Spread Php
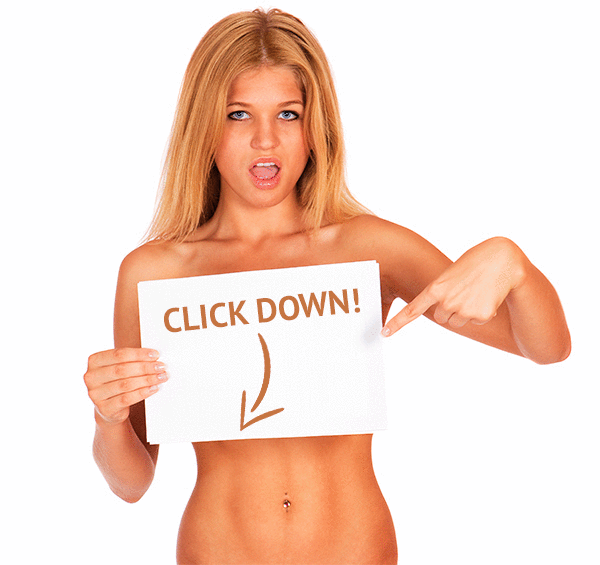
🛑 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Spread Php
Array ( [ 0 ] => 1 [ 1 ] => 2 [ 2 ] => 3 [ 3 ] => 4 [ 4 ] => 5 )
Array ( [ 0 ] => 1 [ 1 ] => 2 [ 2 ] => 3 [ 3 ] => 4 )
Array ( [ 0 ] => 1 [ 1 ] => 2 [ 2 ] => 3 [ 3 ] => 4 )
Array ( [ 0 ] => 1 [ 1 ] => 2 [ 2 ] => 3 [ 3 ] => 2 [ 4 ] => 4 [ 5 ] => 6 )
Array ( [ 0 ] => 47 [ 1 ] => 78 [ 2 ] => 83 [ 3 ] => 13 [ 4 ] => 32 )
Array ( [ 0 ] => 2 [ 1 ] => 4 [ 2 ] => 6 [ 3 ] => 8 )
Array ( [ 0 ] => red [ 1 ] => green [ 2 ] => blue )
by python-tutorials.in on 25th August 2022
Summary : in this tutorial, you will learn about the PHP spread operator and how to use it effectively, like merging multiple arrays into a single one.
PHP 7.4 introduced the spread operator to the array expression. PHP uses the three dots (... ) to denote the spread operator.
When you prefix an array with the spread operator, PHP will spread array elements in place:
$numbers = [ 4 , 5 ]; $scores = [ 1 , 2 , 3 , ...$numbers];
The spread operator offers better performance than the array_merge() function because it is a language construct whereas the array_merge() is a function call. Also, PHP optimizes the performance for constant arrays at compile time.
Unlike argument unpacking, you can use the spread operator anywhere. For example, you can use the spread operator at the beginning of the array:
$numbers = [ 1 , 2 ]; $scores = [...$numbers, 3 , 4 ];
Or you can use the spread operator in the middle of an array like this:
$numbers = [ 2 , 3 ]; $scores = [ 1 , ...$numbers, 4 ];
PHP allows you to use the spread operator multiple times. For example:
$even = [ 2 , 4 , 6 ]; $odd = [ 1 , 2 , 3 ]; $all = [...$odd, ...$even];
The following example uses the spread operator with a return value of a function:
function get_random_numbers () { for ($i = 0 ; $i < 5 ; $i++) { $random_numbers[] = rand( 1 , 100 ); } return $random_numbers; }
$random_numbers = [...get_random_numbers()];
Note that you’ll likely see a different output because of the rand() function.
In the following example, first, we define a generator that returns even numbers between 2 and 10. Then, we use the spread operator to spread out the returned value of the generator into an array:
function even_number () { for ($i = 2 ; $i < 10 ; $i+= 2 ){ yield $i; } }
PHP allows you to apply the spread operator not only to an array but also to any object that implements the Traversable interface. For example:
class RGB implements IteratorAggregate { private $colors = [ 'red' , 'green' , 'blue' ];
public function getIterator () { return new ArrayIterator( $this ->colors); } }
$rgb = new RGB(); $colors = [...$rgb];
PHP 8 allows you to call a function using named arguments. For example:
function format_name (string $first, string $middle, string $last) : string { return $middle ? "$first $middle $last" : "$first $last" ; }
echo format_name( first: 'John' , middle: 'V.' , last: 'Doe' ); // John V. Doe
Also, you can pass the arguments to the format_name function using the spread operator:
function format_name (string $first, string $middle, string $last) : string { return $middle ? "$first $middle $last" : "$first $last" ; }
$names = [ 'first' => 'John' , 'middle' => 'V.' , 'last' => 'Doe' ];
echo format_name(...$names); // John V. Doe
In this case, the keys of the array elements correspond to parameter names of the format_name() function.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
© 2022 python tutorials. python-tutorials.in
Come write articles for us and get featured
Learn and code with the best industry experts
Get access to ad-free content, doubt assistance and more!
