Private Php Do
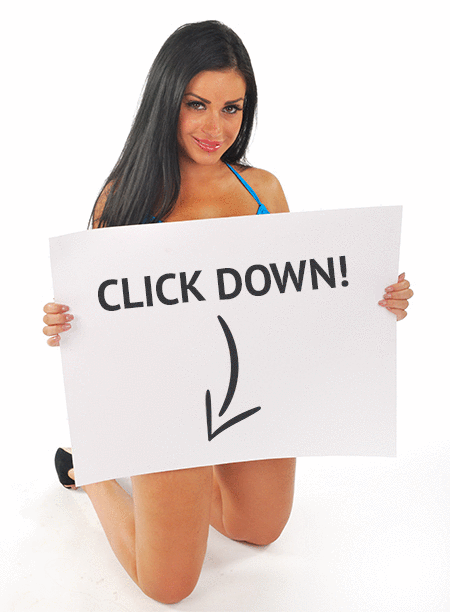
🛑 👉🏻👉🏻👉🏻 INFORMATION AVAILABLE CLICK HERE👈🏻👈🏻👈🏻
Change language:
English
Brazilian Portuguese
Chinese (Simplified)
French
German
Japanese
Romanian
Russian
Spanish
Turkish
Other
Область видимости свойства, метода или константы (начиная c PHP 7.1.0) может быть определена путём использования следующих ключевых слов в объявлении: public, protected или private. Доступ к свойствам и методам класса, объявленным как public (общедоступный), разрешён отовсюду. Модификатор protected (защищённый) разрешает доступ самому классу, наследующим его классам и родительским классам. Модификатор private (закрытый) ограничивает область видимости так, что только класс, где объявлен сам элемент, имеет к нему доступ.
Свойства класса должны быть определены через модификаторы public, private или protected. Если же свойство определено с помощью var, то оно будет объявлено общедоступным свойством.
Пример #1 Объявление свойства класса
public;
echo $this->protected;
echo $this->private;
}
}
$obj = new MyClass();
echo $obj->public; // Работает
echo $obj->protected; // Неисправимая ошибка
echo $obj->private; // Неисправимая ошибка
$obj->printHello(); // Выводит Public, Protected и Private
/**
* Определение MyClass2
*/
class MyClass2 extends MyClass
{
// Мы можем переопределить общедоступные и защищённые свойства, но не закрытые
public $public = 'Public2';
protected $protected = 'Protected2';
function printHello()
{
echo $this->public;
echo $this->protected;
echo $this->private;
}
}
$obj2 = new MyClass2();
echo $obj2->public; // Работает
echo $obj2->private; // Неопределён
echo $obj2->protected; // Неисправимая ошибка
$obj2->printHello(); // Выводит Public2, Protected2, Undefined
?>
Методы класса должны быть определены через модификаторы public, private, или protected. Методы, где определение модификатора отсутствует, определяются как public.
MyPublic();
$this->MyProtected();
$this->MyPrivate();
}
}
$myclass = new MyClass;
$myclass->MyPublic(); // Работает
$myclass->MyProtected(); // Неисправимая ошибка
$myclass->MyPrivate(); // Неисправимая ошибка
$myclass->Foo(); // Работает общедоступный, защищённый и закрытый
/**
* Определение MyClass2
*/
class MyClass2 extends MyClass
{
// Это общедоступный метод
function Foo2()
{
$this->MyPublic();
$this->MyProtected();
$this->MyPrivate(); // Неисправимая ошибка
}
}
$myclass2 = new MyClass2;
$myclass2->MyPublic(); // Работает
$myclass2->Foo2(); // Работает общедоступный и защищённый, закрытый не работает
class Bar
{
public function test() {
$this->testPrivate();
$this->testPublic();
}
public function testPublic() {
echo "Bar::testPublic\n";
}
private function testPrivate() {
echo "Bar::testPrivate\n";
}
}
class Foo extends Bar
{
public function testPublic() {
echo "Foo::testPublic\n";
}
private function testPrivate() {
echo "Foo::testPrivate\n";
}
}
$myFoo = new Foo();
$myFoo->test(); // Bar::testPrivate
// Foo::testPublic
?>
Начиная с PHP 7.1.0, константы класса могут быть определены как public, private или protected. Константы, объявленные без указания области видимости, определяются как public.
Пример #3 Объявление констант, начиная с PHP 7.1.0
foo(); // Выводятся константы public, protected и private
/**
* Объявление класса MyClass2
*/
class MyClass2 extends MyClass
{
// Публичный метод
function foo2()
{
echo self::MY_PUBLIC;
echo self::MY_PROTECTED;
echo self::MY_PRIVATE; // Неисправимая ошибка
}
}
$myclass2 = new MyClass2;
echo MyClass2::MY_PUBLIC; // Работает
$myclass2->foo2(); // Выводятся константы public и protected, но не private
?>
Объекты, которые имеют общий тип (наследуются от одного класса), имеют доступ к элементам с модификаторами private и protected друг друга, даже если не являются одним и тем же экземпляром. Это объясняется тем, что реализация видимости элементов известна внутри этих объектов.
