Js Spread Array
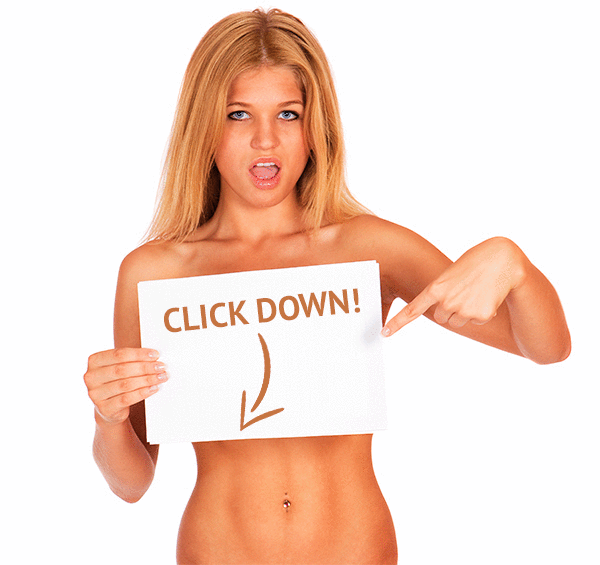
π ALL INFORMATION CLICK HERE ππ»ππ»ππ»
Js Spread Array
// Merge Array
[ ... array1 , ... array2 ]
// Clone Array
[ ... array ]
// String β Array
[ ... 'string' ]
// Set β Array
[ ... new Set ( [ 1 , 2 , 3 ] ) ]
// Node List β Array
[ ... nodeList ]
// Arguments β Array
[ ... arguments ]
[
... [ 1 , 2 , 3 ] // π The dots erases the brackets
]
/* Becoming this: */
[
1 , 2 , 3 // π "Erased"
]
const array1 = [ 1 , 2 , 3 ] ;
const array2 = [ 4 , 5 , 6 ] ;
const attemptToMerge = [ array1 , array ] ;
attemptToMerge ;
// [ [1, 2, 3], [4, 5, 6] ] π π±
const mergedArray = [
... array1 ,
... array2
] ;
mergedArray ;
// [ 1, 2, 3, 4, 5, 6 ] π β
Nice flattened array
const original = [ 'zero' , 'one' ] ;
const newArray = original ;
original ; // ['zero', 'one']
newArray ; // ['zero', 'one']
newArray [ 1 ] = 'π©' ;
newArray ;
// ['zero', 'π©']
original ;
// ['zero', 'π©'] π π± Our original array is affected
const original = [ 'zero' , 'one' ] ;
const newArray = [ ... original ] ;
original ; // ['zero', 'one']
newArray ; // ['zero', 'one']
newArray [ 1 ] = 'π©' ;
newArray ;
// ['zero', 'π©']
original ;
// ['zero', 'one'] β
original array is NOT affected
const string = 'hi' ;
const array = [ ... string ] ;
array ;
// [ 'h' , 'i' ]
const set = new Set ( [ 1 , 2 , 3 ] ) ;
set ;
// Set(3) {1, 2, 3}
const array = [ ... set ] ;
array ;
// [1, 2, 3]
const nodeList = document . querySelectorAll ( 'p' ) ;
nodeList ;
// [p, p, p]
const array = [ ... nodeList ] ;
array ;
function names ( ) {
arguments ;
// Arguments(4)['samantha', 'sam']
arguments . map ( name => ` hi ${ name } ` ) ;
// β TypeError: arguments.map is not a function
}
names ( 'samantha' , 'sam' ) ;
function names ( ) {
const argumentsArray = [ ... arguments ] ;
argumentsArray . map ( name => ` hi ${ name } ` ) ;
// β
['hi samantha', 'hi sam']
}
names ( 'samantha' , 'sam' ) ;
array = [ 1 , 2 , 3 , 4 , 5 ] ;
var minimum = Math . min ( ... array ) ;
var maximum = Math . max ( ... array ) ;
How to Compare 2 Objects in JavaScript
Checking if a string contains substring in JavaScript
2 Ways to Convert to Boolean in JavaScript
My Top 3 Favorite FREE JavaScript Courses
Testing Non-Exported Functions in JavaScript with Rewire
How to Pad a String with padStart and padEnd in JavaScript
Fix Text Overlap with CSS white-space
How to Check if Object is Empty in JavaScript
π Site is currently under construction π
Here are 6 ways to use the Spread operator with Array in JavaScript. You can use it to merge or clone an array. Or use it to convert iterables to an array.
MDN: Spread syntax allows an iterable such as an array expression or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected, or an object expression to be expanded in places where zero or more key-value pairs (for object literals) are expected.
