Golang Private Function
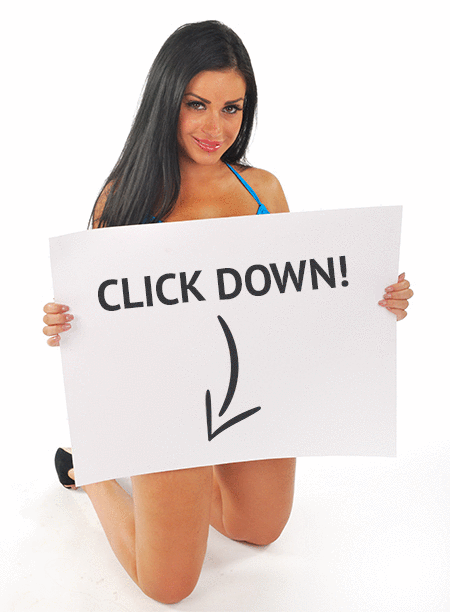
โก ๐๐ป๐๐ป๐๐ป INFORMATION AVAILABLE CLICK HERE ๐๐ป๐๐ป๐๐ป
Clippings
Favorites
Hire Me!
Feel free to
contact me
with questions or feedback regarding this article.
I knew that
naming , specifically as it relates to capitalization, was how we
control exports in Golang.
It was not until I was using the language for a couple weeks that
I realized the implications behind this.
Exports in Go, which are controlled by naming conventions, are
very similar to the concepts of public and
private we see in other languages.
Since a capital letter means something will be exported, and a
lowercase letter means it will not be exported, we can have
structs and other data, functions, and methods, that are private
in the scope of a package.
I think it is important to note that there is different semantic
meaning behind exports in Go vs public and
private in a language like Java. They may seem
similar, but are definitely different concepts. However, using
familiar terms like that helped me understand their power in Go.
It helped me think of the different patterns we can apply in
packages
See an example (using a couple files) below.
I have a cars package used in this
main.go file. By controlling the visibility inside
the cars package, we can encourage patterns like
having a constructor helper function for instantiating new
structs. This way, we can hide the ability to create a struct
literal and control the instantiation.
Here is the cars package used by main.
This begged an interesting question for me. If car is
not exported, how can it be used in main.go ? How does
main.go understand what a car can do?
I experimented by trying to re-define how we handle the result of
the NewCar call.
This seems odd. How can main.go instantiate a car if
it cannot use the unexported cars.car ?
I do not really have an answer to that. I can only assume that
because exported methods like HonkTheHorn exist, we
don't need to have access to cars.car in
order to call methods against it.
It is interesting to note the following behavior.
If we uncomment doThing1 we get a compile error
because, again, cars.car is not exported.
So by keeping the car struct unexported we can call
exported (public-esque) methods on it, but that's about it.
It is also interesting to note these cases.
Although car is not exported, it can have fields that
are exported.
My simple observation is that you can use whatever is exported,
and everything else is blackbox to importing packages.
Not a very technical conclusion, but I think that basically sums
it up, and the way that functionality is enforced in Go does seem
pretty sensible and logical to me.
// Assembly to get into package runtime without using exported symbols.
// +build amd64 amd64p32 arm arm64 386 ppc64 ppc64le
# include "textflag.h"
# ifdef GOARCH_arm
# define JMP B
# endif
# ifdef GOARCH_ppc64
# define JMP BR
# endif
# ifdef GOARCH_ppc64le
# define JMP BR
# endif
TEXT ยท signal_disable ( SB ), NOSPLIT , $ 0
JMP runtime ยท signal_disable ( SB )
TEXT ยท signal_enable ( SB ), NOSPLIT , $ 0
JMP runtime ยท signal_enable ( SB )
TEXT ยท signal_ignore ( SB ), NOSPLIT , $ 0
JMP runtime ยท signal_ignore ( SB )
TEXT ยท signal_recv ( SB ), NOSPLIT , $ 0
JMP runtime ยท signal_recv ( SB )
// +build darwin dragonfly freebsd linux nacl netbsd openbsd solaris windows
package signal
import (
"os"
"syscall"
)
// In assembly.
func signal_disable ( uint32 )
func signal_enable ( uint32 )
func signal_ignore ( uint32 )
func signal_recv () uint32
// The runtime package uses //go:linkname to push a few functions into this
// package but we still need a .s file so the Go tool does not pass -complete
// to the go tool compile so the latter does not complain about Go functions
// with no bodies.
