Js Object Spread
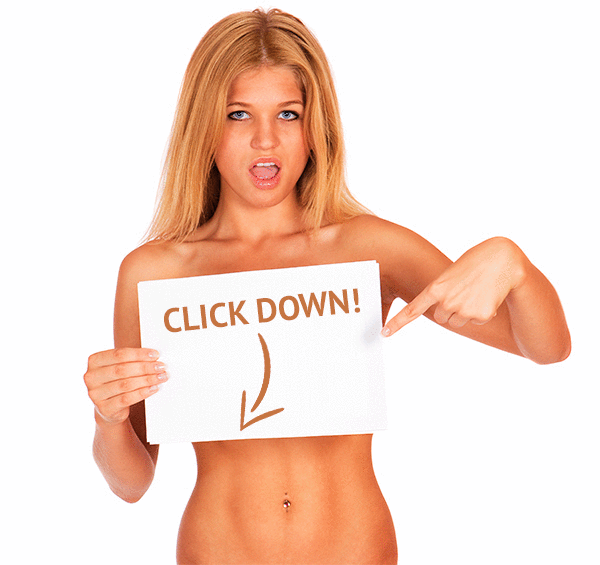
🔞 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Js Object Spread
Learn to code — free 3,000-hour curriculum
In JavaScript, we use objects to store multiple values as a complex data structure. There are hardly any JavaScript applications that do not deal with objects.
Web developers commonly extract values from an object property to use further in programming logic. With ES6, JavaScript introduced object destructuring to make it easy to create variables from an object's properties.
In this article, we will learn about object destructuring by going through many practical examples. We will also learn how to use the spread syntax and the rest parameter . I hope you enjoy it.
We create objects with curly braces {…} and a list of properties. A property is a key-value pair where the key must be a string or a symbol, and the value can be of any type, including another object.
Here we have created a user object with three properties: name, address, and age. The real need in programming is to extract these property values and assign them to a variable.
For example, if we want to get the value of the name and age properties from the user object, we can do this:
This is undoubtedly a bit more typing. We have to explicitly mention the name and age property with the user object in dot(.) notation, then declare variables accordingly and assign them.
We can simplify this process using the new object destructuring syntax introduced in ES6.
By default, the object key name becomes the variable that holds the respective value. So no extra code is required to create another variable for value assignment. Let's see how this works with examples.
Let's take the same user object that we referred to above.
The expression to extract the name property value using object destructuring is the following:
As you see, on the left side of the expression, we pick the object property key ( name in this case) and place it inside the {} . It also becomes the variable name to hold the property value.
The right side of the expression is the actual object that extracts the value. We also mention the keywords, const , let and so on to specify the variable's scope.
So, how do we extract values from more than one object property? Simple – we keep adding the object keys inside the {} with commas separating them. In the example below, we destructure both the name and age properties from the user object.
The keywords let and const are significant in object destructuring syntax. Consider the example below where we have omitted the let or const keyword. It will end up in the error, Uncaught SyntaxError: Unexpected token '=' .
What if we declare the variable in advance and then try to destructure the same name's key from the object? Nope, not much luck here either. It is still syntactically incorrect.
In this case, the correct syntax is to put the destructuring expression inside parenthesis ( (...) ).
We can add a new variable while destructuring and add a default value to it. In the example below, the salary variable is non-existent in the user object. But we can add it in the destructuring expression and add a default value to it.
The alternative way to do the above is this:
There is a considerable advantage to the flexibility of adding a variable with a default value. The default value of this new variable is not necessarily going to be any constant value always. We can compute the value of it from other destructured property values.
Let's take a user object with two properties, first_name and last_name . We can now compute the value of a non-existent full_name using these two properties.
You can give an alias name to your destructured variables. It comes in very handy if you want to reduce the chances of variable name conflicts.
In the example below, we have specified an alias name for the property address as permanentAddress .
Please note, an attempt to access the variable address here will result in this error:
An object can be nested. This means that the value of an object property can be another object, and so on.
Let's consider the user object below. It has a property called department with the value as another object. But let's not stop here! The department has a property with the key address whose value is another object. Quite a real-life scenario, isn't it?
How do we extract the value of the department property? Ok, it should be straight-forward by now.
And here's the output when you log department :
But, let's go one more nested level down. How do we extract the value of the address property of the department ? Now, this may sound a bit tricky. However, if you apply the same object destructuring principles, you'll see that it's similar.
Here's the output when you log address :
In this case, department is the key we focus on and we destructure the address value from it. Notice the {} around the keys you want to destructure.
Now it's time to take it to the next level. How do we extract the value of city from the department's address? Same principle again!
The output when you log city is "Bangalore".
Many times you may not know the property name (key) of an object while destructuring it. Consider this example. We have a user object:
Now the method getValue(key) takes a property key name and should return the value of it.
So, how do we write the definition of the getValue(key) method using the destructuring syntax?
Well, the syntax is very much the same as creating aliases. As we don't know the key name to hard-code in the destructuring syntax, we have to enclose it with square brackets ( [...] ).
