How Spring Boot, Kubernetes, And Docker Helps To Microservices (Quick Guide)
Sandy Williams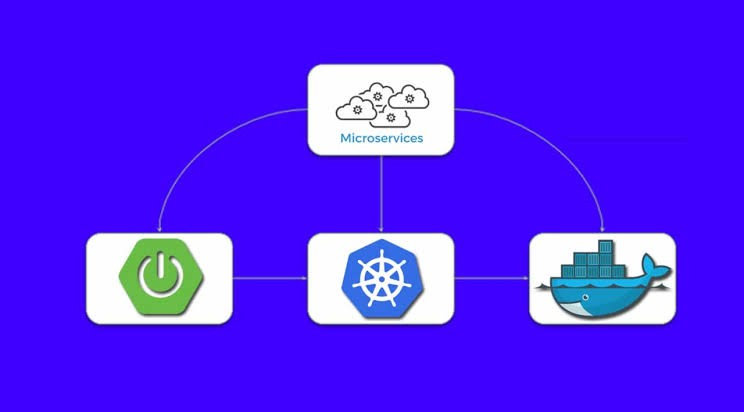
Here’s the next article in a series of “Quick Guide to…” This time, we will discuss and run examples of Spring Boot microservices on Kubernetes. The structure of the article will be quite similar to Quick Guide to Microservices with Spring Boot 2.0 and Spring Cloud, as they are describing the same aspects of application development. I’m going to focus on showing you the differences and similarities in development between Spring Cloud and Kubernetes. The topics covered in this article are:
- Using Spring Boot 2.0 in cloud-native development
- Providing service discovery for all microservices using a Spring Cloud Kubernetes project
- Injecting configuration settings into application pods using Kubernetes Config Maps and Secrets
- Building application images using Docker and deploying them on Kubernetes using YAML configuration files
- Using Spring Cloud Kubernetes together with a Zuul proxy to expose a single Swagger API documentation for all microservices More additional info At Spring Boot Online Training
Spring Cloud and Kubernetes may be threatened as competitive solutions when you build a microservices environment. Such components like Eureka, Spring Cloud Config, or Zuul provided by Spring Cloud may be replaced by built-in Kubernetes objects like services, config maps, secrets, or ingresses. But even if you decide to use Kubernetes components instead of Spring Cloud, you can take advantage of some interesting features provided throughout the whole Spring Cloud project.
The one really interesting project that helps us in development is Spring Cloud Kubernetes. Although it is still in the incubation stage, it is definitely worth dedicating some time to it. It integrates Spring Cloud with Kubernetes. I’ll show you how to use an implementation of the discovery client, inter-service communication with the Ribbon client, and Zipkin discovery using Spring Cloud Kubernetes.
Before we proceed to the source code, let’s take a look at the following diagram. It illustrates the architecture of our sample system. It is quite similar to the architecture presented in the mentioned article about microservices on Spring Cloud. There are three independent applications (employee-service
, department-service
, organization-service
), which communicate with each other through a REST API. These Spring Boot microservices use some built-in mechanisms provided by Kubernetes: config maps and secrets for distributed configuration, etcd for service discovery, and ingresses for the API gateway.
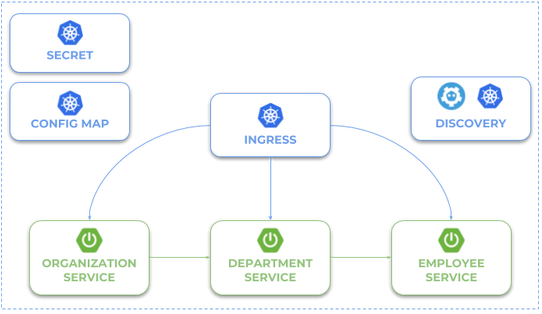
Let’s proceed to the implementation. Currently, the newest stable version of Spring Cloud is Finchley.RELEASE
. This version of spring-cloud-dependencies
should be declared as a BOM for dependency management.
<dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Finchley.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
Spring Cloud Kubernetes is not released under Spring Cloud Release Trains, so we need to explicitly define its version. Because we use Spring Boot 2.0 we have to include the newest SNAPSHOT
version of spring-cloud-kubernetes
artifacts, which is 0.3.0.BUILD-SNAPSHOT
.