...
//go:linkname sync_runtime_doSpin sync.runtime_doSpin
//go:nosplit
func sync_runtime_doSpin () {
procyield ( active_spin_cnt )
}
package sync
import "unsafe"
...
// runtime_doSpin does active spinning.
func runtime_doSpin ()
package net
import (
...
_ "unsafe" // For go:linkname
)
...
// byteIndex is strings.IndexByte. It returns the index of the
// first instance of c in s, or -1 if c is not present in s.
// strings.IndexByte is implemented in runtime/asm_$GOARCH.s
//go:linkname byteIndex strings.IndexByte
func byteIndex ( s string , c byte ) int
package main
import (
_ "unsafe"
"fmt"
"runtime/pprof"
"os"
"time"
)
// Event types in the trace, args are given in square brackets.
const (
traceEvGoBlock = 20 // goroutine blocks [timestamp, stack]
)
type mutex struct {
// Futex-based impl treats it as uint32 key,
// while sema-based impl as M* waitm.
// Used to be a union, but unions break precise GC.
key uintptr
}
//go:linkname lock runtime.lock
func lock ( l * mutex )
//go:linkname unlock runtime.unlock
func unlock ( l * mutex )
//go:linkname goparkunlock runtime.goparkunlock
func goparkunlock ( lock * mutex , reason string , traceEv byte , traceskip int )
func main () {
l := & mutex {}
go func () {
lock ( l )
goparkunlock ( l , "xxx" , traceEvGoBlock , 1 )
}()
for {
pprof . Lookup ( "goroutine" ) . WriteTo ( os . Stdout , 1 )
time . Sleep ( time . Second * 1 )
}
}
Names are as important in Go as in any other language.
They even have semantic effect: the visibility of a name outside a
package is determined by whether its first character is upper case.
Sometimes its necessary to overcome this limitation in
order to organize your code better, or access some hidden
functions in foreign packages.
These techniques are heavily used in golang source code,
and this is the primary source of where it comes from. Its
distinguishable lack of information there are on the internet on this topic.
From the good old days, there are 2 ways of achieving this
bypassing compiler check: cannot refer to unexported name pkg.symbol :
the old one, currently not used - assembly level implicit
linkage to needed symbols, referred as assembly stubs , i.e.
go runtime, os/signal: use //go:linkname instead of assembly stubs to
get access to runtime functions ;
Using these techniques I have managed to bind to internal golang
runtime schedule related functions to over use goroutines threads
parking and internal locking mechanisms.
Idea is simple - provide stubs in assembly with explicit JMPs to
needed symbols. Linker does not know anything about which symbols
are exported and which not.
i.e. old version of src/os/signal/sig.s :
In order to use this, the source file have to import _ โunsafeโ package.
To overcome -complete go compiler limitations one of possible solutions
is to put empty assembly stub file near by the main source to disable this
check.
The format of this instruction is //go:linkname localname linkname . Using
this its possible to introduce new symbols for linkage (export), or bind to
existing symbols (import).
A function implementation in runtime/proc.go
says explicitly to the compiler to add another name to the code which will be
runtime_doSpin in sync package. And the sync reuses it in sync/runtime.go
with simple:
A good example sits in net/parse.go :
https://willhaley.com/blog/private-and-public-visibility-with-go-packages/
http://sitano.github.io/2016/04/28/golang-private/
Slut Fat Erotika
Porno Hd Lady Mom Dress
Nudist Freedom Com Category Photos
Private and Public Visibility with Go Packages - Will Haley
How to call private functions (bind to hidden symbols) in ...