Пример #4 Доступ к элементам с модификатором private из объектов одного типа
foo = $foo;
}
private function bar()
{
echo 'Доступ к закрытому методу.';
}
public function baz(Test $other)
{
// Мы можем изменить закрытое свойство:
$other->foo = 'привет';
var_dump($other->foo);
// Мы также можем вызвать закрытый метод:
$other->bar();
}
}
$test = new Test('test');
$test->baz(new Test('other'));
?>
Результат выполнения данного примера:
string(6) "привет"
Доступ к закрытому методу.
add a note
User Contributed Notes 26 notes
INSIDE CODE and OUTSIDE CODE
label = 'Ink-Jet Tatoo Gun';
$item->price = 49.99;
?>
Ok, that's simple enough... I got it inside and out. The big problem with this is that the Item class is COMPLETELY IGNORANT in the following ways:
* It REQUIRES OUTSIDE CODE to do all the work AND to know what and how to do it -- huge mistake.
* OUTSIDE CODE can cast Item properties to any other PHP types (booleans, integers, floats, strings, arrays, and objects etc.) -- another huge mistake.
Note: we did it correctly above, but what if someone made an array for $price? FYI: PHP has no clue what we mean by an Item, especially by the terms of our class definition above. To PHP, our Item is something with two properties (mutable in every way) and that's it. As far as PHP is concerned, we can pack the entire set of Britannica Encyclopedias into the price slot. When that happens, we no longer have what we expect an Item to be.
INSIDE CODE should keep the integrity of the object. For example, our class definition should keep $label a string and $price a float -- which means only strings can come IN and OUT of the class for label, and only floats can come IN and OUT of the class for price.
label and $item->price,
* by using the protected keyword.
* 2. FORCE the use of public functions.
* 3. ONLY strings are allowed IN & OUT of this class for $label
* via the getLabel and setLabel functions.
* 4. ONLY floats are allowed IN & OUT of this class for $price
* via the getPrice and setPrice functions.
*/
protected $label = 'Unknown Item'; // Rule 1 - protected.
protected $price = 0.0; // Rule 1 - protected.
public function getLabel() { // Rule 2 - public function.
return $this->label; // Rule 3 - string OUT for $label.
}
public function getPrice() { // Rule 2 - public function.
return $this->price; // Rule 4 - float OUT for $price.
}
public function setLabel($label) // Rule 2 - public function.
{
/**
* Make sure $label is a PHP string that can be used in a SORTING
* alogorithm, NOT a boolean, number, array, or object that can't
* properly sort -- AND to make sure that the getLabel() function
* ALWAYS returns a genuine PHP string.
*
* Using a RegExp would improve this function, however, the main
* point is the one made above.
*/
if(is_string($label))
{
$this->label = (string)$label; // Rule 3 - string IN for $label.
}
}
public function setPrice($price) // Rule 2 - public function.
{
/**
* Make sure $price is a PHP float so that it can be used in a
* NUMERICAL CALCULATION. Do not accept boolean, string, array or
* some other object that can't be included in a simple calculation.
* This will ensure that the getPrice() function ALWAYS returns an
* authentic, genuine, full-flavored PHP number and nothing but.
*
* Checking for positive values may improve this function,
* however, the main point is the one made above.
*/
if(is_numeric($price))
{
$this->price = (float)$price; // Rule 4 - float IN for $price.
}
}
}
?>
Now there is nothing OUTSIDE CODE can do to obscure the INSIDES of an Item. In other words, every instance of Item will always look and behave like any other Item complete with a label and a price, AND you can group them together and they will interact without disruption. Even though there is room for improvement, the basics are there, and PHP will not hassle you... which means you can keep your hair!
If you have problems with overriding private methods in extended classes, read this:)
The manual says that "Private limits visibility only to the class that defines the item". That means extended children classes do not see the private methods of parent class and vice versa also.
As a result, parents and children can have different implementations of the "same" private methods, depending on where you call them (e.g. parent or child class instance). Why? Because private methods are visible only for the class that defines them and the child class does not see the parent's private methods. If the child doesn't see the parent's private methods, the child can't override them. Scopes are different. In other words -- each class has a private set of private variables that no-one else has access to.
A sample demonstrating the percularities of private methods when extending classes:
overridden();
}
private function overridden() {
echo 'base';
}
}
class child extends base {
private function overridden() {
echo 'child';
}
}
$test = new child();
$test->inherited();
?>
Output will be "base".
If you want the inherited methods to use overridden functionality in extended classes but public sounds too loose, use protected. That's what it is for:)
A sample that works as intended:
overridden();
}
protected function overridden() {
echo 'base';
}
}
class child extends base {
protected function overridden() {
echo 'child';
}
}
$test = new child();
$test->inherited();
?>
Output will be "child".
Just a quick note that it's possible to declare visibility for multiple properties at the same time, by separating them by commas.
eg:
A class A static public function can access to class A private function :
foo();
}
}
$a = new A();
A::bar($a);
?>
It's working.
1 . If the class member declared as public then it can be accessed everywhere.
2 . If the class members declared as protected then it can be accessed only within the class itself and by inheriting and parent classes.
3 .If the class members declared as private then it may only be accessed by the class that defines the member.
tag_line."";
}
}
// SubClass
class child extends pub {
function show(){
echo $this->tag_line;
}
}
// Object Declaration
$obj= new child;
// A Computer Science Portal for Geeks!
echo $obj->tag_line."";
// A Computer Science Portal for Geeks!
