Private Constructor
⚡ ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Private Constructor
.NET
Languages
C#
F#
Visual Basic
Workloads
Web
Mobile
Cloud
Desktop
Windows Presentation Foundation
Windows Forms
Universal Windows apps
Machine Learning & Data
ML.NET
.NET for Apache Spark
Entity Framework
APIs
.NET Core
.NET Framework
ASP.NET
ML.NET
Resources
What is .NET?
.NET Architecture Guides
Learning Materials
Downloads
Community
Support
Blog
More
Languages
C#
F#
Visual Basic
Workloads
Web
Mobile
Cloud
Desktop
Windows Presentation Foundation
Windows Forms
Universal Windows apps
Machine Learning & Data
ML.NET
.NET for Apache Spark
Entity Framework
APIs
.NET Core
.NET Framework
ASP.NET
ML.NET
Resources
What is .NET?
.NET Architecture Guides
Learning Materials
Downloads
Community
Support
Blog
Download .NET
Yes
No
A private constructor is a special instance constructor. It is generally used in classes that contain static members only. If a class has one or more private constructors and no public constructors, other classes (except nested classes) cannot create instances of this class. For example:
The declaration of the empty constructor prevents the automatic generation of a parameterless constructor. Note that if you do not use an access modifier with the constructor it will still be private by default. However, the private modifier is usually used explicitly to make it clear that the class cannot be instantiated.
Private constructors are used to prevent creating instances of a class when there are no instance fields or methods, such as the Math class, or when a method is called to obtain an instance of a class. If all the methods in the class are static, consider making the complete class static. For more information see Static Classes and Static Class Members .
The following is an example of a class using a private constructor.
Notice that if you uncomment the following statement from the example, it will generate an error because the constructor is inaccessible because of its protection level:
For more information, see Private constructors in the C# Language Specification . The language specification is the definitive source for C# syntax and usage.
Java - private constructor example
Private Constructors - C# Programming Guide | Microsoft Docs
Private Constructor in Java | How Private Constructor Works in Java?
What is the use of private constructor in java? | Code Pumpkin
Java: Private Constructor - BenchResources.Net
Java Training (40 Courses, 29 Projects, 4 Quizzes) 40 Online Courses | 29 Hands-on Projects | 285+ Hours | Verifiable Certificate of Completion | Lifetime Access | 4 Quizzes with Solutions 4.8 (8,152 ratings)
Course Price $129 $599 View Course
In java “Private” denotes within the class and the “Constructor” denotes a method of the class which has the same name as of the class name. Hence private constructor is a method of the class which is only accessible inside the class, not from outside the class.
For a Private Constructor in Java, you should follow the basic syntax of java with annotations. No other specific syntax is required to declare private constructor, just specify “private” before the name of the constructor.
When we specify the access specifier of a constructor as private, we call that private constructor. Therefore as per the access specifier rule, a private member (here constructor) cannot be accessed outside the class. Then you will have a definite question what is the benefit of doing such restrictions?
The answer is to serve the Singleton Class pattern. A singleton class is a type of class where the number of the object is limited to only one. Therefore, by using private constructor, we will ensure that only one object can be created and not more than that. That single object can only be created inside the class only as the access specifier is denoted as private. Now the obvious question arises: how practical is this? The answer is: in case of database connectivity, networking connectivity we will use this concept ensuring the single connection of a particular object. Singleton class is obviously a private class.
The next question to follow is: how we can access the private constructors? To resolve this, we need to create a public method inside the singleton class. Inside that public method, we will create an object as the method is local to the singleton class. Then we can call the public method outside the singleton class and eventually get the object.
We will discuss some code examples of private constructors here. I advise you to read the code line by line as I have mentioned inline comments in code to get better readability and understanding.
In this example, you will see, when a constructor is specified as private, then we cannot create its object outside the class. If we do, then it will give an error as you will see in the output,
class PrivateConstructorDemo
{
//private constructor
private PrivateConstructorDemo(){ }
public void show()
{
System.out.println("This is demo of Private Constructor");
}
}
public class MainClass
{
public static void main(String args[])
{
//Creating the object for the PrivateConstructorDemo class using private constructor
PrivateConstructorDemo obj = new PrivateConstructorDemo();
obj.show();
}
}
To resolve this problem we will create an object inside the public method of the same class and then will call that public method to get an object. Check the below code.
class PrivateConstructorDemo
{
private static PrivateConstructorDemo privateConstructorDemoObj = null;
private PrivateConstructorDemo(){ } //this is a private constructor which is why it is preventing object creation outside this class
public static PrivateConstructorDemo getPrivateConstructorDemo() //this is public method inside this class which we can create only one object
{
if(privateConstructorDemoObj == null)
{
privateConstructorDemoObj = new PrivateConstructorDemo(); //object is created inside public method of singleton class
}
return privateConstructorDemoObj;
}
public void show()
{
System.out.println("This is demo of Private Constructor");
}
}
public class MainClass
{
public static void main(String args[])
{
//Creating the object for the PrivateConstructorDemo class by calling public method of the class inside which object is created
PrivateConstructorDemo obj = PrivateConstructorDemo.getPrivateConstructorDemo();
obj.show();
}
}
Here we will take the example of singleton class and see how the number of object creation will be limited to one only even if we create multiple objects.
import java.io.*;
class MySingletonExample
{
static MySingletonExample instance = null;
public int nm = 16;
private MySingletonExample() { } //this is private constructor
static public MySingletonExample getObject() //this is public method inside which we will create object which can be accessed from outside class by calling this public method
{
if (instance == null)
instance = new MySingletonExample(); //creation object of singleton class
return instance;
}
}
public class MainClass
{
public static void main(String args[])
{
MySingletonExample obj1 = MySingletonExample.getObject(); //calling public method to get the object of the singleton class
MySingletonExample obj2 = MySingletonExample.getObject();//calling public method to get the object of the singleton class
obj1.nm = obj1.nm + 26;
System.out.println("object 1 has value = " + obj1.nm);
System.out.println("object 2 has value = " + obj2.nm);
}
}
When we changed the value of obj1, the value of obj2 also got updated because both ‘obj1’ and ‘obj2’ refer to the same object, which means, they are objects of a singleton class.
Let us take another example of run time polymorphism in case of multilevel inheritance . In this example, we will see how we can limit the number of object creation using the feature of the private constructor.
class ObjectCreationLimitDemo {
public static int objectCount = 0; //counter for instances
/**
* private constructor
* increases the objCount static variable on every object creation
*/
private ObjectCreationLimitDemo(){
objectCount++;
System.out.println("instance number " + objectCount + " is created");
}
/**
* static factory method to return LimitClass instance
* @return instance of LimitClass if not reached to threshold, else returns null
*/
public static synchronized ObjectCreationLimitDemo getLimInstance() {
if(objectCount < 5 ){
return new ObjectCreationLimitDemo();
}
System.out.println("Can not create new Object as you have reached maximum limit");
System.gc();
return null;
}
/**
* decreases the objCount static variable when JVM runs garbage collection
* and destroys unused instances
*/
@Override
public void finalize()
{
System.out.println("Instance is destroyed");
objectCount--;
}
}
public class MyObjectCreationLimitDemo {
public static void main(String[] args) {
ObjectCreationLimitDemo obj;
int iteration=1;
while(iteration<=20)
{
obj = ObjectCreationLimitDemo.getLimInstance();
iteration++;
}
}
}
The output sequence may change as per the allocation in JVM.
This concludes our learning of the topic “Private Constructor in Java”. Write yourself the codes mentioned in the above examples in the java compiler of your machine and verify the output. Learning of codes will be incomplete if you will not write code by yourself.
This is a guide to a Private Constructor in Java. Here we discuss the introduction and how private constructor works in java along with examples and code implementation. You may also look at the following articles to learn more –
Java Training (40 Courses, 29 Projects, 4 Quizzes)
Verifiable Certificate of Completion
© 2020 - EDUCBA. ALL RIGHTS RESERVED. THE CERTIFICATION NAMES ARE THE TRADEMARKS OF THEIR RESPECTIVE OWNERS.