This one is my favorites, and it practically reduces lots of unnecessary code. You may want just a couple of specific property values to pass as a parameter to the function definition, not the entire object. Use object destructuring to function parameter in this case.
Let's take the user object example once again.
Suppose we need a function to return a string using the user's name and age. Say something like Alex is 43 year(s) old! is the return value when we call this:
We can simply use destructuring here to pass the name and age values, respectively, to the function definition. There is no need to pass the entire user object and then extract the values from it one by one. Please have a look:
When a function returns an object and you are interested in specific property values, use destructuring straight away. Here is an example:
It is similar to the basic object destructuring we saw in the beginning.
You can use object destructuring with the for-of loop. Let's take an array of user objects like this:
We can extract the property values with object destructuring using the for-of loop.
In JavaScript, console is a built-in object supported by all browsers. If you have noticed, the console object has many properties and methods, and some are very popular, like console.log() .
Using the destructuring object syntax, we can simplify the uses of these methods and properties in our code. How about this?
The Spread Syntax (also known as the Spread Operator) is another excellent feature of ES6. As the name indicates, it takes an iterable (like an array) and expands (spreads) it into individual elements.
We can also expand objects using the spread syntax and copy its enumerable properties to a new object.
Spread syntax helps us clone an object with the most straightforward syntax using the curly braces and three dots {...} .
With spread syntax we can clone, update, and merge objects in an immutable way. The immutability helps reduce any accidental or unintentional changes to the original (Source) object.
We can create a cloned instance of an object using the spread syntax like this:
You can alternatively use object.assign() to create a clone of an object. However, the spread syntax is much more precise and much shorter.
We can add a new property (key-value pair) to the object using the spread syntax . Note that the actual object never gets changed. The new property gets added to the cloned object.
In the example below, we are adding a new property ( salary ) using the spread syntax.
We can also update an existing property value using the spread syntax. Like the add operation, the update takes place on the object's cloned instance, not on the actual object.
In the example below, we are updating the value of the age property:
As we have seen, updating an object with the spread syntax is easy, and it doesn't mutate the original object. However, it can be a bit tricky when you try to update a nested object using the spread syntax. Let's understand it with an example.
We have a user object with a property department . The value of the department property is an object which has another nested object with its address property.
Now, how can we add a new property called, number with a value of, say, 7 for the department object? Well, we might try out the following code to achieve it (but that would be a mistake):
As you execute it, you will realize that the code will replace the entire department object with the new value as, {'number': 7} . This is not what we wanted!
How do we fix that? We need to spread the properties of the nested object as well as add/update it. Here is the correct syntax that will add a new property number with the value 7 to the department object without replacing its value:
The last practical use of the spread syntax in JavaScript objects is to combine or merge two objects. obj_1 and obj_2 can be merged together using the following syntax:
Note that this way of merging performs a shallow merge . This means that if there is a common property between both the objects, the property value of obj_2 will replace the property value of obj_1 in the merged object.
Let's take the user and department objects to combine (or merge) them together.
Merge the objects using the spread syntax, like this:
If we change the department object like this:
Now try to combine them and observe the combined object output:
The name property value of the user object is replaced by the name property value of the department object in the merged object output. So be careful of using it this way.
As of now, you need to implement the deep-merge of objects by yourself or make use of a library like lodash to accomplish it.
The Rest parameter is kind of opposite to the spread syntax. While spread syntax helps expand or spread elements and properties, the rest parameter helps collect them together.
In the case of objects, the rest parameter is mostly used with destructuring syntax to consolidate the remaining properties in a new object you're working with.
Let's look at an example of the following user object:
We know how to destructure the age property to create a variable and assign the value of it. How about creating another object at the same time with the remaining properties of the user object? Here you go:
In the output we see that the age value is 43 . The rest parameter consolidated the rest of the user object properties, name and address , in a separate object.
I hope you've found this article insightful, and that it helps you start using these concepts more effectively. Let's connect. You will find me active on Twitter (@tapasadhikary) . Please feel free to give a follow.
You can find all the source code examples used in this article in my GitHub repository - js-tips-tricks . Are you interested in doing some hands-on coding based on what we have learned so far? Please have a look at the quiz here , and you may find it interesting.
Writer . YouTuber . Creator . Mentor
If you read this far, tweet to the author to show them you care. Tweet a thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
freeCodeCamp is a donor-supported tax-exempt 501(c)(3) nonprofit organization (United States Federal Tax Identification Number: 82-0779546)
Our mission: to help people learn to code for free. We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. We also have thousands of freeCodeCamp study groups around the world.
Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff.
You can make a tax-deductible donation here .
Come write articles for us and get featured
Learn and code with the best industry experts
Get access to ad-free content, doubt assistance and more!
