Object Spread
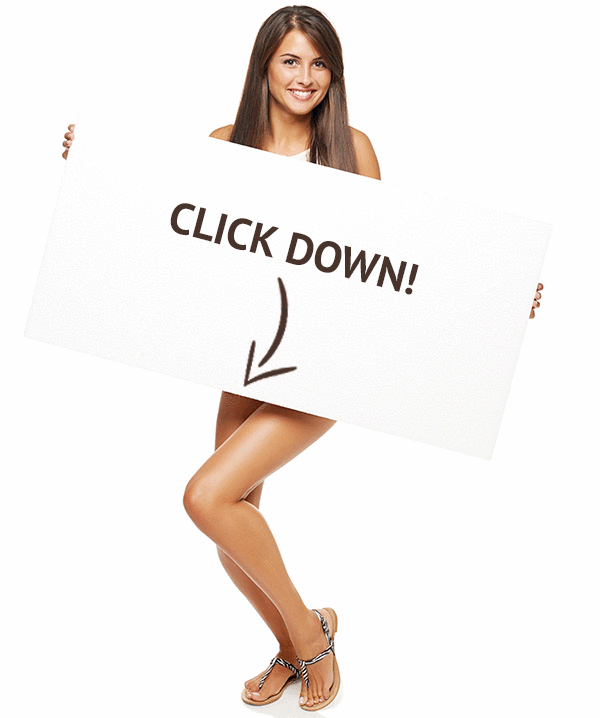
🔞 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Object Spread
// MORE CODE
// array of cities
let cities = ['Philly', 'LA', 'Clemson', 'Arlington'];
cities = [...cities, 'Dublin', 'Killasser'];
console.log(cities);
// MORE CODE
// MORE CODE
{
// all js except for node_modules
test: /\.js$/,
exclude: /node_modules/,
use: {
// use babel to transpile modern js into old js
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env'],
},
},
},
// MORE CODE
// MORE CODE
{
// all js except for node_modules
test: /\.js$/,
exclude: /node_modules/,
use: {
// use babel to transpile modern js into old js
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env'],
plugins: ['transform-object-rest-spread']
},
},
},
// MORE CODE
const house = {
bedrooms: 4,
bathrooms: 2.5,
garage: true,
yearBuilt: 1970,
}
const newHouse = {
basement: true,
...house,
washer: true,
dryer: true,
}
newHouse.dryer = false
newHouse.yearBuilt = 2000
console.log(house)
console.log(newHouse)
$ npm i babel-plugin-transform-object-rest-spread
whatever comes last will take precedent
so if you change bedrooms before it will get overwritten by spread operator
You can also override a property directly
Was this page helpful?
Yes
No
Performance & security by Cloudflare
You cannot access medium.com. Refresh the page or contact the site owner to request access.
Copy and paste the Ray ID when you contact the site owner.
Ray ID:
747188309c5116ab
747188309c5116ab Copy
For help visit Troubleshooting guide
Sign up or log in to customize your list.
more stack exchange communities
company blog
Stack Overflow for Teams
– Start collaborating and sharing organizational knowledge.
Create a free Team
Why Teams?
Asked
6 years, 11 months ago
16.9k 7 7 gold badges 52 52 silver badges 71 71 bronze badges
7,264 4 4 gold badges 19 19 silver badges 21 21 bronze badges
Highest score (default)
Trending (recent votes count more)
Date modified (newest first)
Date created (oldest first)
24.9k 3 3 gold badges 48 48 silver badges 55 55 bronze badges
3,940 7 7 gold badges 25 25 silver badges 42 42 bronze badges
4,023 2 2 gold badges 22 22 silver badges 33 33 bronze badges
const error = new Error();
error instanceof Error // true
const errorExtendedUsingSpread = {
...error,
...{
someValue: true
}
};
errorExtendedUsingSpread instanceof Error; // false
// What the spread operator desugars into
const errorExtendedUsingImmutableObjectAssign = Object.assign({}, error, {
someValue: true
});
errorExtendedUsingImmutableObjectAssign instanceof Error; // false
// The error object is modified and returned here so it keeps its prototypes
const errorExtendedUsingAssign = Object.assign(error, {
someValue: true
});
errorExtendedUsingAssign instanceof Error; // true
5,008 2 2 gold badges 25 25 silver badges 32 32 bronze badges
2,111 1 1 gold badge 12 12 silver badges 23 23 bronze badges
4,070 3 3 gold badges 38 38 silver badges 55 55 bronze badges
406 4 4 silver badges 17 17 bronze badges
13.8k 16 16 gold badges 88 88 silver badges 134 134 bronze badges
4,316 2 2 gold badges 37 37 silver badges 60 60 bronze badges
1,187 1 1 gold badge 23 23 silver badges 38 38 bronze badges
21.9k 9 9 gold badges 139 139 silver badges 130 130 bronze badges
29 1 1 silver badge 4 4 bronze badges
186 2 2 silver badges 7 7 bronze badges
10.2k 1 1 gold badge 39 39 silver badges 53 53 bronze badges
1,702 2 2 gold badges 14 14 silver badges 24 24 bronze badges
446 1 1 gold badge 5 5 silver badges 12 12 bronze badges
47k 27 27 gold badges 121 121 silver badges 136 136 bronze badges
Stack Overflow
Questions
Help
Products
Teams
Advertising
Collectives
Talent
Company
About
Press
Work Here
Legal
Privacy Policy
Terms of Service
Contact Us
Cookie Settings
Cookie Policy
Stack Exchange Network
Technology
Culture & recreation
Life & arts
Science
Professional
Business
API
Data
Accept all cookies
Customize settings
Find centralized, trusted content and collaborate around the technologies you use most.
