Javascript Spread Operator
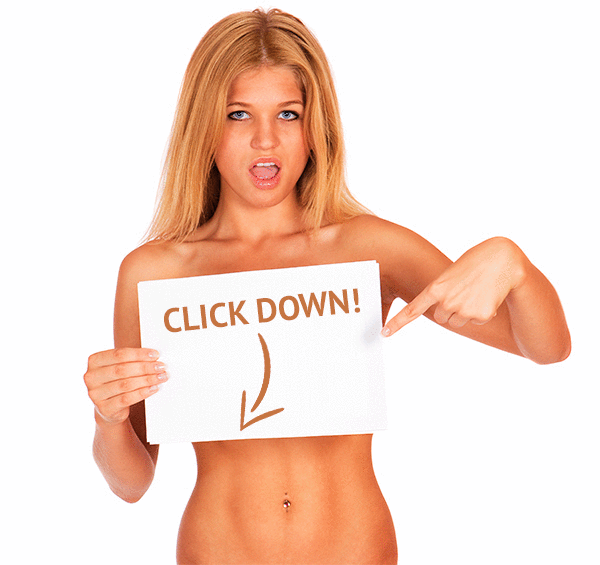
π ALL INFORMATION CLICK HERE ππ»ππ»ππ»
Javascript Spread Operator
Was this page helpful?
Yes
No
Performance & security by Cloudflare
You cannot access medium.com. Refresh the page or contact the site owner to request access.
Copy and paste the Ray ID when you contact the site owner.
Ray ID:
7471d2d5297e165a
7471d2d5297e165a Copy
For help visit Troubleshooting guide
Courses
Tutorials
Examples
Course Index
Explore Programiz
Python
JavaScript
SQL
C
C++
Java
Kotlin
Swift
C#
DSA
Join our newsletter for the latest updates.
Join our newsletter for the latest updates.
Examples
Python Examples
JavaScript Examples
C
Examples
Java Examples
Kotlin Examples
C++ Examples
Company
Change Ad Consent
Do not sell my data
About
Advertising
Privacy Policy
Terms & Conditions
Contact
Blog
Youtube
Apps
Learn Python
Learn C Programming
Learn Java
Get ahead of your peers. Try hands-on coding with Programiz PRO. Claim Discount
In this tutorial, you will learn about JavaScript spread operator with the help of examples.
The spread operator is a new addition to the features available in the JavaScript ES6 version.
The spread operator ... is used to expand or spread an iterable or an array. For example,
You can also use the spread syntax ... to copy the items into a single array. For example,
In JavaScript, objects are assigned by reference and not by values. For example,
Here, both variables arr1 and arr2 are referring to the same array. Hence the change in one variable results in the change in both variables.
However, if you want to copy arrays so that they do not refer to the same array, you can use the spread operator. This way, the change in one array is not reflected in the other. For example,
You can also use the spread operator with object literals. For example,
Here, both obj1 and obj2 properties are added to obj3 using the spread operator.
When the spread operator is used as a parameter, it is known as the rest parameter.
You can also accept multiple arguments in a function call using the rest parameter. For example,
Note : Using the rest parameter will pass the arguments as array elements.
You can also pass multiple arguments to a function using the spread operator. For example,
If you pass multiple arguments using the spread operator, the function takes the required arguments and ignores the rest.
Note : Spread operator was introduced in ES6 . Some browsers may not support the use of spread syntax. Visit JavaScript Spread Operator support to learn more.
JavaScript Destructuring Assignment
Β© Parewa Labs Pvt. Ltd. All rights reserved.
Take advantage of Back to School SALE on Programiz PRO.
Learn to code β free 3,000-hour curriculum
JavaScript has two awesome data structures that help you write clean and efficient code. But handling them can get messy sometimes.
In this blog, I am going to show you how to handle destructuring in arrays and objects in JavaScript. We'll also learn how to use the spread operator as well.
Let's say we have an array that contains five numbers, like this:
To get the elements from the array, we can do something like getting the number according to its indexes:
But this method is old and clunky, and there is a better way to do it β using array destructuring. It looks like this:
Both methods above will yield the same result:
Now, we have five elements in the array, and we print those.Β But what if we want to skip one element in between?
Here, we have skipped indexThird , and there's an empty space between indexTwo and indexFour.
You can see that we are not getting the third element because we have set it as empty.
This destructuring works well with objects too. Let me give you an example.
Let's say we want the name, salary, and weight from this object to be printed out in the console.
We can get them using the keys, which are name, salary, and weight.
But this code becomes difficult to understand sometimes. That's when destructuring comes in handy:
And now, we can just log name, salary, and weight instead of using that old method.
We can also use destructuring to set default values if the value is not present in the object.
Here, we have name and weight present in the object, but not the salary:
We will get an undefined value for the salary.
To correct that issue, we can set default values when we are destructuring the object.
You can see that we get 200 as the Salary. This only works when we don't have that key in the object, and we want to set a default value.
Add salary in the object, and you will get 300 as the salary.
Let's say we have a function that prints all the data in the array to the console.
We are passing the object as a parameter in the function when it gets called:
Normally, we would do something like this β passing the object and logging it in the console.
But again, we can do the same using destructuring.
Here, we are destructuring the object into name, age, salary, height and weight in the function parameters and we print everything on the same line.
You can see how destructuring makes it so much easier to understand.
We have a function here which accepts two numbers. It returns an array adding them and multiplying them and logs them into the console.
Let's use destructuring here instead.
We can destructure it into addition and multiplication variables like this:
And in the output, you can see we get the addition and multiplication of both numbers.