How to access private functions of another package in Golang?
Public vs. private ยท YourBasic Go
Anonymous Functions in GoLang - GoLang Docs
go - Public, Private - Upper Case, Lower Case: - Stack ...
Functions in GoLang - GoLang Docs
Golangไธญ็public๏ผprivate_ไบๆทก้ฃ่ฝป-CSDNๅๅฎข
Access private fields with reflection ยท YourBasic Go
An example of unit testing a private function by golang ...
Golang Private Function

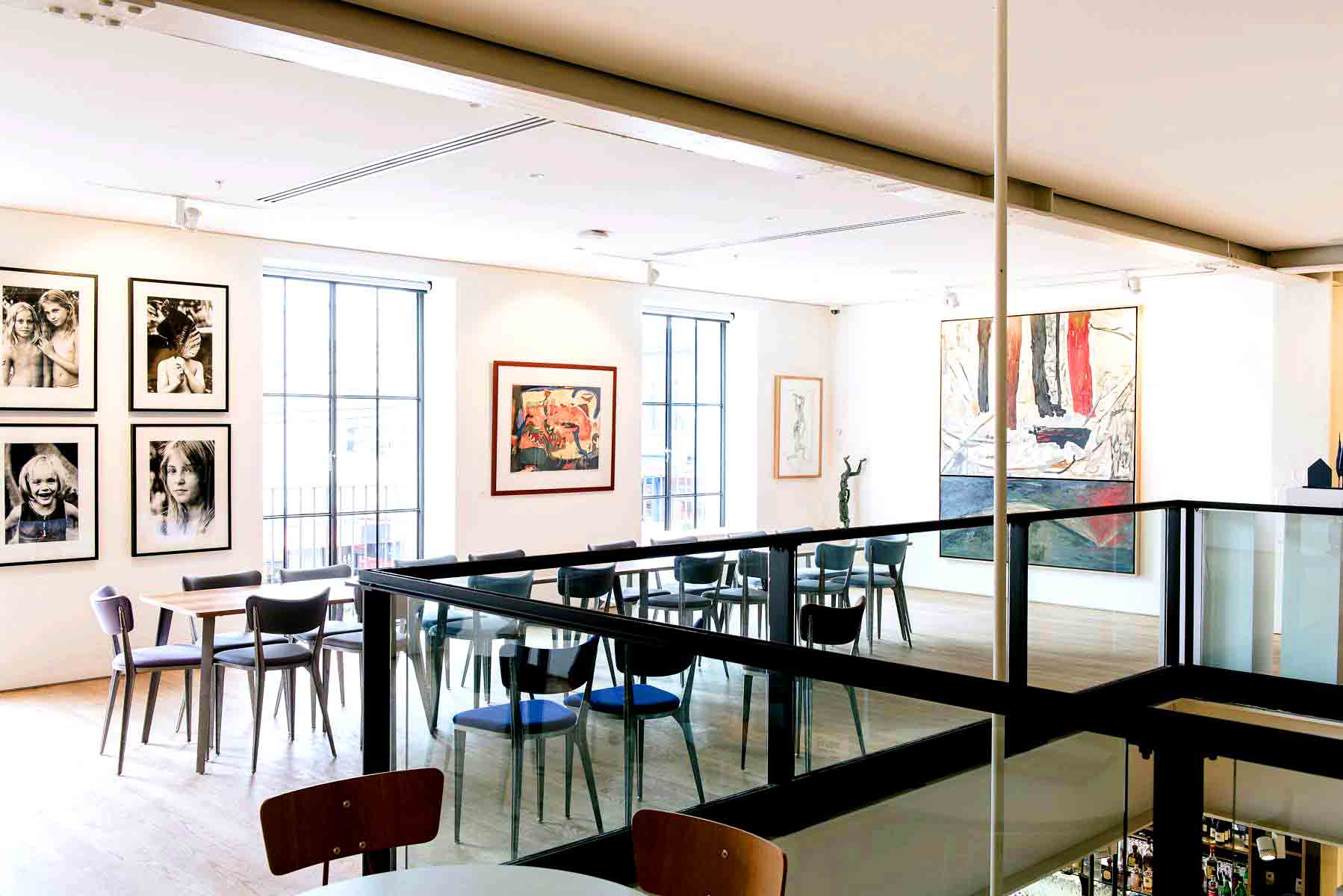
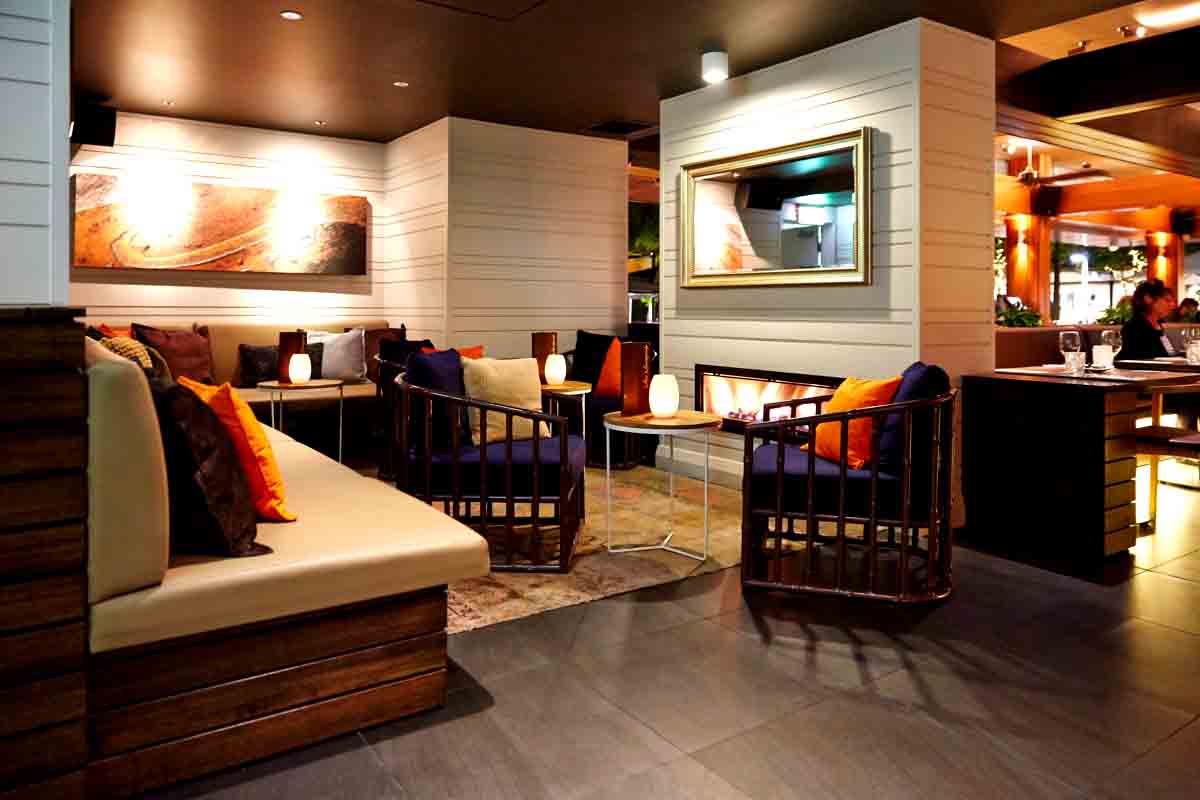



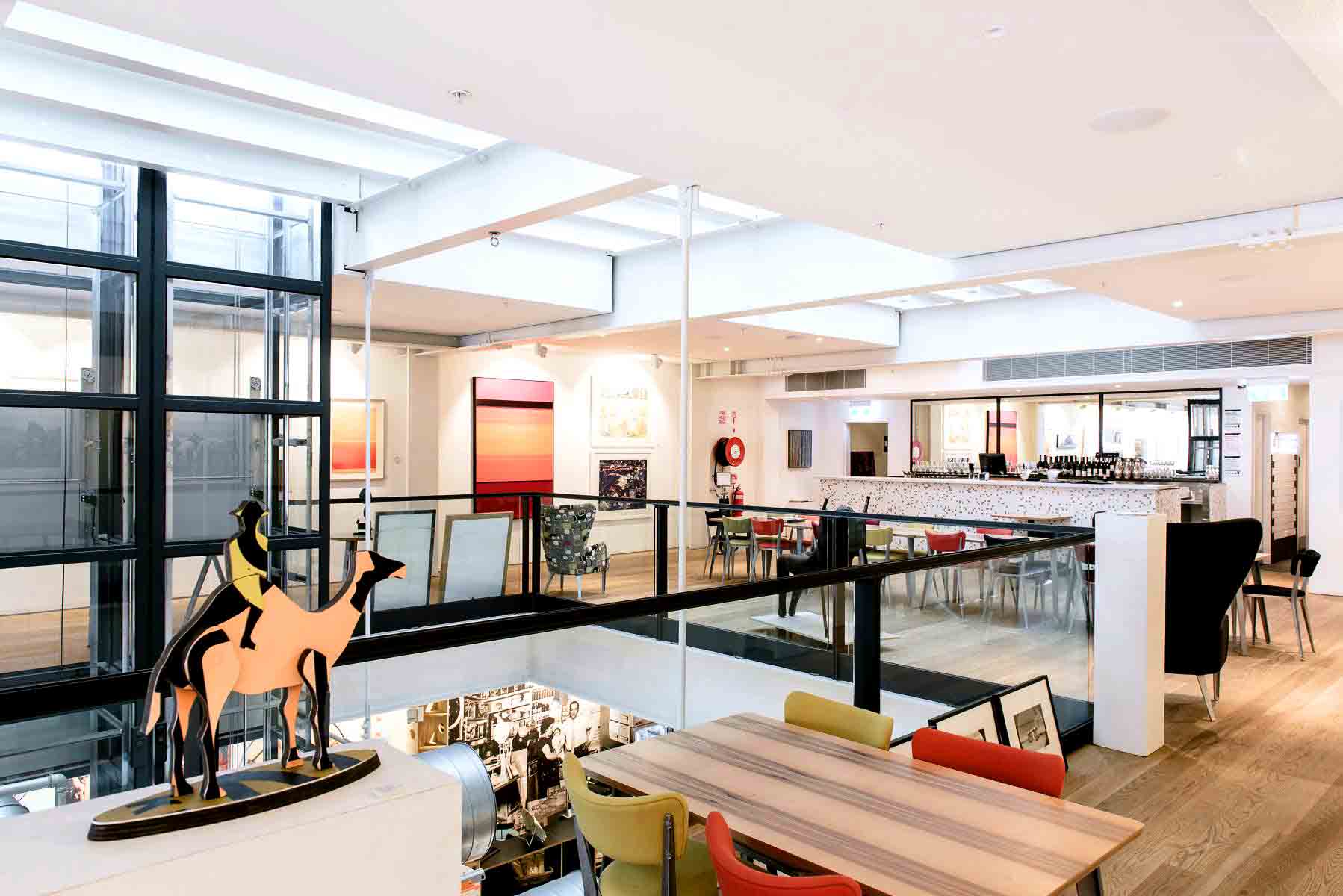