Connect and share knowledge within a single location that is structured and easy to search.
Let’s say I have an options variable and I want to set some default value.
What’s is the benefit / drawback of these two alternatives?
This is the commit that made me wonder.
Trending sort is based off of the default sorting method — by highest score — but it boosts votes that have happened recently, helping to surface more up-to-date answers.
It falls back to sorting by highest score if no posts are trending.
If authoring code for execution in environments without native support, you may be able to just compile this syntax (as opposed to using a polyfill). (With Babel, for example.)
When this answer was originally written, this was a proposal , not standardized. When using proposals consider what you'd do if you write code with it now and it doesn't get standardized or changes as it moves toward standardization. This has since been standardized in ES2018.
This is the commit that made me wonder.
That's not directly related to what you're asking. That code wasn't using Object.assign() , it was using user code ( object-assign ) that does the same thing. They appear to be compiling that code with Babel (and bundling it with Webpack), which is what I was talking about: the syntax you can just compile. They apparently preferred that to having to include object-assign as a dependency that would go into their build.
For reference object rest/spread is finalised in ECMAScript 2018 as a stage 4. The proposal can be found here .
For the most part object assign and spread work the same way, the key difference is that spread defines properties, whilst Object.assign() sets them . This means Object.assign() triggers setters.
It's worth remembering that other than this, object rest/spread 1:1 maps to Object.assign() and acts differently to array (iterable) spread. For example, when spreading an array null values are spread. However using object spread null values are silently spread to nothing.
This is consistent with how Object.assign() would work, both silently exclude the null value with no error.
I think one big difference between the spread operator and Object.assign that doesn't seem to be mentioned in the current answers is that the spread operator will not copy the the source object’s prototype to the target object. If you want to add properties to an object and you don't want to change what instance it is of, then you will have to use Object.assign .
Edit: I've actually realised that my example is misleading. The spread operator desugars to Object.assign with the first parameter set to an empty object. In my code example below, I put error as the first parameter of the Object.assign call so the two are not equivalent. The first parameter of Object.assign is actually modified and then returned which is why it retains its prototype. I have added another example below:
NOTE: Spread is NOT just syntactic sugar around Object.assign. They operate much differently behind the scenes.
Object.assign applies setters to a new object, Spread does not. In addition, the object must be iterable.
Copy
Use this if you need the value of the object as it is at this moment, and you don't want that value to reflect any changes made by other owners of the object.
Use it for creating a shallow copy of the object
good practice to always set immutable properties to copy - because mutable versions can be passed into immutable properties, copy will ensure that you'll always be dealing with an immutable object
Assign
Assign is somewhat the opposite to copy.
Assign will generate a setter which assigns the value to the instance variable directly, rather than copying or retaining it.
When calling the getter of an assign property, it returns a reference to the actual data.
I'd like to summarize status of the "spread object merge" ES feature, in browsers, and in the ecosystem via tools.
Again: At time of writing this sample works without transpilation in Chrome (60+), Firefox Developer Edition (preview of Firefox 60), and Node (8.7+).
I'm writing this 2.5 years after the original question. But I had the very same question, and this is where Google sent me. I am a slave to SO's mission to improve the long tail.
Since this is an expansion of "array spread" syntax I found it very hard to google, and difficult to find in compatibility tables. The closest I could find is Kangax "property spread" , but that test doesn't have two spreads in the same expression (not a merge). Also, the name in the proposals/drafts/browser status pages all use "property spread", but it looks to me like that was a "first principal" the community arrived at after the proposals to use spread syntax for "object merge". (Which might explain why it is so hard to google.) So I document my finding here so others can view, update, and compile links about this specific feature. I hope it catches on. Please help spread the news of it landing in the spec and in browsers.
Lastly, I would have added this info as a comment, but I couldn't edit them without breaking the authors' original intent. Specifically, I can't edit @ChillyPenguin's comment without it losing his intent to correct @RichardSchulte. But years later Richard turned out to be right (in my opinion). So I write this answer instead, hoping it will gain traction on the old answers eventually (might take years, but that's what the long tail effect is all about, after all).
