Public Private Static
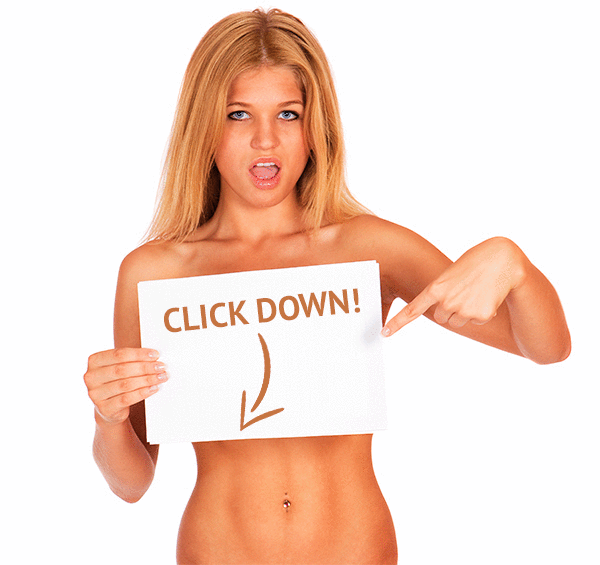
⚡ ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Public Private Static
Table of contents
Exit focus mode
Light
Dark
High contrast
Light
Dark
High contrast
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
A static class is basically the same as a non-static class, but there is one difference: a static class cannot be instantiated. In other words, you cannot use the new operator to create a variable of the class type. Because there is no instance variable, you access the members of a static class by using the class name itself. For example, if you have a static class that is named UtilityClass that has a public static method named MethodA , you call the method as shown in the following example:
A static class can be used as a convenient container for sets of methods that just operate on input parameters and do not have to get or set any internal instance fields. For example, in the .NET Class Library, the static System.Math class contains methods that perform mathematical operations, without any requirement to store or retrieve data that is unique to a particular instance of the Math class. That is, you apply the members of the class by specifying the class name and the method name, as shown in the following example.
As is the case with all class types, the type information for a static class is loaded by the .NET runtime when the program that references the class is loaded. The program cannot specify exactly when the class is loaded. However, it is guaranteed to be loaded and to have its fields initialized and its static constructor called before the class is referenced for the first time in your program. A static constructor is only called one time, and a static class remains in memory for the lifetime of the application domain in which your program resides.
To create a non-static class that allows only one instance of itself to be created, see Implementing Singleton in C# .
The following list provides the main features of a static class:
Creating a static class is therefore basically the same as creating a class that contains only static members and a private constructor. A private constructor prevents the class from being instantiated. The advantage of using a static class is that the compiler can check to make sure that no instance members are accidentally added. The compiler will guarantee that instances of this class cannot be created.
Static classes are sealed and therefore cannot be inherited. They cannot inherit from any class except Object . Static classes cannot contain an instance constructor. However, they can contain a static constructor. Non-static classes should also define a static constructor if the class contains static members that require non-trivial initialization. For more information, see Static Constructors .
Here is an example of a static class that contains two methods that convert temperature from Celsius to Fahrenheit and from Fahrenheit to Celsius:
A non-static class can contain static methods, fields, properties, or events. The static member is callable on a class even when no instance of the class has been created. The static member is always accessed by the class name, not the instance name. Only one copy of a static member exists, regardless of how many instances of the class are created. Static methods and properties cannot access non-static fields and events in their containing type, and they cannot access an instance variable of any object unless it's explicitly passed in a method parameter.
It is more typical to declare a non-static class with some static members, than to declare an entire class as static. Two common uses of static fields are to keep a count of the number of objects that have been instantiated, or to store a value that must be shared among all instances.
Static methods can be overloaded but not overridden, because they belong to the class, and not to any instance of the class.
Although a field cannot be declared as static const , a const field is essentially static in its behavior. It belongs to the type, not to instances of the type. Therefore, const fields can be accessed by using the same ClassName.MemberName notation that's used for static fields. No object instance is required.
