Javascript Spread
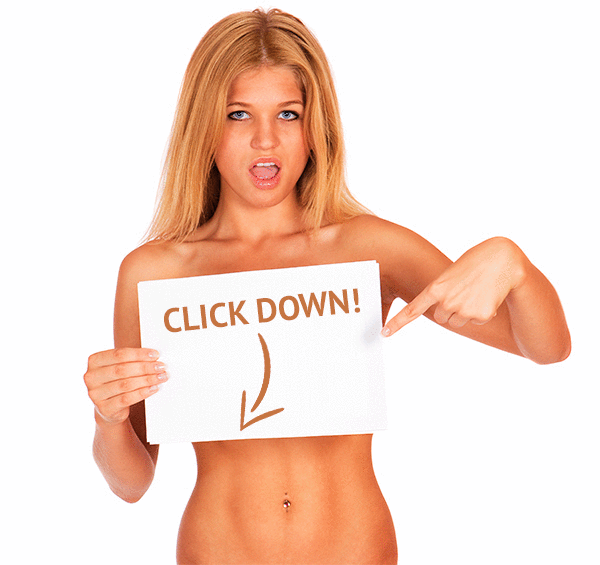
🛑 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Javascript Spread
const odd = [ 1 , 3 , 5 ];
const combined = [ 2 , 4 , 6 , ...odd];
console .log(combined);
function f ( a, b, ...args ) {
console .log(args);
}
f( 1 , 2 , 3 , 4 , 5 );
const odd = [ 1 , 3 , 5 ];
const combined = [...odd, 2 , 4 , 6 ];
console .log(combined);
const odd = [ 1 , 3 , 5 ];
const combined = [ 2 ,...odd, 4 , 6 ];
console .log(combined);
function compare ( a, b ) {
return a - b;
}
let result = compare.apply( null , [ 1 , 2 ]);
console .log(result); // -1
let result = compare(...[ 1 , 2 ]);
console .log(result); // -1
let rivers = [ 'Nile' , 'Ganges' , 'Yangte' ];
let moreRivers = [ 'Danube' , 'Amazon' ];
[].push.apply(rivers, moreRivers);
console .log(rivers);
let initialChars = [ 'A' , 'B' ];
let chars = [...initialChars, 'C' , 'D' ];
console .log(chars); // ["A", "B", "C", "D"]
let numbers = [ 1 , 2 ];
let moreNumbers = [ 3 , 4 ];
let allNumbers = [...numbers, ...moreNumbers];
console .log(allNumbers); // [1, 2, 3, 4]
let scores = [ 80 , 70 , 90 ];
let copiedScores = [...scores];
console .log(copiedScores); // [80, 70, 90]
let chars = [ 'A' , ... 'BC' , 'D' ];
console .log(chars); // ["A", "B", "C", "D"]
The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively.
Home » JavaScript Array Methods » JavaScript Spread Operator
Summary : in this tutorial, you will learn about the JavaScript spread operator that spreads out elements of an iterable object.
ES6 provides a new operator called spread operator that consists of three dots (...) . The spread operator allows you to spread out elements of an iterable object such as an array , map , or set . For example:
In this example, the three dots ( ... ) located in front of the odd array is the spread operator. The spread operator ( ... ) unpacks the elements of the odd array.
Note that ES6 also has the three dots ( ... ) which is a rest parameter that collects all remaining arguments of a function into an array.
In this example, the rest parameter ( ... ) collects the arguments 3, 4, and 5 into an array args . So the three dots ( ... ) represent both the spread operator and the rest parameter.
The rest parameters must be the last arguments of a function . However, the spread operator can be anywhere:
Note that ES2018 expands the spread operator to objects, which is known as object spread .
Let’s look at some scenarios where you can use the spread operators.
See the following compare() function compares two numbers:
In ES5, to pass an array of two numbers to the compare() function, you often use the apply() method as follows:
However, by using the spread operator, you can pass an array of two numbers to the compare() function:
The spread operator spreads out the elements of the array so a is 1 and b is 2 in this case.
Sometimes, a function may accept an indefinite number of arguments. Filling arguments from an array is not convenient.
For example, the push() method of an array object allows you to add one or more elements to an array. If you want to pass an array to the push() method, you need to use apply() method as follows:
The following example uses the spread operator to improve the readability of the code:
As you can see, using the spread operator is much cleaner.
The spread operator allows you to insert another array into the initialized array when you construct an array using the literal form. See the following example:
Also, you can use the spread operator to concatenate two or more arrays:
In addition, you can copy an array instance by using the spread operator:
Note that the spread operator only copies the array itself to the new one, not the elements. This means that the copy is shallow, not deep.
