Private String
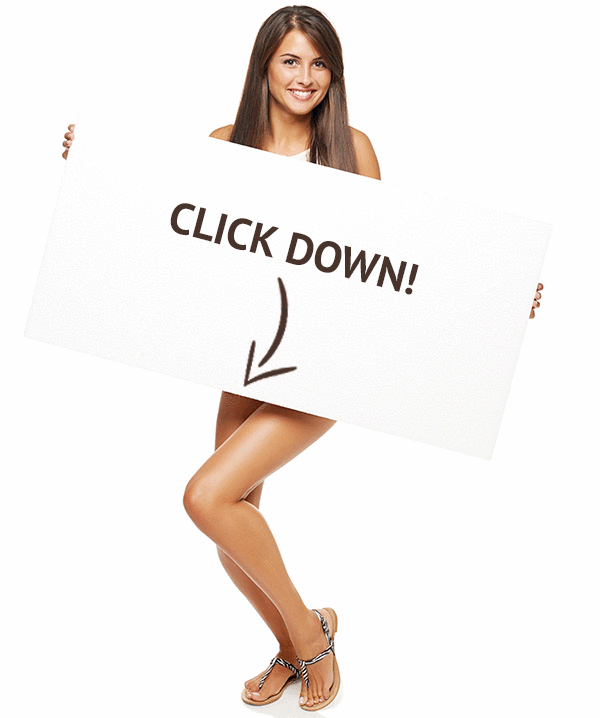
🛑 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Private String
HTML
CSS
JAVASCRIPT
SQL
PYTHON
PHP
BOOTSTRAP
HOW TO
W3.CSS
JAVA
JQUERY
C
C++
C#
R
React
w 3 s c h o o l s C E R T I F I E D . 2 0 2 2
W3Schools is optimized for learning and training. Examples might be simplified to improve reading and learning.
Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness of all content.
While using W3Schools, you agree to have read and accepted our terms of use ,
cookie and privacy policy .
Copyright 1999-2022 by Refsnes Data. All Rights Reserved.
W3Schools is Powered by W3.CSS .
The private keyword is an access modifier used for attributes, methods and constructors, making them only accessible within the declared class.
Read more about modifiers in our Java Modifiers Tutorial .
Get certified by completing a Java course today!
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Your message has been sent to W3Schools.
Table of contents
Exit focus mode
Light
Dark
High contrast
Light
Dark
High contrast
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
The private keyword is a member access modifier.
This page covers private access. The private keyword is also part of the private protected access modifier.
Private access is the least permissive access level. Private members are accessible only within the body of the class or the struct in which they are declared, as in this example:
Nested types in the same body can also access those private members.
It is a compile-time error to reference a private member outside the class or the struct in which it is declared.
For a comparison of private with the other access modifiers, see Accessibility Levels and Access Modifiers .
In this example, the Employee class contains two private data members, _name and _salary . As private members, they cannot be accessed except by member methods. Public methods named GetName and Salary are added to allow controlled access to the private members. The _name member is accessed by way of a public method, and the _salary member is accessed by way of a public read-only property. (See Properties for more information.)
For more information, see Declared accessibility in the C# Language Specification . The language specification is the definitive source for C# syntax and usage.
Table of contents
Exit focus mode
Light
Dark
High contrast
Light
Dark
High contrast
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
A string is an object of type String whose value is text. Internally, the text is stored as a sequential read-only collection of Char objects. There's no null-terminating character at the end of a C# string; therefore a C# string can contain any number of embedded null characters ('\0'). The Length property of a string represents the number of Char objects it contains, not the number of Unicode characters. To access the individual Unicode code points in a string, use the StringInfo object.
In C#, the string keyword is an alias for String . Therefore, String and string are equivalent, regardless it's recommended to use the provided alias string as it works even without using System; . The String class provides many methods for safely creating, manipulating, and comparing strings. In addition, the C# language overloads some operators to simplify common string operations. For more information about the keyword, see string . For more information about the type and its methods, see String .
You can declare and initialize strings in various ways, as shown in the following example:
You don't use the new operator to create a string object except when initializing the string with an array of chars.
Initialize a string with the Empty constant value to create a new String object whose string is of zero length. The string literal representation of a zero-length string is "". By initializing strings with the Empty value instead of null , you can reduce the chances of a NullReferenceException occurring. Use the static IsNullOrEmpty(String) method to verify the value of a string before you try to access it.
String objects are immutable : they can't be changed after they've been created. All of the String methods and C# operators that appear to modify a string actually return the results in a new string object. In the following example, when the contents of s1 and s2 are concatenated to form a single string, the two original strings are unmodified. The += operator creates a new string that contains the combined contents. That new object is assigned to the variable s1 , and the original object that was assigned to s1 is released for garbage collection because no other variable holds a reference to it.
