Private Class Public Class
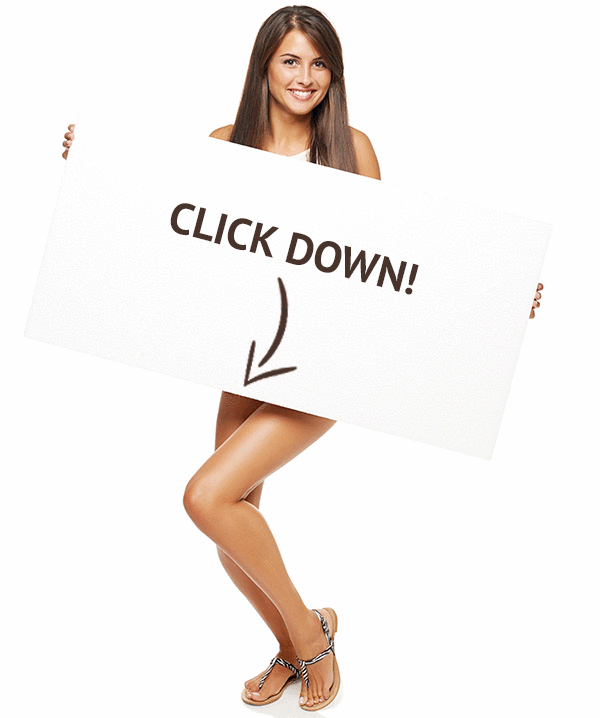
🔞 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Private Class Public Class
© Copyright 2022 W3schools.blog. All rights reserved. SiteMap
Yes, we can declare a class as private but these classes can be only inner or nested classes. We can’t a top-level class as private because it would be completely useless as nothing would have access to it.
private class Main
{
public static void main(String[] args) {
System.out.println("Inside private class");
}
}
Main.java:1: error: modifier private not allowed here
private class Main
^
1 error
private class Show{
void display(){
System.out.println("Inside display method.");
}
}
public class Main
{
public static void main(String[] args) {
Show show = new Show();
show.display();
}
}
Main.java:1: error: modifier private not allowed here
private class Show{
^
1 error
class Display {
//Private nested or inner class
private class InnerDisplay {
public void display() {
System.out.println("Private inner class method called");
}
}
void display() {
System.out.println("Outer class (Display) method called");
// Access the private inner class
InnerDisplay innerDisplay = new InnerDisplay();
innerDisplay.display();
}
}
public class Main { public static void main(String args[]) {
// Create object of the outer class (Display)
Display object = new Display();
// method invocation
object.display();
}
}
Outer class (Display) method called
Private inner class method called
private class Main
{
public static void main ( String [ ] args ) {
System . out . println ( "Inside private class" ) ;
}
}
Main. java : 1 : error : modifier private not allowed here
private class Main
^
1 error
private class Show {
void display ( ) {
System . out . println ( "Inside display method." ) ;
}
}
public class Main
{
public static void main ( String [ ] args ) {
Show show = new Show ( ) ;
show. display ( ) ;
}
}
Main. java : 1 : error : modifier private not allowed here
private class Show {
^
1 error
class Display {
//Private nested or inner class
private class InnerDisplay {
public void display ( ) {
System . out . println ( "Private inner class method called" ) ;
}
}
void display ( ) {
System . out . println ( "Outer class (Display) method called" ) ;
// Access the private inner class
InnerDisplay innerDisplay = new InnerDisplay ( ) ;
innerDisplay. display ( ) ;
}
}
public class Main {
public static void main ( String args [ ] ) {
// Create object of the outer class (Display)
Display object = new Display ( ) ;
// method invocation
object. display ( ) ;
}
}
Outer class ( Display ) method called
Private inner class method called
class ClassWithPrivateField {
#privateField ;
}
class ClassWithPrivateMethod {
#privateMethod ( ) {
return 'hello world' ;
}
}
class ClassWithPrivateStaticField {
static # PRIVATE_STATIC_FIELD ;
}
class ClassWithPrivateStaticMethod {
static #privateStaticMethod ( ) {
return 'hello world' ;
}
}
class ClassWithPrivateField {
#privateField ;
constructor ( ) {
this . #privateField = 42 ;
delete this . #privateField ; // Syntax error
this . #undeclaredField = 444 ; // Syntax error
}
}
const instance = new ClassWithPrivateField ( )
instance . #privateField === 42 ; // Syntax error
class ClassWithPrivateField {
#privateField ;
constructor ( ) {
this . #privateField = 42 ;
}
}
class SubClass extends ClassWithPrivateField {
#subPrivateField ;
constructor ( ) {
super ( ) ;
this . #subPrivateField = 23 ;
}
}
new SubClass ( ) ;
// SubClass {#subPrivateField: 23}
class ClassWithPrivateStaticField {
static # PRIVATE_STATIC_FIELD ;
static publicStaticMethod ( ) {
ClassWithPrivateStaticField . # PRIVATE_STATIC_FIELD = 42 ;
return ClassWithPrivateStaticField . # PRIVATE_STATIC_FIELD ;
}
publicInstanceMethod ( ) {