This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy
Special Offer - Java Training (40 Courses, 29 Projects, 4 Quizzes) Learn More
Meet And Fuck Overwatch
M Private
Teen Self Pee
Private 39
Ass Fucked 2
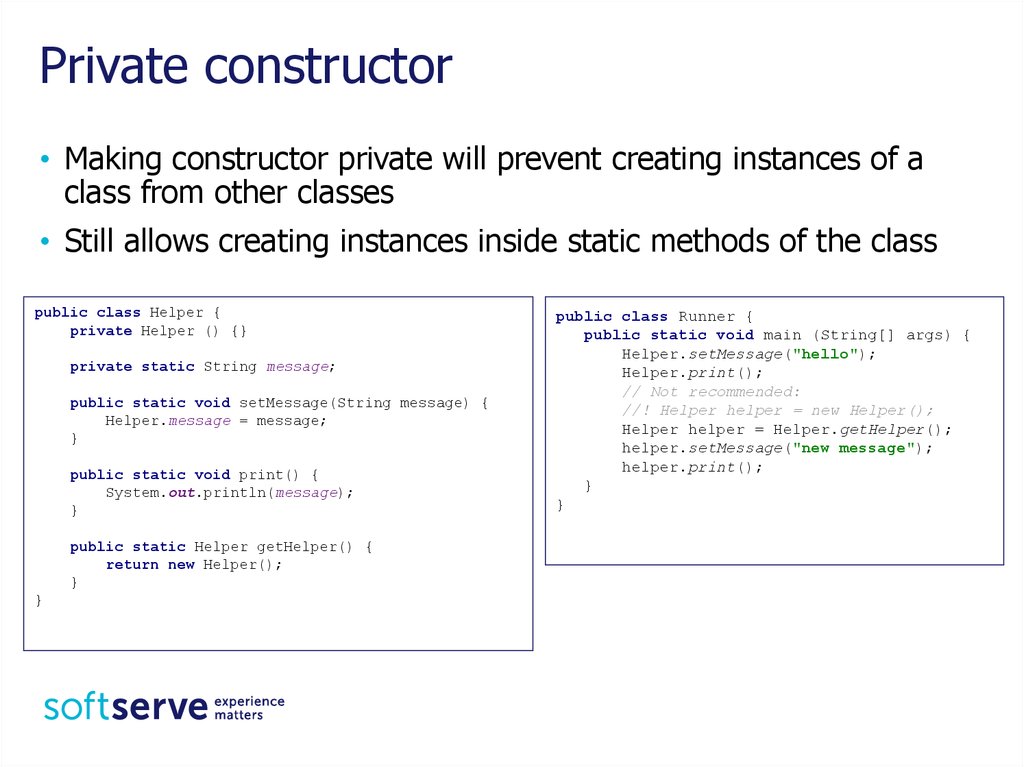
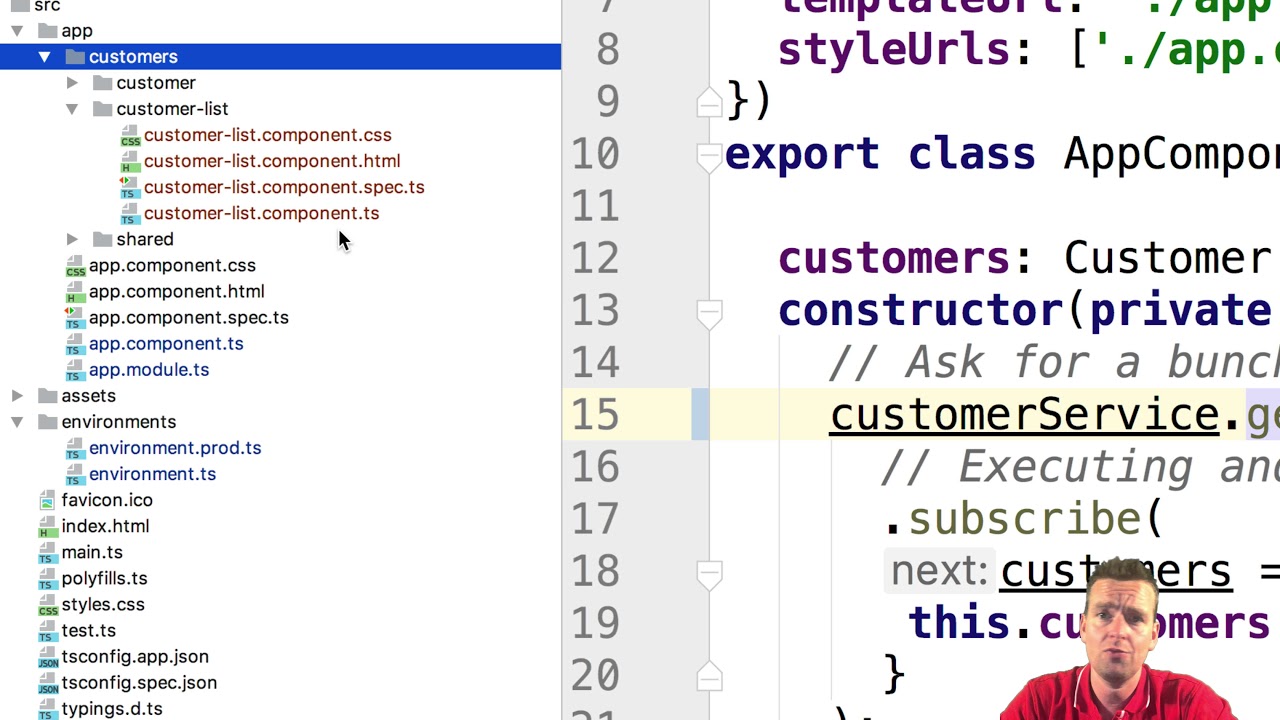
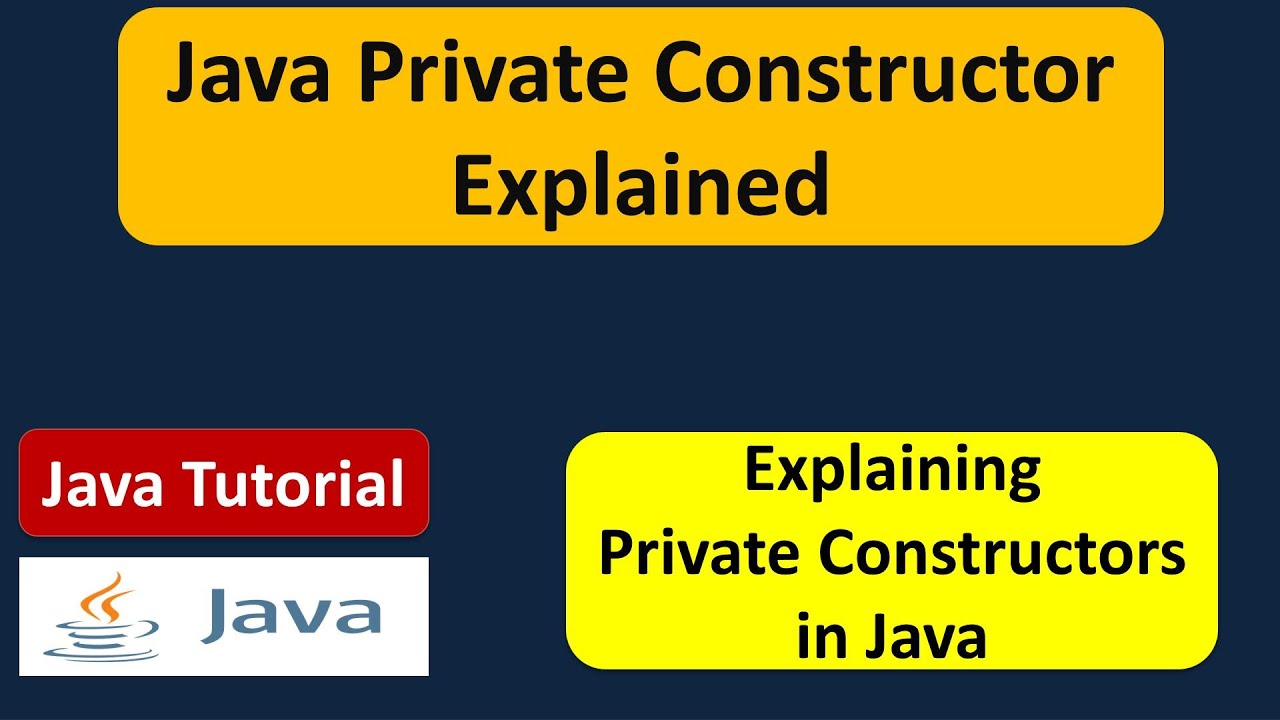
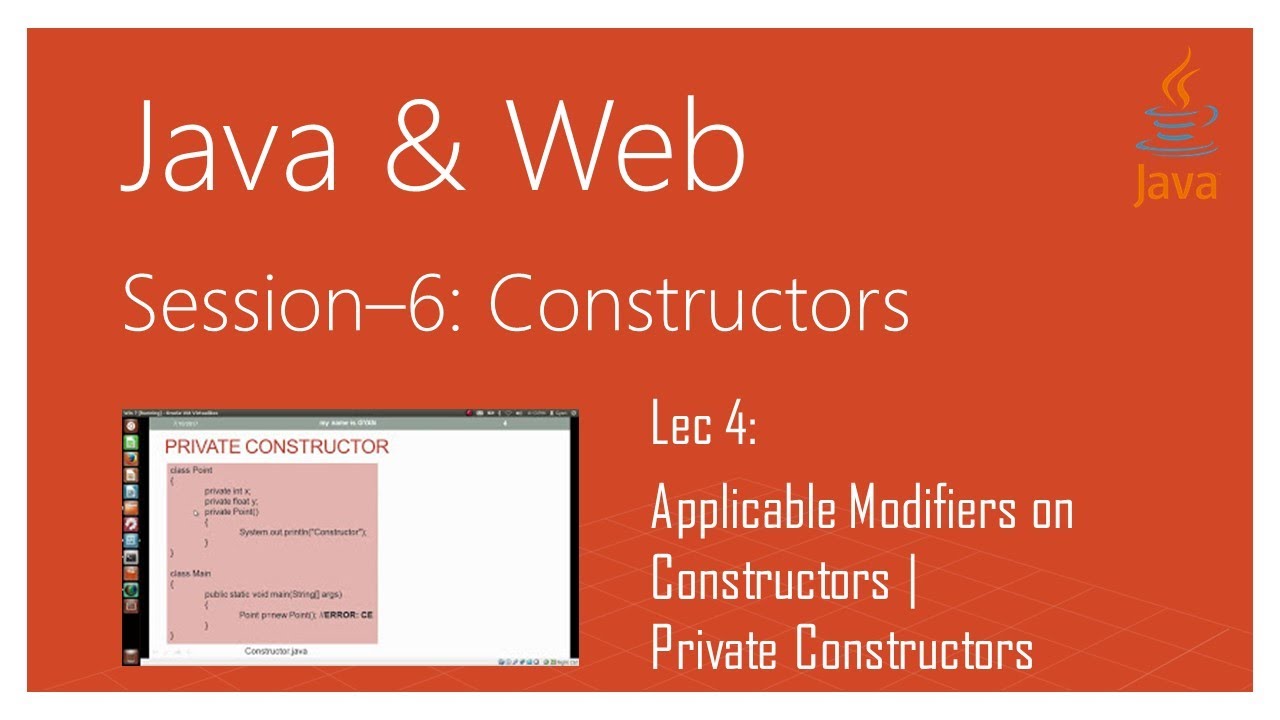
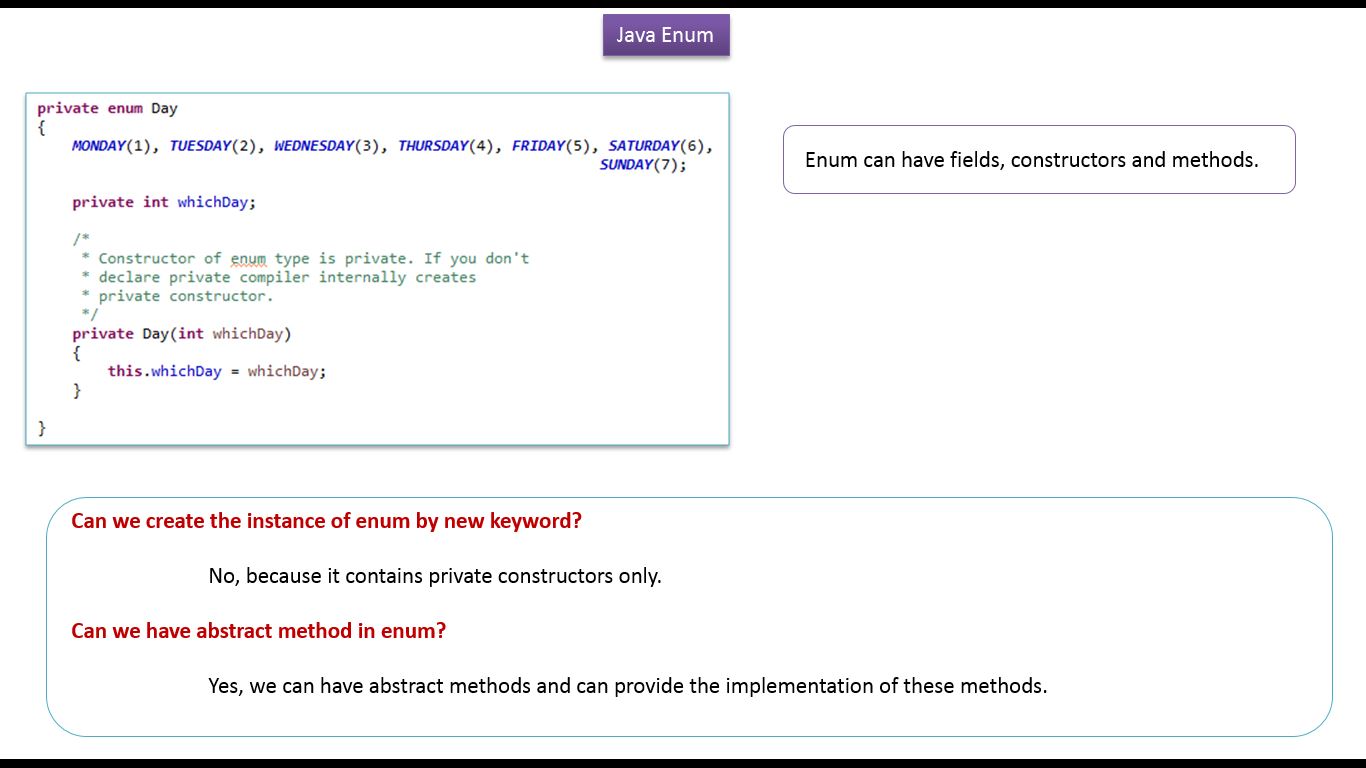