As others have mentioned, at this moment of writing, Object.assign() requires a polyfill and object spread ... requires some transpiling (and perhaps a polyfill too) in order to work.
These both produce the same output.
Here is the output from Babel, to ES5:
This is my understanding so far. Object.assign() is actually standardised, where as object spread ... is not yet. The only problem is browser support for the former and in future, the latter too.
There's a huge difference between the two, with very serious consequences. The most upvoted questions do not even touch this, and the information about object spread being a proposal is not relevant in 2022 anymore.
First, let's see the effect, and then I'll give a real-world example of how important it is to understand this fundamental difference.
Now the same exercise with the spread operator:
It's easy to see what is going on, because on the parentObject.childObject = {...} we are cleary assigning the value of the childObject key in parentObject to a brand new object literal , and the fact it's being composed by the old childObject content's is irrelevant. It's a new object.
And if you assume this is irrelevant in practice, let me show a real world scenario of how important it is to understand this.
In a very large Vue.js application, we started noticing a lot of sluggishness when typing the name of the customer in an input field.
After a lot of debugging, we found out that each char typed in that input triggered a hole bunch of computed properties to re-evaluate.
This wasn't anticipated, since the customer's name wasn't used at all in those computeds functions. Only other customer data (like age, sex) was being used. What was goin on? Why was vue re-evaluating all those computed functions when the customer's name changed?
Well, we had a Vuex store that did this:
So, voilá! When the customer name changed, the Vuex mutation was actually changing it to a new object entirely; and since the computed relied on that object to get the customer age, Vue counted on that very specific object instance as a dependency, and when it was changed to a new object (failing the === object equality test), Vue decided it was time to re-run the computed function.
The fix? Use Object.assign to not discard the previous object, but to change it in place ...
BTW, if you are in Vue2, you shouldn't use Object.assign because Vue 2 can't track those object changes directly, but the same logic applies, just using Vue.set instead of Object.assign:
The object spread operator (...) doesn't work in browsers, because it isn't part of any ES specification yet, just a proposal. The only option is to compile it with Babel (or something similar).
As you can see, it's just syntactic sugar over Object.assign({}).
As far as I can see, these are the important differences.
Other answers are old, could not get a good answer.
Below example is for object literals, helps how both can complement each other, and how it cannot complement each other (therefore difference):
Object.assign is necessary when the target object is a constant and you want to set multiple properties at once.
Now, suppose you need to mutate the target with all properties from a source:
Keep in mind that you are mutating the target obj, so whenever possible use Object.assign with empty target or spread to assign to a new obj.
It's very convenient to use and makes a lot of sense alongside object destructuring.
The one remaining advantage listed above is the dynamic capabilities of Object.assign(), however this is as easy as spreading the array inside of a literal object. In the compiled babel output it uses exactly what is demonstrated with Object.assign()
So the correct answer would be to use object spread since it is now standardized, widely used (see react, redux, etc), is easy to use, and has all the features of Object.assign()
The ways to (1) create shallow copies of objects and (2) merge multiple objects into a single object have evolved a lot between 2014 and 2018.
The approaches outlined below became available and widely used at different times. This answer provides some historical perspective and is not exhaustive.
Without any help from libraries or modern syntax, you would use for-in loops, e.g.
Object.assign is supported by Chrome (45), Firefox (34) and Node.js (4) . Polyfill is required for older runtimes though.
The Object Rest/Spread Properties proposal reaches stage 2.
The Object Rest/Spread Properties syntax did not get included in ES2017, but is usable in Chrome (60), Firefox (55), and Node.js (8.3) . Some transpilation is needed for older runtimes though.
I'd like to add this simple example when you have to use Object.assign.
It can be not clear when you use JavaScript. But with TypeScript it is easier if you want to create instance of some class
The spread operator spread the Array into the separate arguments of a function.
We’ll create a function called identity that just returns whatever parameter we give it.
If you call identity with arr, we know what’ll happen
Thanks for contributing an answer to Stack Overflow!
By clicking “Post Your Answer”, you agree to our terms of service , privacy policy and cookie policy
To subscribe to this RSS feed, copy and paste this URL into your RSS reader.
Site design / logo © 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA . rev 2022.9.7.42961
By clicking “Accept all cookies”, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy .
Learn to code — free 3,000-hour curriculum
In JavaScript, we use objects to store multiple values as a complex data structure. There are hardly any JavaScript applications that do not deal with objects.
Web developers commonly extract values from an object property to use further in programming logic. With ES6, JavaScript introduced object destructuring to make it easy to create variables from an object's properties.
In this article, we will learn about object destructuring by going through many practical examples. We will also learn how to use the spread syntax and the rest parameter . I hope you enjoy it.
We create objects with curly braces {…}
Mature Sisters
Wife Orgy Sperm
Chubby Mature Double Penetration Online