ClassWithPrivateStaticField . # PRIVATE_STATIC_FIELD = 42 ;
return ClassWithPrivateStaticField . # PRIVATE_STATIC_FIELD ;
}
}
console . log ( ClassWithPrivateStaticField . publicStaticMethod ( ) ) ; // 42
console . log ( new ClassWithPrivateStaticField ( ) . publicInstanceMethod ( ) ) ; // 42
class BaseClassWithPrivateStaticField {
static # PRIVATE_STATIC_FIELD ;
static basePublicStaticMethod ( ) {
this . # PRIVATE_STATIC_FIELD = 42 ;
return this . # PRIVATE_STATIC_FIELD ;
}
}
class SubClass extends BaseClassWithPrivateStaticField { } ;
let error = null ;
try {
SubClass . basePublicStaticMethod ( ) ;
} catch ( e ) {
error = e ;
}
console . log ( error instanceof TypeError ) ;
// true
console . log ( error ) ;
// TypeError: Cannot write private member #PRIVATE_STATIC_FIELD
// to an object whose class did not declare it
class ClassWithPrivateMethod {
#privateMethod ( ) {
return 'hello world' ;
}
getPrivateMessage ( ) {
return this . #privateMethod ( ) ;
}
}
const instance = new ClassWithPrivateMethod ( ) ;
console . log ( instance . getPrivateMessage ( ) ) ;
// hello world
class ClassWithPrivateAccessor {
#message ;
get #decoratedMessage ( ) {
return ` 🎬 ${ this . #message } 🛑 ` ;
}
set #decoratedMessage ( msg ) {
this . #message = msg ;
}
constructor ( ) {
this . #decoratedMessage = 'hello world' ;
console . log ( this . #decoratedMessage ) ;
}
}
new ClassWithPrivateAccessor ( ) ;
// 🎬hello world🛑
class ClassWithPrivateStaticMethod {
static #privateStaticMethod ( ) {
return 42 ;
}
static publicStaticMethod1 ( ) {
return ClassWithPrivateStaticMethod . #privateStaticMethod ( ) ;
}
static publicStaticMethod2 ( ) {
return this . #privateStaticMethod ( ) ;
}
}
console . log ( ClassWithPrivateStaticMethod . publicStaticMethod1 ( ) === 42 ) ;
// true
console . log ( ClassWithPrivateStaticMethod . publicStaticMethod2 ( ) === 42 ) ;
// true
class Base {
static #privateStaticMethod ( ) {
return 42 ;
}
static publicStaticMethod1 ( ) {
return Base . #privateStaticMethod ( ) ;
}
static publicStaticMethod2 ( ) {
return this . #privateStaticMethod ( ) ;
}
}
class Derived extends Base { }
console . log ( Derived . publicStaticMethod1 ( ) ) ;
// 42
console . log ( Derived . publicStaticMethod2 ( ) ) ;
// TypeError: Cannot read private member #privateStaticMethod
// from an object whose class did not declare it
Web technology reference for developers
Code used to describe document style
Protocol for transmitting web resources
Interfaces for building web applications
Developing extensions for web browsers
Web technology reference for developers
Learn to structure web content with HTML
Learn to run scripts in the browser
Learn to make the web accessible to all
Frequently asked questions about MDN Plus
Class fields are public by default, but private class members can be created
by using a hash # prefix. The privacy encapsulation of these class features is
enforced by JavaScript itself.
Private members are not native to the language before this syntax existed. In prototypical inheritance, its behavior may be emulated with WeakMap objects or closures , but they can't compare to the # syntax in terms of ergonomics.
Private fields include private instance fields and private static fields.
Private instance fields are declared with # names (pronounced
" hash names "), which are identifiers prefixed with # . The
# is a part of the name itself. Private fields are accessible on
the class constructor from inside the class
declaration itself. They are used for declaration of field names as well
as for accessing a field's value.
It is a syntax error to refer to # names from out of scope.
It is also a syntax error to refer to private fields
that were not declared before they were called, or to attempt to remove
declared fields with delete .