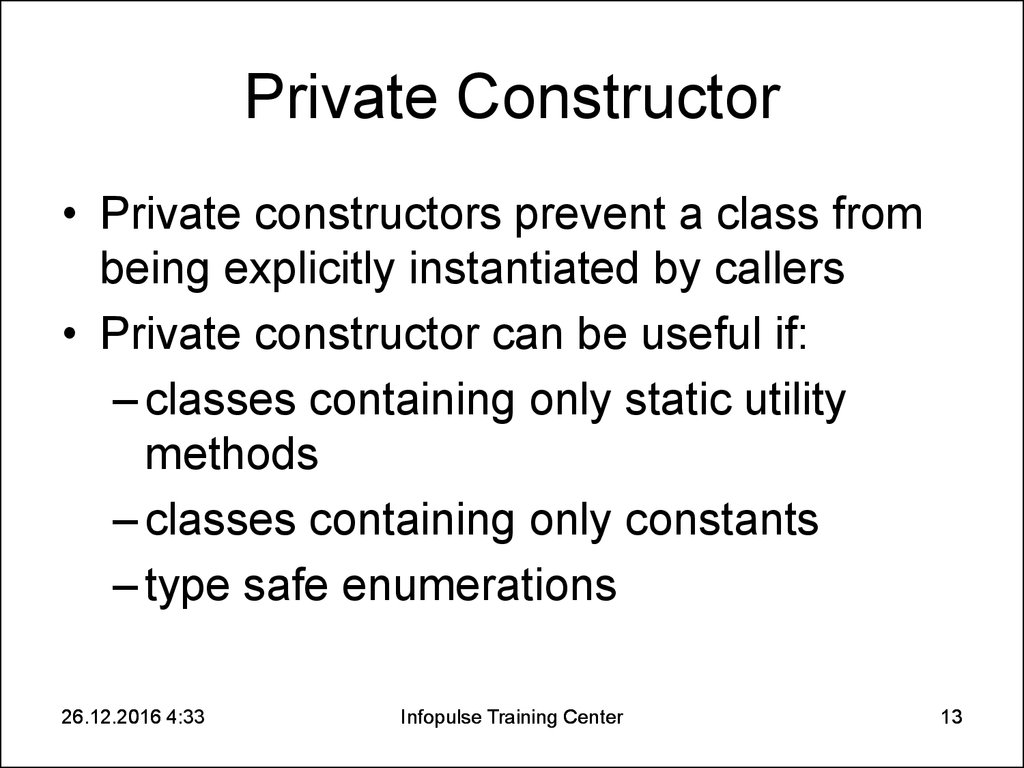


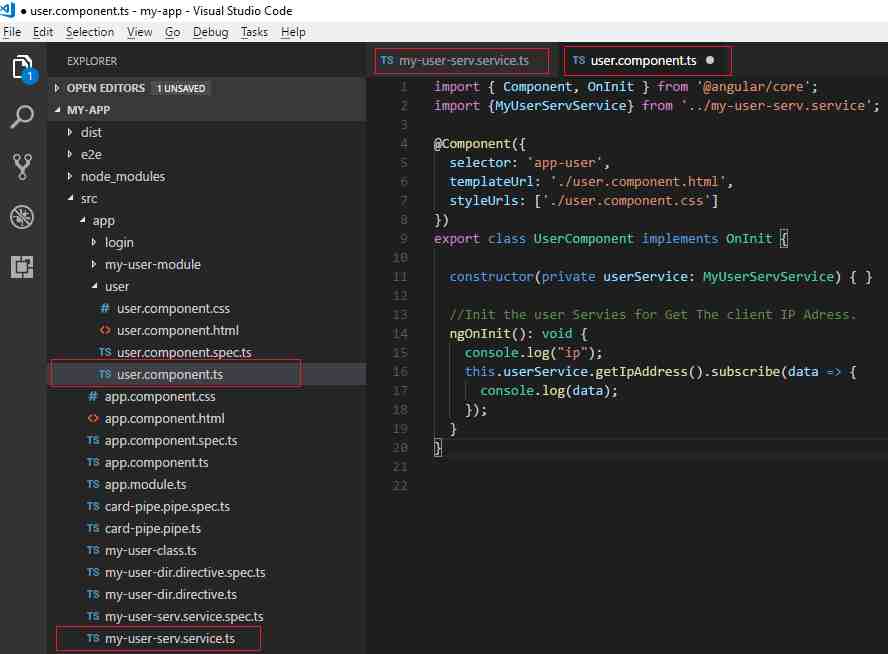



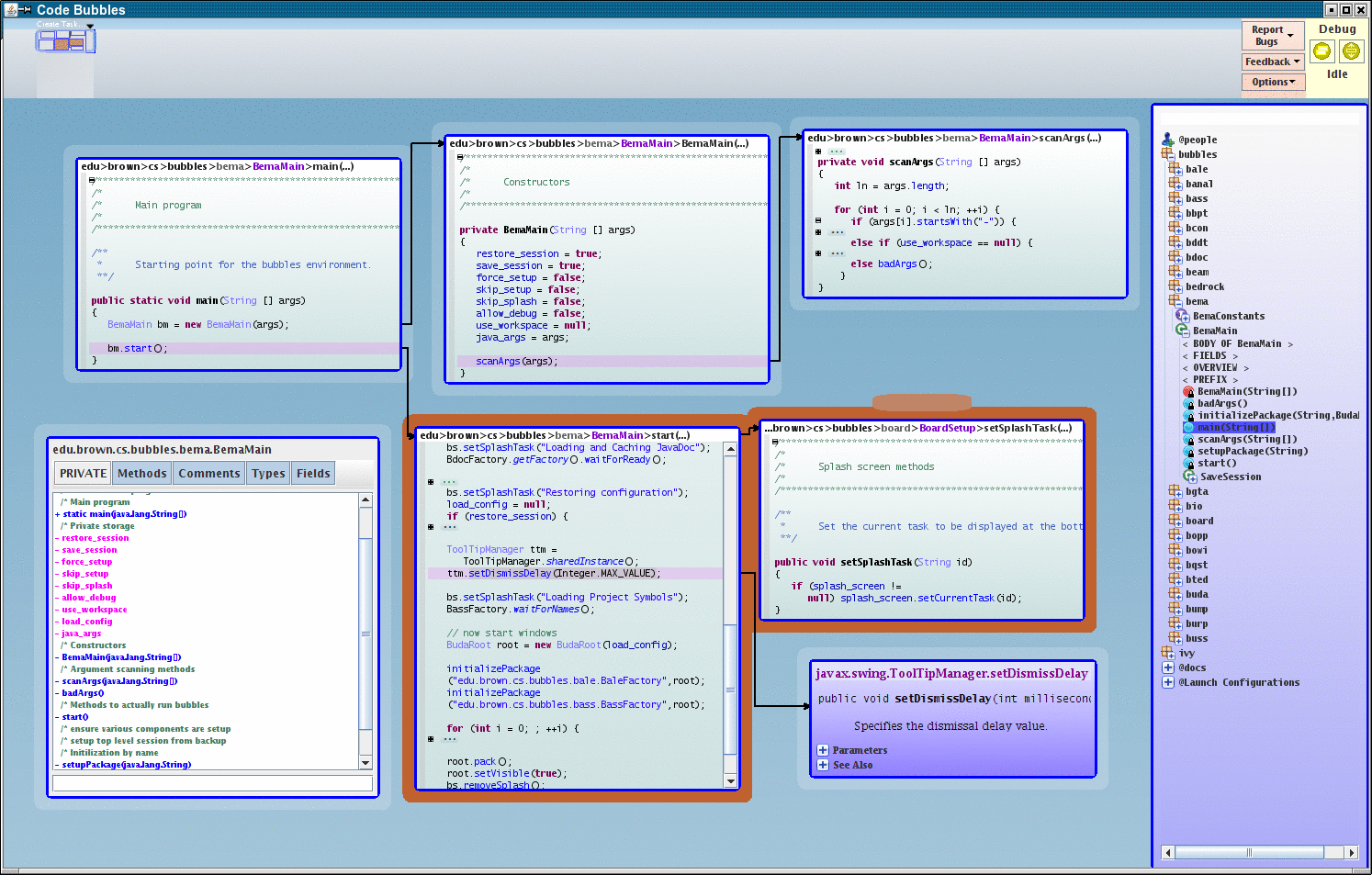

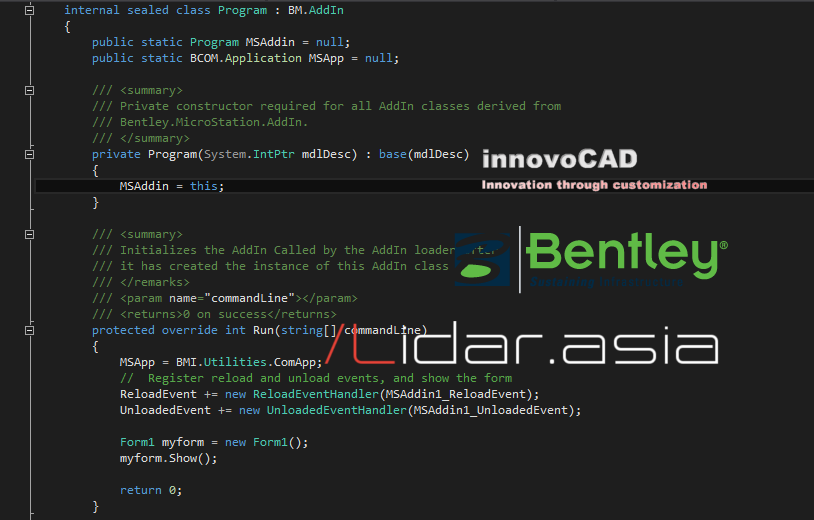


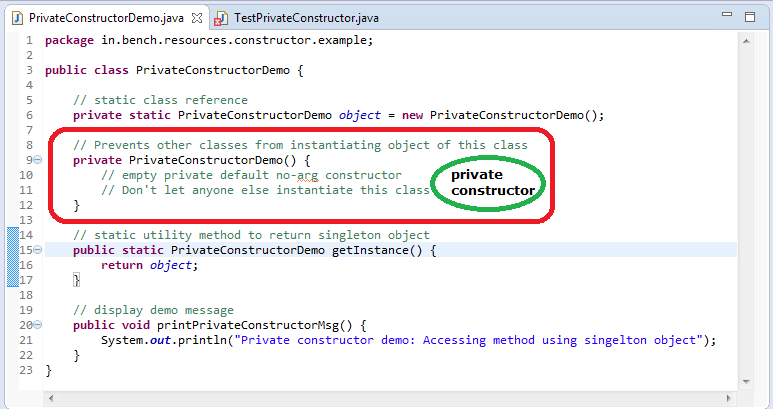