Because a string "modification" is actually a new string creation, you must use caution when you create references to strings. If you create a reference to a string, and then "modify" the original string, the reference will continue to point to the original object instead of the new object that was created when the string was modified. The following code illustrates this behavior:
For more information about how to create new strings that are based on modifications such as search and replace operations on the original string, see How to modify string contents .
Quoted string literals start and end with a single double quote character ( " ) on the same line. Quoted string literals are best suited for strings that fit on a single line and don't include any escape sequences . A quoted string literal must embed escape characters, as shown in the following example:
Verbatim string literals are more convenient for multi-line strings, strings that contain backslash characters, or embedded double quotes. Verbatim strings preserve new line characters as part of the string text. Use double quotation marks to embed a quotation mark inside a verbatim string. The following example shows some common uses for verbatim strings:
Beginning with C# 11, you can use raw string literals to more easily create strings that are multi-line, or use any characters requiring escape sequences. Raw string literals remove the need to ever use escape sequences. You can write the string, including whitespace formatting, how you want it to appear in output. A raw string literal :
The following examples demonstrate these rules:
The following examples demonstrate the compiler errors reported based on these rules:
The first two examples are invalid because multiline raw string literals require the opening and closing quote sequence on its own line. The third example is invalid because the text is outdented from the closing quote sequence.
You should consider raw string literals when you're generating text that includes characters that require escape sequences when using quoted string literals or verbatim string literals. Raw string literals will be easier for you and others to read because it will more closely resemble the output text. For example, consider the following code that includes a string of formatted JSON:
Compare that text with the equivalent text in our sample on JSON serialization , which doesn't make use of this new feature.
When using the \x escape sequence and specifying less than 4 hex digits, if the characters that immediately follow the escape sequence are valid hex digits (i.e. 0-9, A-F, and a-f), they will be interpreted as being part of the escape sequence. For example, \xA1 produces "¡", which is code point U+00A1. However, if the next character is "A" or "a", then the escape sequence will instead be interpreted as being \xA1A and produce "ਚ", which is code point U+0A1A. In such cases, specifying all 4 hex digits (e.g. \x00A1 ) will prevent any possible misinterpretation.
At compile time, verbatim strings are converted to ordinary strings with all the same escape sequences. Therefore, if you view a verbatim string in the debugger watch window, you will see the escape characters that were added by the compiler, not the verbatim version from your source code. For example, the verbatim string @"C:\files.txt" will appear in the watch window as "C:\\files.txt".
A format string is a string whose contents are determined dynamically at run time. Format strings are created by embedding interpolated expressions or placeholders inside of braces within a string. Everything inside the braces ( {...} ) will be resolved to a value and output as a formatted string at run time. There are two methods to create format strings: string interpolation and composite formatting.
Available in C# 6.0 and later, interpolated strings are identified by the $ special character and include interpolated expressions in braces. If you're new to string interpolation, see the String interpolation - C# interactive tutorial for a quick overview.
Use string interpolation to improve the readability and maintainability of your code. String interpolation achieves the same results as the String.Format method, but improves ease of use and inline clarity.
Beginning with C# 10, you can use string interpolation to initialize a constant string when all the expressions used for placeholders are also constant strings.
Beginning with C# 11, you can combine raw string literals with string interpolations. You start and end the format string with three or more successive double quotes. If your output string should contain the { or } character, you can use extra $ characters to specify how many { and } characters start and end an interpolation. Any sequence of fewer { or } characters is included in the output. The following example shows how you can use that feature to display the distance of a point from the origin, and place the point inside braces:
C# also allows verbatim string interpolation, for example across multiple lines, using the $@ or @$ syntax.
To interpret escape sequences literally, use a verbatim string literal. An interpolated verbatim string starts with the $ character followed by the @ character. Starting with C# 8.0, you can use the $ and @ tokens in any order: both $@"..." and @$"..." are valid interpolated verbatim strings.
The String.Format utilizes placeholders in braces to create a format string. This example results in similar output to the string interpolation method used above.
For more information on formatting .NET types, see Formatting Types in .NET .
A substring is any sequence of characters that is contained in a string. Use the Substring method to create a new string from a part of the original string. You can search for one or more occurrences of a substring by using the IndexOf method. Use the Replace method to replace all occurrences of a specified substring with a new string. Like the Substring method, Replace actually returns a new string and doesn't modify the original string. For more information, see How to search strings and How to modify string contents .