Pre-Requirements
In order to be able to deploy and test our sample microservices, we need to prepare a development environment. We can realize that in the following steps:
- You need at least a single node cluster instance of Kubernetes (Minikube) or Openshift (Minishift) running on your local machine. You should start it and expose the embedded Docker client provided by both of them. The detailed instructions for Minishift may be found in my Quick guide to deploying Java apps on OpenShift. You can also use that description to run Minikube — just replace word "minishift" with "minikube." In fact, it does not matter if you choose Kubernetes or Openshift — the next part of this tutorial will be applicable for both of them.
- Spring Cloud Kubernetes requires access to the Kubernetes API in order to be able to retrieve a list of addresses for pods running for a single service. If you use Kubernetes, you should just execute the following command:
$ kubectl create clusterrolebinding admin --clusterrole=cluster-admin --serviceaccount=default:default
If you deploy your microservices on Minishift, you should first enable admin-user add-on, then log in as a cluster admin and grant the required permissions.
$ minishift addons enable admin-user $ oc login -u system:admin $ oc policy add-role-to-user cluster-reader system:serviceaccount:myproject:default apiVersion: apps/v1
kind: Deployment metadata: name: mongodb labels: app: mongodb spec: replicas: 1 selector: matchLabels: app: mongodb template: metadata: labels: app: mongodb
1. Inject the Configuration With Config Maps and Secrets
When using Spring Cloud, the most obvious choice for realizing a distributed configuration in your system is Spring Cloud Config. With Kubernetes, you can use Config Map. It holds key-value pairs of configuration data that can be consumed in pods or used to store configuration data. It is used for storing and sharing non-sensitive, unencrypted configuration information. To use sensitive information in your clusters, you must use Secrets. Use of both these Kubernetes objects can be perfectly demonstrated based on the example of MongoDB connection settings. Inside a Spring Boot application, we can easily inject it using environment variables. Here's a fragment of application.yml
file with URI configuration. To get in-depth knowledge on Java Online Training
spring: data: mongodb: uri: mongodb://${MONGO_USERNAME}:${MONGO_PASSWORD}@mongodb/${MONGO_DATABASE}
While username and password are sensitive fields, a database name is not, so we can place it inside the config map.
apiVersion: v1 kind: ConfigMap metadata: name: mongodb data: database-name: microservices
Of course, username and password are defined as secrets.
apiVersion: v1 kind: Secret metadata: name: mongodb type: Opaque data: database-password: MTIzNDU2 database-user: cGlvdHI=
To apply the configuration to the Kubernetes cluster, we run the following commands.
$ kubectl apply -f kubernetes/mongodb-configmap.yaml $ kubectl apply -f kubernetes/mongodb-secret.yaml
After that, we should inject the configuration properties into the application's pods. When defining the container configuration inside the Deployment YAML file, we have to include references to environment variables and secrets, as shown below.
apiVersion: apps/v1 kind: Deployment metadata: name: employee labels: app: employee spec: replicas: 1 selector: matchLabels: app: employee template: metadata: labels: app: employee
2. Building Service Discovery With Kubernetes
We are usually running microservices on Kubernetes using Docker containers. One or more containers are grouped by pods, which are the smallest deployable units created and managed in Kubernetes. A good practice is to run only one container inside a single pod. If you would like to scale up your microservice, you would just have to increase the number of running pods. All running pods that belong to a single microservice are logically grouped with Kubernetes Service. This service may be visible outside the cluster and is able to load balance incoming requests between all running pods. The following service definition groups all pods labeled with the field app
equal to employee
.
apiVersion: v1 kind: Service metadata name: employee labels: app: employee spec: ports: - port: 8080 protocol: TCP selector: app: employee
Service can be used to access the application outsidethe Kubernetes cluster or for inter-service communication inside a cluster. However, the communication between microservices can be implemented more comfortably with Spring Cloud Kubernetes. First, we need to include the following dependency in the project pom.xml
.