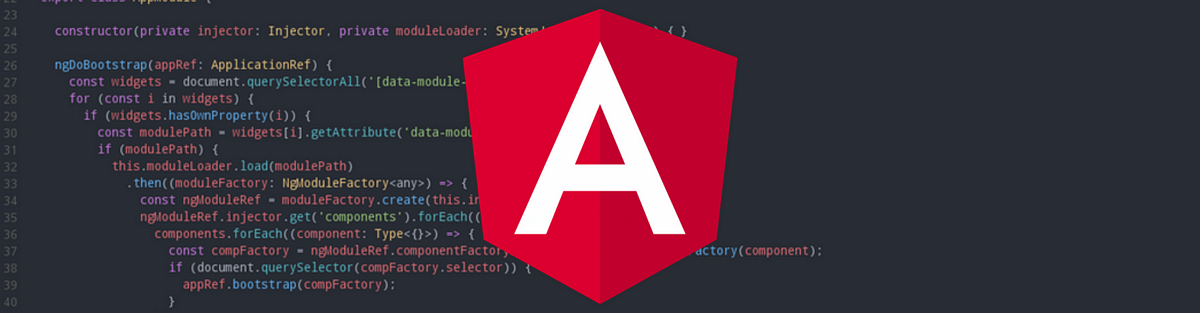
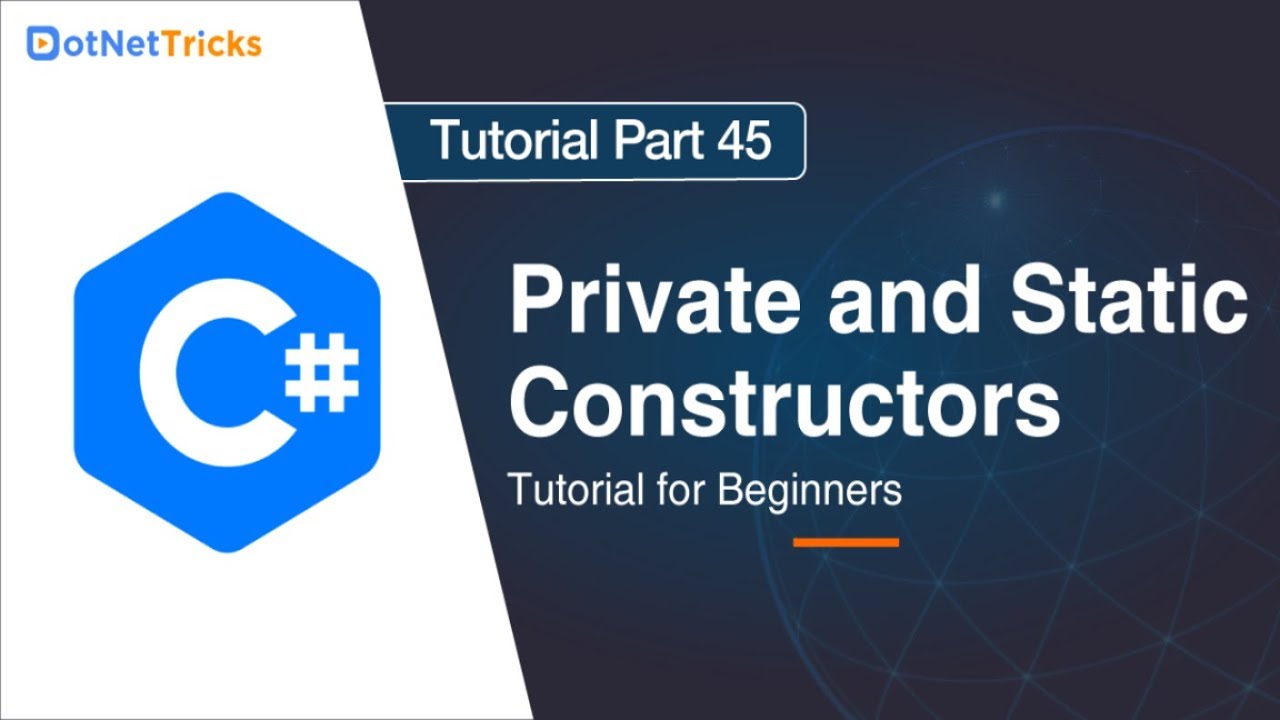

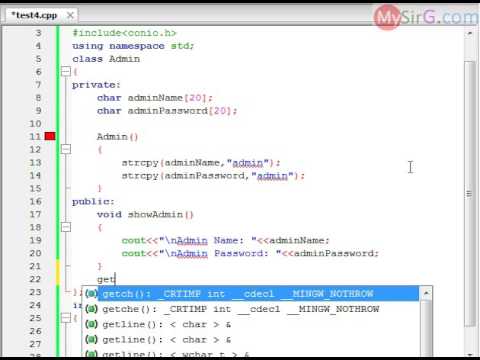
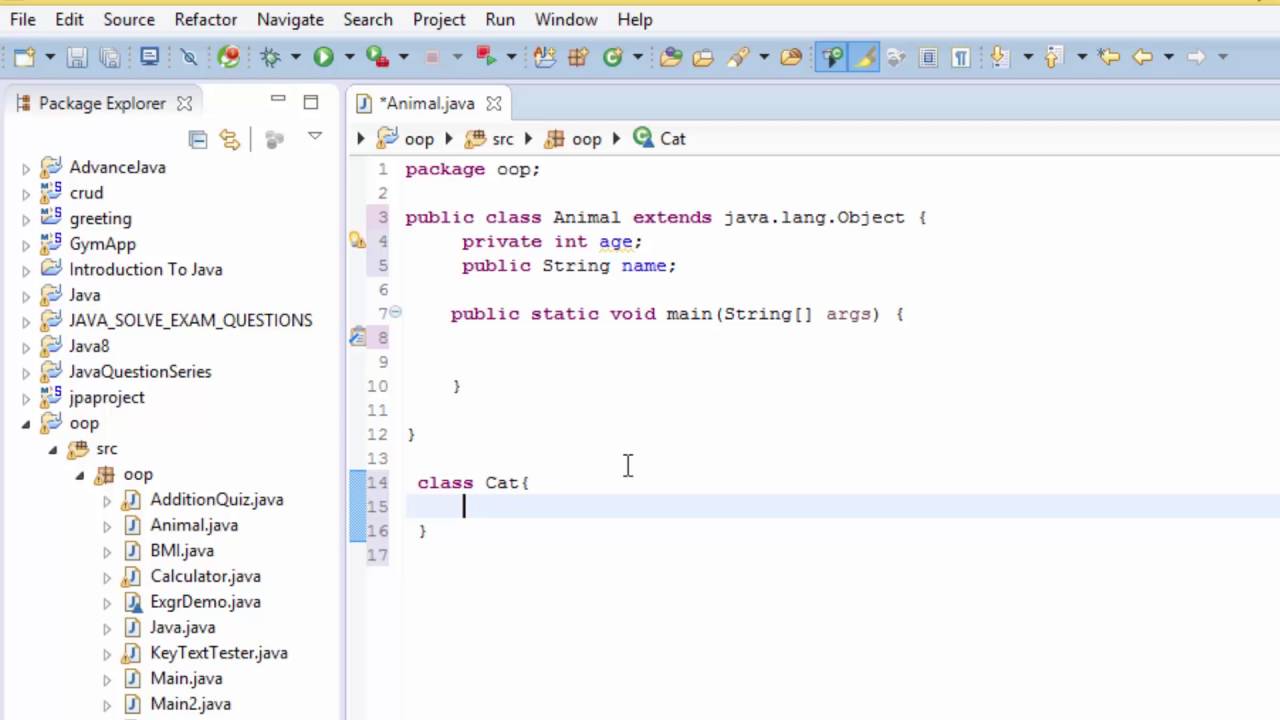

