Private Test
⚡ ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Private Test
How to Test Private and Protected methods in .NET
This article explains some theory behind testing/not testing private methods, and then provides and walks through a downloadable code sample to demonstrate these testing techniques.
Tim Stall is a Chicago-based technical consultant for Computer Sciences Corporation (www.csc.com), a leading global IT services company. In addition to his expertise in Microsoft.Net development projects and enterprise architecture, Tim's .Net experience includes, writing technical publications, leading internal training, and having MCAD certification. His blog is at http://timstall.dotne...
You must Sign In to use this message board.
Spacing
Relaxed Compact Tight
Layout
Normal Open Topics Open All Thread View
Per page
10 25 50
Article Copyright 2005 by TimStall Everything else
Copyright © CodeProject , 1999-2021
Web03
2.8.20210202.1
Test Driven Development is the
practice of (1) writing tests, (2) writing code that passes those tests, and (3)
then refactoring. This concept is becoming very popular in the .NET community
due to the quality assurance that it adds. While it is easy to test public
methods, the natural question emerges "How do I test protected and private
methods?"
A Google
search will show you that there's a lot of debate about using private
methods, let alone testing them. The table below summarizes some of the common
views of the pro and con for both issues.
There are bright and experienced people on both sides of the issue. So while
I have no intention or expectation of ending the "should I test private method"
debate, there is still value for both sides to know how to test them. Even if
you think that private methods should not be tested:
Andrew Hunt and David Thomas explain in their book , Pragmatic
Unit Testing in C# with NUnit , that good unit tests are ATRIP:
There are three additional criteria that any testing overhead for
private/protected methods should meet:
Keeping these criteria in mind, there are several strategies that fall
short:
A protected method is visible only to derived classes, therefore it is not
immediately available to a test suite. For example, suppose we wanted to test
the method from ClassLibrary1.MyObject :
The book Pragmatic
Unit Testing in C# with NUnit explains one solution: make a derived class
MyObjectTester that inherits class MyObject , and then
create a public method TestMyProtectedMethod that wraps the
protected one. For example:
This approach is simple, yet meets all the criteria:
Testing private methods is a little more involved; but we can still do it
using System.Reflection .
You can use Reflection to dynamically access methods of a type, including both
instance and static private methods. Note that accessing private methods does
require the ReflectionPermission ,
but that is not a problem for Unit Tests running on a development machine or
build server.
Suppose we wanted to test the private method MyPrivateMethod
from ClassLibrary1.MyObject :
One solution is to create a UnitTestUtilities project with a helper class to
call the test method via reflection. For example, the download solution has the
following methods in UnitTestUtilities.Helper :
Private method RunMethod takes in the necessary parameters that
Reflection needs to invoke a method, and then returns the value. It has two
public methods that wrap this: RunStaticMethod and
RunInstanceMethod for static and instance methods respectively.
Walking through RunMethod , it first gets the
We could use this Utility in an NUnit test like so:
While there is a debate on whether or not to test private methods, at least
we have the ability to do so. We can test protected methods using inheritance to
create a derived TesterClass that wraps the base protected methods
with public ones. We can test private methods using Reflection, which can be
abstracted to a UnitTestUtility helper class. Both of these
techniques can help to improve test coverage.
This article has no explicit license attached to it but may contain usage terms in the article text or the download files themselves. If in doubt please contact the author via the discussion board below.
A list of licenses authors might use can be found here
General News Suggestion Question Bug Answer Joke Praise Rant Admin
Use Ctrl+Left/Right to switch messages, Ctrl+Up/Down to switch threads, Ctrl+Shift+Left/Right to switch pages.
Encapsulation - Private methods provide encapsulation, which makes
the code easier for the end client to use
Refactoring - It is easier to refactor private methods because they
are never directly called by external clients, therefore changing the signature
won't break any method calls.
Validation - Unlike public methods that must validate all input
because they are called by external clients, private methods are called safely
within the class and don't require the same rigor of validation - the inputs
should have been already validated in the public methods.
Test Scope - Exposing every method as public greatly increases the
test scope. Private methods are used only how the developer intended them,
whereas public methods need to be tested for every possible case which required
a broader test scope.
Didn't Refactor - If a class is complicated enough to merit private
members, then it should be refactored.
Hides Functionality - Private methods (if designed correctly) provide
useful features that clients may want access to, so any private method worth
testing should really be public.
Test Control - Private methods can contain complex logic and it
increases test control to be able to directly access the method and test it,
instead of needing to indirectly accessing it through a public method.
Principle - Unit Testing is about testing the smallest functional
piece of code; private methods are functional pieces of code, therefore on
principle private methods should be testable.
Already Covered - Only the public interface should be tested. Private
methods should already have thorough test coverage from being called by the
public methods that are tested.
Brittle Code - If you refactor the code, and juggle around the
private methods, and if you had tests linked to those private methods, then you
need to juggle around your tests too.
Use the directives #if DEBUG ... #endif
to wrap a public method which in turns wraps the private method. The unit tests
can now indirectly access that private method through the public wrapper. (This
is a method that I myself have used many times, and found it to be tedious and
non-object oriented).
This only works in Debug mode.
This is procedural and not object-oriented. We would need to wrap the
individual methods in both the production code and unit tests.
This alters the SUT by adding the public method
wrappers.
Use the [Conditional("DEBUG")] attribute
on public methods that wrap the private methods.
Create internal methods to access the private method;
then have a public test class elsewhere in the assembly that wraps those
internal methods with public ones.
This alters the release code by adding the internal hooks, ultimately making
the private methods accessible in production.
This requires a lot of extra coding, and is hence
brittle.
By using inheritance and putting the
MyObjectTester class in the UnitTests assembly, it
doesn't add any new code to the production assembly.