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-kubernetes</artifactId> <version>0.3.0.BUILD-SNAPSHOT</version> </dependency>
Then we should enable the discovery client for an application, the same as we have always done for discovery in Spring Cloud Netflix Eureka. This allows you to query Kubernetes endpoints (services) by name. This discovery feature is also used by Spring Cloud Kubernetes Training Ribbon or Zipkin projects to fetch, respectively, the list of the pods defined for a microservice to be load balanced or the Zipkin servers available to send the traces or spans.
@SpringBootApplication @EnableDiscoveryClient @EnableMongoRepositories @EnableSwagger2 public class EmployeeApplication { public static void main(String[] args) { SpringApplication.run(EmployeeApplication.class, args); } // ... }
The last important thing in this section is to guarantee that the Spring application name will be exactly the same as the Kubernetes service name for the application. For the application employee-service
, it is employee
.
spring: application: name: employee
3. Building Microservices Using Docker and Deploying on Kubernetes
There is nothing unusual in our sample microservices. We have included some standard Spring dependencies for building REST-based microservices, integrating with MongoDB, and generating API documentation using Swagger2.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
In order to integrate with MongoDB, we should create an interface that extends standard Spring Data CrudRepository
.
public interface EmployeeRepository extends CrudRepository { List findByDepartmentId(Long departmentId); List findByOrganizationId(Long organizationId); }
The entity class should be annotated with Mongo @Document
and a primary key field with @Id
.
@Document(collection = "employee") public class Employee { @Id private String id; private Long organizationId; private Long departmentId; private String name; private int age; private String position; // ... }
The repository bean has been injected to the controller class. Here's the full implementation of our REST API inside employee-service.
@RestController public class EmployeeController { private static final Logger LOGGER = LoggerFactory.getLogger(EmployeeController.class); @Autowired EmployeeRepository repository; @PostMapping("/") public Employee add(@RequestBody Employee employee) { LOGGER.info("Employee add: {}", employee); return repository.save(employee); }
In order to run our microservices on Kubernetes, we should first build the whole Maven project with the mvn clean install
command. Each microservice has a Dockerfile placed in the root directory. Here's the Dockerfile definition for employee-service
.
FROM openjdk:8-jre-alpine ENV APP_FILE employee-service-1.0-SNAPSHOT.jar ENV APP_HOME /usr/apps EXPOSE 8080 COPY target/$APP_FILE $APP_HOME/ WORKDIR $APP_HOM ENTRYPOINT ["sh", "-c"] CMD ["exec java -jar $APP_FILE"]
Let's build Docker images for all three sample microservices.
$ cd employee-service $ docker build -t piomin/employee:1.0 . $ cd department-service $ docker build -t piomin/department:1.0 . $ cd organization-service $ docker build -t piomin/organization:1.0 .
The last step is to deploy Docker containers with applications on Kubernetes. To do that, just execute the commands kubectl apply
on YAML configuration files. The sample deployment file for employee-service
has been demonstrated in step 1. All required deployment fields are available inside the project repository in the kubernetes
directory.
$ kubectl apply -f kubernetes\employee-deployment.yaml $ kubectl apply -f kubernetes\department-deployment.yaml $ kubectl apply -f kubernetes\organization-deployment.yaml
4. Communication Between Microservices With Spring Cloud Kubernetes Ribbon
All the microservices are deployed on Kubernetes. Now, it's worth it to discuss some aspects related to inter-service communication. The application employee-service
, in contrast to other microservices, did not invoke any other microservices. Let's take a look at other microservices that call the API exposed by employee-service
and communicate between each other ( organization-service
calls department-service
API).
First, we need to include some additional dependencies in the project. We use Spring Cloud Ribbon and OpenFeign. Alternatively, you can also use Spring@LoadBalancedRestTemplate
.
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-kubernetes-ribbon</artifactId> <version>0.3.0.BUILD-SNAPSHOT</version> </dependency>
Here's the main class of department-service
. It enables Feign client using the @EnableFeignClients
annotation. It works the same as with discovery based on Spring Cloud Netflix Eureka. OpenFeign uses Ribbon for client-side load balancing. Spring Cloud Kubernetes Ribbon provides some beans that force Ribbon to communicate with the Kubernetes API through Fabric8 KubernetesClient
.
