Ruedunu
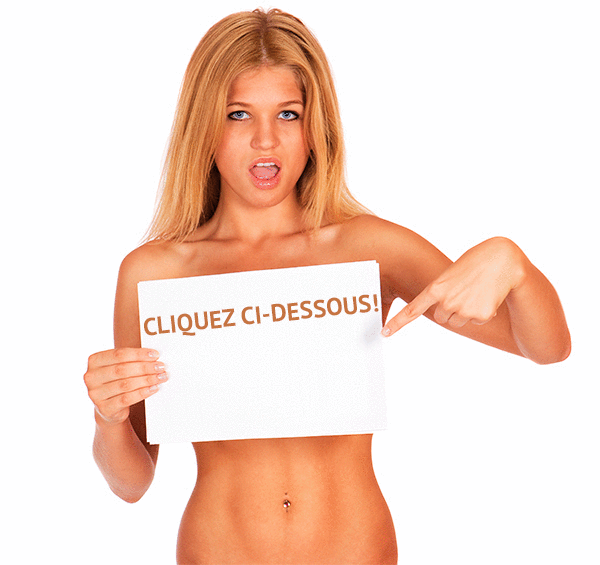
🛑 TOUTES LES INFORMATIONS CLIQUEZ ICI 👈🏻👈🏻👈🏻
Ruedunu
React redux is an advanced state management library for React. As we learned earlier, React only supports component level state management. In a big and complex application, large number of components are used. React recommends to move the state to the top level component and pass the state to the nested component using properties. It helps to some extent but it becomes complex when the components increases.
React redux chips in and helps to maintain state at the application level. React redux allows any component to access the state at any time. Also, it allows any component to change the state of the application at any time.
Let us learn about the how to write a React application using React redux in this chapter.
React redux maintains the state of the application in a single place called Redux store. React component can get the latest state from the store as well as change the state at any time. Redux provides a simple process to get and set the current state of the application and involves below concepts.
Store − The central place to store the state of the application.
Actions − Action is an plain object with the type of the action to be done and the input (called payload) necessary to do the action. For example, action for adding an item in the store contains ADD_ITEM as type and an object with item’s details as payload. The action can be represented as −
Reducers − Reducers are pure functions used to create a new state based on the existing state and the current action. It returns the newly created state. For example, in add item scenario, it creates a new item list and merges the item from the state and new item and returns the newly created list.
Action creators − Action creator creates an action with proper action type and data necessary for the action and returns the action. For example, addItem action creator returns below object −
Component − Component can connect to the store to get the current state and dispatch action to the store so that the store executes the action and updates it’s current state.
The workflow of a typical redux store can be represented as shown below.
Redux provides a single api, connect which will connect a components to the store and allows the component to get and set the state of the store.
The signature of the connect API is −
All parameters are optional and it returns a HOC (higher order component). A higher order component is a function which wraps a component and returns a new component.
Let us see the first two parameters which will be enough for most cases.
mapStateToProps − Accepts a function with below signature.
Here, state refers current state of the store and Object refers the new props of the component. It gets called whenever the state of the store is updated.
mapDispatchToProps − Accepts a function with below signature.
Here, dispatch refers the dispatch object used to dispatch action in the redux store and Object refers one or more dispatch functions as props of the component.
React Redux provides a Provider component and its sole purpose to make the Redux store available to its all nested components connected to store using connect API. The sample code is given below −
Now, all the component inside the App component can get access to the Redux store by using connect API.
Let us recreate our expense manager application and uses the React redux concept to maintain the state of the application.
First, create a new react application, react-message-app using Create React App or Rollup bundler by following instruction in Creating a React application chapter.
Next, install Redux and React redux library.
Next, install uuid library to generate unique identifier for new expenses.
Next, open the application in your favorite editor.
Next, create src folder under the root directory of the application.
Next, create actions folder under src folder.
Next, create a file, types.js under src/actions folder and start editing.
