Private Class
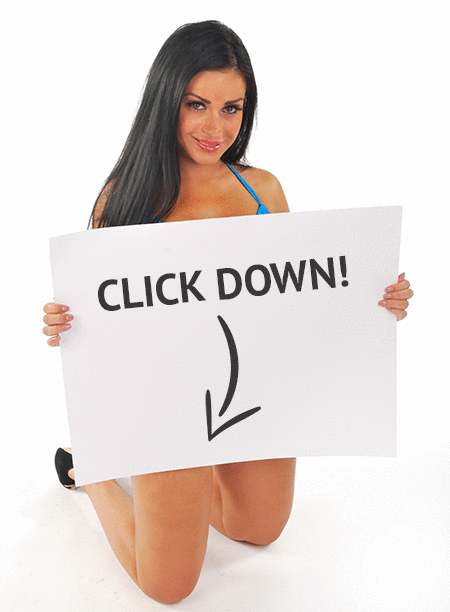
⚡ ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Private Class
class PublicCounter {
constructor ( start = 0 ) {
let _count = start ;
let _init = start ;
this . increase = ( x = 1 ) => _count += x ;
this . decrease = ( x = 1 ) => _count -= x ;
this . reset = ( x = _init ) => _count = x ;
this . getCount = ( ) => _count ;
}
get current ( ) {
return this . getCount ( ) ;
}
}
class PrivateCounter {
#count ;
#init ;
constructor ( start = 0 ) {
this . #init = start ;
this . reset ( start ) ;
}
increase ( x = 1 ) { this . #count += x ; }
decrease ( x = 1 ) { this . #count -= x ; }
reset ( x = this . #init ) { this . #count = x ; }
get current ( ) {
return this . #count ;
}
}
const total = new PrivateCounter ( 7 ) ;
console . log ( total . current ) ; // expected output: 7
total . increase ( ) ; // #count now = 8
total . increase ( 5 ) ; // #count now = 13
console . log ( total . current ) ; // expected output: 13
total . reset ( ) ; // #count now = 7
const score = new PrivateCounter ( ) ; // #count and #init are now both 0
console . log ( score . #count ) ;
// output:
// "Uncaught SyntaxError: Private field '#count' must be declared in an enclosing class"
class BadIdea {
constructor ( arg ) {
this . #init = arg ; // syntax error occurs here
#startState = arg ; // syntax error would also occur here
} // because private fields weren't defined
} // before being referenced
class BadIdeas {
#firstName ;
#firstName ; // syntax error occurs here
#lastName ;
constructor ( ) {
delete this . #lastName ; // also a syntax error
}
}
const planet = {
name : 'Terra' ,
radiusKm : 6371 ,
radiusMiles : 3959 ,
} ;
const planet = {
name : 'Terra' ,
radiusKm : 6371 ,
radiusMiles : 3959 ,
#secret : 'central inner core' ,
} ;
// result:
// "Uncaught SyntaxError: Unexpected identifier"
class colorMixer {
static #red = "rgba(1,0,0,1)" ;
static #green = "rgba(0,1,0,1)" ;
static #blue = "rgba(0,0,1,1)" ;
#mixedColor ;
constructor ( ) {
// …
}
}
class CustomClick extends HTMLElement {
#handleClicked ( ) {
// do complicated stuff in here
}
constructor ( ) {
super ( ) ;
this . #handleClicked ( ) ;
}
connectedCallback ( ) {
this . addEventListener ( 'click' , this . #handleClicked )
}
}
customElements . define ( 'chci-interactive' , CustomClick ) ;
class Counter extends HTMLElement {
#xValue = 0 ;
get #x ( ) { return this . #xValue ; }
set #x ( value ) {
this . #xValue = value ;
window . requestAnimationFrame ( this . #render . bind ( this ) ) ;
}
#clicked ( ) {
this . #x ++ ;
}
constructor ( ) {
super ( ) ;
this . onclick = this . #clicked . bind ( this ) ;
}
connectedCallback ( ) { this . #render ( ) ; }
#render ( ) {
this . textContent = this . #x . toString ( ) ;
}
}
customElements . define ( 'num-counter' , Counter ) ;
class Scalar {
#total = 0 ;
constructor ( value ) {
this . #total = value || this . #total ;
}
add ( s ) {
// check the passed object defines #total
if ( ! ( #total in s ) ) {
throw new TypeError ( "Expected an instance of Scalar" ) ;
}
this . #total += s . #total ;
}
}
const scalar1 = new Scalar ( 1 ) ;
scalar1 . add ( scalar1 )
scalar1 . add ( { } ) // throws informative exception
Web technology reference for developers
Code used to describe document style
Protocol for transmitting web resources
Interfaces for building web applications
Developing extensions for web browsers
Web technology reference for developers
Learn to structure web content with HTML
Learn to run scripts in the browser
Learn to make the web accessible to all
Frequently asked questions about MDN Plus
It's common to want to make fields or methods private, but JavaScript has lacked such a feature since its inception. Conventions have arisen — such as prefixing fields and methods that should be treated as private with an underscore, like _hidden — but these are merely conventions. The underscored features are still fully public.