@SpringBootApplication @EnableDiscoveryClient @EnableFeignClients @EnableMongoRepositories @EnableSwagger2 public class DepartmentApplication { public static void main(String[] args) { SpringApplication.run(DepartmentApplication.class, args); } // ... }
Here's the implementation of Feign client for calling the method exposed by employee-service
.
@FeignClient(name = "employee") public interface EmployeeClient { @GetMapping("/department/{departmentId}") List findByDepartment(@PathVariable("departmentId") String departmentId); }
Finally, we have to inject Feign client's beans into the REST controller. Now, we may call the method defined inside EmployeeClient
, which is equivalent to calling REST endpoints.
@RestController public class DepartmentController { private static final Logger LOGGER = LoggerFactory.getLogger(DepartmentController.class); @Autowired DepartmentRepository repository; @Autowired EmployeeClient employeeClient; // ...
5. Building API Gateway Using Kubernetes Ingress
Ingress is a collection of rules that allow incoming requests to reach the downstream services. In our microservices architecture, ingress is playing the role of an API gateway. To create it, we should first prepare a YAML description file. The descriptor file should contain the hostname under which the gateway will be available and mapping rules to the downstream services.
apiVersion: extensions/v1beta1 kind: Ingress metadata: name: gateway-ingress annotations: nginx.ingress.kubernetes.io/rewrite-target: / spec: backend: serviceName: default-http-backend servicePort: 80
You have to execute the following command to apply the configuration above to the Kubernetes cluster.
Take your career to new heights of success with an Spring Boot, enroll for live free demo on Spring Boot Certification
$ kubectl apply -f kubernetes\ingress.yaml
To test this solution locally, we have to insert the mapping between the IP address and hostname set in the ingress definition inside the hosts
file, as shown below. After that, we can test services through ingress using defined hostname just like that: http://microservices.info/employee
.
192.168.99.100 microservices.info
You can check the details of the created ingress just by executing the command kubectl describe ing gateway-ingress
.

6. Enabling API Specification on the Gateway Using Swagger2
What if we would like to expose a single Swagger documentation for all microservices deployed on Kubernetes? Well, here things are getting complicated... We can run a container with Swagger UI, and map all paths exposed by the ingress manually, but it is not a good solution...
In that case, we can use Spring Cloud Kubernetes Ribbon one more time, this time together with Spring Cloud Netflix Zuul. Zuul will act as a gateway only for serving the Swagger API.
Here's the list of dependencies used in my gateway-service
project.
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-zuul</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-kubernetes</artifactId> <version>0.3.0.BUILD-SNAPSHOT</version> </dependency>
Kubernetes discovery client will detect all services exposed on the cluster. We would like to display documentation only for our three microservices. That's why I defined the following routes for Zuul.
zuul: routes: department: path: /department/** employee: path: /employee/** organization: path: /organization/**
Now we can use the ZuulProperties
bean to get the routes' addresses from Kubernetes discovery and configure them as Swagger resources, as shown below.
@Configuration public class GatewayApi { @Autowired ZuulProperties properties; @Primary @Bean public SwaggerResourcesProvider swaggerResourcesProvider() { return () -> { List resources = new ArrayList(); properties.getRoutes().values().stream() .forEach(route -> resources.add(createResource(route.getId(), "2.0"))); return resources; }; }
The application gateway-service
should be deployed on the cluster the same as the other applications. You can see the list of running services by executing the command kubectl get svc
. Swagger documentation is available under the address http://192.168.99.100:31237/swagger-ui.html
.

Conclusion
I'm actually rooting for the Spring Cloud Kubernetes project, which is still at the incubation stage. Kubernetes's popularity as a platform has been rapidly growing over the last few months, but it still has some weaknesses. One of them is inter-service communication. Kubernetes doesn't give us many mechanisms out-of-the-box which allow us to configure more advanced rules. This is a reason for creating frameworks for service mesh on Kubernetes, like Istio or Linkerd. While these projects are still relatively new solutions, Spring Cloud is a stable, opinionated framework. Why not use it to provide service discovery, inter-service communication, or load balancing? Thanks to Spring Cloud Kubernetes, it is possible.