Come and find your dream job with us
Difficulty Level :
Easy Last Updated :
21 Jul, 2021
console.log(arr); // [ 1, 2, 3, 4, 5 ]
// spread operator doing the concat job
console.log(arr); // [ 1, 2, 3, 4, 5 ]
// copying without the spread operator
console.log(arr2); // [ 'a', 'b', 'c' ]
console.log(arr); // even affected the original array(arr)
console.log(arr); // [ 'a', 'b', 'c' ]
arr2.push( 'd' ); //inserting an element at the end of arr2
console.log(arr2); // [ 'a', 'b', 'c', 'd' ]
console.log(arr); // [ 'a', 'b', 'c' ]
console.log(arr2); // [ [ 'a', 'b' ], 'c', 'd' ]
console.log(arr2); // [ 'a', 'b', 'c', 'd' ]
console.log(Math.min(1,2,3,-1)); //-1
// min in an array using Math.min()
console.log(Math.min(...arr)); //-1
const mergedUsers = {...user1, ...user2};
What is the rest parameter and spread operator in JavaScript ?
What is the meaning of spread operator (...) in Reactjs?
Explain spread operator in ES6 with an Example
What is spread, default and rest parameters in JavaScript ?
Explain the benefits of spread syntax & how it is different from rest syntax in ES6 ?
JavaScript Course | Conditional Operator in JavaScript
Difference between == and === operator in JavaScript
What is the !! (not not) operator in JavaScript?
How to get negative result using modulo operator in JavaScript ?
Difference between != and !== operator in JavaScript
What is JavaScript >>> Operator and How to use it ?
JavaScript Nullish Coalescing Operator
What does +_ operator mean in JavaScript?
JavaScript Unsigned Right Shift Assignment Operator
Advanced JavaScript- Self Paced Course
Data Structures & Algorithms- Self Paced Course
Complete Interview Preparation- Self Paced Course
Improve your Coding Skills with Practice Try It!
A-143, 9th Floor, Sovereign Corporate Tower, Sector-136, Noida, Uttar Pradesh - 201305
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Got It !
Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 values are expected. It allows us the privilege to obtain a list of parameters from an array. Syntax of Spread operator is same as Rest parameter but it works completely opposite of it.
In the above syntax, … is spread operator which will target all values in particular variable. When … occurs in function call or alike,its called a spread operator. Spread operator can be used in many cases,like when we want to expand,copy,concat,with math object . Let’s look at each of them one by one:
Note : In order to run the code in this article make use of the console provided by the browser.
The concat() method provided by javascript helps in concatenation of two or more strings(String concat() ) or is used to merge two or more arrays. In case of arrays,this method does not change the existing arrays but instead returns a new array.
Output: We can achieve the same output with the help of the spread operator, the code will look something like this:
Output: Note : Though we can achieve the same result, but it is not recommended to use the spread in this particular case, as for a large data set it will work slower as when compared to the native concat() method.
In order to copy the content of array to another we can do something like this:
The above code works fine because we can copy the contents of one array to another, but under the hood, it’s very different as when we mutate new array it will also affect the old array(the one which we copied). See the code below:
In the above code we can clearly see that when we tried to insert an element inside the array, the original array is also altered which we didn’t intended and is not recommended. We can make use of the spread operator in this case, like this:
By using the spread operator we made sure that the original array is not affected whenever we alter the new array.
Whenever we want to expand an array into another we do something like this:
Output: Even though we get the content on one array inside the other one, but actually it is array inside another array which is definitely what we didn’t want. If we want the content to be inside a single array we can make use of the spread operator.
The Math object in javascript has different properties that we can make use of to do what we want like finding the minimum from a list of numbers, finding maximum etc. Consider the case that we want to find the minimum from a list of numbers,we will write something like this:
Output: Now consider that we have an array instead of a list, this above Math object method won’t work and will return NaN, like:
Output: When …arr is used in the function call, it “expands” an iterable object arr into the list of arguments In order to avoid this NaN output, we make use of spread operator, like:
Example of spread operator with objects
ES6 has added spread property to object literals in javascript. The spread operator ( … ) with objects is used to create copies of existing objects with new or updated values or to make a copy of an object with more properties. Let’s take at an example of how to use the spread operator on an object,
Here we are spreading the user1 object. All key-value pairs of the user1 object are copied into the clonedUser object. Let’s look on another example of merging two objects using the spread operator,
mergedUsers is a copy of user1 and user2 . Actually, every enumerable property on the objects will be copied to mergedUsers object. The spread operator is just a shorthand for the Object.assign() method but, they are some differences between the two.
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples .
Writing code in comment?
Please use ide.geeksforgeeks.org ,
generate link and share the link here.
Was this page helpful?
Yes
No
Performance & security by Cloudflare
You cannot access medium.com. Refresh the page or contact the site owner to request access.
Copy and paste the Ray ID when you contact the site owner.
Ray ID:
7471b7503bb29db0
7471b7503bb29db0 Copy
For help visit Troubleshooting guide
Was this page helpful?
Yes
No
Performance & security by Cloudflare
You cannot access blog.devgenius.io. Refresh the page or contact the site owner to request access.
Copy and paste the Ray ID when you contact the site owner.
Ray ID:
7471b7b5eae67b2b
7471b7b5eae67b2b Copy
For help
Http Www Private
Lesbian Spread
Russian Double Penetration Chloe