Private class features deliver truly private fields and methods, with that privacy enforced by the language instead of convention. This confers benefits such as avoiding naming collisions between class features and the rest of the code base, and allowing classes to expose a very small interface to the rest of the code.
To understand how private fields work, let's first consider a class that has only public fields, but uses the constructor to encapsulate data—a somewhat common technique, even if it is a bit of a hack. The following class creates a basic count that accepts a starting number, allows that number to be increased or decreased, and can be reset to the original starting value or any other value.
The idea here is that once a new counter of this type is spawned, its starting value and its current value are not available to code outside the counter. The only way to modify the value of _count is through the defined methods, such as increase() and reset() . Similarly, _init can't be modified, because there are no methods inside the class to do so, and outside code is unable to reach it.
Here's the same idea, only this time, we'll use private fields.
The "hash mark" ( # ) is what marks a field as being private. It also prevents private fields and property names from ever being in conflict: private names must start with # , whereas property names can never start that way.
Having declared the private fields, they act as we saw in the public example. The only way to change the #count value is via the publicly available methods like decrease() , and because (in this example) there are no defined ways to alter it, the #init value is immutable. It's set when a new PrivateCounter is constructed, and can never be changed thereafter.
You cannot read a private value directly from code outside the class object. Consider:
If you wish to read private data from outside a class, you must first invent a method or other function to return it. We had already done that with the current() getter that returns the current value of #count , but #init is locked away. Unless we add something like a getInit() method to the class, we can't even see the initial value from outside the class, let alone alter it, and the compiler will throw errors if we try.
What are the other restrictions around private fields? For one, you can't refer to a private field you didn't previously define. You might be used to inventing new fields on the fly in JavaScript, but that just won't fly with private fields.
You can't define the same name twice in a single class, and you can't delete private fields.
There is another limitation: you can't declare private fields or methods via object literals . You might be used to something like this:
If you try to include a private class feature when doing this, an error will be thrown.
On the other hand, you can have static private fields, for things you want to be both private and set in stone at construction.
Just like private fields, private methods are marked with a leading # and cannot be accessed from outside their class. They're useful when you have something complex that the class needs to do internally, but it's something that no other part of the code should be allowed to call.
For example, imagine creating HTML custom elements that should do something somewhat complicated when clicked/tapped/otherwise activated. Furthermore, the somewhat complicated things that happen when the element is clicked should be restricted to this class, because no other part of the JavaScript will (or should) ever access it. Therefore, something like:
This can also be done for getters and setters, which is useful in any situation where you want to only get or set things from within the same class. As with fields and methods, prefix the name of the getter/setter with # .
In this case, pretty much every field and method is private to the class. Thus, it presents an interface to the rest of the code that's essentially just like a built-in HTML element. No other part of the JavaScript has the power to affect any of its internals.