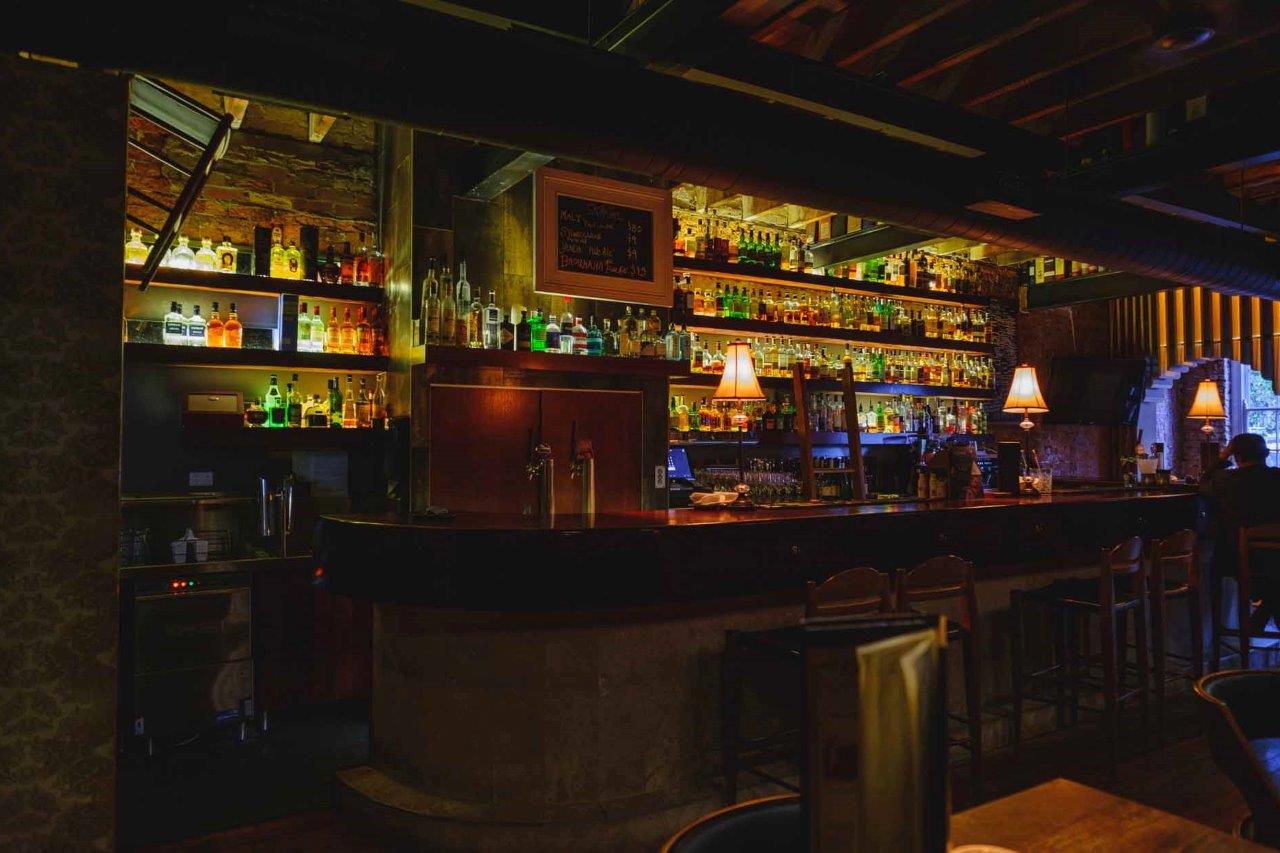
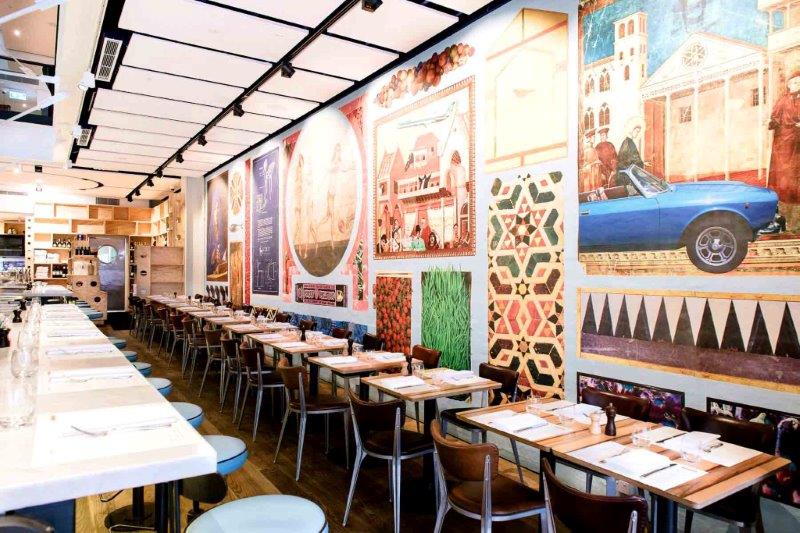
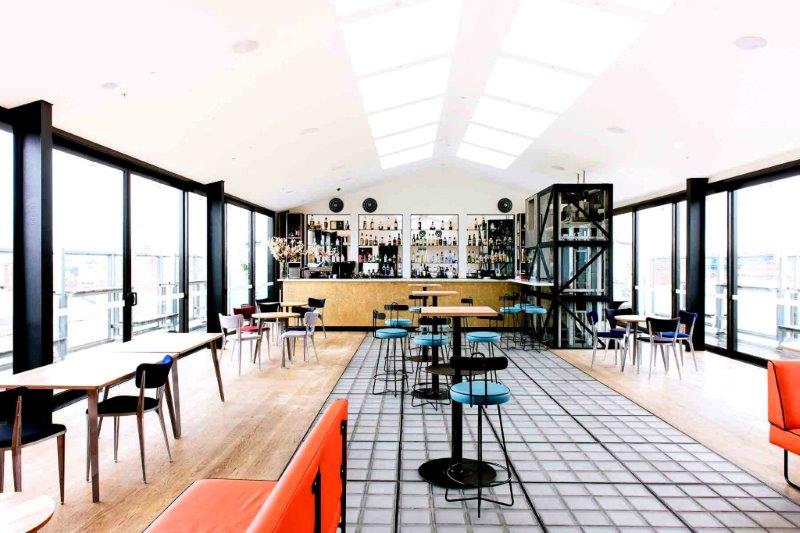
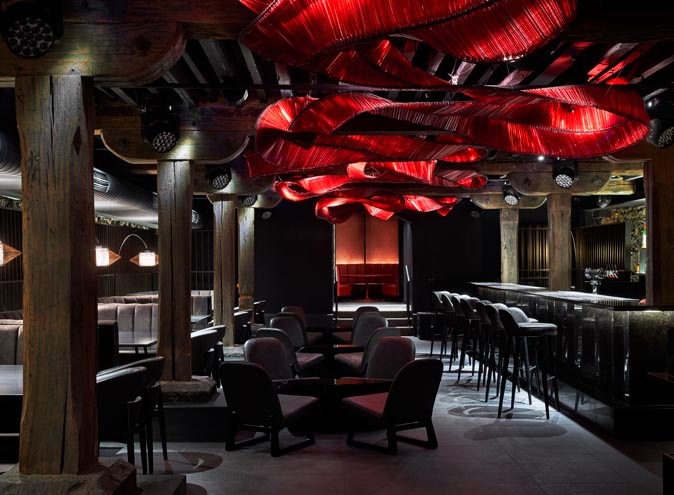
