Java Private
π ALL INFORMATION CLICK HERE ππ»ππ»ππ»
Java Private
Java Training (40 Courses, 29 Projects, 4 Quizzes) 40 Online Courses | 29 Hands-on Projects | 285+ Hours | Verifiable Certificate of Completion | Lifetime Access | 4 Quizzes with Solutions 4.8 (8,141 ratings)
Course Price $129 $599 View Course
The keyword βprivateβ in Java is used to establish the limitation of accessibility of the class, method or a variable in the java code block. If a class, method or a variable is entitled as private in the program, that means that particular class, method or the variable cannot be accessed by outside the class or method, unlike the public method. The Private keyword is typically used in Java in a fully encapsulated class.
Private Keyword in Java works within a particular class. It canβt be accessed outside of the class. It doesnβt work outside the class/classes and interface/ interfaces. Private Keyword works well if the members of the class are PRIVATE and that too in a fully encapsulated class. Private Keyword or variable or method can also be overridden to the sub-class/classes using some access modifiers to invoke PRIVATE METHOD outside of the class. With this, Private Keyword also works outside of the class only using the Private Access Modifiers.
Here are some examples of private modifier which are as follows:
Here we are illustrating the example of Private Access Modifier which shows compilation error because of private data member accessing from the class AB which is shown in the below example. Private methods or Private members can only be accessible within the particular class.
class AB{
private double number = 100;
private int squares(int a){
return a*a;
}
}
public class Main{
public static void main(String args[]){
AB obj = new AB();
System.out.println(obj.number);
System.out.println(obj.squares(10));
}
}
This is the example of illustrating the use of the PRIVATE keyword with the program below:
class Emp{
private int id1;
private String name14;
private int age14;
public int getId1() {
return id1;
}
public void setId(int id1) {
this.id1 = id1;
}
public String getName14() {
return name14;
}
public void setName14(String name14) {
this.name14 = name14;
}
public int getAge14() {
return age14;
}
public void setAge14(int age14) {
this.age14 = age14;
}
}
public class Main{
public static void main(String args[]){
Emp e=new Emp();
e.setId(1473);
e.setName14("Pavan Kumar Sake");
e.setAge14(24);
System.out.println(e.getId1()+" "+e.getName14()+" "+e.getAge14());
}
}
Here in this example, you can see how the PRIVATE METHOD is overridden to the sub-class using the access modifier which is the default. We are not even allowed to invoke the parent class method/methods from the sub-class.
class ABC{
private void msg()
{
System.out.println("Hey Buddy this is the parent class method"); //Output of the Private Method
}
}
public class Main extends ABC{ // Private method is overridden using the access modifier
void msg()
{
System.out.println("Buddy this is the child class method");
}
public static void main(String args[]){
Main obj=new Main();
obj.msg();
}
}
Here in this example, I am illustrating that the PRIVATE METHOD cannot be invoked/called outside of the class. Here now the private method is calling from the Outside class by changing class runtime behavior.
import java.lang.reflect.Method;
class ABC {
private void display()
{
System.out.println("Hey Now private method is invoked/Called");
}
}
public class Main{
public static void main(String[] args)throws Exception{
Class d = Class.forName("ABC");
Object p= d.newInstance();
Method n =d.getDeclaredMethod("display", null);
n.setAccessible(true);
n.invoke(p, null);
}
}
This is an example of a private method and field in Java Programming Language . Here private method/methods use static binding at the compile-time and it even canβt be overridden. Donβt confuse with the Private variable output because the Private variable is actually accessible inside the inner class/classes. If PRIVATE variable/variables are invoked/called outside of the class definitely the compiler will produce an error.
public class Main {
private String i_m_private1 = " \n Hey Buddy I am a private member and i am not even accessible outside of this Class";
private void privateMethod1() {
System.out.println("Outer Class Private Method");
}
public static void main(String args[]) {
Main outerClass = new Main();
NestedClass nestc = outerClass.new NestedClass();
nestc.showPrivate(); //This syntax shows private method/methods are accessible inside the class/inner class.
outerClass = nestc;
nestc.privateMethod1(); //It don't call/invoke private method from the inner class because
// you can not override the private method inside the inner class.
}
class NestedClass extends Main {
public void showPrivate() {
System.out.println("Now we are going to access Outer Class Private Method: " + i_m_private1);
privateMethod1();
}
private void privateMethod1() {
System.out.println("Nested Class's Private Method");
}
}
}
Here we are going to explain the advantages of using Private methods/fields in Java below.
Here are some rules and regulations for private that you should know.
Coming to the end of the main topic we actually happy to know how helpful and easy using Private Keyword in Java. In this article, I hope you understand what is private keyword, private variable, access modifier, private constructor, How to use these private keywords in Programs.
This is a guide to Private constructor in java. Here we discuss the basic concept, working, advantages, rules, and regulations of private in java along with their examples and implementation. You may also look at the following articles to learn more β
Java Training (40 Courses, 29 Projects, 4 Quizzes)
Verifiable Certificate of Completion
Β© 2020 - EDUCBA. ALL RIGHTS RESERVED. THE CERTIFICATION NAMES ARE THE TRADEMARKS OF THEIR RESPECTIVE OWNERS.
This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy
Special Offer - Java Training (40 Courses, 29 Projects, 4 Quizzes) Learn More
Java β private β Access Modifier | Baeldung
Private in Java | Comprehensive Guide to Private in Java with Examples
Controlling Access to Members of a Class (The Java β’ Tutorials > Learning...
Java Access Modifiers - Public, Private , Protected & Default
Java private , protected, public and default
Controlling Access to Members of a Class
Home Page
>
Learning the Java Language
>
Classes and Objects
Controlling Access to Members of a Class
Β« Previous
β’
Trail
β’
Next Β»
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Access level modifiers determine whether other classes can use a particular field or invoke a particular method. There are two levels of access control:
A class may be declared with the modifier public , in which case that class is visible to all classes everywhere. If a class has no modifier (the default, also known as package-private ), it is visible only within its own package (packages are named groups of related classes β you will learn about them in a later
lesson.)
At the member level, you can also use the public modifier or no modifier ( package-private ) just as with top-level classes, and with the same meaning. For members, there are two additional access modifiers: private and protected . The private modifier specifies that the member can only be accessed in its own class. The protected modifier specifies that the member can only be accessed within its own package (as with package-private ) and, in addition, by a subclass of its class in another package.
The following table shows the access to members permitted by each modifier.
The first data column indicates whether the class itself has access to the member defined by the access level. As you can see, a class always has access to its own members. The second column indicates whether classes in the same package as the class (regardless of their parentage) have access to the member. The third column indicates whether subclasses of the class declared outside this package have access to the member. The fourth column indicates whether all classes have access to the member.
Access levels affect you in two ways. First, when you use classes that come from another source, such as the classes in the Java platform, access levels determine which members of those classes your own classes can use. Second, when you write a class, you need to decide what access level every member variable and every method in your class should have.
Let's look at a collection of classes and see how access levels affect visibility.
The following figure shows the four classes in this example and how they are related.
Classes and Packages of the Example Used to Illustrate Access Levels
The following table shows where the members of the Alpha class are visible for each of the access modifiers that can be applied to them.
If other programmers use your class, you want to ensure that errors from misuse cannot happen. Access levels can help you do this.