$obj->display();
// A Computer Science Portal for Geeks!
$obj->show();
?>
x-$this->y . "";
}
}
// SubClass - Inherited Class
class child extends pro {
function mul() //Multiply Function
{
echo $sub=$this->x*$this->y;
}
}
$obj= new child;
$obj->sub();
$obj->mul();
?>
name;
}
}
// Object Declaration
$obj= new child;
// Uncaught Error: Call to private method demo::show()
$obj->show();
//Undefined property: child::$name
$obj->display();
?>
private , $this->public\n";
}
}
class B extends A
{
function __construct()
{
$this->private = 2;
$this->public = 2;
}
function set()
{
$this->private = 3;
$this->public = 3;
}
function get()
{
return parent::get() . "B: $this->private , $this->public\n";
}
}
$B = new B;
echo $B->get();
echo $B->set();
echo $B->get();
?>
?>
Result is
A: 1 , 2
B: 2 , 2
A: 1 , 3
B: 3 , 3
This is correct code and does not warn you to use any private.
"$this->private" is only in A private. If you write it in class B it's a runtime declaration of the public variable "$this->private", and PHP doesn't even warn you that you create a variable in a class without declaration, because this is normal behavior.
Please note that protected methods are also available from sibling classes as long as the method is declared in the common parent. This may also be an abstract method.
In the below example Bar knows about the existence of _test() in Foo because they inherited this method from the same parent. It does not matter that it was abstract in the parent.
_test();
}
}
class Foo extends Base {
