ) => ( { ... objects } ) ; const mergedObj1 = merge ( obj1 , obj2 ) ; // Object { 0: { foo: 'bar', x: 42 }, 1: { foo: 'baz', y: 13 } } const mergedObj2 = merge ( { } , obj1 , obj2 ) ; // Object { 0: {}, 1: { foo: 'bar', x: 42 }, 2: { foo: 'baz', y: 13 } } const obj1 = { foo : 'bar' , x : 42 } ; const obj2 = { foo : 'baz' , y : 13 } ; const merge = ( ... objects ) => objects . reduce ( ( acc , cur ) => ( { ... acc , ... cur } ) ) ; const mergedObj1 = merge ( obj1 , obj2 ) ; // Object { foo: 'baz', x: 42, y: 13 } Web technology reference for developers Code used to describe document style Protocol for transmitting web resources Interfaces for building web applications Developing extensions for web browsers Web technology reference for developers Learn to structure web content with HTML Learn to run scripts in the browser Learn to make the web accessible to all Frequently asked questions about MDN Plus Spread syntax ( ... ) allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created. Spread syntax looks exactly like rest syntax. In a way, spread syntax is the opposite of rest syntax. Spread syntax "expands" an array into its elements, while rest syntax collects multiple elements and "condenses" them into a single element. See rest parameters and rest property . Spread syntax can be used when all elements from an object or array need to be included in a new array or object, or should be applied one-by-one in a function call's arguments list. There are three distinct places that accept the spread syntax: Although the syntax looks the same, they come with slightly different semantics. Only iterable objects, like Array , can be spread in array and function parameters. Many objects are not iterable, including all plain objects that lack a Symbol.iterator method: On the other hand, spreading in object literals enumerates the own properties of the object. For typical arrays, all indices are enumerable own properties, so arrays can be spread into objects. When using spread syntax for function calls, be aware of the possibility of exceeding the JavaScript engine's argument length limit. See Function.prototype.apply() for more details. It is common to use Function.prototype.apply() in cases where you want to use the elements of an array as arguments to a function. With spread syntax the above can be written as: Any argument in the argument list can use spread syntax, and the spread syntax can be used multiple times. When calling a constructor with new , it's not possible to directly use an array and apply() , because apply() calls the target function instead of constructing it, which means, among other things, that new.target will be undefined . However, an array can be easily used with new thanks to spread syntax: Without spread syntax, to create a new array using an existing array as one part of it, the array literal syntax is no longer sufficient and imperative code must be used instead using a combination of push() , splice() , concat() , etc. With spread syntax this becomes much more succinct: Just like spread for argument lists, ... can be used anywhere in the array literal, and may be used more than once. Note: Spread syntax effectively goes one level deep while copying an array. Therefore, it may be unsuitable for copying multidimensional arrays. The same is true with Object.assign() — no native operation in JavaScript does a deep clone. Array.prototype.concat() is often used to concatenate an array to the end of an existing array. Without spread syntax, this is done as: Array.prototype.unshift() is often used to insert an array of values at the start of an existing array. Without spread syntax, this is done as: Note: Unlike unshift() , this creates a new arr1 , instead of modifying the original arr1 array in-place. Shallow-cloning (excluding prototype) or merging of objects is possible using a shorter syntax than Object.assign() . Note that Object.assign() can be used to mutate an object, whereas spread syntax can't. In addition, Object.assign() triggers setters on the target object, whereas spread syntax does not. You cannot naively re-implement the Object.assign() function through a single spreading: In the above example, the spread syntax does not work as one might expect: it spreads an array of arguments into the object literal, due to the rest parameter. Here is an implementation of merge using the spread syntax, whose behavior is similar to Object.assign() , except that it doesn't trigger setters, nor mutates any object: BCD tables only load in the browser Last modified: Aug 16, 2022 , by MDN contributors Your blueprint for a better internet. Visit Mozilla Corporation’s not-for-profit parent, the Mozilla Foundation . Portions of this content are ©1998– 2022 by individual mozilla.org contributors. Content available under a Creative Commons license . Was this page helpful? Yes No Performance & security by Cloudflare You cannot access medium.com. Refresh the page or contact the site owner to request access. Copy and paste the Ray ID when you contact the site owner. Ray ID: 7471ad406b7a9d63 7471ad406b7a9d63 Copy For help visit Troubleshooting guide Was this page helpful? Yes No Performance & security by Cloudflare You cannot access medium.com. Refresh the page or contact the site owner to request access. Copy and paste the Ray ID when you contact the site owner. Ray ID: 7471ada3b8249dae 7471ada3b8249dae Copy For help visit Troubleshooting guide Spread Betting Companies Uk Lolita Angel Nudist Fkk Naked
tag, for several lines – wrap them in