Overwatch 1920
Brutal Ass
Muscle Jock
Outdoor Wall Light
Outdoor Inn Restaurant

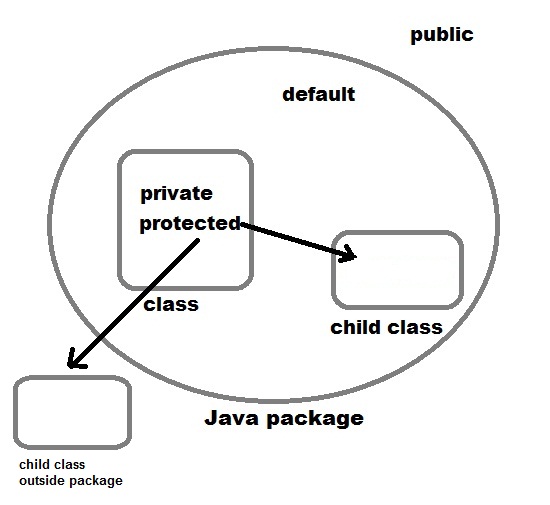

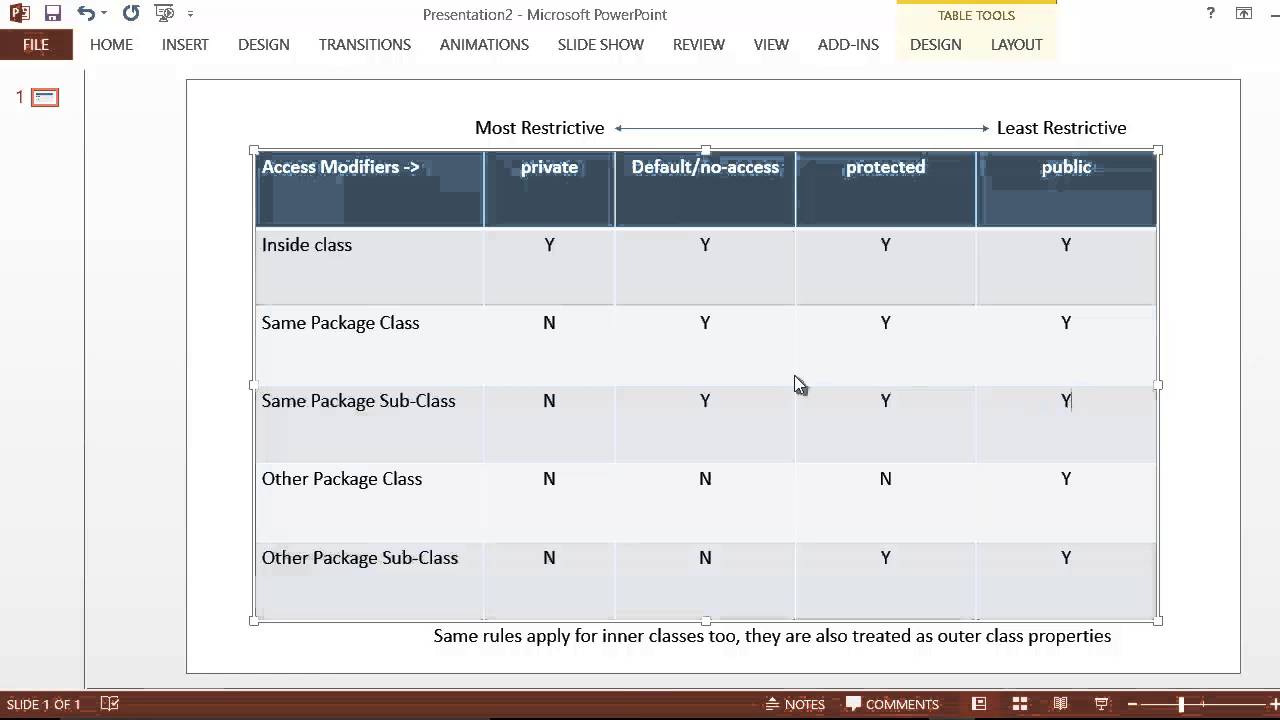
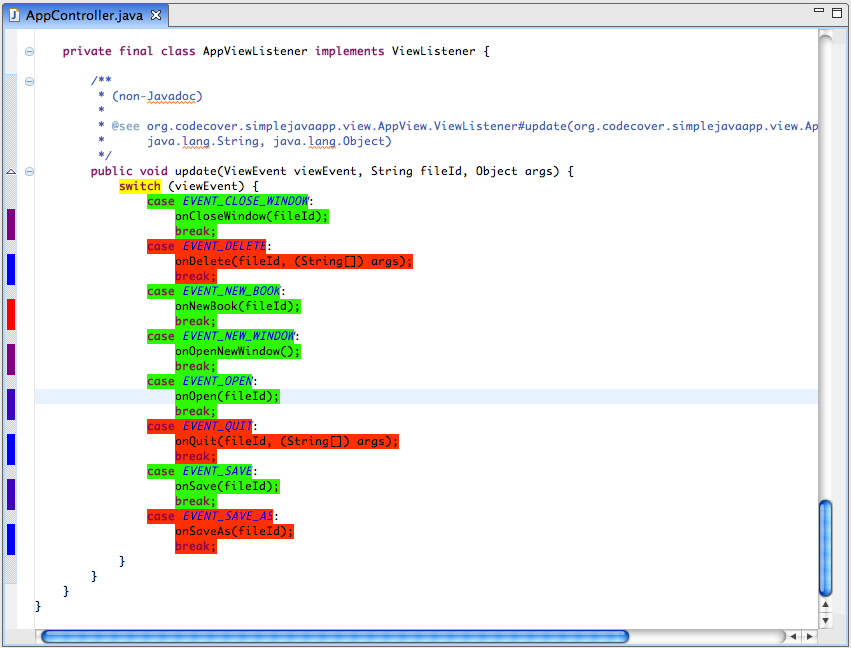