Nothing in this approach depends on Debug-only
techniques.
Although this approach requires a new derived class,
and an additional public wrapper method for each protected method, it is
object-oriented and type safe.
The only extra code we created -
UnitTestUtilities , is not shipped in production.
Nothing in this approach depends on Debug-only
techniques.
This approach can call any method with a single call.
Once you have the UnitTestUtilities , the only complication is
creating the correct parameters (method name, data types, etc...) for
RunInstanceMethod or RunStaticMethod . Because the
method is being dynamically called, the parameters aren't checked at compile
time.
Last Visit: 31-Dec-99 19:00 Last Update: 2-Feb-21 18:09
java - How do you unit test private methods? - Software Engineering...
How to Test Private and Protected methods in .NET - CodeProject
How to test private methods. To test a method you need to execute | Medium
On testing private methods
Accessing Private Test Class Members | Apex Developer Guide | Salesforce...
Why make things simple when you can also make them hard and long-winded? Haven't you always been dreaming of needing 10…
How do I use JUnit to test a class that has internal private methods, fields or nested classes? It seems bad to change…
To test a method you need to execute it, but calling private methods directly can be hard or even impossible, depending on the programming language you use. In this article I’ll primarily speak about Python and Java, but the described techniques surely can be applied to many other languages (but I can’t be sure about all of them ).
Have you ever asked yourself, why do you even need private methods? You need private data to maintain consistency, but why private methods?
