Learn Python From Scratch -Day 3
AceDommete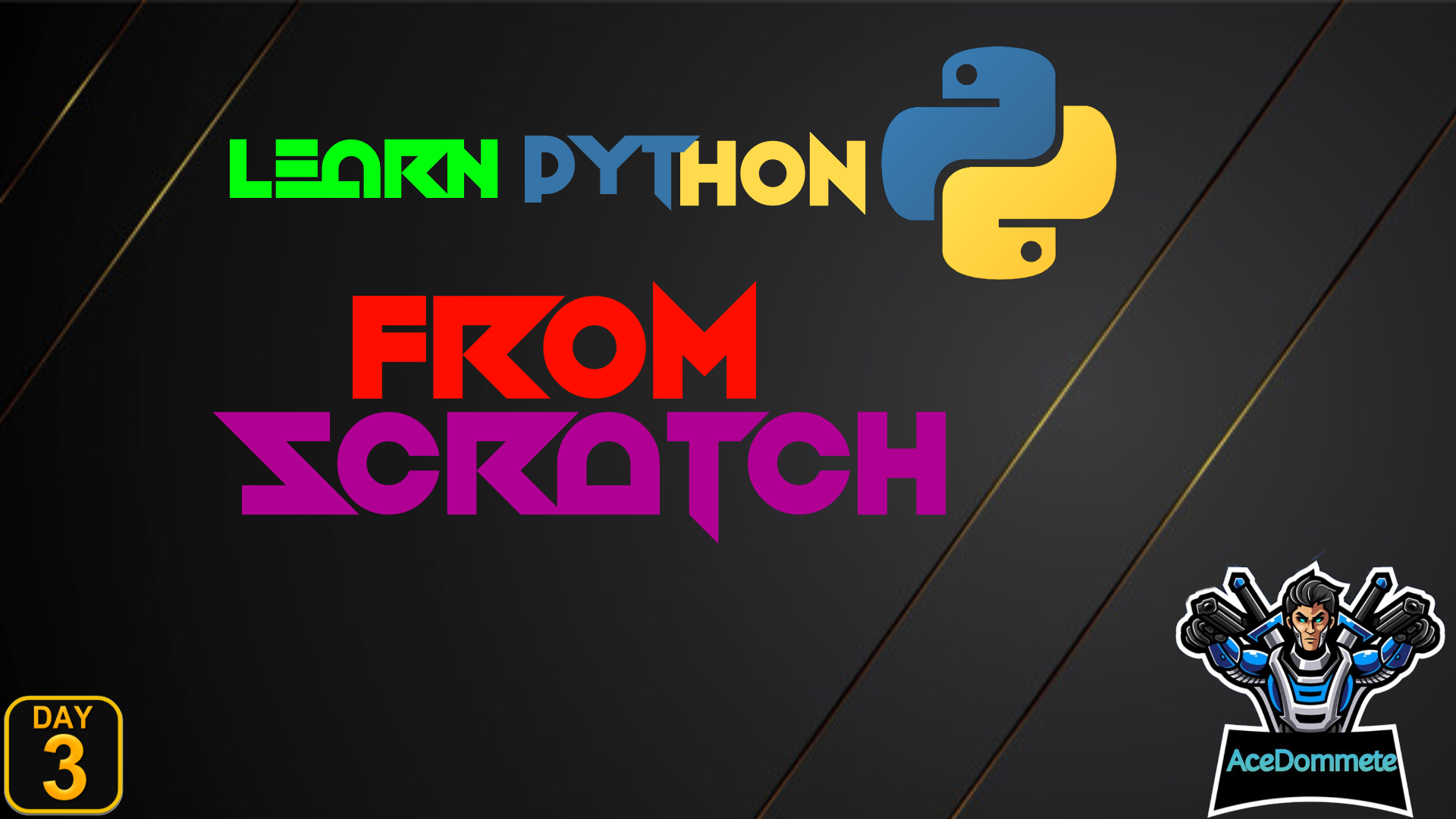
Hello Guys Welcome Back to another Chapter Of Learn Python From Scratch Series Today We Will Learn About Basic Operators And Lists In Python So Let's Jump Into It Right Now...XD
Basic Operators
This section explains how to use basic operators in Python.
Arithmetic Operators
Just like any other programming language, the addition, subtraction, multiplication, and division operators can be used with numbers.
Eg:-
number = 1 + 2 * 3 / 4.0
print(number)
Try to predict what the answer will be. Does python follow the order of operations?
Another operator available is the modulo (%) operator, which returns the integer remainder of the division. dividend % divisor = remainder.
remainder = 11 % 3
print(remainder)
Using two multiplication symbols makes a power relationship.
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
Using Operators with Strings
Python supports concatenating strings using the addition operator:
helloworld = "hello" + " " + "world"
print(helloworld)
Python also supports multiplying strings to form a string with a repeating sequence:
lotsofhellos = "hello" * 10
print(lotsofhellos)
Using Operators with Lists
Lists can be joined with the addition operators:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
Just as in strings, Python supports forming new lists with a repeating sequence using the multiplication operator:
print([1,2,3] * 3)
Lists
Lists are very similar to arrays. They can contain any type of variable, and they can contain as many variables as you wish. Lists can also be iterated over in a very simple manner. Here is an example of how to build a list.
mylist = []
mylist.append(1)
mylist.append(2)
mylist.append(3)
print(mylist[0]) # prints 1
print(mylist[1]) # prints 2
print(mylist[2]) # prints 3
# prints out 1,2,3 --acedommete
for x in mylist:
print(x)
Accessing an index which does not exist generates an exception (an error).
mylist = [1,2,3]
print(mylist[10])
Thanks for all your love and support that's it for today...
DONATE ME :- CLICK ME