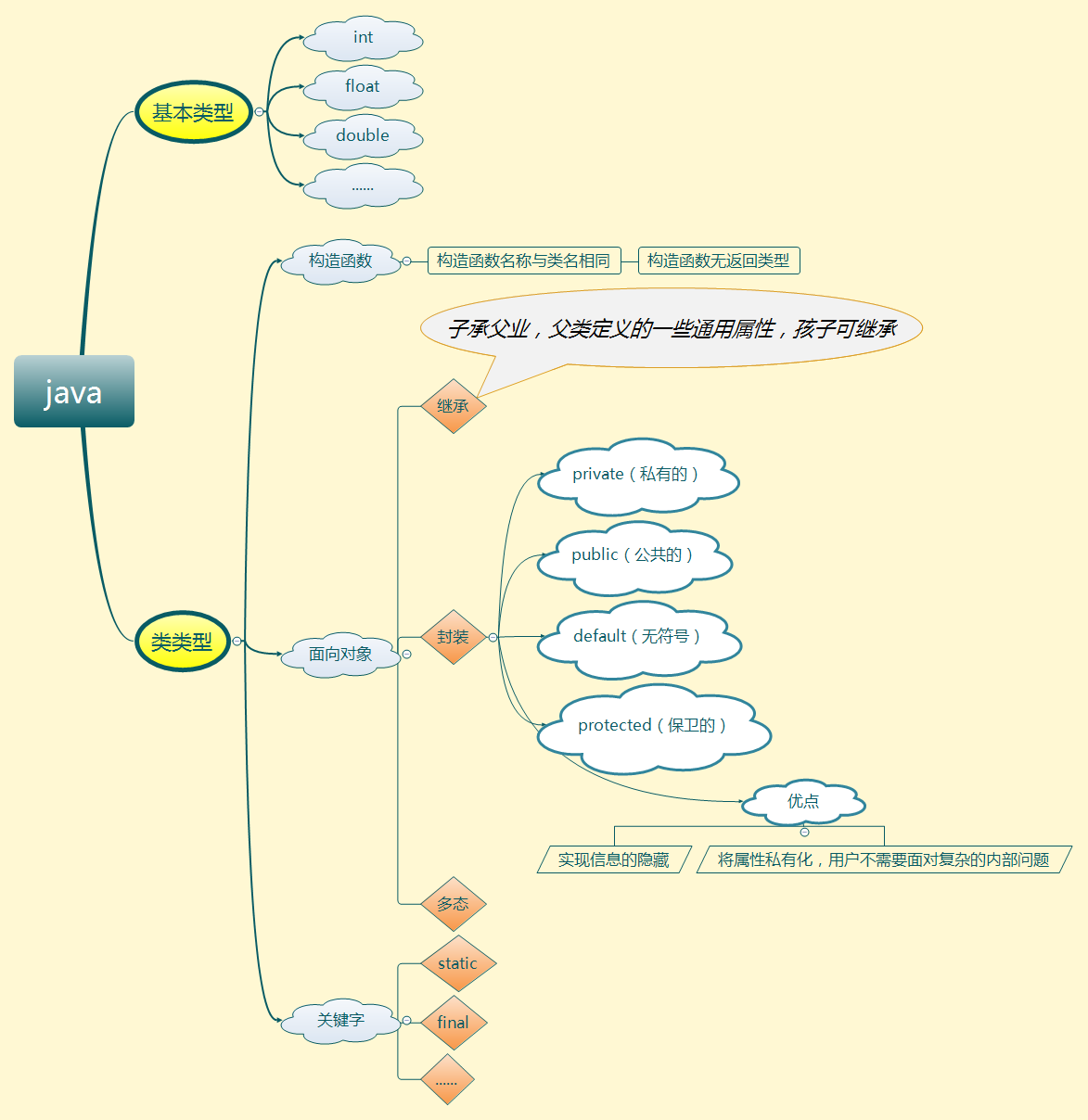

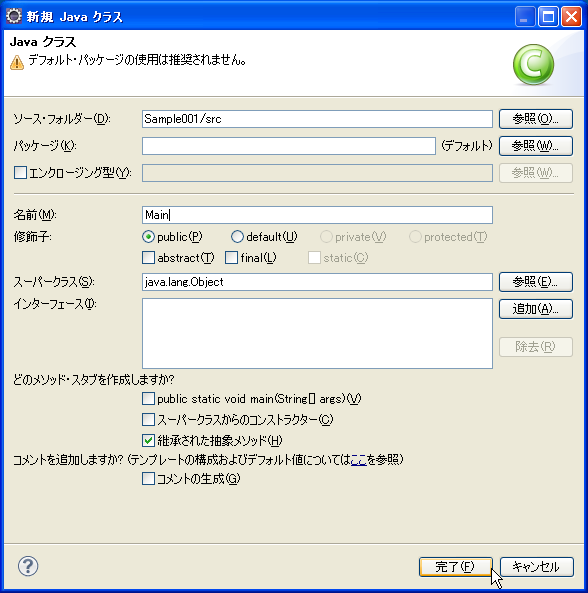
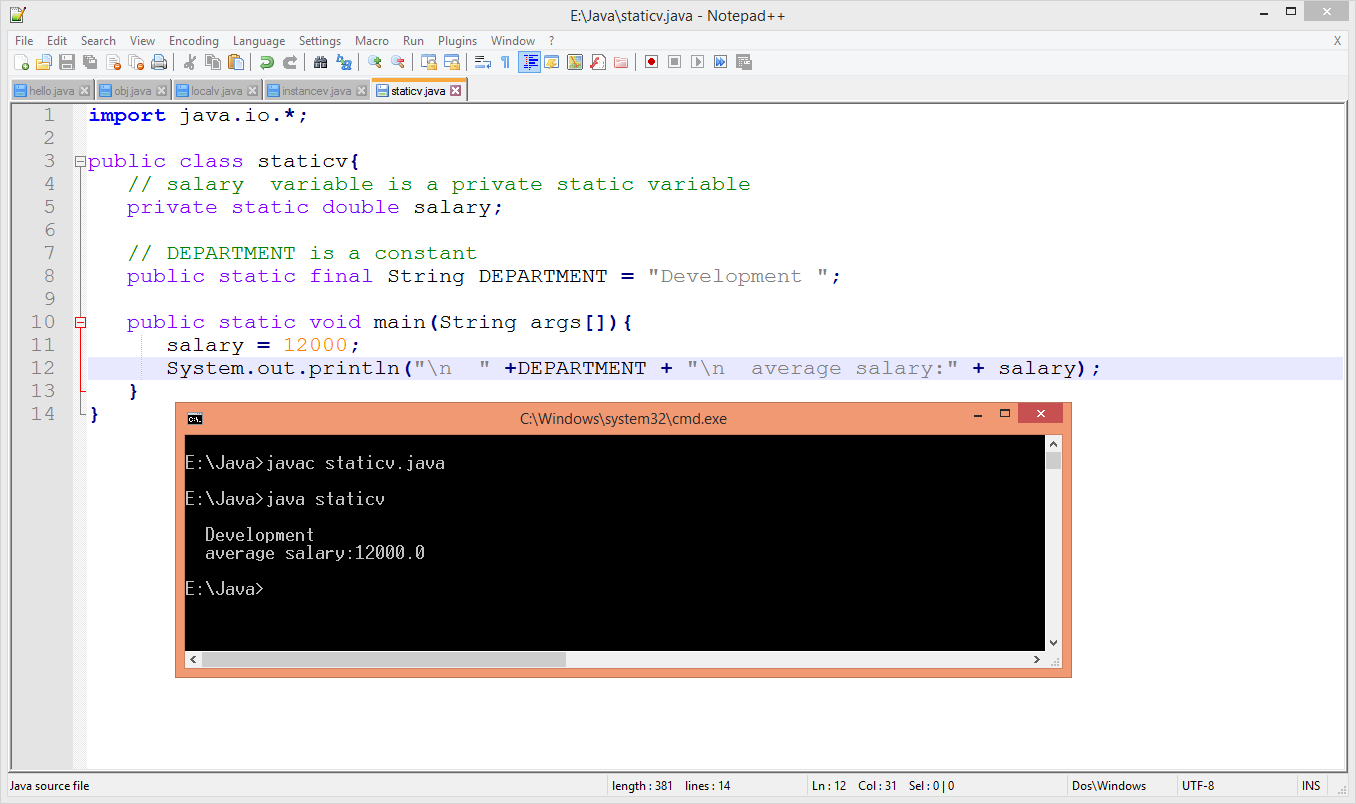
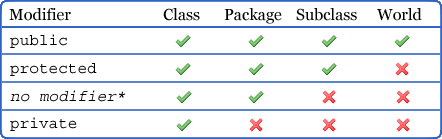