Clear as mud right π Spread took me a long time to understand. So let me try to explain with 2 analogies that helped me. Hopefully, it can also help you π€
The spread syntax takes your array and expands it into elements. Imagine your array is like those Russian Dolls. When you call the spread syntax on it, it will take the nested individual doll out and lay it out in its own individual pieces.
If the Russian Dolls analogy didn't help and Spread is still muddy for you π΅ In that case, just think of the ... syntax as an eraser that removes the brackets of the array π
The best thing about the Spread syntax is to use it for array manipulation π
Let's see what happens when we try to merge the array without the spread syntax.
π When you try to merge an array without the spread syntax, you wind up with a nested or multi-dimensional array.
So let's use the Spread syntax to "erase" the brackets.
Cloning array in JavaScript isn't as straight forward. So let's see it done in 2 paths - the wrong way and the right way π£
In JavaScript, arrays are reference types, so you can't just create a new copy of an array using = . Let's see what problem happens if we try to do it that way.
πSo everything looks okay so far, but let's see what happens if we change the element.
OH yikes π± Changing the newArray will mutate the original array π
Think of references as addresses. When we create a copy of an array using = , we've only copied the address. We have NOT copied the underlying array, which is what we want. To do that, we need to copy the array at a new address. That way, when we make changes to our new array, it won't affect the original array -- because they're at different addresses.
So if we did this correctly, our original array shouldn't be affected if we changed the newArray . Alright, let's give this a try πͺ
With Spread, converting different data types to an Array has never been easier π
When we spread a string , it will return an array of individual substrings.
Using Spread, we can convert the set into an array:
Now we can use Spread to convert our node list into an array:
Before we begin, let's take some time to understand what the arguments objects is.
MDN: arguments is an Array-like object accessible inside functions that contains the values of the arguments passed to that function.
π Notice the key there, array-like -- So it looks like an array, but it isn't (oh JS, always making things so fun for us to understand you π
). The benefit of converting the arguments object to an array means we have access to all the awesome array methods (ie. map , filter , find ) π
Alright, let's convert our arguments into an array so we can apply array methods on it π
@harmleprinze : Split will give more options, considering it takes in separator and limit
@mohammed_mamoun_98 : If you merged two arrays without spread it's gonna be two-dimensional array but flat make it one dimension so it's more effective I guess. You can try it
@bukazoltan : The min() and max() method cannot take an array as an input, so spreading it can solve that problem too.
- Learn Flexbox with 30 Code Tidbits β¨
- 30 days of the best JS, CSS, HTML tidbits π
- Web Basics Explained with Tidbits π
The spread (β¦) syntax allow us to expand an iterable like array in places where 0+ arguments are expected. It allows us to pass a number of parameters as an array to a
function.
Following is the code to implement spread operator for arrays in JavaScript β
On clicking the βCLICK HEREβ button β
Β© Copyright 2022. All Rights Reserved.
We make use of First and third party cookies to improve our user experience. By using this website, you agree with our Cookies Policy.
Agree
Learn more
const arr = [ 1 , 2 , 3 ] ;
const arr2 = [ ... arr ] ;
// [1, 2, 3]
const arr1 = [ 1 , 2 , 3 ] ;
const arr2 = [ 4 , 5 , 6 ] ;
const combined = [ ... arr1 , ... arr2 ] ;
// [1, 2, 3, 4, 5, 6]
const arr = [ 1 , 2 , 3 ] ;
const arr2 = [ 0 , ... arr , 4 ] ;
// [0, 1, 2, 3, 4]
Angelos Chalaris Β· JavaScript, Array Β· Apr 17, 2022
The spread operator can be used to clone an array into a new array. This trick can come in handy when working with arrays of primitives. However, it only shallow clones the array, meaning nested non-primitive values will not be cloned.
Using the spread operator, itβs possible to combine two or more arrays into one. You should think of this trick as cloning two arrays into a new one. Due to that, the shallow cloning limitation mentioned previously applies here, too.
Similarly to previous tricks, itβs possible to spread an array into a new one and add individual elements, too. This can also be combined with merging multiple arrays, if desired.
Creates an object from an array, using a function to map each value to a key.
Maps the values of an array to an object using a function.
Creates an object from an array, using the specified key and excluding it from each value.
Website, name & logo Β© 2017-2022 30 seconds of code Individual snippets licensed under CC-BY-4.0 Powered by Netlify , Next.js & GitHub
Come write articles for us and get featured
Learn and code with the best industry experts
Get access to ad-free content, doubt assistance and more!