protected function _test() {
echo 'Foo';
}
}
$bar = new Bar();
$bar->TestFoo(); // result: Foo
?>
Beware: Visibility works on a per-class-base and does not prevent instances of the same class accessing each others properties!
bar, "\n";
}
public function setBar($value)
{
// Neccessary method, for $bar is invisible outside the class
$this->bar = $value;
}
public function setForeignBar(Foo $object, $value)
{
// this does NOT violate visibility!
$object->bar = $value;
}
}
$a = new Foo();
$b = new Foo();
$a->setBar(1);
$b->setBar(2);
$a->debugBar($b); // 2
$b->debugBar($a); // 1
$a->setForeignBar($b, 3);
$b->setForeignBar($a, 4);
$a->debugBar($b); // 3
$b->debugBar($a); // 4
?>
The code prints
test Object ( [public] => Public var [protected:protected] => protected var [private:test:private] => Private var )
Functions like print_r(), var_dump() and var_export() prints public, protected and private variables, but not the static variables.
if not overwritten, self::$foo in a subclass actually refers to parent's self::$foo
tell_me(); // bar
$first->change_foo("restaurant");
$second->tell_me(); // restaurant
?>
Some Method Overriding rules :
1. In the overriding, the method names and arguments (arg’s) must be same.
Example:
class p { public function getName(){} }
class c extends P{ public function getName(){} }
2. final methods can’t be overridden.
3. private methods never participate in the in the overriding because these methods are not visible in the child classes.
Example:
class a {
private function my(){
print "parent:my";
}
public function getmy(){
$this->my();
}
}
class b extends a{
private function my(){
print "base:my";
}
}
$x = new b();
$x->getmy(); // parent:my
4. While overriding decreasing access specifier is not allowed
class a {
public function my(){
print "parent:my";
}
}
class b extends a{
private function my(){
print "base:my";
}
}
//Fatal error: Access level to b::my() must be public (as in class a)
I have simplified the last method (Example #4) showing how to call private function outside the class. I think this is an idea of that example:
bar();
}
}
$test=new Test();
$test->baz();
$test->bar();
?>
This has already been noted here, bu
Www Teenikini Nude Hd Solo Skinny Nu
Pov Humiliation Videos
Pantyhose Candid Pics
Harley Quinn Naked Gif
Czech Granny Orgy
PHP: Область видимости - Manual
forum.littleone.ru
forums.drom.ru
parapa.ru
П.А.К.Т. - Личный кабинет
Private Php Do


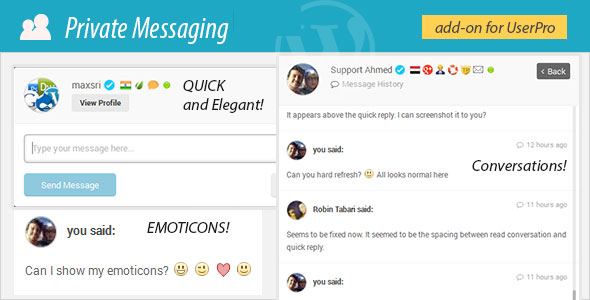
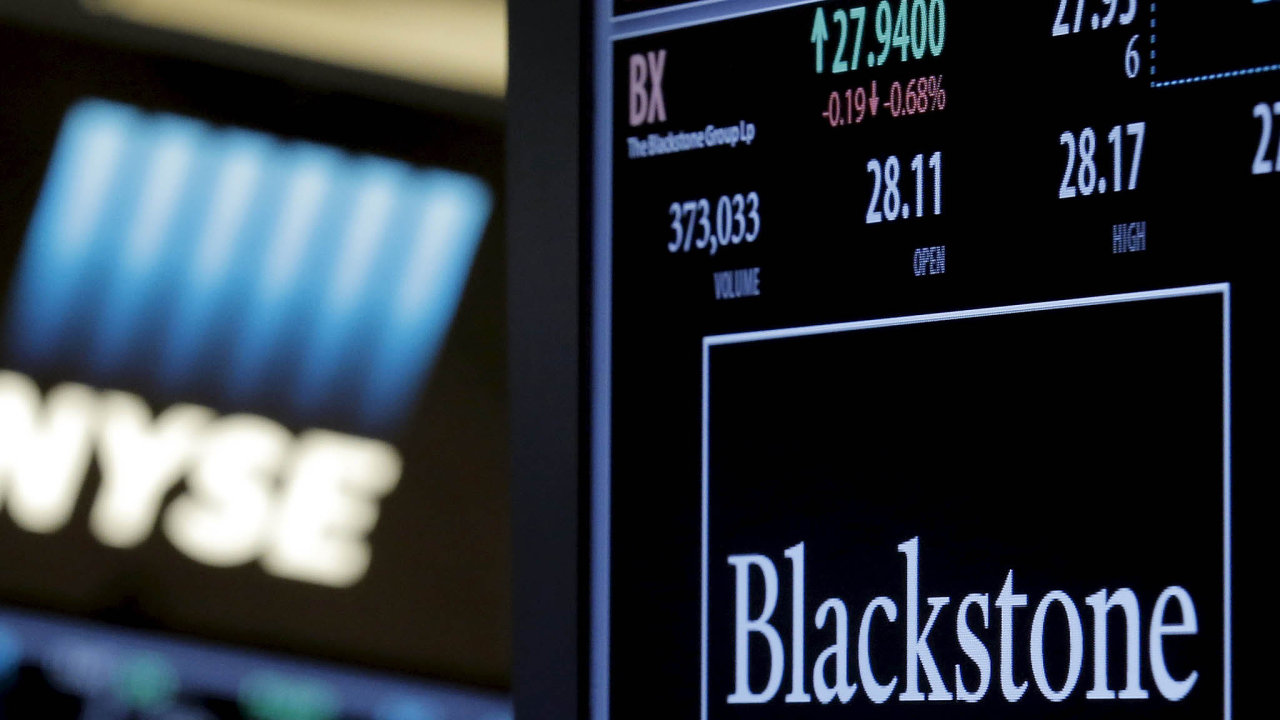
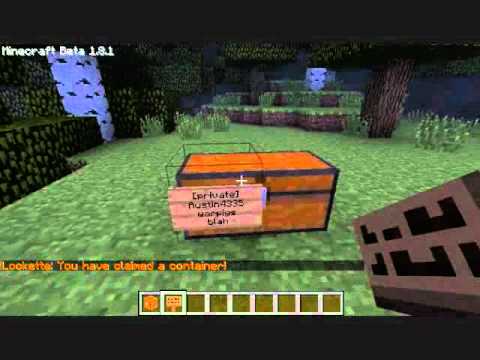








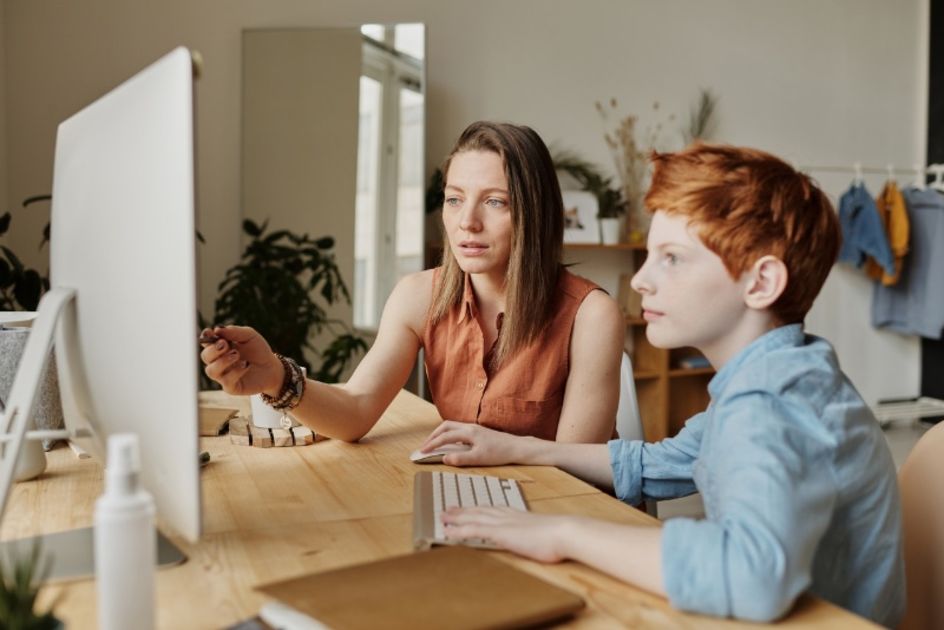


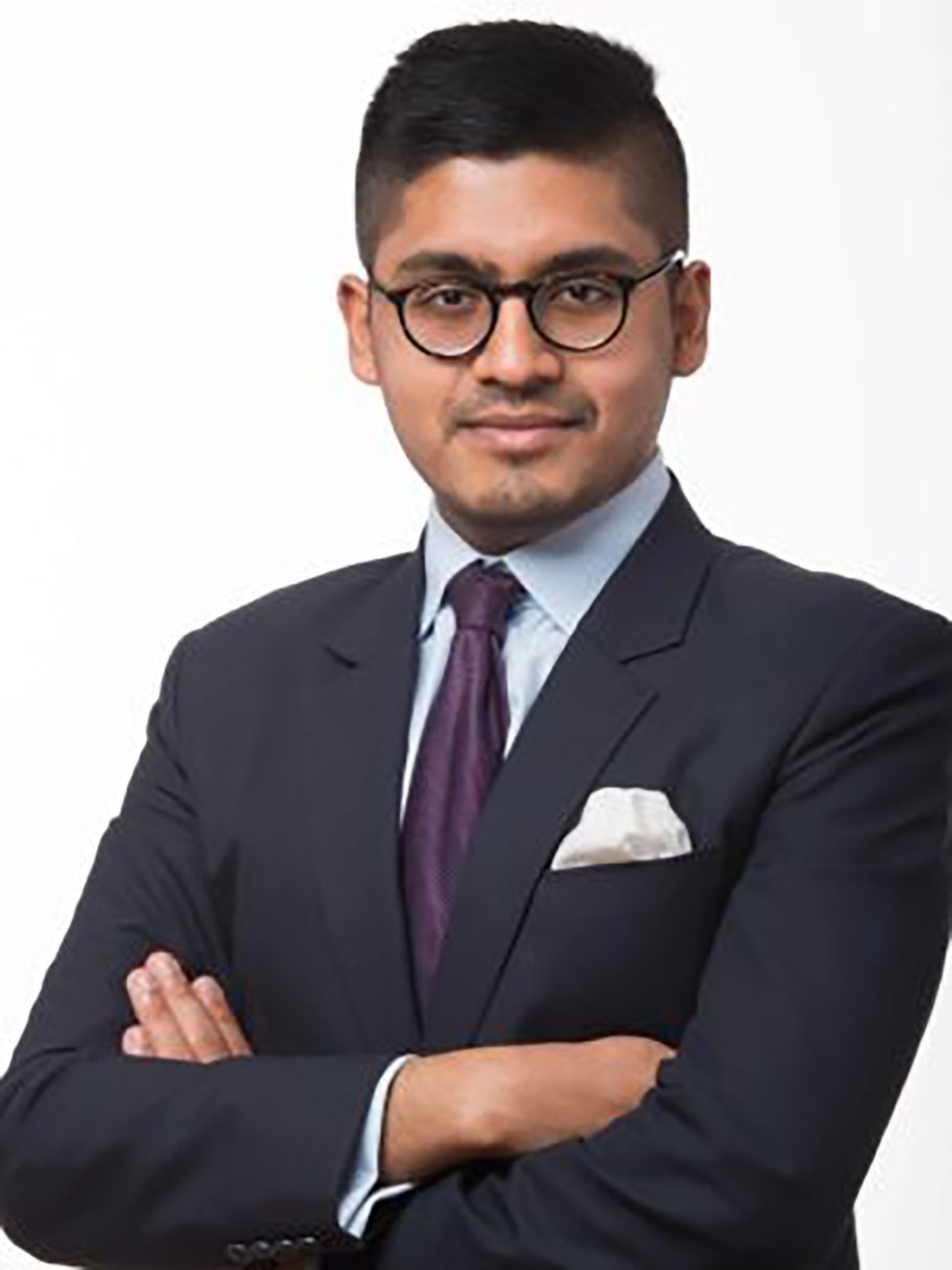






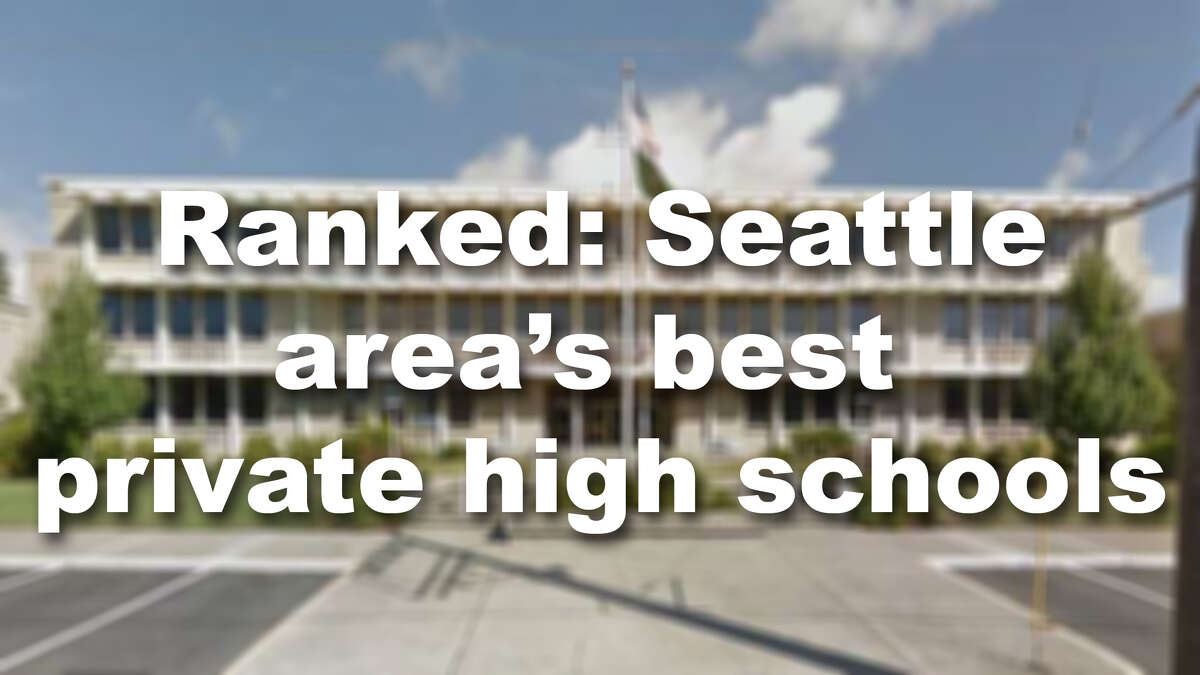


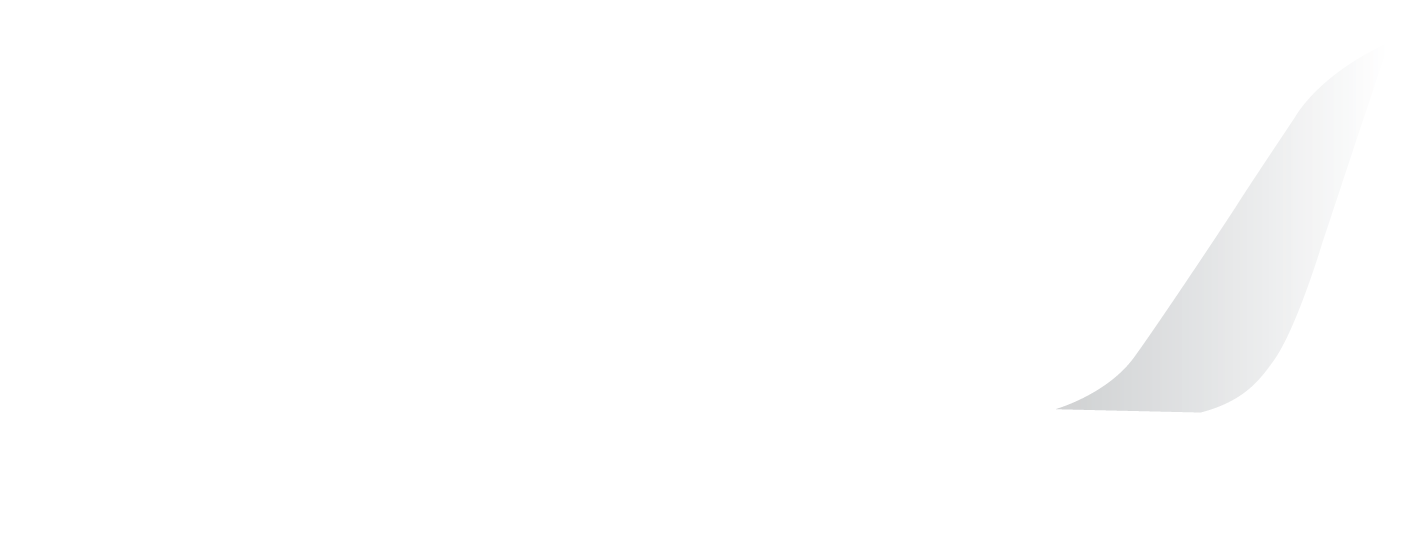



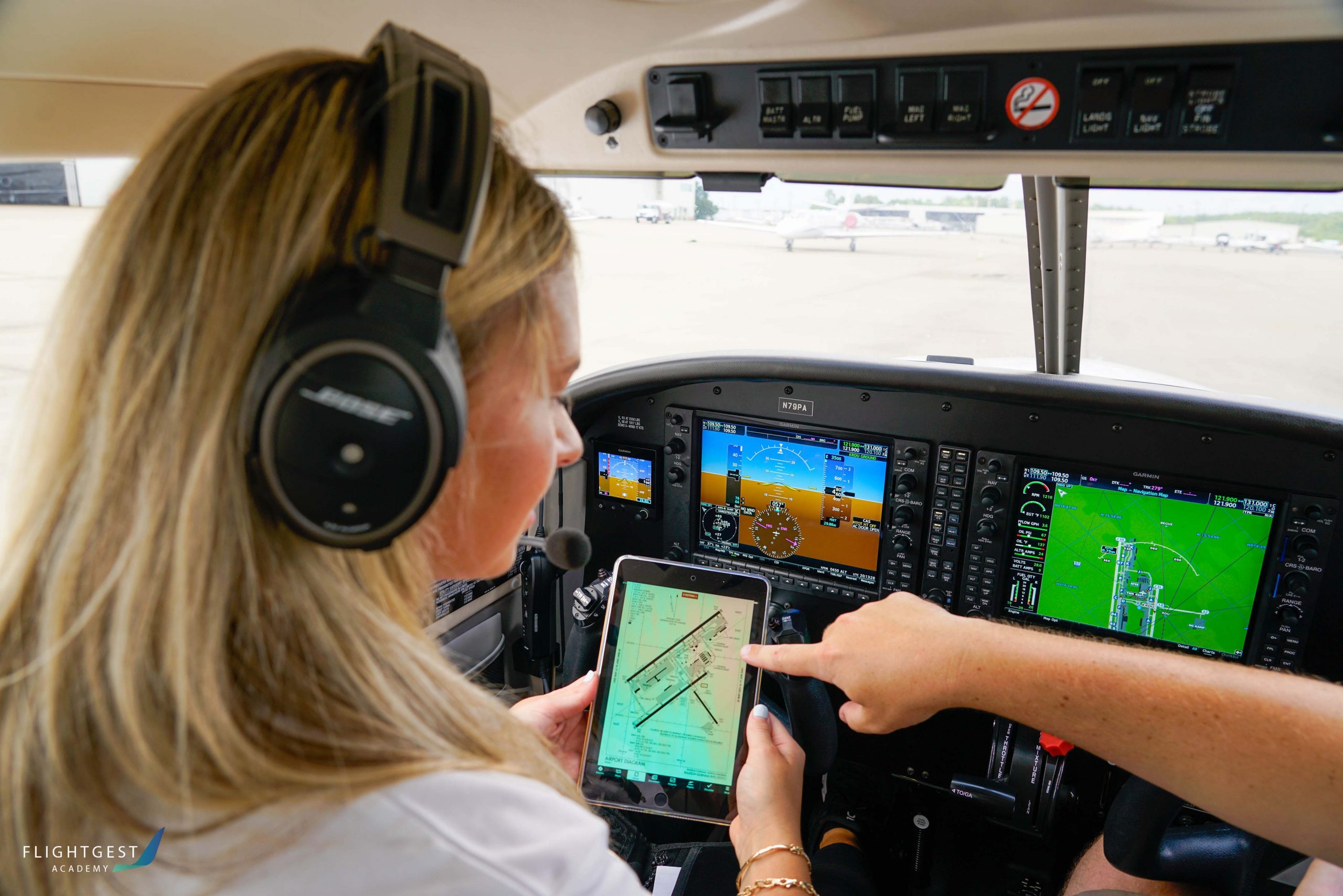
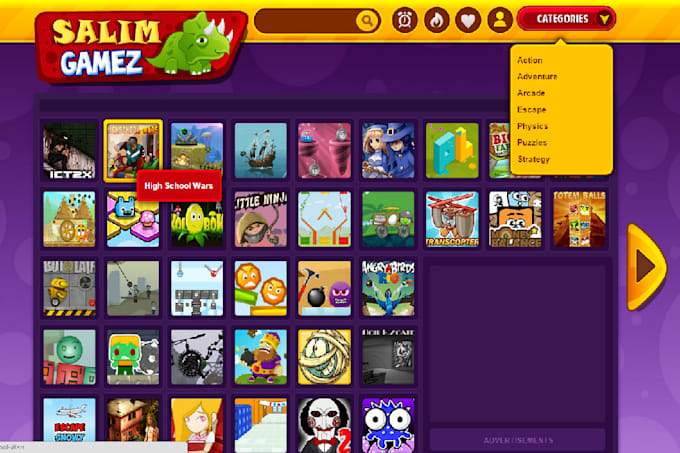









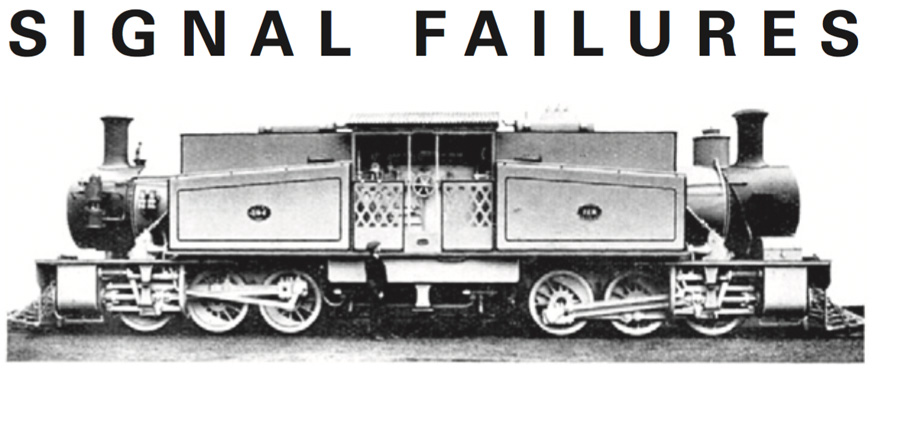
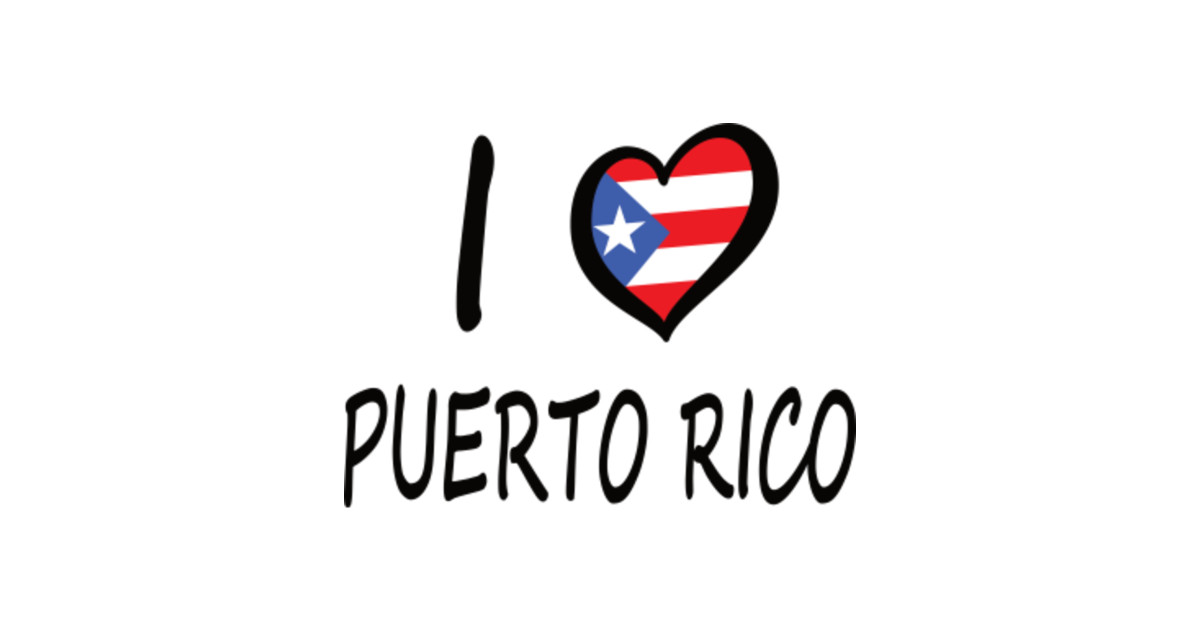

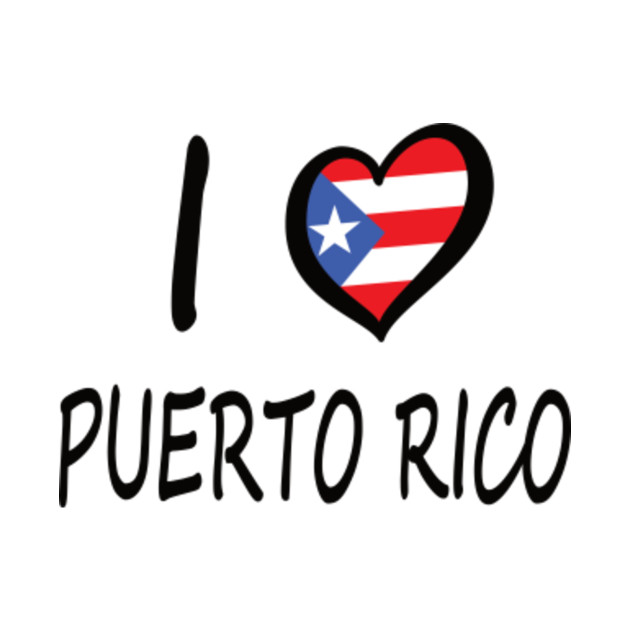




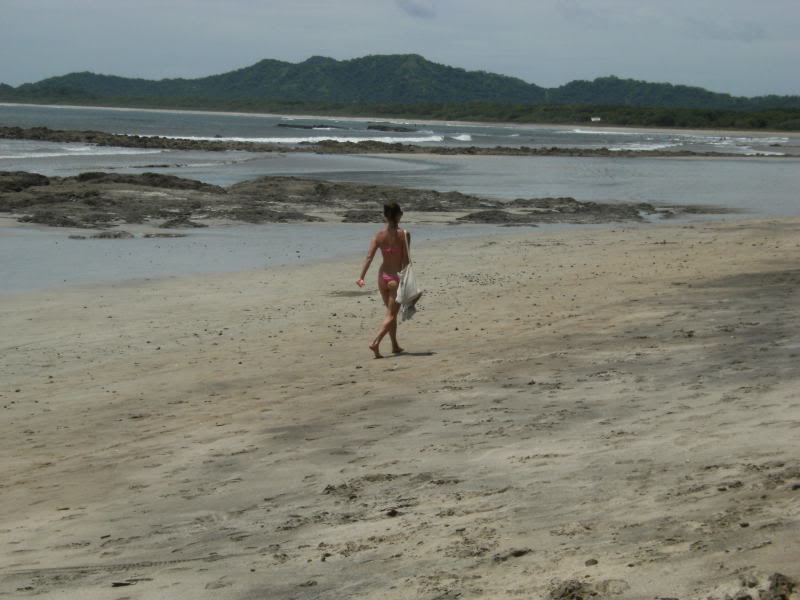