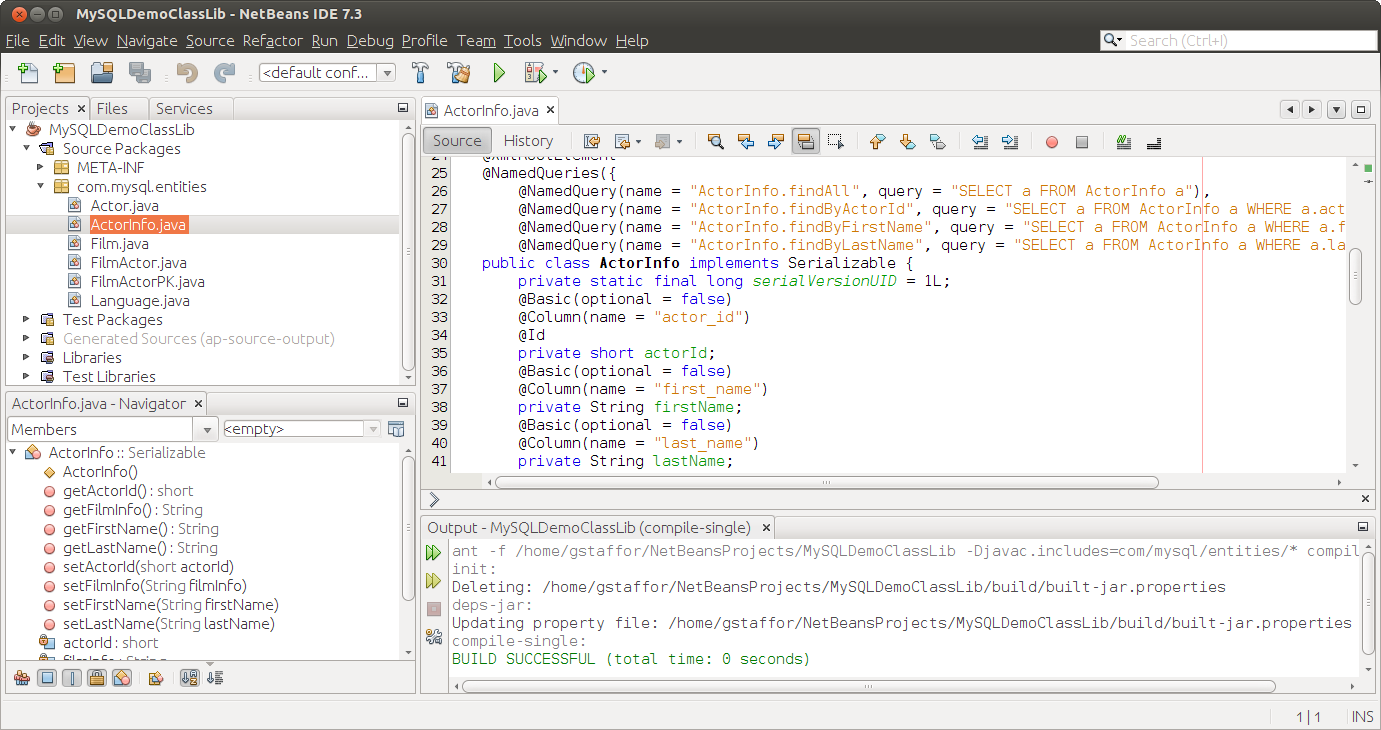

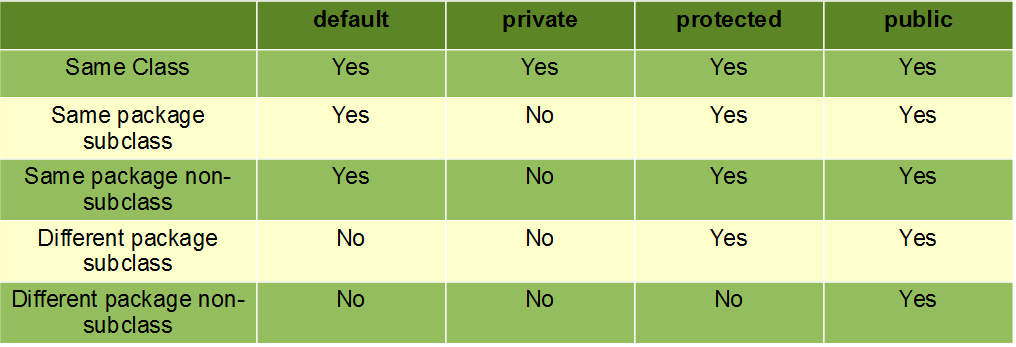

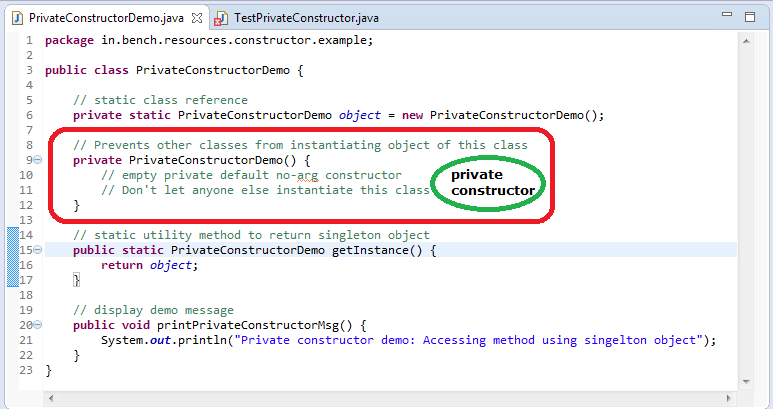
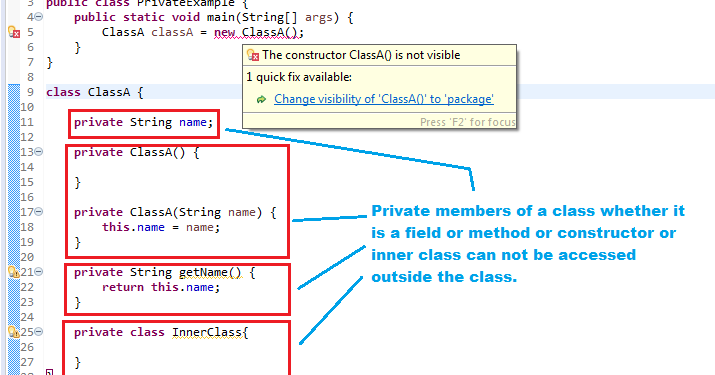
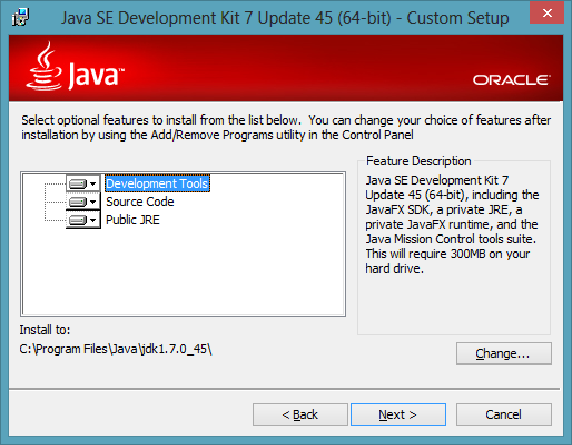