Note: Use the in operator to check for potentially missing private fields (or private methods). This will return true if the private field or method exists, and false otherwise.
Like public fields, private fields are added at construction time in a base class, or at the point where super() is invoked in a subclass.
Note: #privateField from the ClassWithPrivateField base class is private to ClassWithPrivateField and is not accessible from the derived Subclass .
Private static fields are added to the class constructor at class evaluation time. Like their public counterparts, private static fields are only accessible on the class itself or on the this context of static methods, but not on the this context of instance methods.
There is a restriction on private static fields: Only the class which
defines the private static field can access the field. This can lead to unexpected behavior when using this .
In the following example, this refers to the SubClass class (not
the BaseClassWithPrivateStaticField class) when we try to call
SubClass.basePublicStaticMethod() , and so causes a TypeError .
Private instance methods are methods available on class instances whose access is
restricted in the same manner as private instance fields.
Private instance methods may be generator, async, or async generator functions. Private
getters and setters are also possible, although not in generator, async, or
async generator forms.
Like their public equivalent, private static methods are called on the class itself,
not instances of the class. Like private static fields, they are only accessible from
inside the class declaration.
Private static methods may be generator, async, and async generator functions.
The same restriction previously mentioned for private static fields holds
for private static methods, and similarly can lead to unexpected behavior when using
this .
In the following example, when we try to call Derived.publicStaticMethod2() ,
this refers to the Derived class (not
the Base class) and so causes a TypeError .
BCD tables only load in the browser
Last modified: Aug 8, 2022 , by MDN contributors
Your blueprint for a better internet.
Visit Mozilla Corporation’s not-for-profit parent, the Mozilla Foundation . Portions of this content are ©1998– 2022 by individual mozilla.org contributors. Content available under a Creative Commons license .
For Videos Join Our Youtube Channel: Join Now
Like/Subscribe us for latest updates or newsletter
In Java, public and private are keywords that are known as an access modifier or specifier . It restricts the scope or accessibility of a class, constructor , variables , method s, and data members. It depends on which it is applied. Java provides the four types of access modifiers: public, private, protected , and default . But in this section, we will discuss only two public and private, and also discuss the difference between public and private access specifier with example.
Access modifiers control whether other classes can use a particular field or invoke a particular method. Java provides two levels of access control:
The following table shows the access level to members permitted by the public and private modifiers.
The following table describes the visibility of the classes if we make the classes public and private one by one. In the above figure the Demo1 is the only class that is visible for each access modifiers.
It can be specified by using the public keyword. Its scope or accessibility is the widest among other access specifiers. The variables, classes, and methods declared as public can be accessed from everywhere in the program. It does not impose restrictions on the scope of public data members. If we declare methods and classes as public, they also violate the principle of encapsulation . We can also use it with the top-level classes.
Let's use the private access specifier in a Java program for better understanding.
Let's use the private access specifier in a Java program for better understanding.
In the following example, we have declared two classes: Demo1 and Demo2. In the class Demo1, we have defined a method show() as private. The class Demo2 contains the main() method in which we have created an object of the class Demo1. After that, we are trying to access the private method of the class Demo1 from the class Demo2, that is not possible. But still, we will execute the program to see which error it shows.
When we execute the above program, it shows the following error:
The major difference between public and private modifiers is its visibility. Java categories the visibility for class members as follows:
JavaTpoint offers too many high quality services. Mail us on hr@javatpoint.com , to get more information about given services.
JavaTpoint offers college campus training on Core Java, Advance Java, .Net, Android, Hadoop, PHP, Web Technology and Python. Please mail your requirement at hr@javatpoint.com. Duration: 1 week to 2 week
Contact No: 0120-4256464, 9990449935
© Copyright 2011-2021 www.javatpoint.com. All rights reserved. Developed by JavaTpoint.
Downloads
Visual Studio
SDKs
Trial software
Free downloads
Office resources
Programs
Subscriptions
Overview
Administrators
Students
Microsoft Imagine
Microsoft Student Partners
ISV
Startups
Events
Community
Magazine
Forums
Blogs
Channel 9
Documentation
APIs and reference
Dev centers
Samples
Retired content
We’re sorry. The content you requested has been removed. You’ll be auto redirected in 1 second.
Marked as answer by
Anonymous
Thursday, October 7, 2021 12:00 AM
Marked as answer by
Anonymous
Thursday, October 7, 2021 12:00 AM
Dev centers
Windows
Office
Visual Studio
Microsoft Azure
More...
Learning resources
Microsoft Virtual Academy
Channe
4k Lingerie Porn
Whitesnake Cheap An Nasty
Naked 13 Old