In this example, we constructed the chars array from individual strings. When we applied the spread operator to the 'BC' string, it spreads out each individual character of the string 'BC' into individual characters.
Copyright © 2022 by JavaScript Tutorial Website. All Right Reserved.
Browse Bootcamps ▾
〈 Back
Popular Bootcamps ›
Bootcamps Near You ›
Explore by Subject ›
〈 Back
Browse Popular Bootcamps
Best Coding Bootcamps
Best Online Bootcamps
Best Web Design Bootcamps
Best Data Science Bootcamps
Best Technology Sales Bootcamps
Best Data Analytics Bootcamps
Best Cybersecurity Bootcamps
Best Project Management Bootcamps
〈 Back
Find a Bootcamp
New York
Los Angeles
San Francisco
Atlanta
Chicago
Seattle
San Diego
Houston
View All Locations
〈 Back
Explore by Subject
Python
Web Development
Digital Marketing
iOS
Java
JavaScript
SQL
Machine Learning
View All Subjects
Learn for Free ▾
〈 Back
Browse Questions from Others ›
Browse Top Careers ›
Choosing a Bootcamp ›
Paying for a Bootcamp ›
〈 Back
Explore Questions from Others
Bootcamps 101
Data Science
Software Engineering
Full-Stack Development
JavaScript
Job Search
Career Changes
View All Career Discussions
〈 Back
Browse Top Careers
Software Engineering
Web Development
Mobile App Development
Data Science
Cybersecurity
Product Management
Digital Marketing
UX/UI Design
View All Careers
〈 Back
Choosing a Bootcamp
What is a Coding Bootcamp?
Are Coding Bootcamps Worth It?
How to Choose a Coding Bootcamp
Best Online Coding Bootcamps and Courses
Best Free Bootcamps and Coding Training
Coding Bootcamp vs. Community College
Coding Bootcamp vs. Self-Learning
Bootcamps vs. Certifications: Compared
〈 Back
Paying for a Bootcamp
How to Pay for Coding Bootcamp
Ultimate Guide to Coding Bootcamp Loans
Best Coding Bootcamp Scholarships and Grants
Education Stipends for Coding Bootcamps
Get Your Coding Bootcamp Sponsored by Your Employer
GI Bill and Coding Bootcamps
Start My Journey ▾
〈 Back
Community
Career Guides
Schools & Application
Prep Courses & Scholarships
Resources
About ▾
〈 Back
Company
Jobs
Values
Publication
Press
Reskill America
Partner With Us
Bootcamps ▾
Graduate Stories
Partner Spotlights
Bootcamp Prep
Bootcamp Admissions
University Bootcamps
Research
Coding ▾
Python
Git
JavaScript
CSS
Java
HTML
C++
SQL
Ruby
Coding Tools
Tech Fields ▾
Software Engineering
Web Development
Data Science
Design
Tech Guides
Tech Resources
Tech Tools
Career Resources ▾
Career Advice
Online Learning
Resume
Interviews
Tech Jobs
Internships
Apprenticeships
Tech Salaries
Job Market
Higher Ed ▾
Degrees
Associate Degree
Bachelor's Degree
Master's Degree
Doctoral
University Admissions
Best Schools
Certifications
Ed Financing ▾
Bootcamp Financing
Higher Ed Financing
Scholarships
Financial Aid
var names = ["John", "Lisa", "Harry"];
var new_names = [...names, "Leslie"];
console.log(new_names);
const lemon_drizzle = {
name: "Lemon Drizzle",
price: 1.95,
vegan: true
}
const new_lemon_drizzle = {...lemon_drizzle};
console.log(new_lemon_drizzle);
{ name: "Lemon Drizzle", price: 1.95, vegan: true }
const cupcakes = [
'Lemon Drizzle',
'Chocolate Chip',
'Vanilla Iced'
];
const new_cupcakes = [...cupcakes, 'Red Velvet', 'Raspberry Dark Chocolate'];
console.log(new_cupcakes);
["Lemon Drizzle", "Chocolate Chip", "Vanilla Iced", "Red Velvet", "Raspberry Dark Chocolate"]
const old_menu = [
'Lemon Drizzle',
'Chocolate Chip',
'Vanilla Iced'
];
const new_menu = [
'Red Velvet',
'Raspberry Dark Chocolate'
]
const final_menu = [...old_menu, ...new_menu];
console.log(final_menu);
["Lemon Drizzle", "Chocolate Chip", "Vanilla Iced", "Red Velvet", "Raspberry Dark Chocolate"]
function placeOrder(name, order, price) {
console.log('Name: ' + name);
console.log('Order: ' + order);
console.log('Price: ' + price);
}
placeOrder('Geoff', 'Red Velvet', 1.85);
Name: Geoff
Order: Red Velvet
Price 1.85
function placeOrder(name, order, price) {
console.log('Name: ' + name);
console.log('Order: ' + order);
console.log('Price: ' + price);
}
const order = ['Geoff', 'Red Velvet', 1.85];
placeOrder(...order);
Name: Geoff
Order: Red Velvet
Price 1.85
Apply to top tech training programs in one click
Get Matched
Home
About
Careers
Mission
Resource Center
Press
Sitemap
Terms & Conditions
© 2022 Career Karma
Rankings
Best Coding Bootcamps