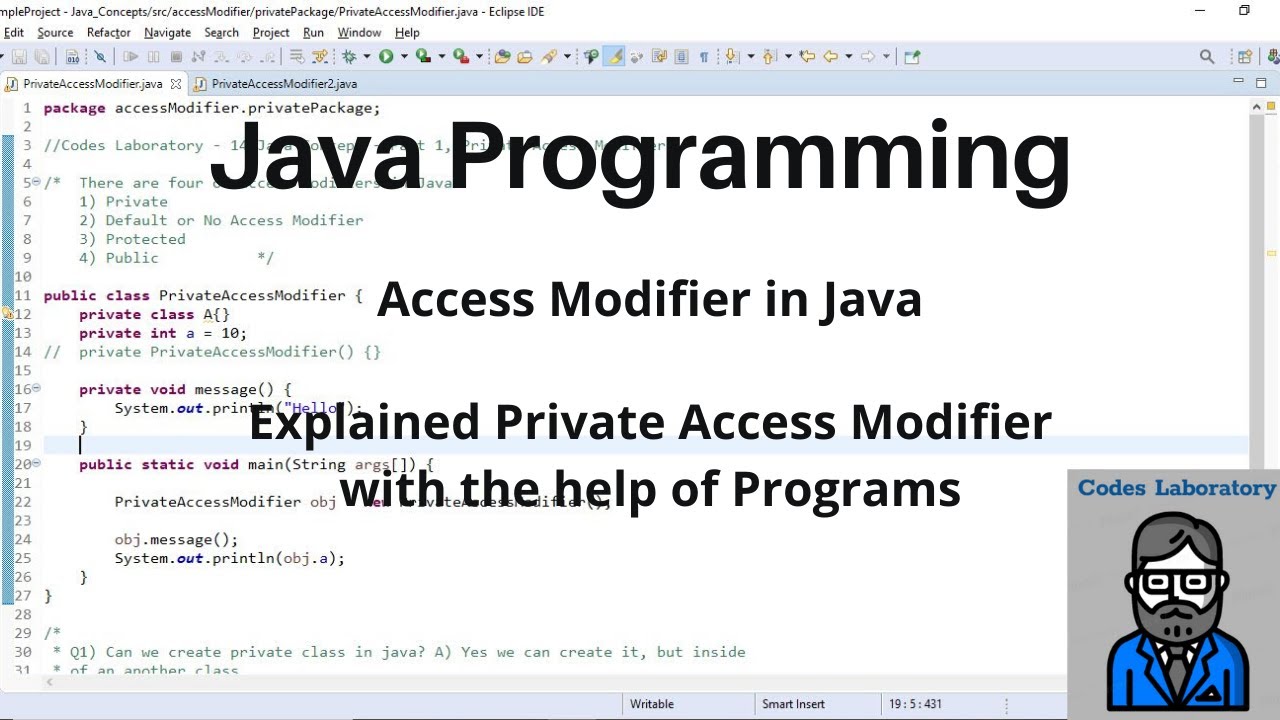
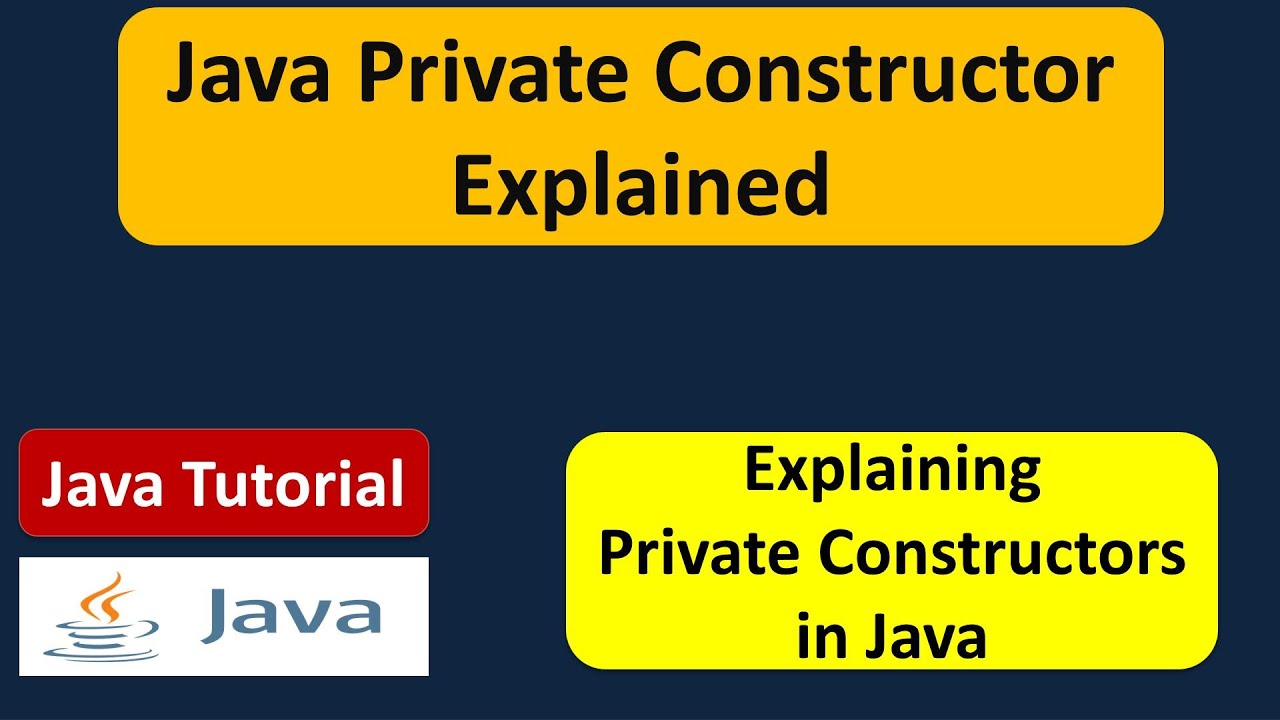
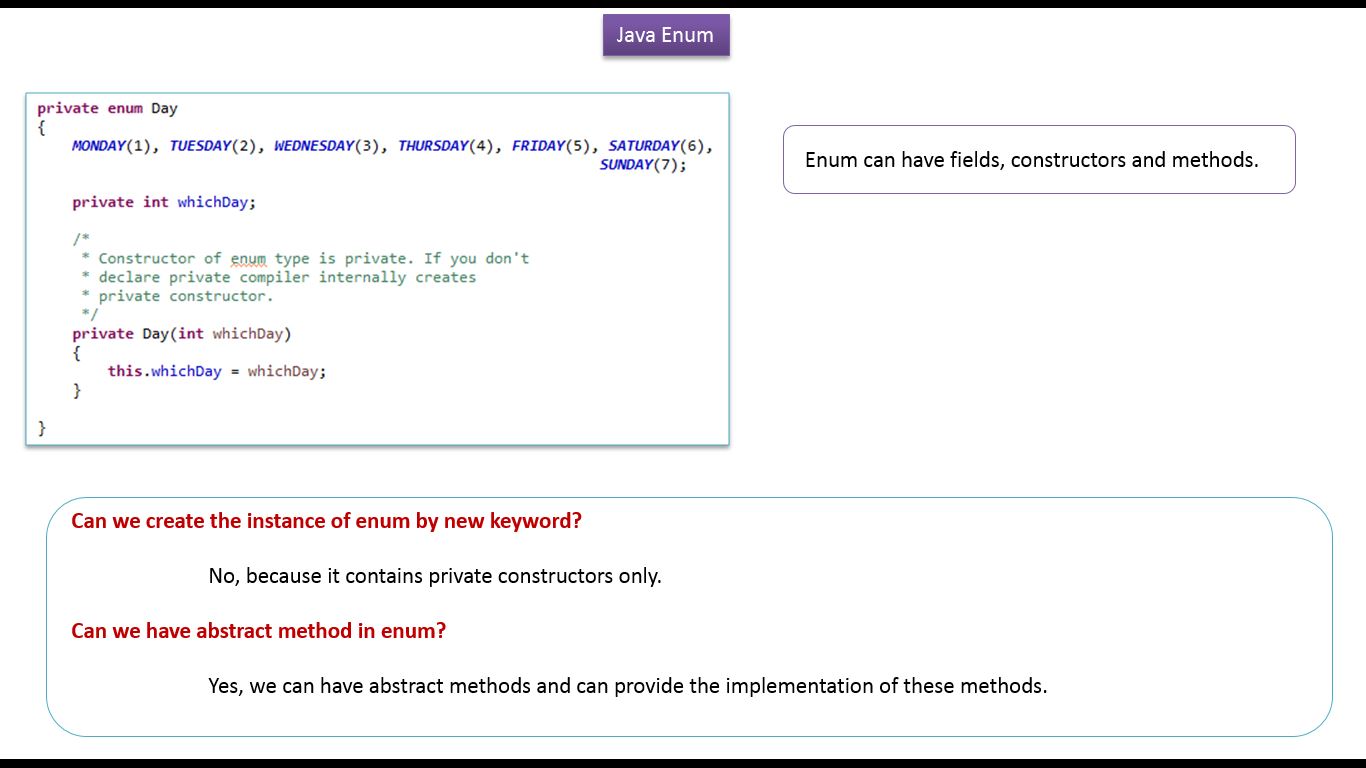

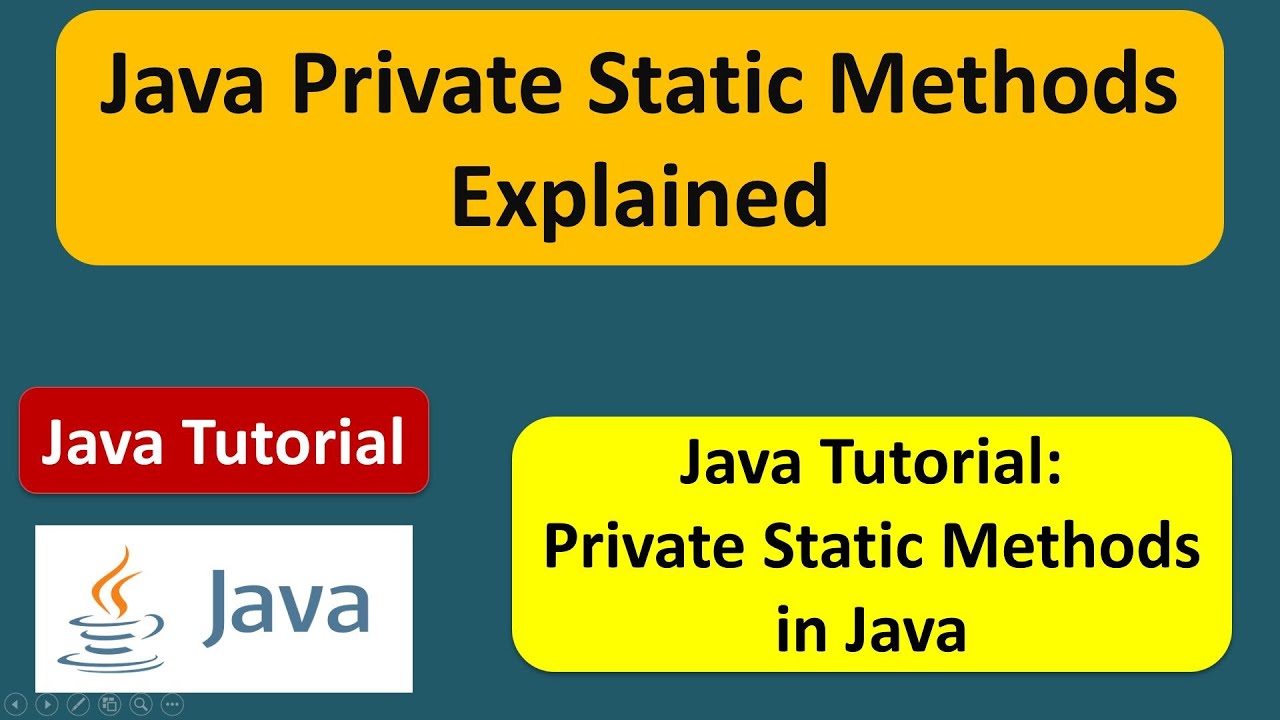