Strictly speaking, you don’t. But they still be helpful at least for two major reasons:
Whil e the first one prevents private data corruption, the second merely makes your API cleaner.
Surprisingly, when I first came to Java programming and googled “How to test private method in Java”, the most popular answer was something like “Why do you want to test private methods? Users call public methods, test them instead”.
Well, that sounds crazy for me. I surely can just ignore the fact that a public methods call a private one, just imagine that private method code is inlined, but that means that code of the private method will be tested again and again with every public method that calls the private one.
In the following example you really want to test private method, not the two almost identical public ones.
One can notice that some refactoring can allow me not to test the private method. That’s true and we will talk about it later. But testing _set_status without any changes is still clean and reasonable way to do. I don’t buy this “don’t test private methods”.
The simplest and most straightforward way to call a private method is, you know, call it. And that’s exactly what we do in Python and other languages that has private by convention (e. g. Perl).
To “just call it” in Java we need to change visibility of the method.
The first way is to make method package private (no access modifier) and put tests into the same package. This is a fairly common practice, but you still might want (or already have) another code structure.
The second way is to make method public. To let people know you still don’t want to call this method you can use @VisibleForTesting annotation from Guava or any other convention you like (that’s actually what Python and Perl do). By the way, IDEA totally understands that annotation and will warn you about using such public method outside of tests.
You can also put a test class inside a tested one (at least in Java), but that doesn’t look great. You have to use the same file for both classes, and your production binaries will actually contain test code.
In some languages reflections will do fine, in Ruby it’s that simple:
But in Java such code is so heavy I gave up on idea of providing an example here. More than this, you have to abandon all cool stuff your favourite IDE does for you.
Personally I prefer the “just call it” method, and I like to use @VisibleForTesting in Java to make it happen.
But let us talk again about refactoring that allows me avoiding testing private methods (by eliminating them).