Best Online Bootcamps
Best Web Design Bootcamps
Best Data Science Bootcamps
Best Data Analytics Bootcamps
Best Cybersecurity Bootcamps
Best ISA Bootcamps
Locations
San Francisco Bootcamps
New York Bootcamps
Los Angeles Bootcamps
Chicago Bootcamps
Seattle Bootcamps
Atlanta Bootcamps
Austin Bootcamps
See all
Careers
Software Engineering
UX/UI Design
Data Science
Web Development
Cybersecurity
Product Management
See all
Comparisons
Flatiron School vs Fullstack Academy
Hack Reactor vs App Academy
Fullstack Academy vs Hack Reactor
Flatiron School vs Thinkful
General Assembly vs Flatiron School
App Academy vs Lambda School
General Assembly vs Hack Reactor
Springboard vs Thinkful
See all
Schools
Kenzie Academy
Flatiron School
Thinkful
General Assembly
Springboard
Galvanize
Hack Reactor
App Academy
Bloc
See all
Subjects
JavaScript
Python
Ruby
Java
Swift
Android
.NET
React
Angular
See all
Explore your training options in 10 minutes Get Matched
James Gallagher
- January 04, 2021
avaScript spread operator expands an array in syntax that accepts arguments. The spread operator is commonly used to duplicate objects, merge arrays or pass the contents of a list into a function.
The spread operator. No, it’s got nothing to do with toast. In JavaScript, the spread operator has a specific meaning: it’s a way to access the contents of an iterable object. While this may not sound as fun as pasting a spread on toast, it’s an incredibly useful tool to know about.
In this guide, we’re going to talk about the JavaScript spread operator and how it works. We’ll walk through a few examples of common use cases to help you get started.
By continuing you agree to our
Terms of Service
and
Privacy Policy
, and you consent to receive offers and opportunities from Career Karma by telephone, text message, and email.
A JavaScript spread operator lets you access the contents of an iterable object. The spread operator is a set of three dots (an ellipsis) followed by the name of the iterable you want to access. This operator was introduced in JavaScript ES6.
The three types of iterable objects are arrays, object literals, and strings. Using a for loop, you can loop through all of these types of data and run a common process on them.
Iterable objects are useful because you can execute the same process on them multiple times. You can loop through a string and replace certain characters. You can loop through an array and create a total of all the values stored in the array.
The syntax for the spread operator is:
In this syntax, we use …names to pass the contents of our “names” list into a list called “new_names”. The “new_names” list contains all the items in the “names” list, as well as a new name: Leslie.
Three common use cases for the spread operator are to:
The spread syntax represents all the individual elements in a list.
The spread operator is an effective method of duplicating an iterable. While there are other ways to approach this problem, the spread operator is really easy to use. To create a copy of an iterable, specify three dots and the name of the array you want to create.
In our last example, we showed how this works with an array. We can also use the spread operator to duplicate JavaScript objects:
Our code prints the following to the
JavaScript console
:
There are a few important distinctions we need to make in this example. We are creating a copy of a JavaScript object. This means that we need to use curly braces ({}) instead of square brackets ([]).
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Second, instead of specifying a single array, we have specified an object. This object contains three keys and values, each of which is related to our “Lemon Drizzle” cupcake.
The use cases of the spread operator don’t stop with copying iterables! There’s more to explore. The spread operator is commonly used to add items from one iterable to the next. Consider the following example:
We’ve created a copy of our original
JavaScript array
called “new_cupcakes”, which also includes a few additional values that we’ve created.
You could use the same syntax to merge two iterables together as well. All you would need to do is specify the two iterables inside square brackets using the spread operator:
Sarah Vandella Lesbian
Private Studio
Little Naked Models