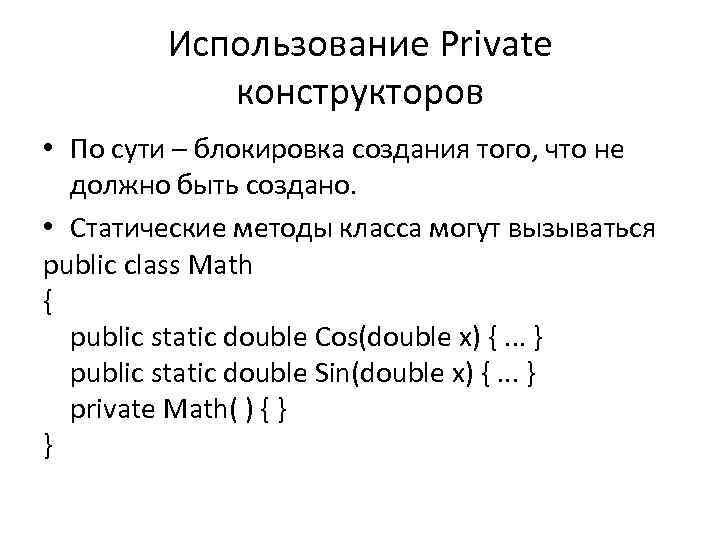
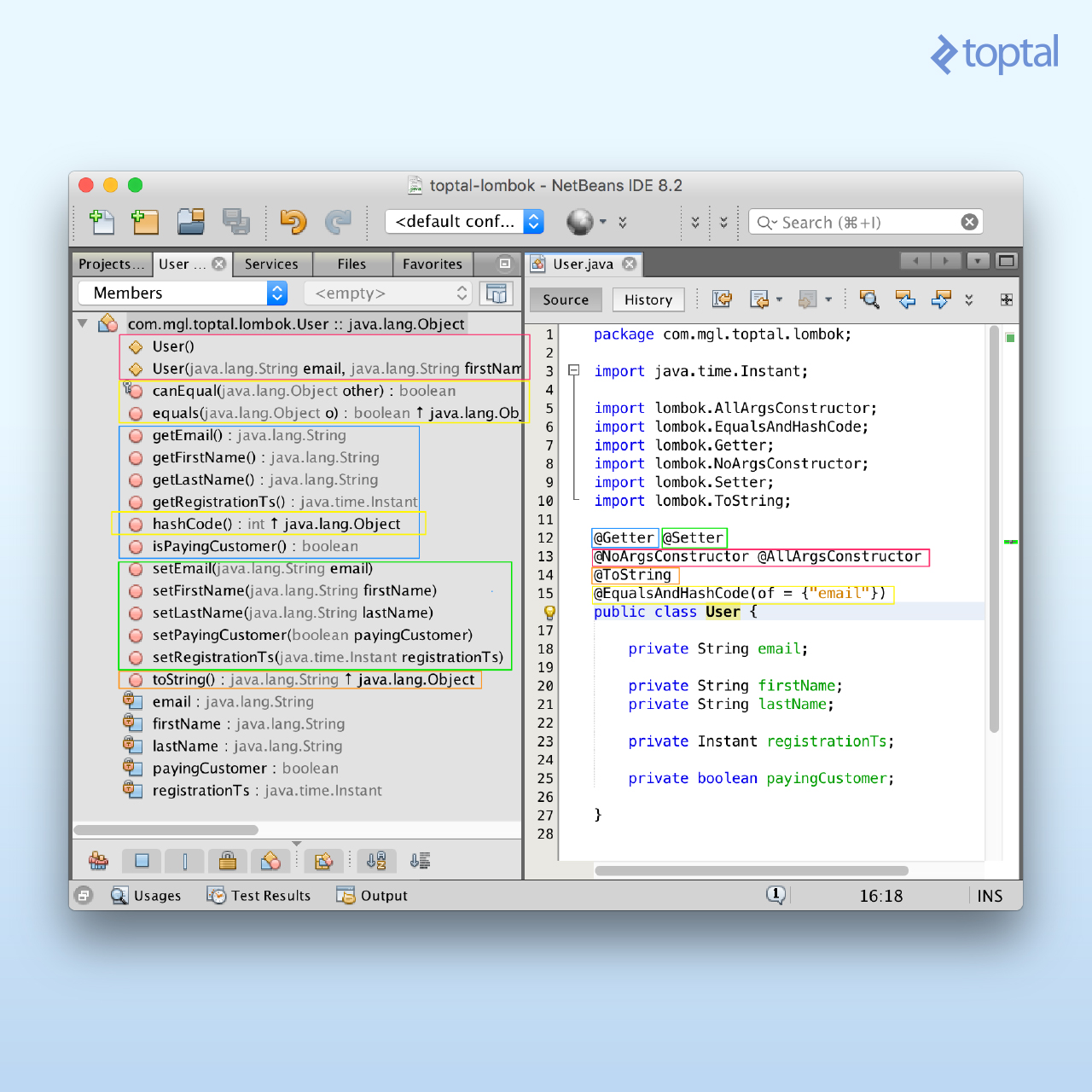



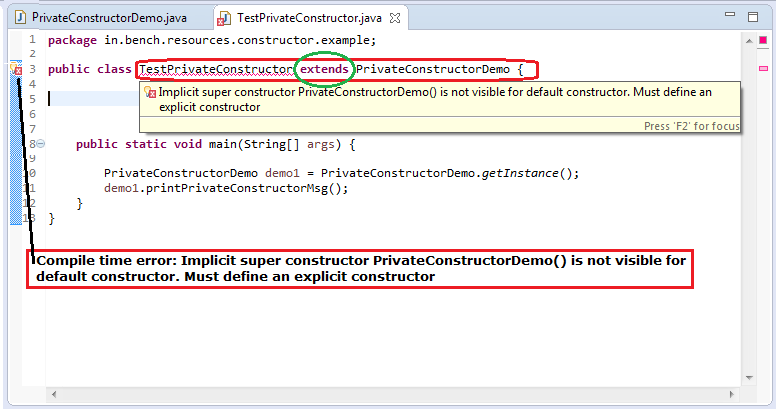


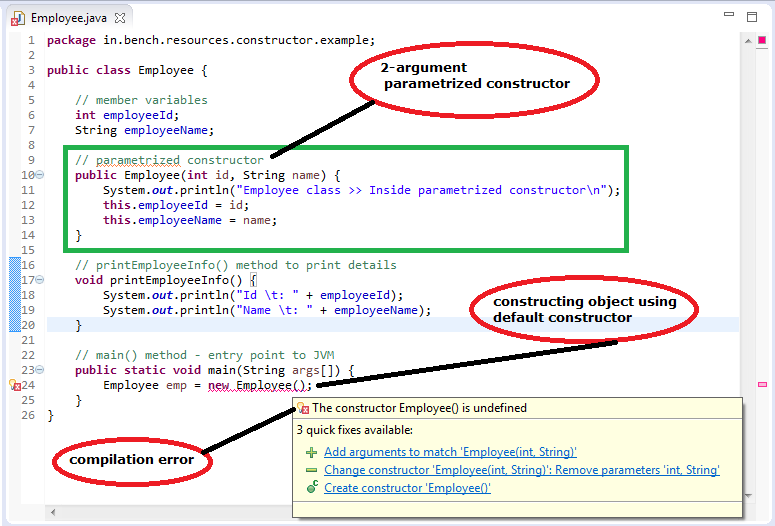


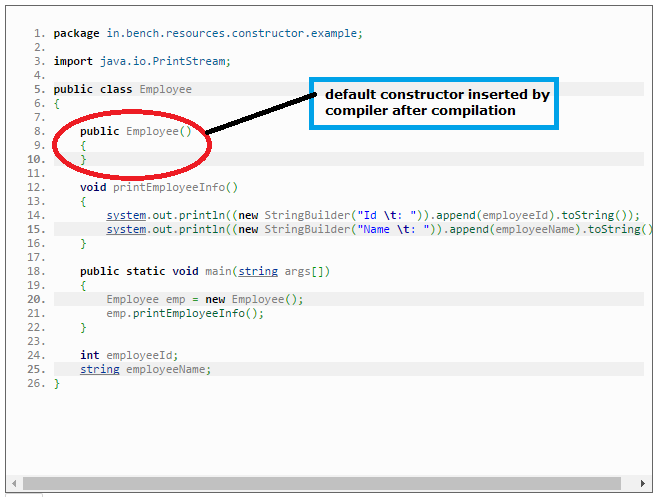