The point is to merge all private data and private methods into an object of another class that contains no private methods or data, but put the instance of that class into the only private attribute of the original class. Doesn’t sound simple, but it is, consider examples:
So now you can freely test EntityPrivateData , the only thing that remains private is data attribute. Like I said before, you actually don’t need private methods, only private data.
The described method can be useful not only for testing private methods, but also for more expressive design of your software. You can use any number of such private data classes so they have more sense semantically, not only technically.
For me, the most important thing about this pattern is that it proves that you technically can eliminate all private methods. But I still doubt that it’s reasonable to do it every time, your code can bloat without any significant benefit.
This article was written with the help of Nikolay Rys .
Hot Girl Ass
Lingerie Hairy Porno
Tumblr Slut Wife Loves Pee In Mouth
Tune Brothers Dirty Nasty
Lesbian Video


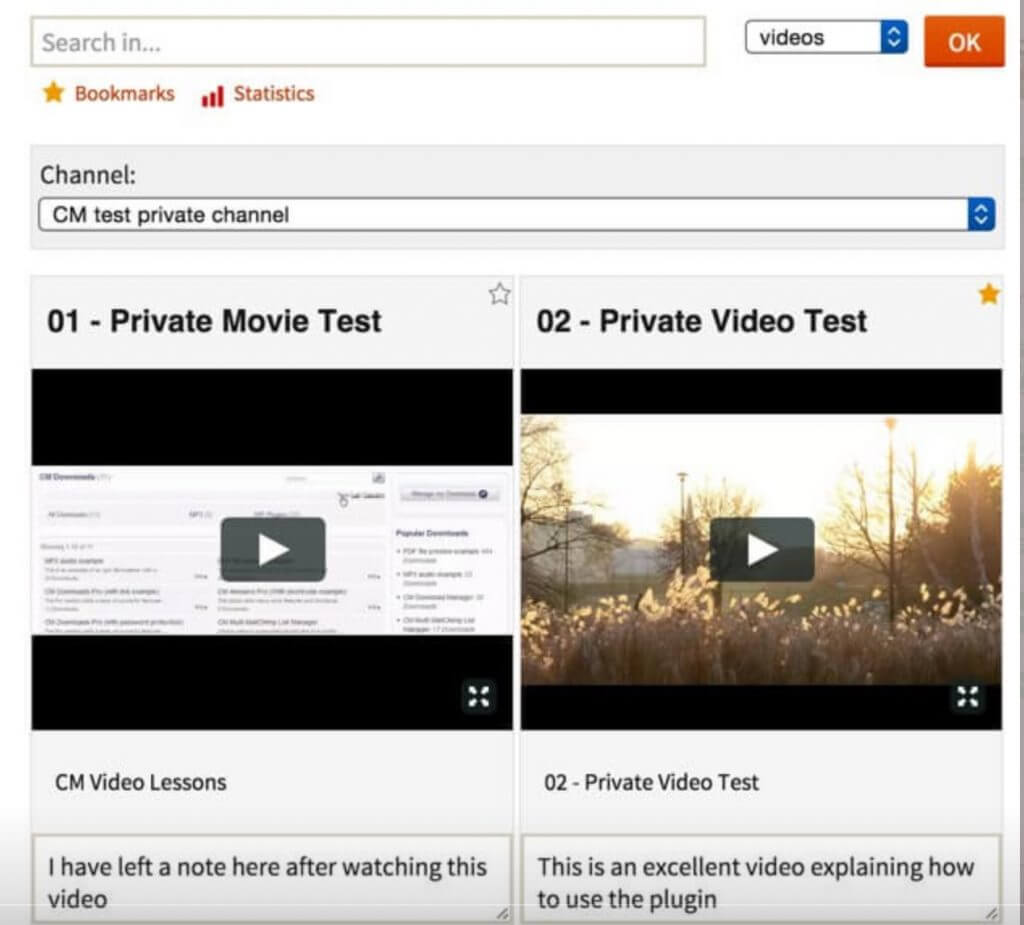

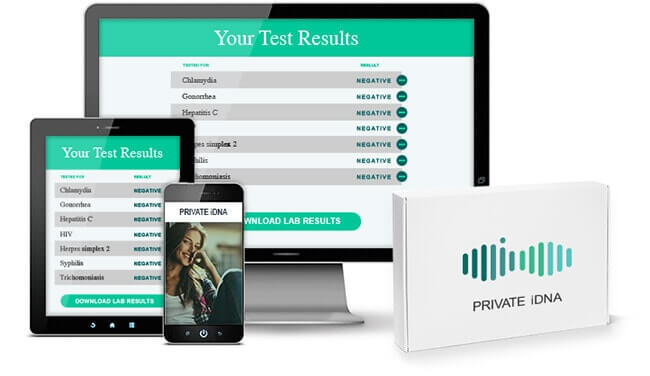
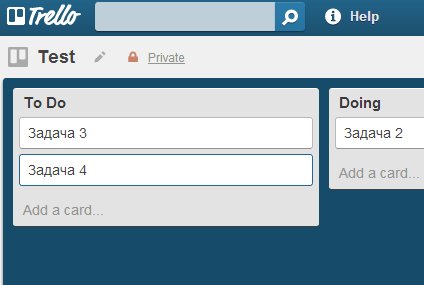





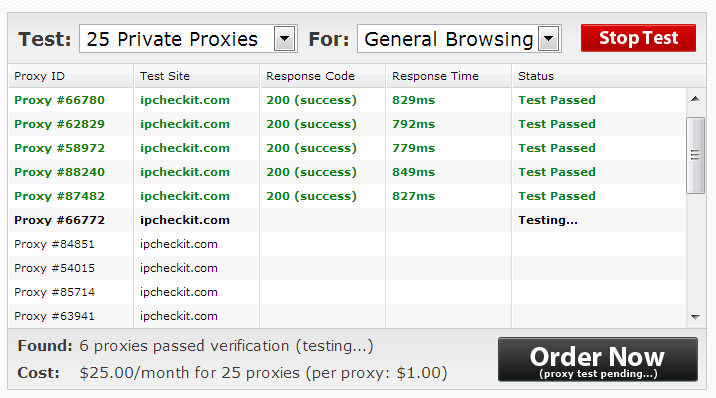
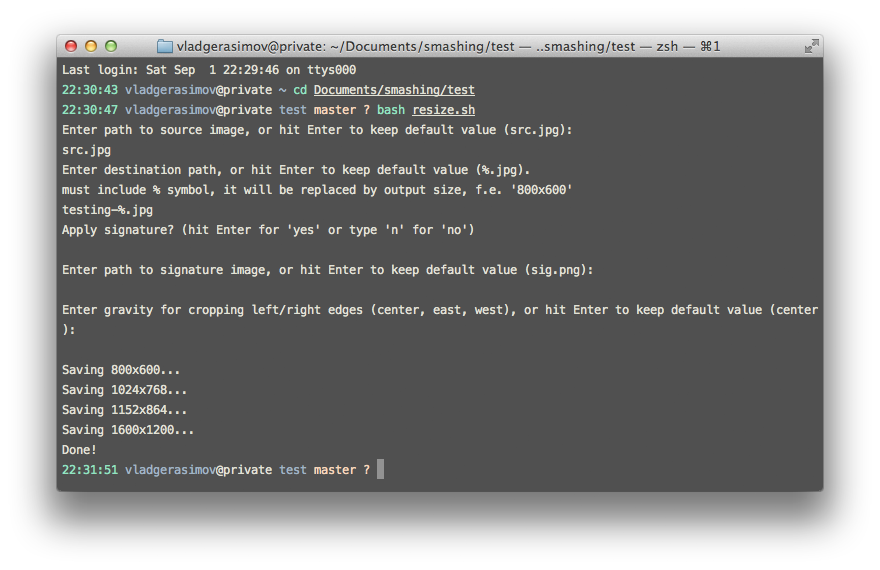






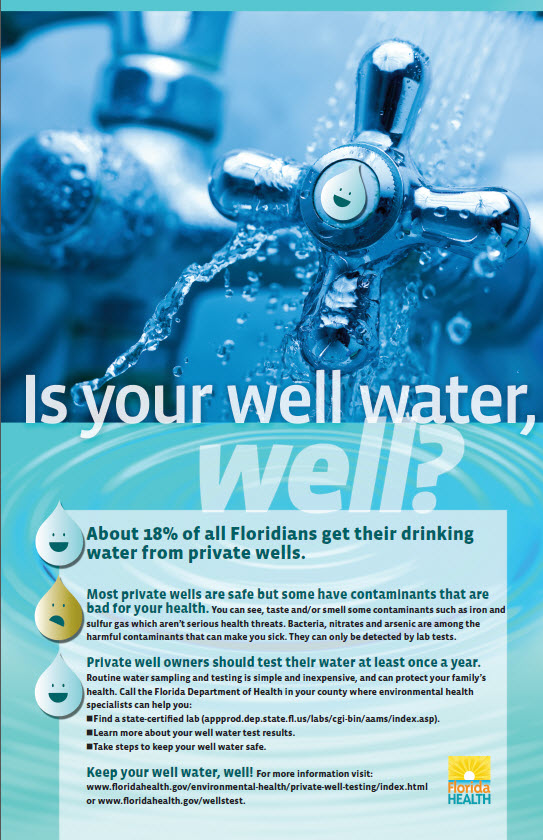


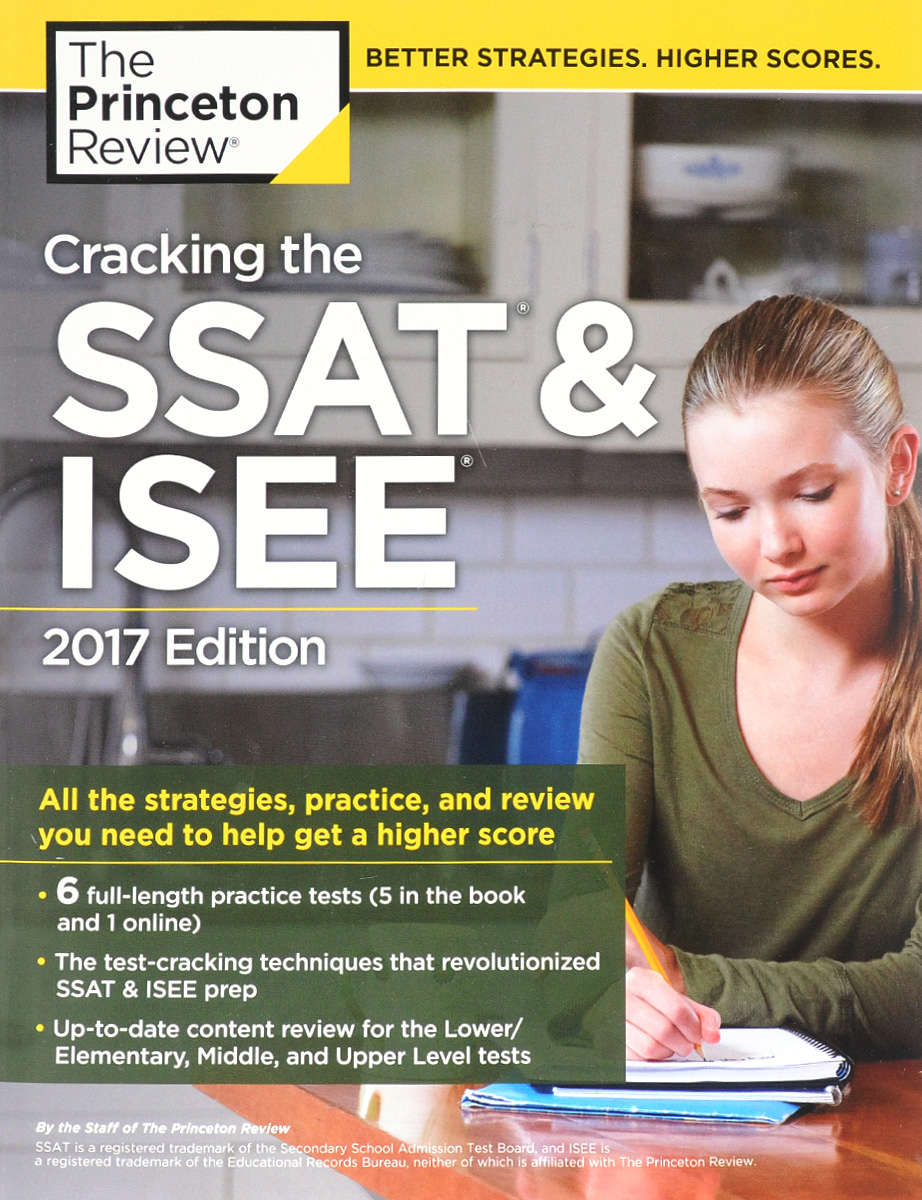
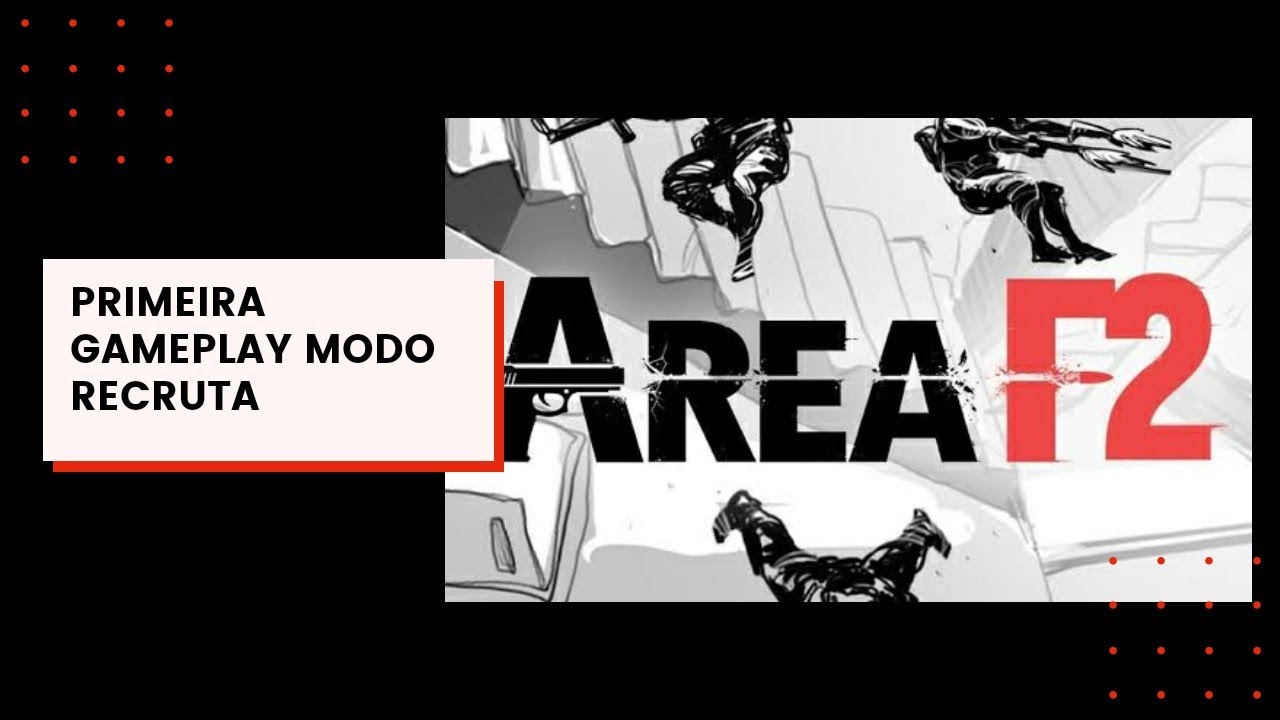




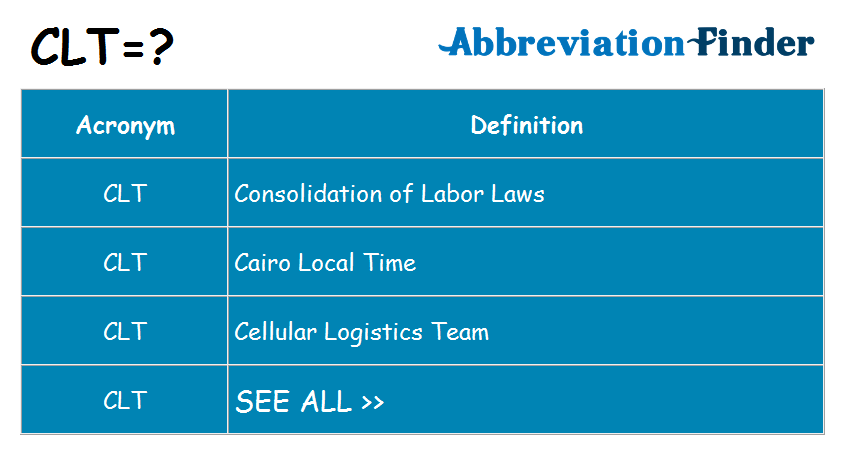



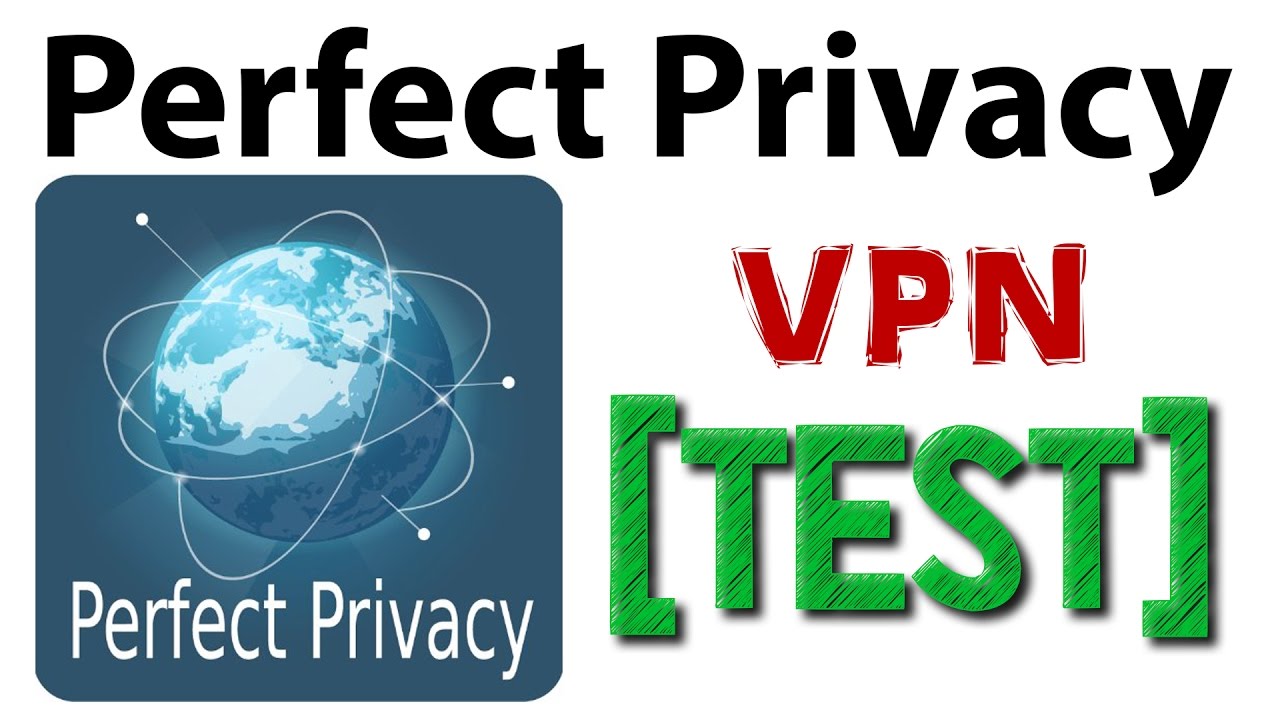

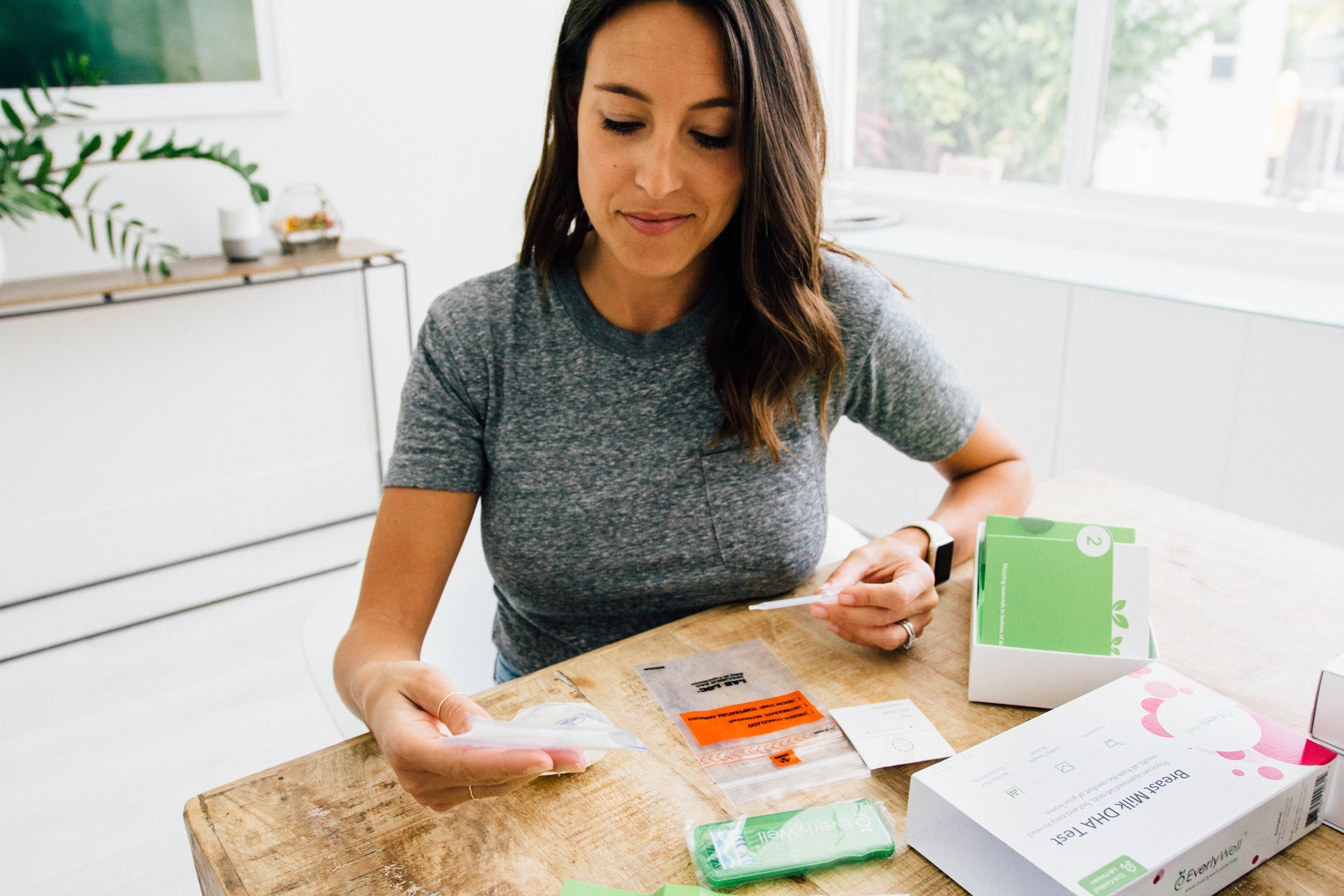
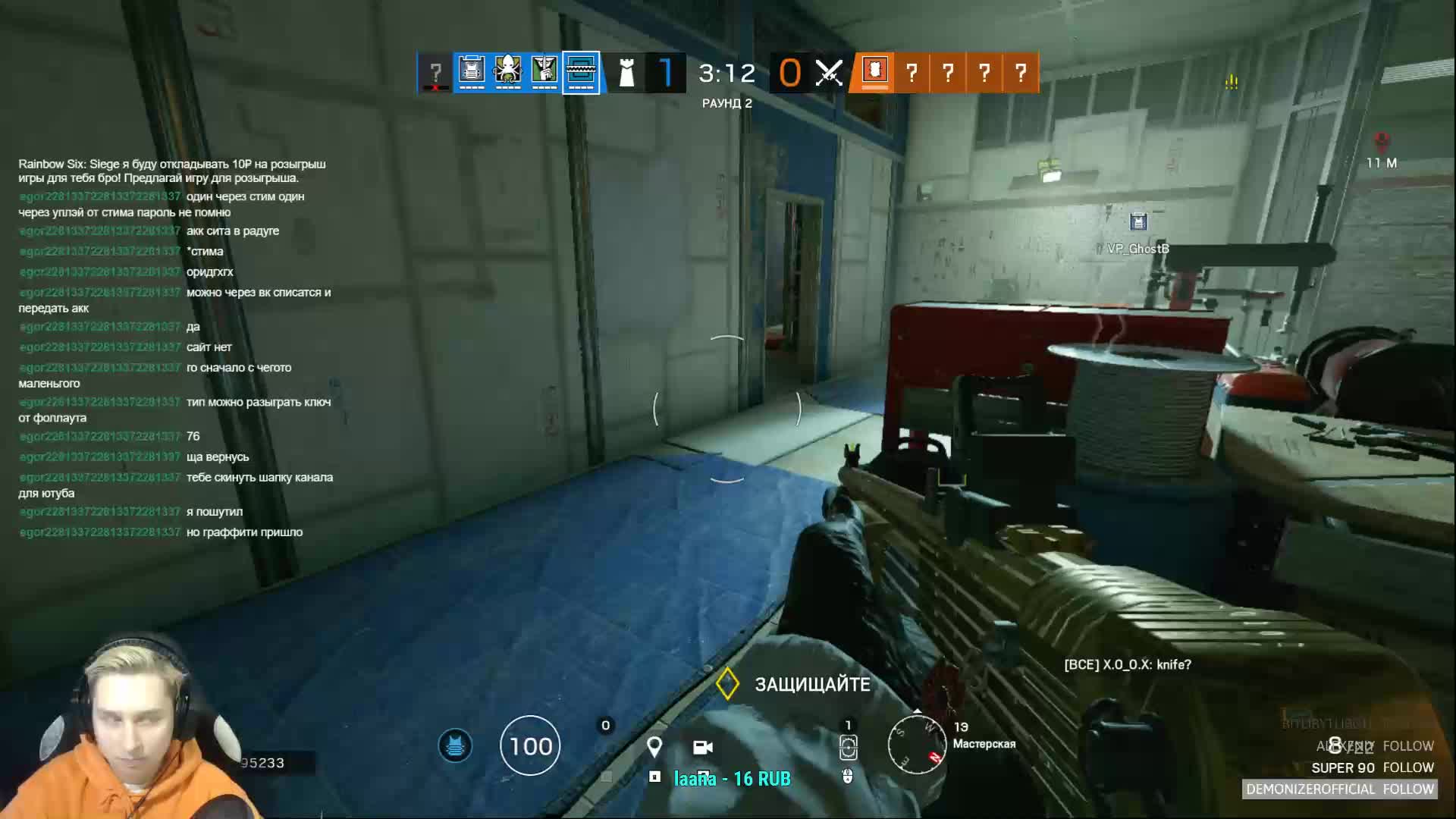
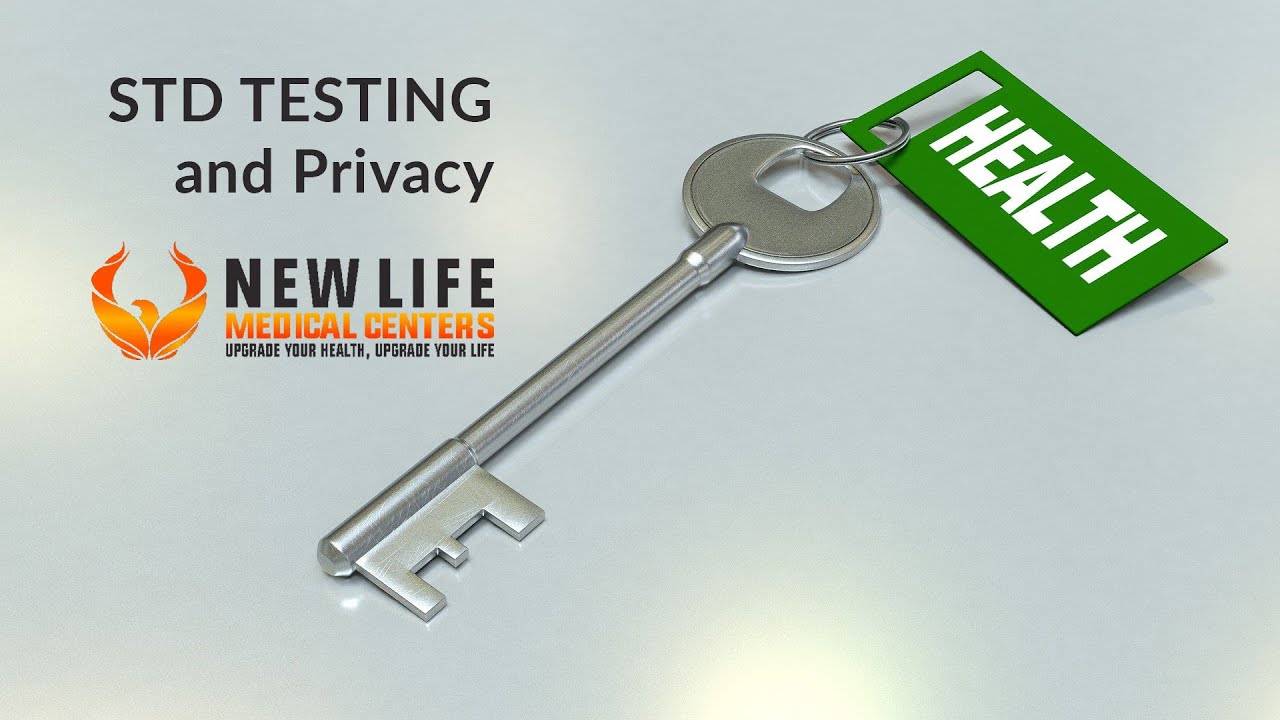



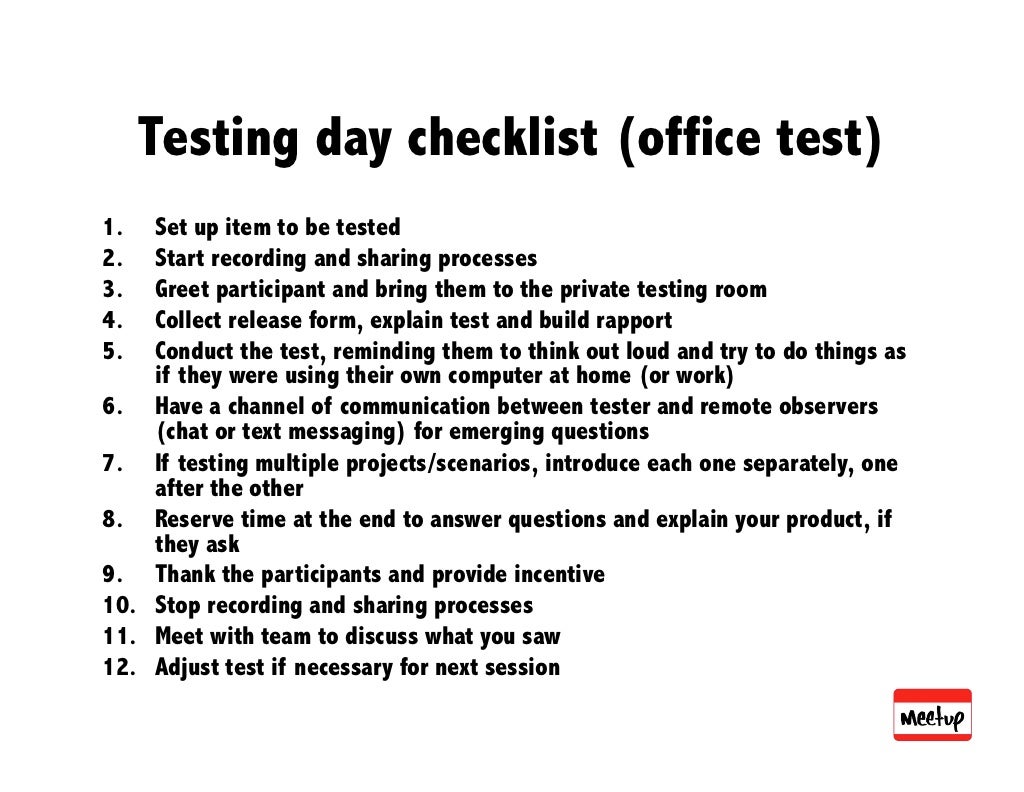






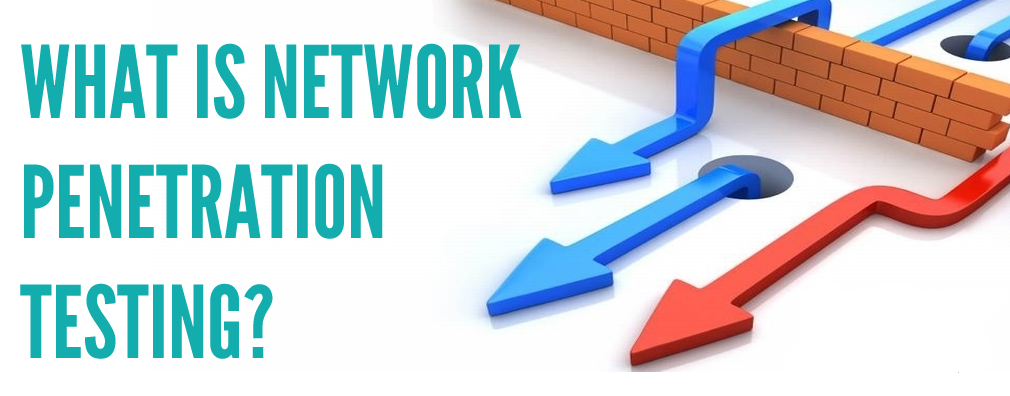
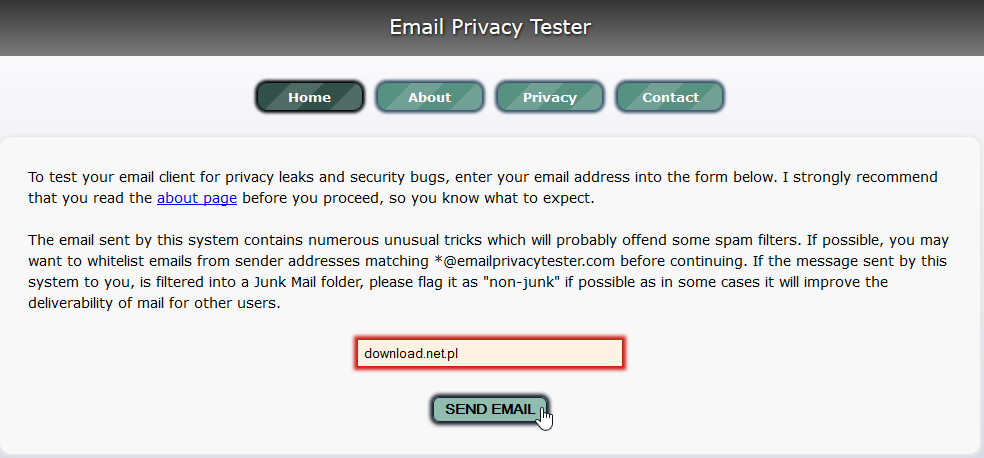