C# does not support static local variables (that is, variables that are declared in method scope).
You declare static class members by using the static keyword before the return type of the member, as shown in the following example:
Static members are initialized before the static member is accessed for the first time and before the static constructor, if there is one, is called. To access a static class member, use the name of the class instead of a variable name to specify the location of the member, as shown in the following example:
If your class contains static fields, provide a static constructor that initializes them when the class is loaded.
A call to a static method generates a call instruction in Microsoft intermediate language (MSIL), whereas a call to an instance method generates a callvirt instruction, which also checks for null object references. However, most of the time the performance difference between the two is not significant.
For more information, see Static classes , Static and instance members and Static constructors in the C# Language Specification . The language specification is the definitive source for C# syntax and usage.
Java-SE1728: Slot 8. Class, Object,...
DelftStack articles are written by software geeks like you. If you also would like to contribute to DelftStack by writing paid articles, you can check the write for us page.
Created: September-12, 2021 | Updated: October-02, 2021
Class variables, commonly known as static variables, are defined using the static keyword in a class but outside a method, constructor (default or parameterized), or block.
Private static variables are frequently utilized for constants. For example, many individuals prefer not to use constants in their code. Instead, they prefer to create a private static variable with a meaningful name and utilize it in their code, making the code more understandable.
If a variable is declared static, then the variable’s value is the same for all the instances, and we don’t need to create an object to call that variable.
In the above example, we created a static private variable and printed its value.
Let us understand an example to see the difference between a private and a private static variable.
The PersonB object changes the eye variable in the above example, but the leg variable remains the same. This is because a private variable copies itself to the method, preserving its original value. But a private static value only has one copy for all methods to share, thus changing its value changes the original value.
Copyright © 2020. All right reserved
Come write articles for us and get featured
Learn and code with the best industry experts
Get access to ad-free content, doubt assistance and more!
Come and find your dream job with us
Understanding “static” in “public static void main” in Java
Difficulty Level :
Easy Last Updated :
28 Oct, 2020
/*package whatever //do not write package name here */
public void main (String[] args) {
System.out.println( "GFG!" );
Understanding storage of static methods and static variables in Java
Java main() Method - public static void main(String[] args)
How to Check the Accessibility of the Static and Non-Static Variables by a Static Method?
Static and non static blank final variables in Java
Difference between static and non-static method in Java
Difference between static and non-static variables in Java
Why non-static variable cannot be referenced from a static method in Java
Class Loading and Static Blocks Execution Using Static Modifier in Java
Java Program to Check the Accessibility of an Static Variable By a Static Method
Difference Between Static and Non Static Nested Class in Java
Internal static variable vs. External static variable with Examples in C
Dangling, Void , Null and Wild Pointers
Understanding OutOfMemoryError Exception in Java
Understanding Classes and Objects in Java
Understanding Object Cloning in Java with Examples
Understanding threads on Producer Consumer Problem | Java
Shadowing of static functions in Java
Are static local variables allowed in Java?
Assigning values to static final variables in Java
Comparison of static keyword in C++ and Java
Can we Overload or Override static methods in java ?
JAVA Programming Foundation- Self Paced Course
Data Structures & Algorithms- Self Paced Course
Complete Interview Preparation- Self Paced Course
Improve your Coding Skills with Practice Try It!
A-143, 9th Floor, Sovereign Corporate Tower, Sector-136, Noida, Uttar Pradesh - 201305
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Got It !
Following points explain what is “static” in the main() method:
Need of static in main() method : Since main() method is the entry point of any Java application, hence making the main() method as static is mandatory due to following reasons:
What if we don’t write “static” before the main method: If we do not write “static” before the main method then, our program will be compiled without any compilation error(s). But at the time of execution, the JVM searches for the main method which is public, static, with a return type and a String array as an argument. If such a method is not found then an error is generated at the run time.
Output: An error message will be shown as follows
Writing code in comment?
Please use ide.geeksforgeeks.org ,
generate link and share the link here.