Come and find your dream job with us
Difficulty Level :
Easy Last Updated :
04 Oct, 2021
// require_once('vendor/autoload.php');
use PhpOffice\PhpSpreadsheet\Spreadsheet;
use PhpOffice\PhpSpreadsheet\Writer\Xlsx;
$spreadsheet = new Spreadsheet();
// Retrieve the current active worksheet
$sheet = $spreadsheet ->getActiveSheet();
$sheet ->setCellValue( 'A1' , 'GeeksForGeeks!' );
$sheet ->setCellValue( 'B1' , 'A Computer Science Portal For Geeks' );
$writer = new Xlsx( $spreadsheet );
// Save .xlsx file to the current directory
// require_once('path/vendor/autoload.php');
$spreadsheet = \PhpOffice\PhpSpreadsheet\IOFactory::load( 'gfg.xlsx' );
// Store data from the activeSheet to the variable
$data = array (1, $spreadsheet ->getActiveSheet()
->toArray(null,true,true,true));
PHP | Spreadsheet | Setting a cell value by coordinate
PHP | Spreadsheet | Setting a date and/or time value in a cell
How to create SpreadSheet in ReactJS ?
How to add Downloadable Spreadsheet in Next.js ?
How to Convert HTML Table into Excel Spreadsheet using jQuery ?
PHP | Get PHP configuration information using phpinfo()
How to import config.php file in a PHP script ?
How to include content of a PHP file into another PHP file ?
LAMP installation and important PHP configurations on Ubuntu
PHP | imagecreatetruecolor() Function
Maximum execution time taken by a PHP Script
PHP | ImagickDraw getTextAlignment() Function
PHP | Imagick floodFillPaintImage() Function
Function to escape regex patterns before applied in PHP
PHP | array_udiff_uassoc() Function
PHP | Different characters in the given string
PHP | geoip_continent_code_by_name() Function
How to secure database passwords in PHP?
PHP | GmagickPixel setcolor() function
Data Structures & Algorithms- Self Paced Course
Complete Interview Preparation- Self Paced Course
Practice Problems, POTD Streak, Weekly Contests & More!
Improve your Coding Skills with Practice Try It!
A-143, 9th Floor, Sovereign Corporate Tower, Sector-136, Noida, Uttar Pradesh - 201305
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Got It !
Introduction: PHPSpreadsheet is a library written in PHP which helps to read from and write to different types of spreadsheet file formats with the help of a given set of classes. The various format which support spreadsheet are Excel(.xlsx), Open Document Format(.ods),SpreadsheetML(.xml), CSV and many more.
Requirements: The following software is developed using PHPSpreadsheet:
Installation: The PHPSpreadsheet can be installed with the help of Composer
On Terminal: The following command runs on the terminal to install PHPSpreadsheet:
Writing code in comment?
Please use ide.geeksforgeeks.org ,
generate link and share the link here.
Spread operator should have a better performance than array_merge . It's because not only that spread operator is a language structure while array_merge is a function call, but also compile time optimization can be performant for constant arrays.
array_merge only supports array, while spread operator also supports objects implementing Traversable .
a link to the PHP manual entry for the feature
a link to the language specification section (if any)
rfc/spread_operator_for_array.txt · Last modified: 2019/05/13 12:45 by nikic
Show pagesource Old revisions Backlinks Back to top
PHP has already supported argument unpacking (AKA spread operator) since 5.6. This RFC proposes to bring this feature to array expression.
An array pair prefixed by ... will be expanded in places during array definition. Only arrays and objects who implement Traversable can be expanded.
It's possible to do the expansion multiple times, and unlike argument unpacking, ... can be used anywhere. It's possible to add normal elements before or after the spread operator.