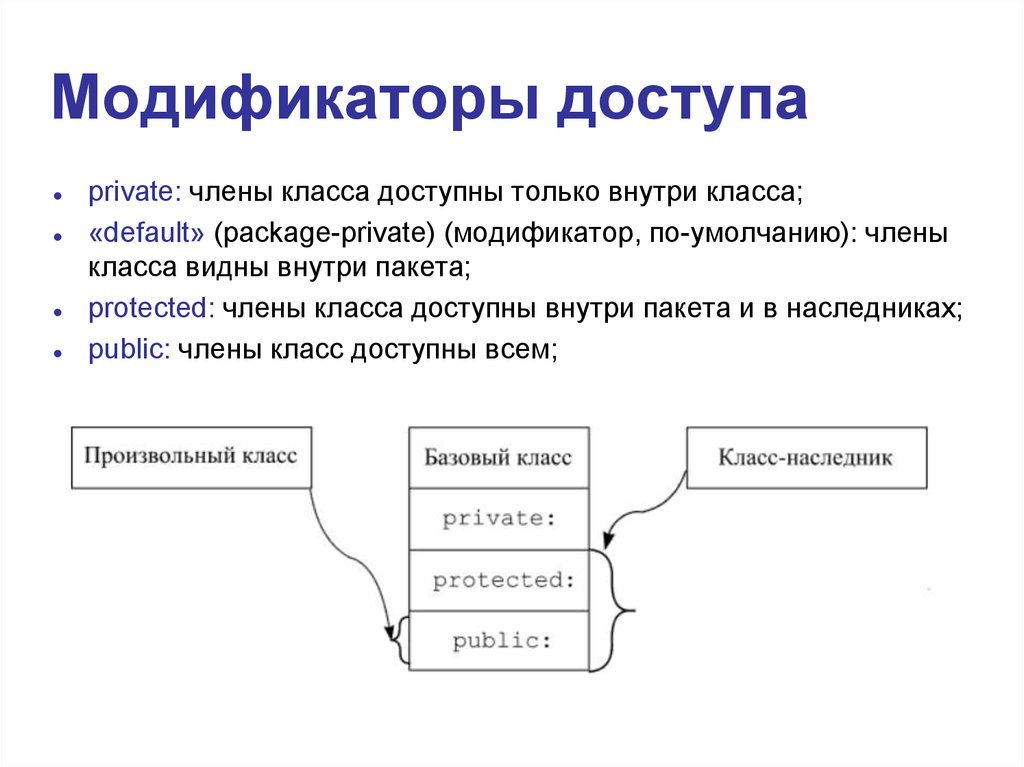

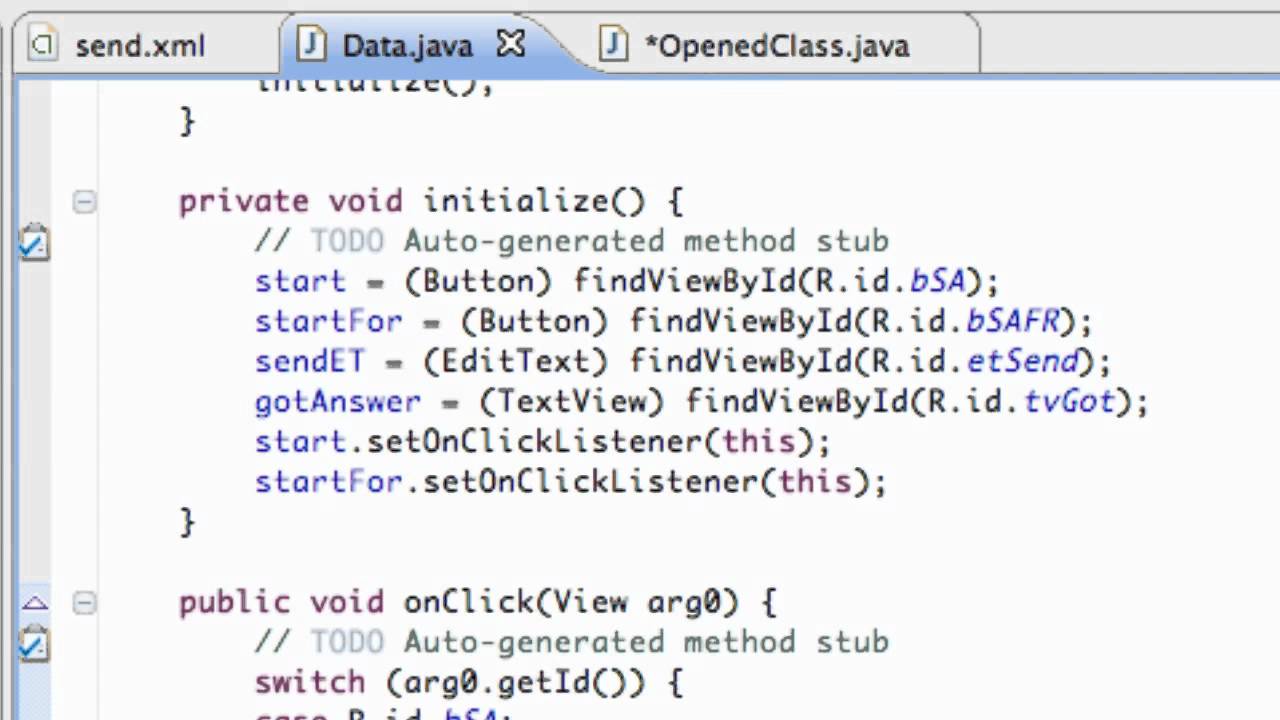
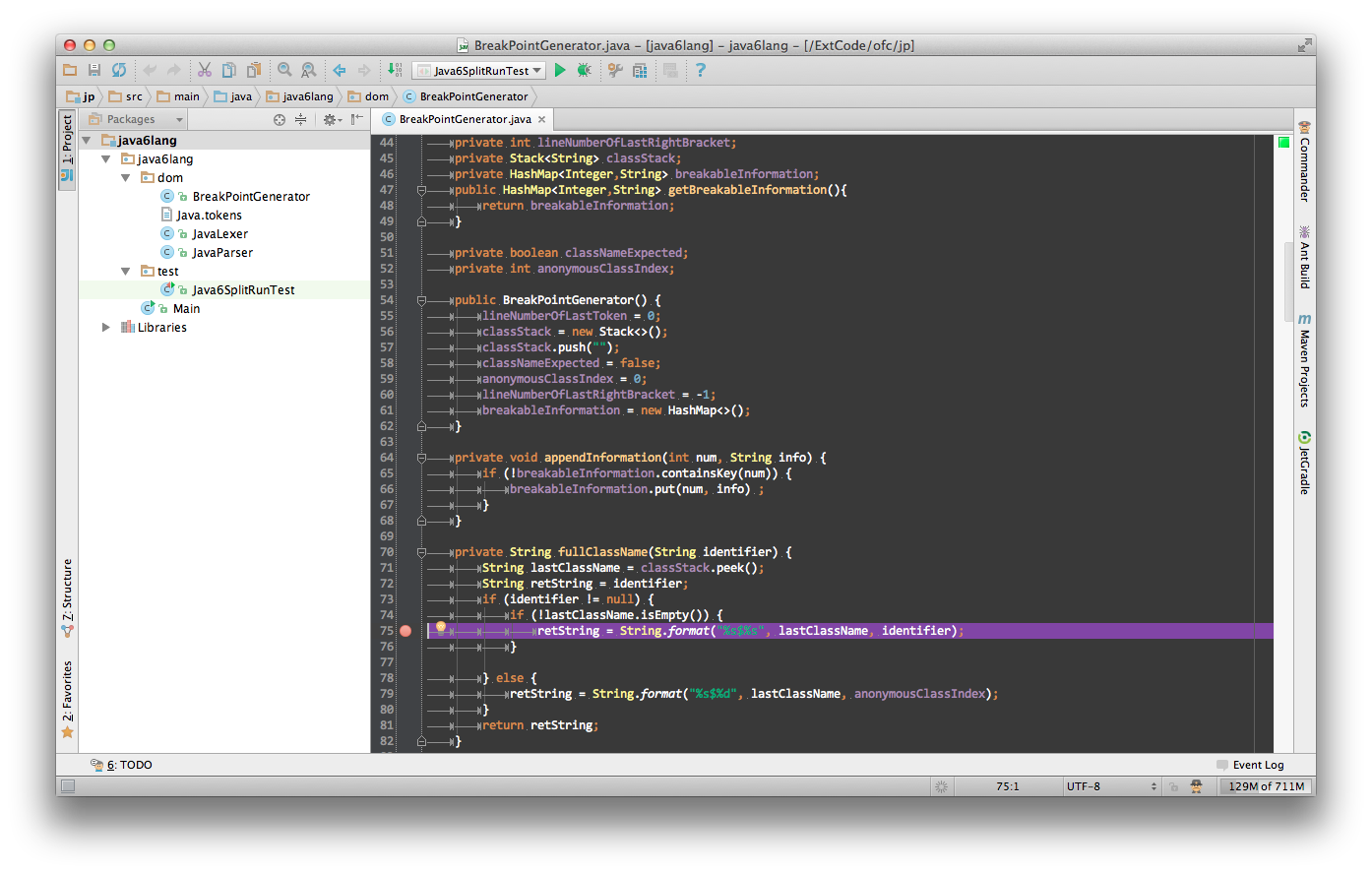
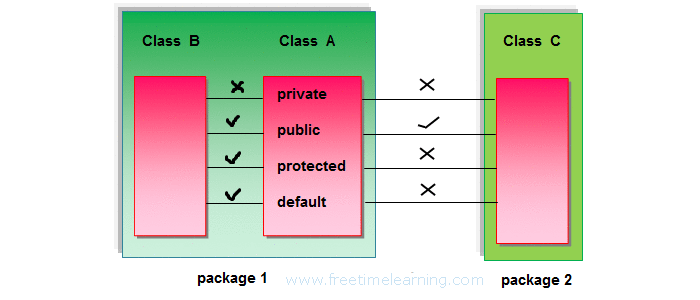



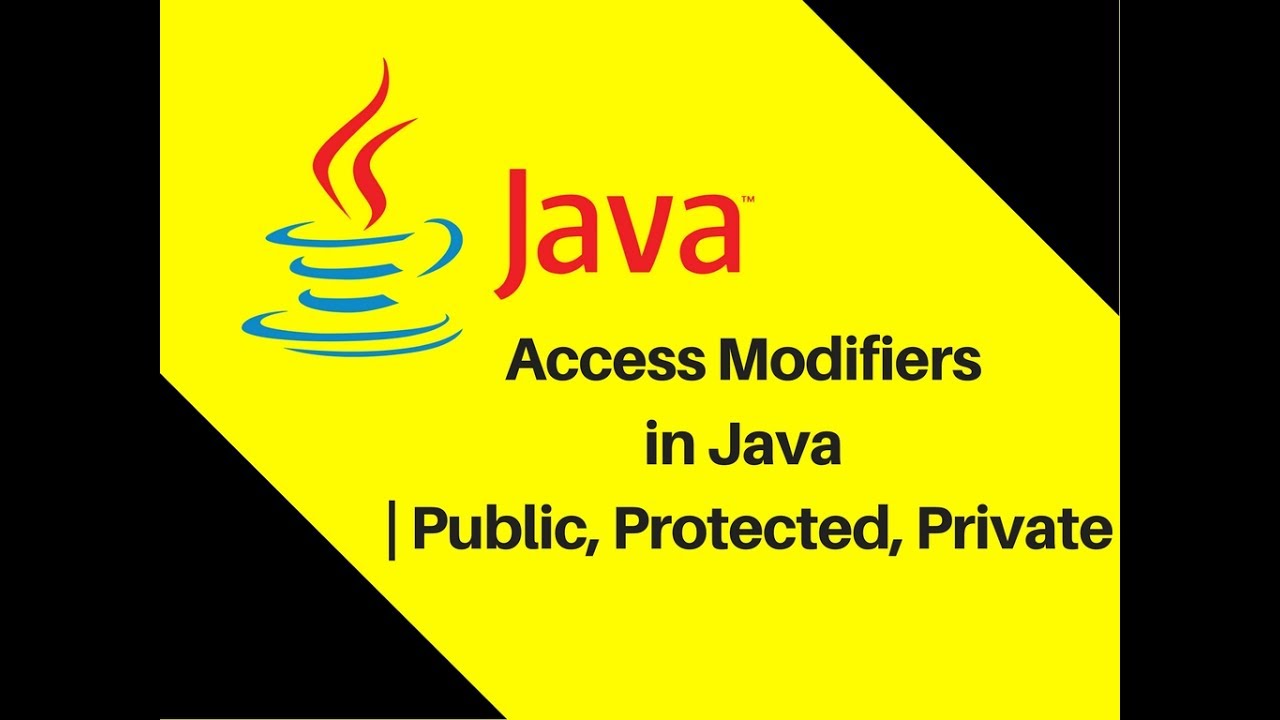