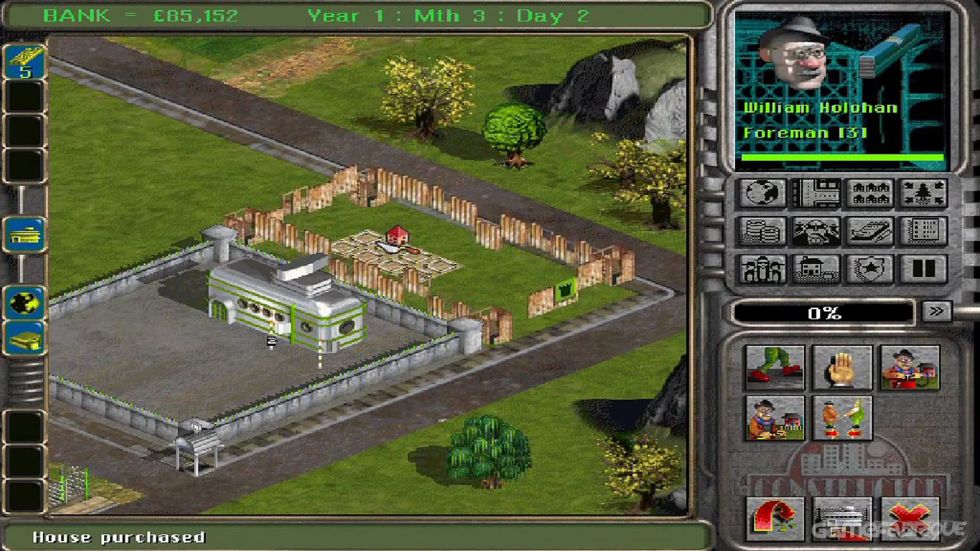

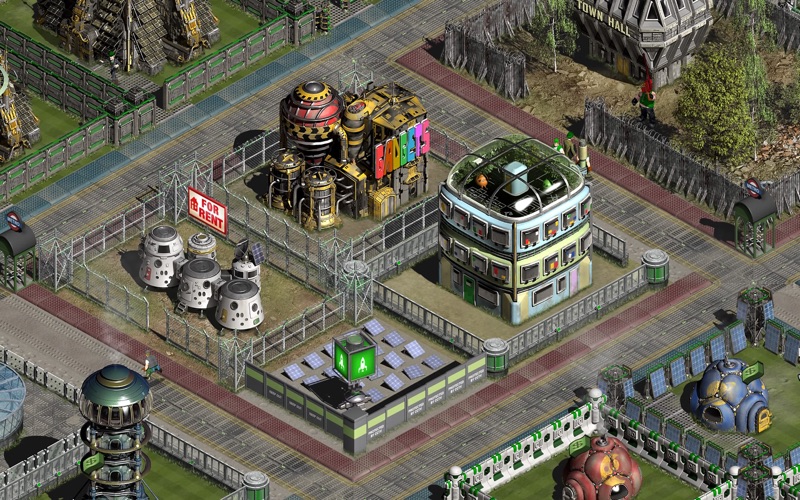
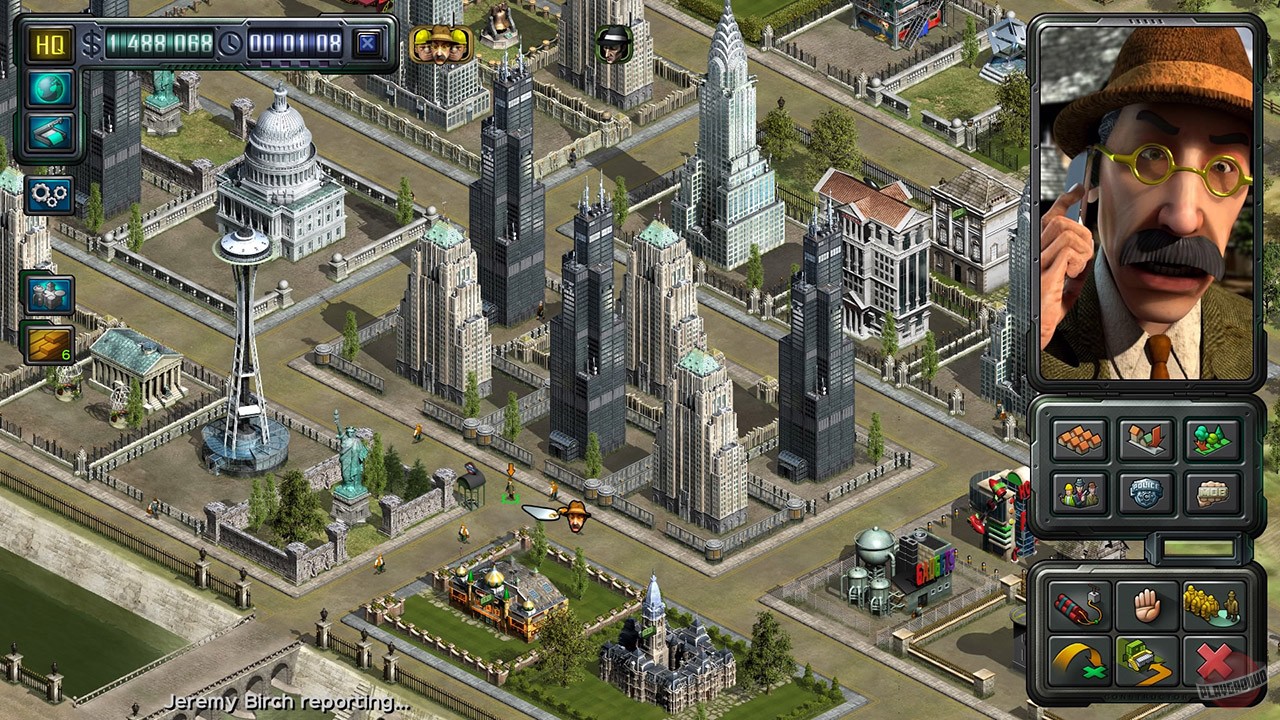
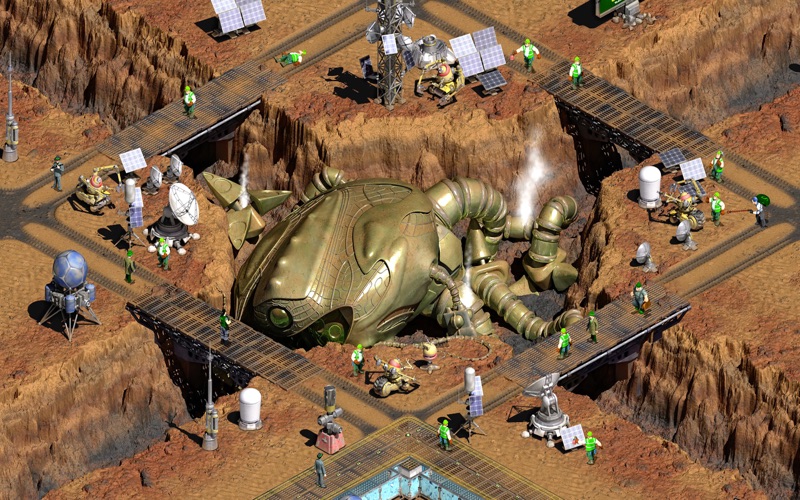

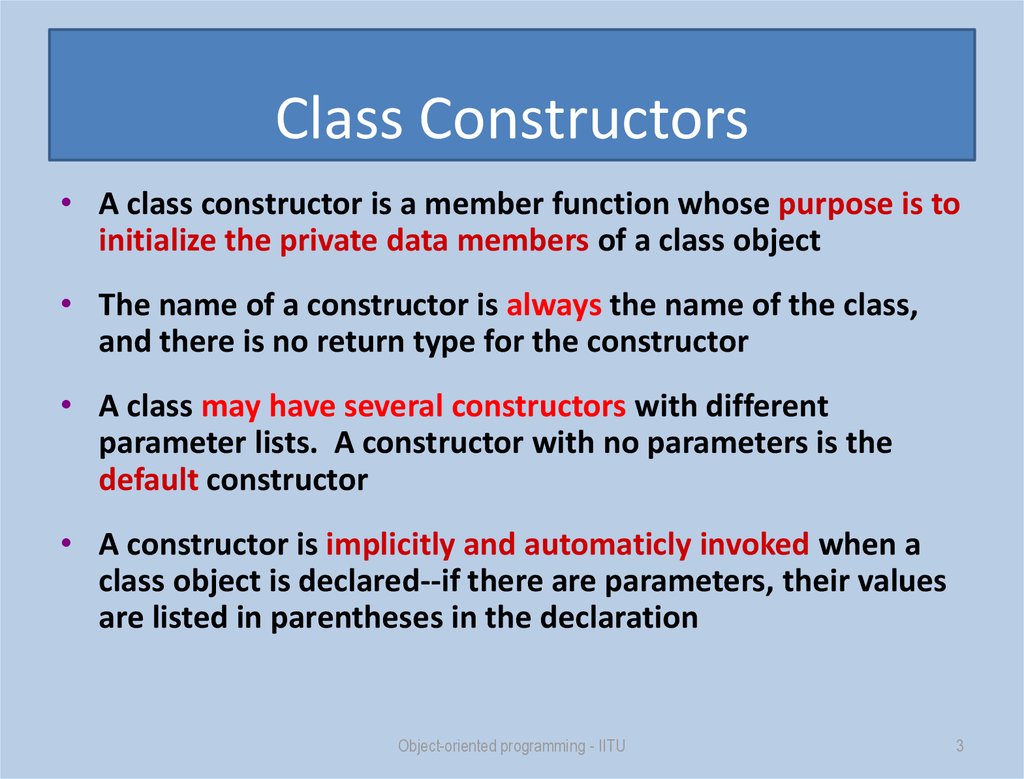