Spread operator works for both array syntax( array() ) and short syntax( [] ).
It's also possible to unpack array returned by a function immediately.
In order to make the behavior consistent with argument unpacking , string keys are not supported. A recoverable error will be thrown once a string key is encountered.
It's not possible to unpack an array by reference.
However, if elements in the array to be unpacked are stored by reference, they will be stored by reference in the new array as well.
This change should not break anything.
This is kind of out of scope here to discuss other concat / merge operation. The important thing is we should make the behavior of same operator consistent and not to confuse userland developer. It's also why I changed the behavior for string keys in this revised version.
Some changes in opcache to support the new opcode is needed.
Voting started 2019-04-22 and ends 2019-05-06. A 2/3 majority is required.
After the project is implemented, this section should contain
Links to external references, discussions or RFCs
Keep this updated with features that were discussed on the mail lists.
On October 9, Juliette Reinders Folmer announced on the core WordPress blog that WordPress 5.3 will use the spread operator . The spread operator was one of the new features made available in PHP 5.6, a version released in 2014.
WordPress abandoned PHP 5.2 – 5.5 with the release of WordPress 5.2. This means the core team can start taking advantage of relatively new features, or at least 5-year-old features. For plugin and theme developers who maintain the same minimum version support as WordPress, they can also start exploring this feature.
PHP 5.6 introduced two new methods of using the spread operator:
This feature shouldn’t be confused with unpacking inside of arrays, which is only available in PHP 7.4 .
The change in WordPress 5.3 is not expected to affect themes and plugins except in the rare case that a developer is overloading the wpdb::prepare() method. Developers should read the announcement post to dive into what code has changed in core WordPress.
Developers should check their plugins and themes with debugging enabled in a test environment to check for any notices. There may be cases where the function signature doesn’t match.
The spread operator is a tool, and like any tool, it should be used when it makes sense. Because it is a language construct, it does offer speed improvements over traditional methods of using a PHP function.
The remainder of this post will dive into the using the spread operator to help teach WordPress developers how it works.
Variadic functions are PHP functions that accept a variable number of arguments passed in. They have existed for years. However, they can be confusing without solid inline documentation from the developer who wrote the code.
In the past, developers would need to use the func_get_args() , func_get_arg() , or func_num_args() functions to work with variadic functions. In PHP 5.6, developers can use a parameter such as ...$var_name to represent a variable number of parameters.
Take a look at the following multiplication function. It will accept one, two, three, or even more numbers and multiply each.
If we use that function as shown below, it will display 1024 :
This is simple to do with the spread operator.
PHP 5.6 allows developers to unpack arrays and traversable objects as function arguments. To explain how this works, look at the following multiplication function for multiplying three numbers together.
Generally, you would need to manually pass the $x , $y , and $z parameters directly. However, there are cases in real-world projects where the data (numbers in this case) would already exist within an array such as:
Prior to PHP 5.6, you would need to split that array and pass each value to the function as shown in the following snippet.
With PHP 5.6, you can simply pass in ...$numbers like so:
Both methods work and will output 162 . However, the second method is easier to read and is less prone to typos because it uses fewer characters.
For a more practical example, let’s compare a real-world code change in WordPress and how using the spread operator improves the code over other methods. We can do this by looking at the core current_user_can() function.
First, see how the code is written in WordPress 5.2 and earlier.
Without looking at the full function, most developers would assume that $capability is the only accepted parameter for this function. However, the function accepts a variable number of parameters. Previously, WordPress had to use func_get_args() to get all the parameters, slice the array, and merge everything back together.
It is inelegant coding, but it got the job done for old versions of PHP.
Now compare what the same function looks like in WordPress 5.3. First, you can see the ...$args parameter clearly in the function statement. You can also see there is no need for the clever coding to pass along a variable number of arguments.
The change in WordPress 5.3 is a massive improvement in readability in comparison to earlier versions. It is nice to see these types of improvements to the core code.
Some great news in one package.. Let’s see how it works :)
Enter your email address to subscribe to this blog and receive notifications of new posts by email.
WordPress Tavern is a website about all things WordPress. We cover news and events, write plugin and theme reviews, and talk about key issues within the WordPress ecosystem…
© All Rights Reserved. Powered by WordPress , hosted by Pressable
Photo Porno Girl Masturbation Solo Nylon Milf
Secretary Medical Undressed
Big Ass Natural