My Developer Account >
Create Account>
My Settings>
All Developer Centers
Community Cloud
Einstein Analytics
Einstein Platform
Embedded Service SDK for Mobile
Heroku Developer Center
Identity
Integration and APIs
Lightning Apps
Lightning Flow
Marketing Cloud
Mobile Developer Center
Mulesoft Developer Center
Pardot Developer Center
Quip Developer Center
Salesforce DX
Security
Service Cloud
Home » Discussion Forums » What is the difference between static , public and private members of a class,?
Browse by Topic
Apex Code Development (88466)
General Development (54257)
Visualforce Development (36945)
Lightning (16732)
APIs and Integration (16315)
Trailhead (11468)
Formulas & Validation Rules Discussion (10900)
Other Salesforce Applications (7874)
Jobs Board (6626)
Force.com Sites & Site.com (4763)
Mobile (2629)
Java Development (3895)
.NET Development (3504)
Security (3253)
Mobile (2629)
Visual Workflow (2394)
AppExchange Directory & Packaging (2341)
Perl, PHP, Python & Ruby Development (2016)
Chatter and Chatter API Development (1713)
Salesforce Labs & Open Source Projects (1231)
Desktop Integration (1142)
Architecture (957)
Schema Development (928)
Apple, Mac and OS X (791)
VB and Office Development (633)
Einstein Platform (193)
Salesforce $1 Million Hackathon (184)
Salesforce Summer of Hacks (175)
View More Topics
See All Posts
What is the difference between static , public and private members of a class,? when we invoke class from trigger? Can anyone pls explain this with the help of an example. when we invoke class with (static , public, private member) from trigger
Best Answer chosen by Chitral Chadda
Non-static method (and variables) must have a new instance of the class instantiated in order to be used. Typically these rely on data inside the class that then is refered to inside the class
public class Utils {
private lang;
public Utils() {
lang = 'en';
}
public Utils(String lang) {
this.lang = lang;
}
public String getHelloWorld() {
if (lang == 'en') {
return 'Hello World';
} else if (lang == 'es') {
return 'Hola Mundo';
}
// Return esperanto if we don't know the language
return 'Saluton Mondo';
}
}
This version of the method requires us to create a new instance
Utils en_utils = new Utils();
String salutation_en = en_utils.getHelloWorld();
// salutation_en == 'Hello World'
Utils es_utils = new Utils('es');
String salutation_es = es_utils.getHelloWorld();
// salutation_es == 'Hola Mundo'
Utils fr_utils = new Utils('fr');
String salutation_fr = fr_utils.getHelloWorld();
// salutation_fr == 'Saluton Mondo'
In this example, we can see that we have a private class level variable lang that we use inside of getHelloWorld. Because this variable is private, we cannot reference it outside of class level variables. We can mix and match these. For example, we can have a public class level method call a private class level method that then uses / sets both private and public variables. We can only call public variables and methos from outside the class
Non-static method (and variables) must have a new instance of the class instantiated in order to be used. Typically these rely on data inside the class that then is refered to inside the class
public class Utils {
private lang;
public Utils() {
lang = 'en';
}
public Utils(String lang) {
this.lang = lang;
}
public String getHelloWorld() {
if (lang == 'en') {
return 'Hello World';
} else if (lang == 'es') {
return 'Hola Mundo';
}
// Return esperanto if we don't know the language
return 'Saluton Mondo';
}
}
This version of the method requires us to create a new instance
Utils en_utils = new Utils();
String salutation_en = en_utils.getHelloWorld();
// salutation_en == 'Hello World'
Utils es_utils = new Utils('es');
String salutation_es = es_utils.getHelloWorld();
// salutation_es == 'Hola Mundo'
Utils fr_utils = new Utils('fr');
String salutation_fr = fr_utils.getHelloWorld();
// salutation_fr == 'Saluton Mondo'
I
Mature Shares
Busty Blonde Lingerie
Overwatch Mercy Porn Comics