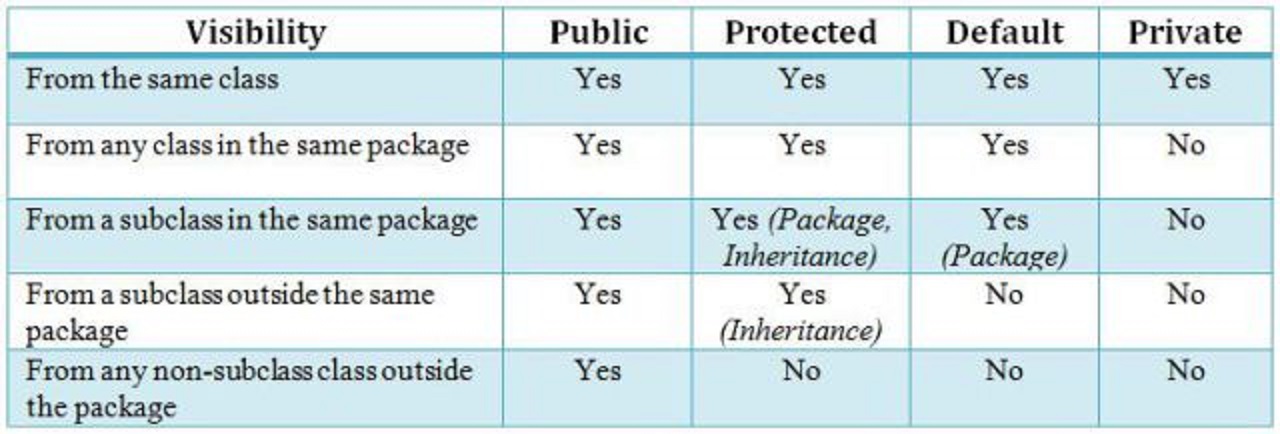
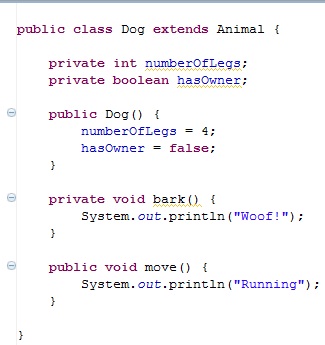

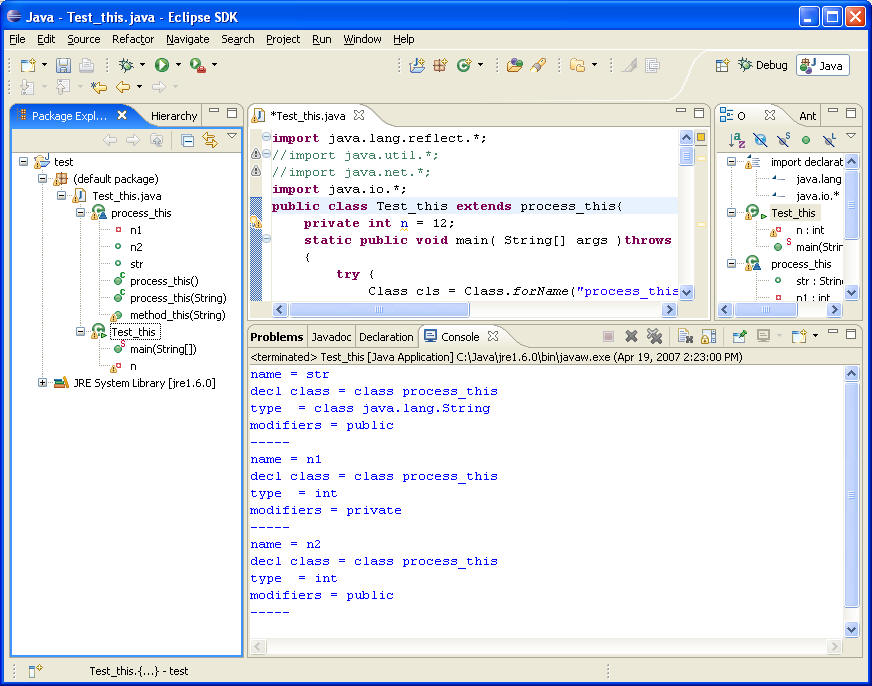
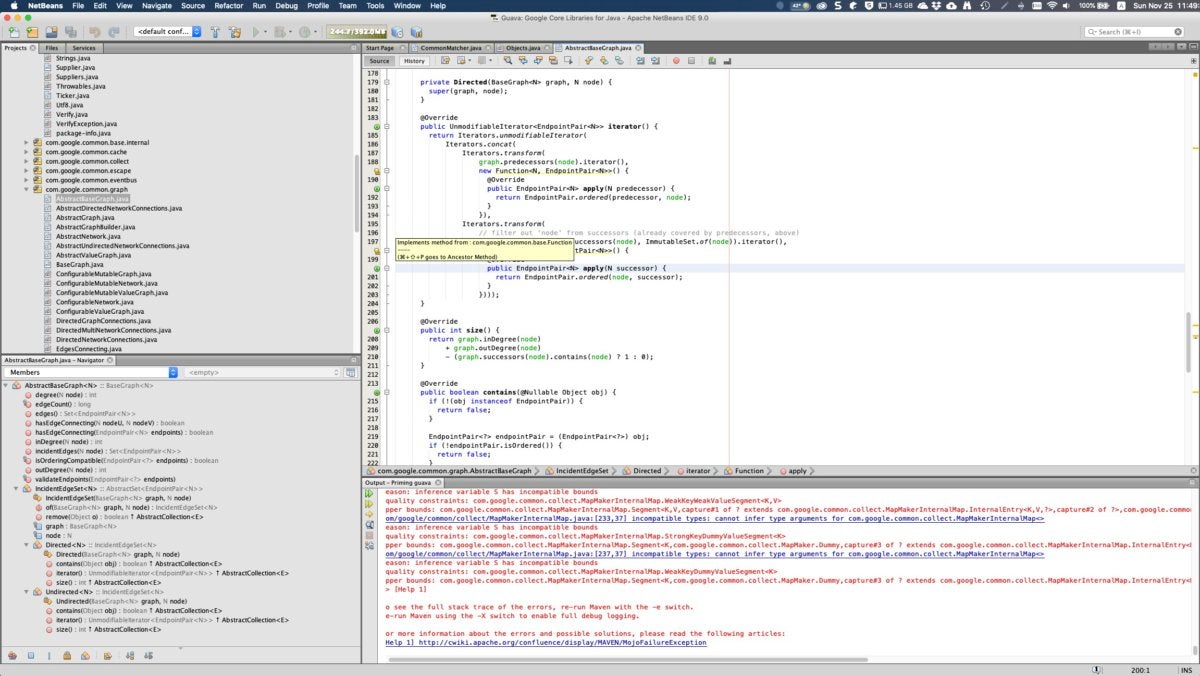