Come and find your dream job with us
Difficulty Level :
Easy Last Updated :
21 Jul, 2021
console.log(arr); // [ 1, 2, 3, 4, 5 ]
// spread operator doing the concat job
console.log(arr); // [ 1, 2, 3, 4, 5 ]
// copying without the spread operator
console.log(arr2); // [ 'a', 'b', 'c' ]
console.log(arr); // even affected the original array(arr)Β
console.log(arr); // [ 'a', 'b', 'c' ]
arr2.push( 'd' ); //inserting an element at the end of arr2
console.log(arr2); // [ 'a', 'b', 'c', 'd' ]
console.log(arr); // [ 'a', 'b', 'c' ]
console.log(arr2); // [ [ 'a', 'b' ], 'c', 'd' ]
console.log(arr2); // [ 'a', 'b', 'c', 'd' ]
console.log(Math.min(1,2,3,-1)); //-1Β
// min in an array using Math.min()
console.log(Math.min(...arr)); //-1
const mergedUsers = {...user1, ...user2};
What is the rest parameter and spread operator in JavaScript ?
What is the meaning of spread operator (...) in Reactjs?
Explain spread operator in ES6 with an Example
What is spread, default and rest parameters in JavaScript ?
Explain the benefits of spread syntax & how it is different from rest syntax in ES6 ?
JavaScript Course | Conditional Operator in JavaScript
Difference between == and === operator in JavaScript
What is the !! (not not) operator in JavaScript?
How to get negative result using modulo operator in JavaScript ?
Difference between != and !== operator in JavaScript
What is JavaScript >>> Operator and How to use it ?
JavaScript Nullish Coalescing Operator
What does +_ operator mean in JavaScript?
JavaScript Unsigned Right Shift Assignment Operator
Advanced JavaScript- Self Paced Course
Data Structures & Algorithms- Self Paced Course
Complete Interview Preparation- Self Paced Course
Improve your Coding Skills with Practice Try It!
A-143, 9th Floor, Sovereign Corporate Tower, Sector-136, Noida, Uttar Pradesh - 201305
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Got It !
Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 values are expected. It allows us the privilege to obtain a list of parameters from an array. Syntax of Spread operator is same as Rest parameter but it works completely opposite of it.
In the above syntax, β¦ is spread operator which will target all values in particular variable. When β¦ occurs in function call or alike,its called a spread operator. Spread operator can be used in many cases,like when we want to expand,copy,concat,with math object . Letβs look at each of them one by one:
Note : In order to run the code in this article make use of the console provided by the browser.
The concat() method provided by javascript helps in concatenation of two or more strings(String concat() ) or is used to merge two or more arrays. In case of arrays,this method does not change the existing arrays but instead returns a new array.
Output: We can achieve the same output with the help of the spread operator, the code will look something like this:
Output: Note : Though we can achieve the same result, but it is not recommended to use the spread in this particular case, as for a large data set it will work slower as when compared to the native concat() method.
In order to copy the content of array to another we can do something like this:
The above code works fine because we can copy the contents of one array to another, but under the hood, itβs very different as when we mutate new array it will also affect the old array(the one which we copied). See the code below:
In the above code we can clearly see that when we tried to insert an element inside the array, the original array is also altered which we didnβt intended and is not recommended. We can make use of the spread operator in this case, like this:
By using the spread operator we made sure that the original array is not affected whenever we alter the new array.
Whenever we want to expand an array into another we do something like this:
Output: Even though we get the content on one array inside the other one, but actually it is array inside another array which is definitely what we didnβt want. If we want the content to be inside a single array we can make use of the spread operator.
The Math object in javascript has different properties that we can make use of to do what we want like finding the minimum from a list of numbers, finding maximum etc. Consider the case that we want to find the minimum from a list of numbers,we will write something like this:
Output: Now consider that we have an array instead of a list, this above Math object method wonβt work and will return NaN, like:
Output: When β¦arr is used in the function call, it βexpandsβ an iterable object arr into the list of arguments In order to avoid this NaN output, we make use of spread operator, like:
Example of spread operator with objects
ES6 has added spread property to object literals in javascript. The spread operator ( β¦ ) with objects is used to create copies of existing objects with new or updated values or to make a copy of an object with more properties. Letβs take at an example of how to use the spread operator on an object,
Here we are spreading the user1 object. All key-value pairs of the user1 object are copied into the clonedUser object. Letβs look on another example of merging two objects using the spread operator,
mergedUsers is a copy of user1 and user2 . Actually, every enumerable property on the objects will be copied to mergedUsers object. The spread operator is just a shorthand for the Object.assign() method but, they are some differences between the two.
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples .
Writing code in comment?
Please use ide.geeksforgeeks.org ,
generate link and share the link here.
Tokyo Face Fuck Sperm Gangbang
Mature Lesbi Video
Step Mom Masturbate