Spread means spreading or expanding. And the spread operator in JavaScript is denoted by three dots.
This spread operator has many different uses. Let's see them one by one.
Let's say we have two arrays and we want to merge them.
We are getting the combination of both arrays, which are array1 and array2.
But there is an easier way to do this:
In this case, we are using the spread operator to merge both arrays.
And you can see, we will get the same output.
Let's imagine another use case where we have to insert array1 between the elements of array2 .
For example, we want to insert array2 between the second and third element of array1 .
So, how do we do that? We can do something like this:
And you can see, we get the array1 elements between 7 and 8.
Now, let's merge two objects together using the spread operator.
We have two objects here. One contains firstName, age, and salary. The second one contains lastName, height, and weight.
We have now merged both objects using the spread operator, and we've logged the value in the console.
You can see that we are getting the combination of both objects.
Lastly, we can also copy one array into another using the spread operator. Let me show you how it works:
Here, we are copying array1 into array2 using the spread operator.
We are logging array2 in the console, and we are getting the items of array1 .
That's all, folks! In this article, we learned about array and object destructuring and the spread operator.
You can also watch my Youtube video on Array and Object Destructuring and the Spread Operator if you want to supplement your learning.
I build projects to learn how code works.
And while I am not coding, I enjoy writing poetry and stories, playing the piano, and cooking delicious meals.
If you read this far, tweet to the author to show them you care. Tweet a thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
freeCodeCamp is a donor-supported tax-exempt 501(c)(3) nonprofit organization (United States Federal Tax Identification Number: 82-0779546)
Our mission: to help people learn to code for free. We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. We also have thousands of freeCodeCamp study groups around the world.
Donations to freeCodeCamp go toward our education initiatives, and help pay for servers, services, and staff.
You can make a tax-deductible donation here .
const odd = [ 1 , 3 , 5 ];
const combined = [ 2 , 4 , 6 , ...odd];
console .log(combined);
function f ( a, b, ...args ) {
console .log(args);
}
f( 1 , 2 , 3 , 4 , 5 );
const odd = [ 1 , 3 , 5 ];
const combined = [...odd, 2 , 4 , 6 ];
console .log(combined);
const odd = [ 1 , 3 , 5 ];
const combined = [ 2 ,...odd, 4 , 6 ];
console .log(combined);
function compare ( a, b ) {
return a - b;
}
let result = compare.apply( null , [ 1 , 2 ]);
console .log(result); // -1
let result = compare(...[ 1 , 2 ]);
console .log(result); // -1
let rivers = [ 'Nile' , 'Ganges' , 'Yangte' ];
let moreRivers = [ 'Danube' , 'Amazon' ];
[].push.apply(rivers, moreRivers);
console .log(rivers);
let initialChars = [ 'A' , 'B' ];
let chars = [...initialChars, 'C' , 'D' ];
console .log(chars); // ["A", "B", "C", "D"]
let numbers = [ 1 , 2 ];
let moreNumbers = [ 3 , 4 ];
let allNumbers = [...numbers, ...moreNumbers];
console .log(allNumbers); // [1, 2, 3, 4]
let scores = [ 80 , 70 , 90 ];
let copiedScores = [...scores];
console .log(copiedScores); // [80, 70, 90]
let chars = [ 'A' , ... 'BC' , 'D' ];
console .log(chars); // ["A", "B", "C", "D"]
The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively.
Home Β» JavaScript Array Methods Β» JavaScript Spread Operator
Summary : in this tutorial, you will learn about the JavaScript spread operator that spreads out elements of an iterable object.
ES6 provides a new operator called spread operator that consists of three dots (...) . The spread operator allows you to spread out elements of an iterable object such as an array , map , or set . For example:
In this example, the three dots ( ... ) located in front of the odd array is the spread operator. The spread operator ( ... ) unpacks the elements of the odd array.
Note that ES6 also has the three dots ( ... ) which is a rest parameter that collects all remaining arguments of a function into an array.
In this example, the rest parameter ( ... ) collects the arguments 3, 4, and 5 into an array args . So the three dots ( ... ) represent both the spread operator and the rest parameter.
The rest parameters must be the last arguments of a function . However, the spread operator can be anywhere:
Note that ES2018 expands the spread operator to objects, which is known as object spread .
Letβs look at some scenarios where you can use the spread operators.
See the following compare() function compares two numbers:
In ES5, to pass an array of two numbers to the compare() function, you often use the apply() method as follows:
However, byΒ using the spread operator, you can pass an array of two numbers to the compare() function:
The spread operator spreads out the elements of the array so a is 1 and b is 2 in this case.
Sometimes, aΒ function may accept an indefinite number of arguments. Filling arguments from an array is not convenient.
For example, the push() method of an array object allows you to add one or more elements to an array. If you want to pass an array to the push() method, you need to use apply() method as follows:
The following example uses the spread operator to improve the readability of the code:
As you can see, using the spread operator is much cleaner.
The spread operator allows you to insert another array into the initialized array when you construct an array using the literal form. See the following example:
Also, you can use the spread operator to concatenate two or more arrays:
In addition, you can copy an array instance by using the spread operator:
Note that the spread operator only copies the array itself to the new one, not the elements. This means that the copy is shallow, not deep.
In this example, we constructed the chars array from individual strings. When we applied the spread operator to the 'BC' string, it spreads out each individual character of the string 'BC' into individual characters.
Copyright Β© 2022 by JavaScript Tutorial Website. All Right Reserved.
Katy Jayne Jules Jordan Porn
Masturbating 720 Hd
Tony Tucker Sensual Rain