Next, add two action type, one for add expense and one for remove expense.
Next, create a file, index.js under src/actions folder to add action and start editing.
Next, import uuid to create unique identifier.
Next, add a new function to return action type for adding an expense and export it.
Here, the function expects expense object and return action type of ADD_EXPENSE along with a payload of expense information.
Next, add a new function to return action type for deleting an expense and export it.
Here, the function expects id of the expense item to be deleted and return action type of ‘DELETE_EXPENSE’ along with a payload of expense id.
The complete source code of the action is given below −
Next, create a new folder, reducers under src folder.
Next, create a file, index.js under src/reducers to write reducer function and start editing.
Next, add a function, expensesReducer to do the actual feature of adding and updating expenses in the redux store.
The complete source code of the reducer is given below −
Here, the reducer checks the action type and execute the relevant code.
Next, create components folder under src folder.
Next, create a file, ExpenseEntryItemList.css under src/components folder and add generic style for the html tables.
Next, create a file, ExpenseEntryItemList.js under src/components folder and start editing.
Next, import React and React redux library.
Next, import ExpenseEntryItemList.css file.
Next, create a class, ExpenseEntryItemList and call constructor with props .
Next, create mapStateToProps function.
Here, we copied the input state to expenses props of the component.
Next, create mapDispatchToProps function.
Here, we created two function, one to dispatch add expense (addExpense) function and another to dispatch delete expense (deleteExpense) function and mapped those function to props of the component.
Next, export the component using connect api.
Now, the component gets three new properties given below −
onAddExpense − function to dispatch addExpense function
onDelete − function to dispatch deleteExpense function
Next, add few expense into the redux store in the constructor using onAddExpense property.
Next, add an event handler to delete the expense item using expense id.
Here, the event handler calls the onDelete dispatcher, which call deleteExpense along with the expense id.
Next, add a method to calculate the total amount of all expenses.
Next, add render() method and list the expense item in the tabular format.
Here, we set the event handler handleDelete to remove the expense from the store.
The complete source code of the ExpenseEntryItemList component is given below −
Next, create a file, App.js under the src/components folder and use ExpenseEntryItemList component.
Next, create a file, index.js under src folder.
Create a store using createStore by attaching the our reducer.
Used Provider component from React redux library and set the store as props, which enables all the nested component to connect to store using connect api.
Finally, create a public folder under the root folder and create index.html file.
Next, serve the application using npm command.
Next, open the browser and enter http://localhost:3000 in the address bar and press enter.
Clicking the remove link will remove the item from redux store.
© Copyright 2022. All Rights Reserved.
We make use of First and third party cookies to improve our user experience. By using this website, you agree with our Cookies Policy.
Agree
Learn more
This can take up to 60 seconds. Please wait...
• M.ruedux.com resolves to the IP addresses 87.98.135.175 .
• M.ruedux.com has servers located in France .
Title: Ruedux.com - Page d'accueil - Tube *** *** Videos et film de *** en streaming gratuit
This can take up to 60 seconds. Please wait...
*HypeStat.com is not linking to, promoting or affiliated with ruedux.com in any way. Only publicly available statistics data are displayed.
Summary of the ad experience rating of a site for a specific platform.
Time to Interactive 0.4 s
Time to interactive is the amount of time it takes for the page to become fully interactive. Learn more.
Estimated Input Latency 10 ms
Estimated Input Latency is an estimate of how long your app takes to respond to user input, in milliseconds, during the busiest 5s window of page load. If your latency is higher than 50 ms, users may perceive your app as laggy. Learn more.
Total Blocking Time 0 ms
Sum of all time periods between FCP and Time to Interactive, when task length exceeded 50ms, expressed in milliseconds.
Speed Index 0.4 s
Speed Index shows how quickly the contents of a page are visibly populated. Learn more.
First CPU Idle 0.4 s
First CPU Idle marks the first time at which the page's main thread is quiet enough to handle input. Learn more.