You can use array notation with an index value to acquire read-only access to individual characters, as in the following example:
If the String methods don't provide the functionality that you must have to modify individual characters in a string, you can use a StringBuilder object to modify the individual chars "in-place", and then create a new string to store the results by using the StringBuilder methods. In the following example, assume that you must modify the original string in a particular way and then store the results for future use:
An empty string is an instance of a System.String object that contains zero characters. Empty strings are used often in various programming scenarios to represent a blank text field. You can call methods on empty strings because they're valid System.String objects. Empty strings are initialized as follows:
By contrast, a null string doesn't refer to an instance of a System.String object and any attempt to call a method on a null string causes a NullReferenceException . However, you can use null strings in concatenation and comparison operations with other strings. The following examples illustrate some cases in which a reference to a null string does and doesn't cause an exception to be thrown:
String operations in .NET are highly optimized and in most cases don't significantly impact performance. However, in some scenarios such as tight loops that are executing many hundreds or thousands of times, string operations can affect performance. The StringBuilder class creates a string buffer that offers better performance if your program performs many string manipulations. The StringBuilder string also enables you to reassign individual characters, something the built-in string data type doesn't support. This code, for example, changes the content of a string without creating a new string:
In this example, a StringBuilder object is used to create a string from a set of numeric types:
Because the String type implements IEnumerable , you can use the extension methods defined in the Enumerable class on strings. To avoid visual clutter, these methods are excluded from IntelliSense for the String type, but they're available nevertheless. You can also use LINQ query expressions on strings. For more information, see LINQ and Strings .
\uHHHH (range: 0000 - FFFF; example: \u00E7 = "ç")
\U00HHHHHH (range: 000000 - 10FFFF; example: \U0001F47D = "👽")
Unicode escape sequence similar to "\u" except with variable length
\xH[H][H][H] (range: 0 - FFFF; example: \x00E7 or \x0E7 or \xE7 = "ç")
Last edited on Mar 8, 2013 at 6:16pm
Never did .net before...Just got hooked on trying C++.
My research said that you only declare in the header and define in the class .cpp.
Are you saying that the constructor definition go in the header?
Last edited on Mar 8, 2013 at 6:42pm
Last edited on Mar 8, 2013 at 6:43pm
@Peter87
Well, that was embarrassing, and I know better then those 6 errors.
@mutexe
Thank you for the sample. I understand the process of objects. What I am
trying to figure out is how to get the name to change through the setters and getters.
Last edited on Mar 8, 2013 at 6:56pm
Last edited on Mar 8, 2013 at 7:22pm
Last edited on Mar 8, 2013 at 7:32pm
There's actually several false practices in this thread.
1. Getters and setters are bad. For one, you don't need getters - other code should not change its behavior just because the object it is dealing with has a different state; that goes against encapsulation. For two, you should not use setters - it means external code has to calculate the new state of the object, which is also against encapsulation.
Instead, prefer objects that explain themselves (e.g. they have their own function for drawing themself to the screen) and which allow you to change their state indirectly. You can attack an object, but you shouldn't perform calculations for it and set its new health.
Basically, your getters and setters are bad, but your "behaviors" are good.
2. Prefer to define functions inline when possible. If you define everything in the CPP file, that makes it much much harder for the compiler to optimize your code. If everything is available inline in the header with the class definition, then the compiler can easily optimize your code and make it run faster.
Obviously for writing things like libraries, you should use PIMPL, but for making the classes for your game you should try to inline as much as possible to take advantage of compiler optimizations.
The whole "always define functions in CPP file" can also backfire: many people who try to learn templates often end up making a templated class and defining the function in the source file, which is wrong - it will not work. Templates must be defined inline, or their definition must be visible wherever they are used.
You can feel free to dispute or disagree with my points, but they're from my personal experience and readings, and they're working very well for me. Obviously they don't apply 100% of the time, but they usually do.
Last edited on Mar 8, 2013 at 7:44pm
@ AbstractionAnon
I told myself to stop copying and pasting I don't know how but that could be the only reason for line 22. Thank you....
@ L B
????????
I thought getters and setters to protect the private data was supposed to be a good thing. That is the reason I have been making sure to do it. Isn't it standard?
Last edited on Mar 8, 2013 at 7:52pm
Last edited on Mar 8, 2013 at 8:05pm
I guess that the way I am doing it is more coding and typing...but I looked around the I-net and they say it is worth the extra work. I don't know....
I don't really see anything wrong with it.
Thank you everyone I am not sure I am not missing anything, but I am not stuck anymore which is awesome.
What's wrong with it is th
Nasty Tube
Gaming Naked
Naked Holiday