JavaScript code will throw if you attempt to access a private method or field that does not exist (this differs from a normal/public method, which will return undefined ). If you need to write code to test whether a private feature has been defined you might use try / catch , but it is more compact to use the in operator. This returns true or false depending on whether or not the property is defined.
The code below demonstrates the approach using the example of a class for adding Scalar values. The class uses the in operator to check that added objects have the #total private class field, and throws an informative exception message if a different type of object is passed.
BCD tables only load in the browser
Last modified: Aug 23, 2022 , by MDN contributors
Your blueprint for a better internet.
Visit Mozilla Corporation’s not-for-profit parent, the Mozilla Foundation . Portions of this content are ©1998– 2022 by individual mozilla.org contributors. Content available under a Creative Commons license .
Sign up or log in to customize your list.
more stack exchange communities
company blog
Stack Overflow for Teams
– Start collaborating and sharing organizational knowledge.
Create a free Team
Why Teams?
Asked
9 years, 7 months ago
Modified
3 years, 11 months ago
This question already has answers here :
383 1 1 gold badge 3 3 silver badges 6 6 bronze badges
Highest score (default)
Trending (recent votes count more)
Date modified (newest first)
Date created (oldest first)
787 8 8 silver badges 30 30 bronze badges
6,230 5 5 gold badges 37 37 silver badges 53 53 bronze badges
39.9k 12 12 gold badges 75 75 silver badges 101 101 bronze badges
22.7k 28 28 gold badges 117 117 silver badges 181 181 bronze badges
739 1 1 gold badge 7 7 silver badges 19 19 bronze badges
63.2k 15 15 gold badges 116 116 silver badges 208 208 bronze badges
28.3k 56 56 gold badges 206 206 silver badges 376 376 bronze badges
113 1 1 gold badge 1 1 silver badge 9 9 bronze badges
Stack Overflow
Questions
Help
Products
Teams
Advertising
Collectives
Talent
Company
About
Press
Work Here
Legal
Privacy Policy
Terms of Service
Contact Us
Cookie Settings
Cookie Policy
Stack Exchange Network
Technology
Culture & recreation
Life & arts
Science
Professional
Business
API
Data
Accept all cookies
Customize settings
Find centralized, trusted content and collaborate around the technologies you use most.
Connect and share knowledge within a single location that is structured and easy to search.
Why can't we declare a private outer class? If we can have inner private class then why can't we have outer private class...?
Trending sort is based off of the default sorting method — by highest score — but it boosts votes that have happened recently, helping to surface more up-to-date answers.
It falls back to sorting by highest score if no posts are trending.
Private outer class would be useless as nothing can access it.
private modifier will make your class inaccessible from outside, so there wouldn't be any advantage of this and I think that is why it is illegal and only public , abstract & final are permitted.
Note : Even you can not make it protected .
If we can have inner private class then why can't we have outer
private class...?
You can, the distinction is that the inner class is at the "class" access level, whereas the "outer" class is at the "package" access level. From the Oracle Tutorials :
If a class has no modifier (the default, also known as package-private), it is visible only within its own package (packages are named groups of related classes — you will learn about them in a later lesson.)
Thus, package-private (declaring no modifier) is the effect you would expect from declaring an "outer" class private, the syntax is just different.
You can't have private class but you can have second class:
Also remember that static inner class is indistinguishable of separate class except it's name is OuterClass.InnerClass . So if you don't want to use "closures", use static inner class.
private makes the class accessible only to the class in which it is declared. If we make entire class private no one from outside can access the class and makes it useless.
Inner class can be made private because the outer class can access inner class where as it is not the case with if you make outer class private.
Site design / logo © 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA . rev 2022.9.6.42960
By clicking “Accept all cookies”, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy .
Downloads
Visual Studio
SDKs
Trial software
Free downloads
Office resources
Programs
Subscriptions
Overview
Administrators
Students
Microsoft Imagine
Microsoft Student Partners
ISV
Startups
Kissing Missionary
Www Ass Fucking Com
Extreme Double Penetration