First Contentful Paint 0.4 s
First Contentful Paint marks the time at which the first text or image is painted. Learn more.
Performance budget
Keep the quantity and size of network requests under the targets set by the provided performance budget. Learn more.
First Meaningful Paint 0.4 s
First Meaningful Paint measures when the primary content of a page is visible. Learn more.
Max Potential First Input Delay 40 ms
The maximum potential First Input Delay that your users could experience is the duration, in milliseconds, of the longest task. Learn more.
First Contentful Paint (FCP) 916ms
0% of loads for this page have a fast (<1s) First Contentful Paint (FCP)
78%
17% of loads for this page have an average (1s ~ 3s) First Contentful Paint (FCP)
17%
3% of loads for this page have a slow (>3s) First Contentful Paint (FCP)
3%
First Input Delay (FID) 105ms
94% of loads for this page have a fast (<100ms) First Input Delay (FID)
94%
3% of loads for this page have an average (100ms ~ 300ms) First Input Delay (FID)
3%
1% of loads for this page have a slow (>300ms) First Input Delay (FID)
1%
First Contentful Paint (FCP) 670ms
90% of loads for this page have a fast (<1s) First Contentful Paint (FCP)
90%
7% of loads for this page have an average (1s ~ 3s) First Contentful Paint (FCP)
7%
1% of loads for this page have a slow (>3s) First Contentful Paint (FCP)
1%
First Input Delay (FID) 58ms
0% of loads for this page have a fast (<100ms) First Input Delay (FID)
98%
0% of loads for this page have an average 100ms ~ 300ms) First Input Delay (FID)
0%
0% of loads for this page have a slow (>300ms) First Input Delay (FID)
0%
Performance budget
Keep the quantity and size of network requests under the targets set by the provided performance budget. Learn more.
First Meaningful Paint 1.2 s
First Meaningful Paint measures when the primary content of a page is visible. Learn more.
Max Potential First Input Delay 110 ms
The maximum potential First Input Delay that your users could experience is the duration, in milliseconds, of the longest task. Learn more.
Time to Interactive 5.5 s
Time to interactive is the amount of time it takes for the page to become fully interactive. Learn more.
First Contentful Paint (3G) 2531 ms
First Contentful Paint 3G marks the time at which the first text or image is painted while on a 3G network. Learn more.
Estimated Input Latency 10 ms
Estimated Input Latency is an estimate of how long your app takes to respond to user input, in milliseconds, during the busiest 5s window of page load. If your latency is higher than 50 ms, users may perceive your app as laggy. Learn more.
Total Blocking Time 40 ms
Sum of all time periods between FCP and Time to Interactive, when task length exceeded 50ms, expressed in milliseconds.
Speed Index 1.8 s
Speed Index shows how quickly the contents of a page are visibly populated. Learn more.
First CPU Idle 2.9 s
First CPU Idle marks the first time at which the page's main thread is quiet enough to handle input. Learn more.
First Contentful Paint 1.2 s
First Contentful Paint marks the time at which the first text or image is painted. Learn more.
Original size: 130.22 KB
Compressed size: 40.83 KB
File reduced by: 89.39 KB (68%)
m.ruedux.com does not support HTTPS
Verifying SSL Support. Please wait...
m.ruedux.com does not support HTTP/2
Verifying HTTP/2.0 Support. Please wait...
Date: Wed, 01 Jan 2020 23:09:10 GMT
Server: Apache/2.2.22 (Debian)
X-Powered-By: PHP/5.4.45-0+deb7u5
Set-Cookie: PHPSESSID=gjpr49nbne0avd1mejac33op22; path=/
Expires: Thu, 19 Nov 1981 08:52:00 GMT
Cache-Control: no-store, no-cache, must-revalidate, post-check=0, pre-check=0
Pragma: no-cache
Set-Cookie: disclaimer2=tata; expires=Thu, 02-Jan-2020 23:09:10 GMT; path=/
Vary: Accept-Encoding
Content-Encoding: gzip
Content-Length: 41805
Content-Type: text/html
This can take up to 60 seconds. Please wait...