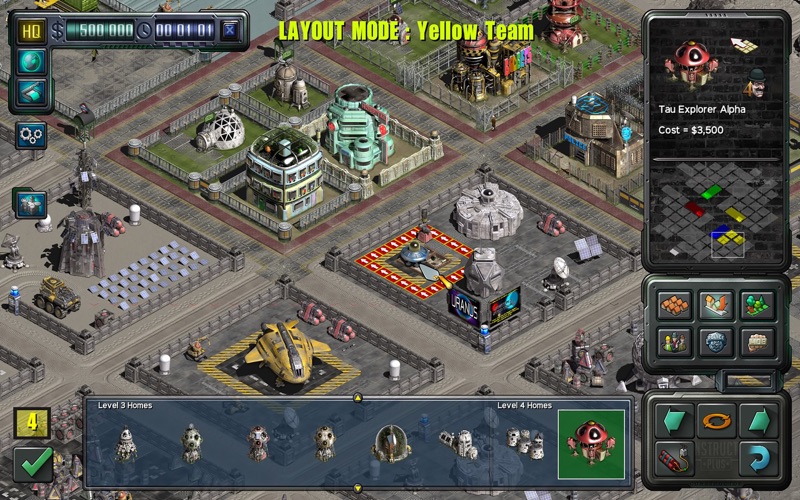
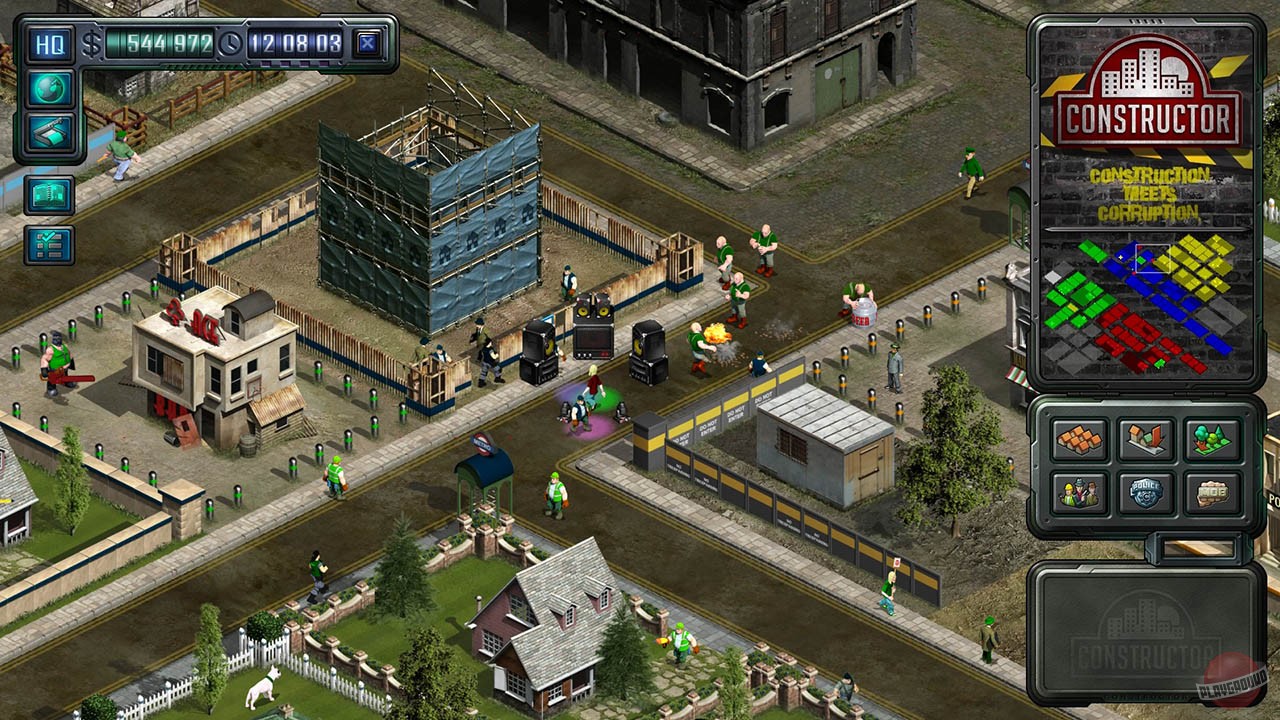
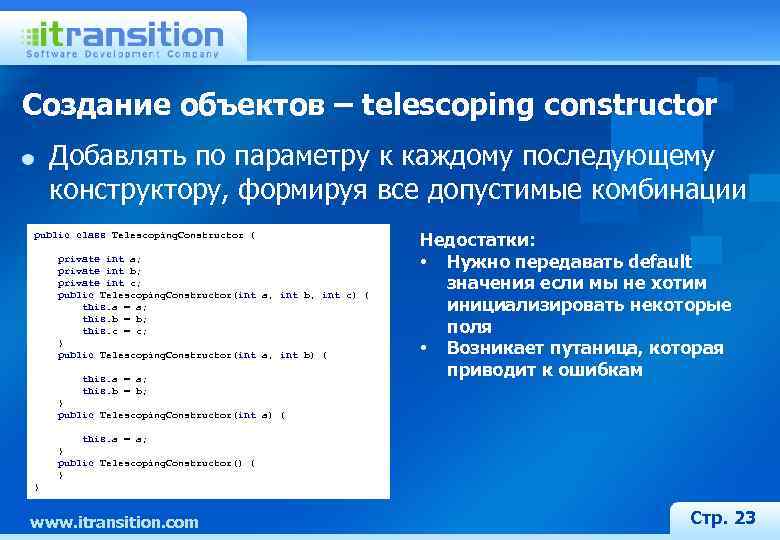
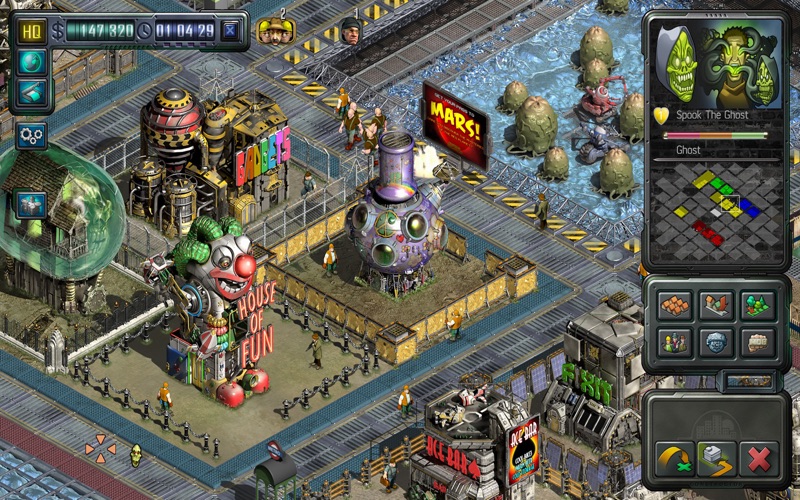
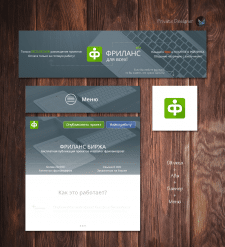