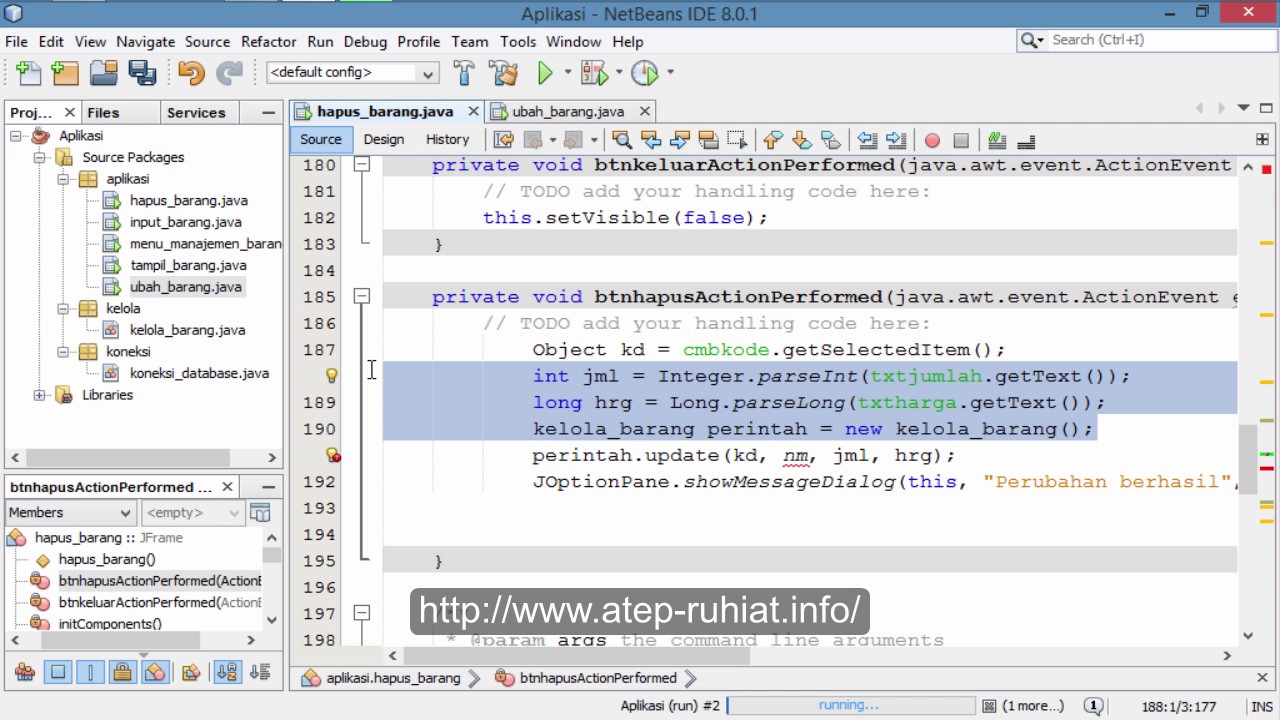

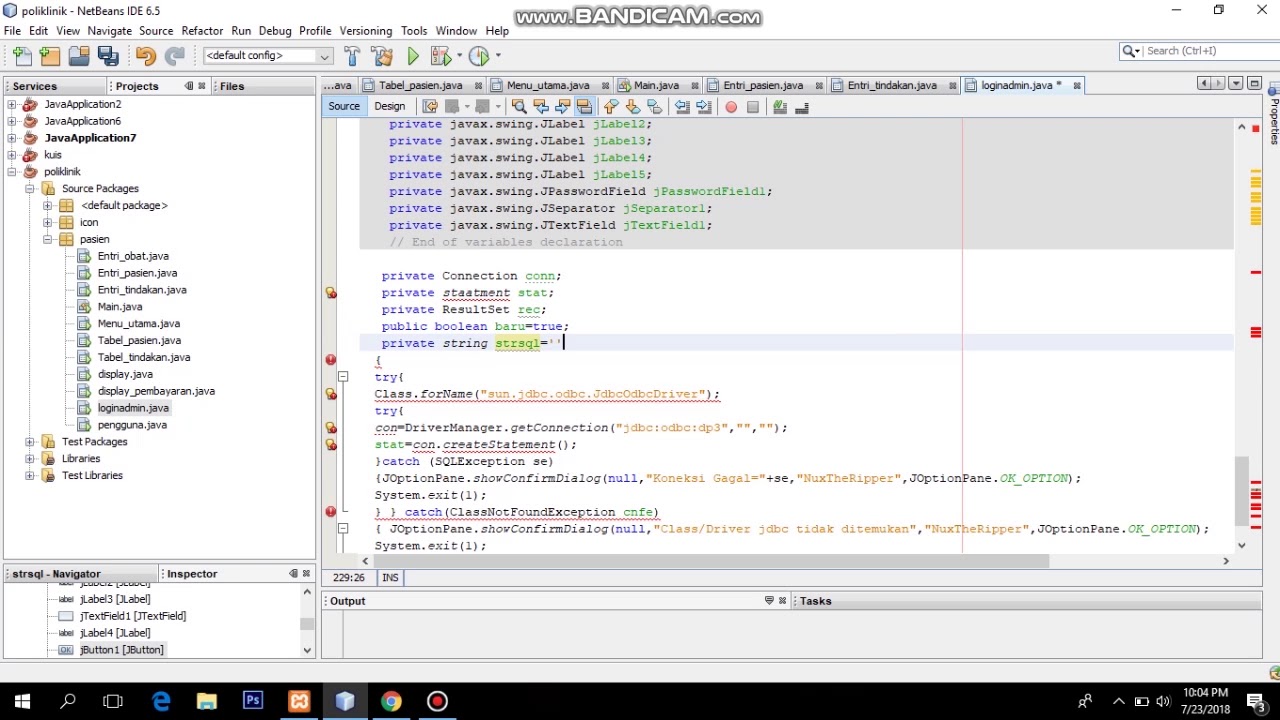
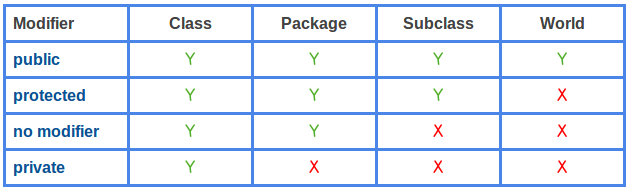

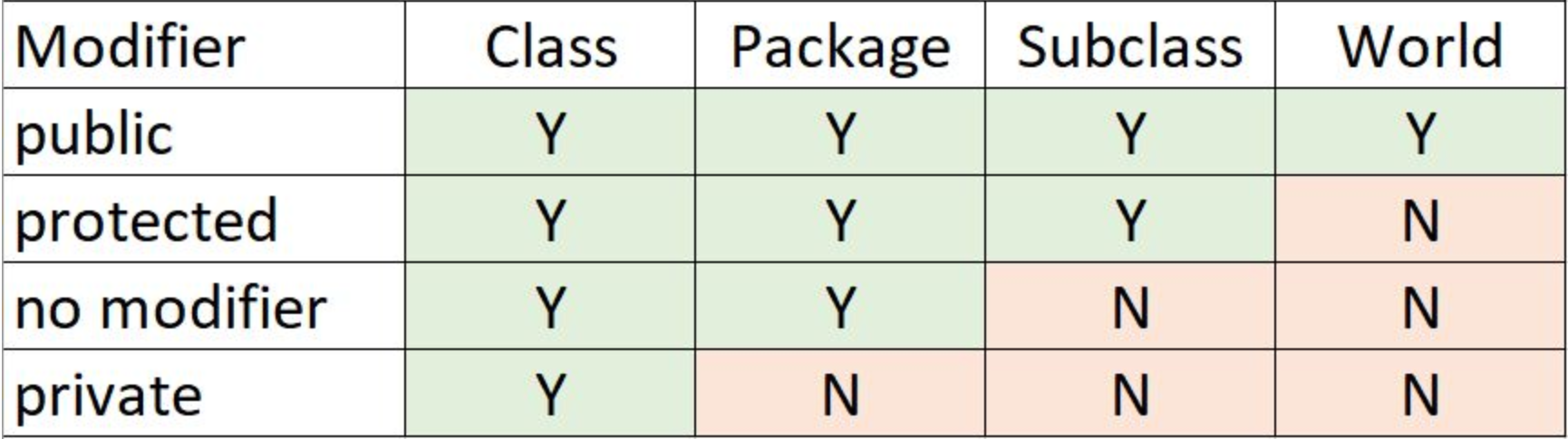

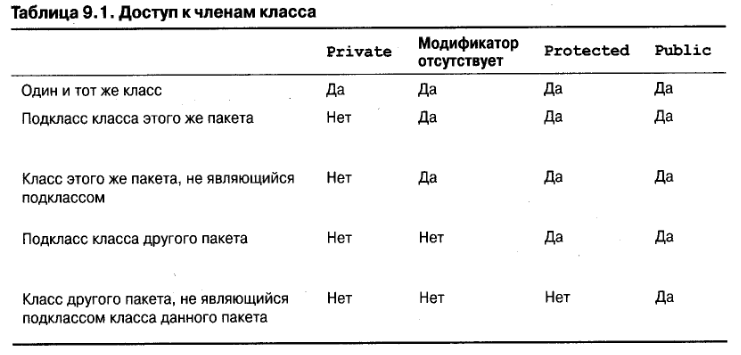