*HypeStat.com is not linking to, promoting or affiliated with ruedux.com in any way. Only publicly available statistics data are displayed.
• Use Show/Hide ESTIMATED data form to hide (Website worth, Daily ads revenue, Daily Visits, Daily Pageviews)
• Use Show/Hide WHOIS data form to hide whois data
• Use Remove form to remove all data
• If you have any problem with REMOVE/HIDE your data just drop an email at support (at) hypestat.com and we will remove/hide your site data manualy.
Make custom Widget for your website Get the code now!
Build Your PC
Reviews
Best Sellers
Financing
Support
About Us
Build Your PC
Reviews
Best Sellers
Financing
Support
About Us
Best Gaming PCs Optimized for your budget.
START BUILD
BEST SELLERS
CONTINUE BUILD
*Example payment is based on the listed product price(s) assuming a 36 month term loan and a 9.99% APR. Subject to approval of credit application. Rates range from 0.00% to 29.99% APR. APRs will vary depending on credit qualifications, loan amount, and term. Bread® pay-over-time plans are loans made by Comenity Capital Bank. Get My Rate
*Example payment is based on the listed product price(s) assuming a 36 month term loan and a 9.99% APR. Subject to approval of credit application. Rates range from 0.00% to 29.99% APR. APRs will vary depending on credit qualifications, loan amount, and term. Bread® pay-over-time plans are loans made by Comenity Capital Bank. Get My Rate
*Example payment is based on the listed product price(s) assuming a 36 month term loan and a 9.99% APR. Subject to approval of credit application. Rates range from 0.00% to 29.99% APR. APRs will vary depending on credit qualifications, loan amount, and term. Bread® pay-over-time plans are loans made by Comenity Capital Bank. Get My Rate
*Example payment is based on the listed product price(s) assuming a 36 month term loan and a 9.99% APR. Subject to approval of credit application. Rates range from 0.00% to 29.99% APR. APRs will vary depending on credit qualifications, loan amount, and term. Bread® pay-over-time plans are loans made by Comenity Capital Bank. Get My Rate
FOLLOW THE JOURNEY @buildredux
Facebook
Twitter
Instagram
Are white PCs still popular? Maybe we should start carrying it. Hmmm 🤔
#pcgaming #pcbuilds #gamingpc #pcmr #gamingsetup
#pcmasterrace #custompcbuilds #pcbuildsetup #pcgamer
#gamers #rgb #gaming #battlerig #nvidiageforce
#custompc #gamingrig #gamersetup #gamerpc #setupwars
#rgblights #pcparts #battlestation #pcgamingsetup #whitepcbuild
#whitepcsetup #allwhitepcbuild
How awesome will Spider-Man Remastered look on PC? 😍
#pcgaming #pcbuilds #gamingpc #pcmr #gamingsetup
#pcmasterrace #custompcbuilds #pcbuildsetup #pcgamer
#gamers #rgb #gaming #battlerig #nvidiageforce #rtx
#gamingrig #gamersetup #gamerpc #setupwars
#rgblights #pcparts #battlestation #pcgamingsetup #spiderman
#spidermanremastered #ps5
Here's a simple guide on how to select the best GPU for you.
#pctips #pctechtips #gpu #pcparts #pcbuildguide #pcgaming #pcbuilds
#gamingpc #pcmr #gamingsetup
#pcmasterrace #custompcbuilds #pcbuildsetup #pcgamer
#gamers #rgb #gaming #battlerig #nvidiageforce #rtx
#custompc #gamingrig #gamersetup #gamerpc #setupwars
#rgblights #battlestation #pcgamingsetup #pcgp
Les Nichons De Ma Mere
Il Sodomise Sa Belle Soeur
%D9%86%D8%A7%D9%Be%D8%B1%D9%88%Da%A9%D8%B3%D9%86