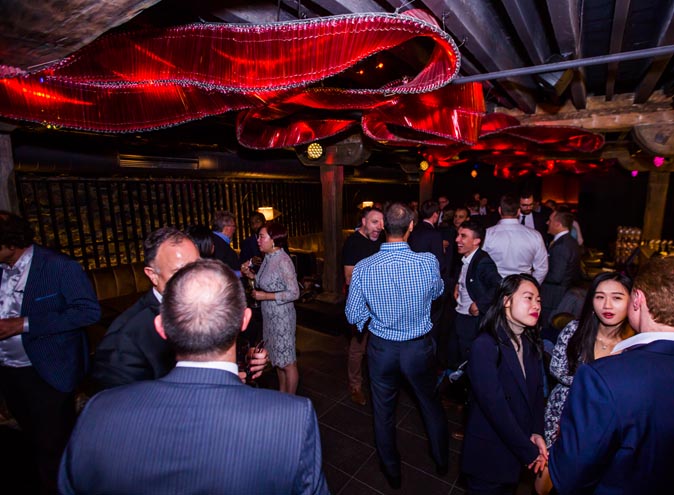
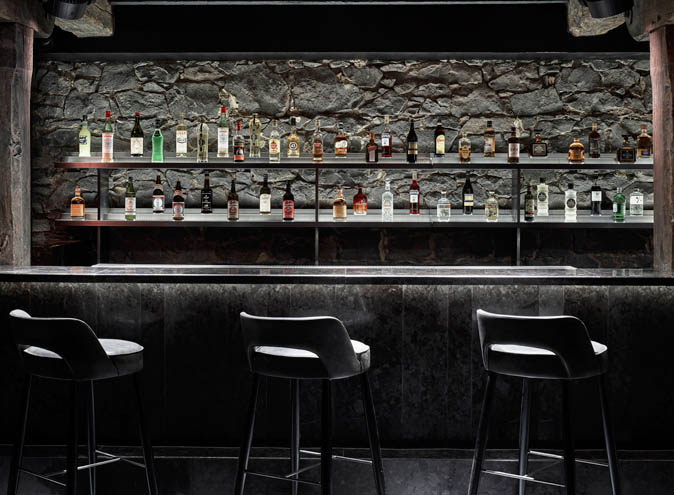
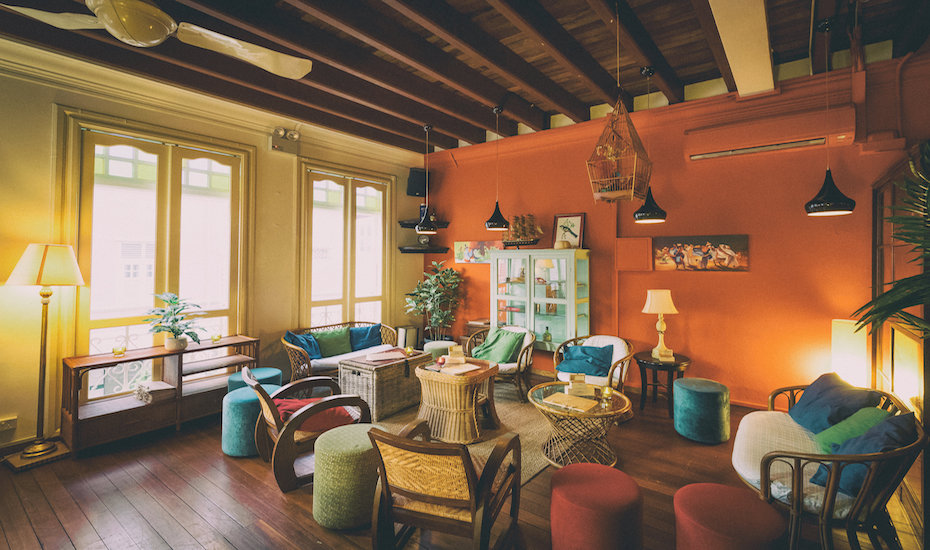

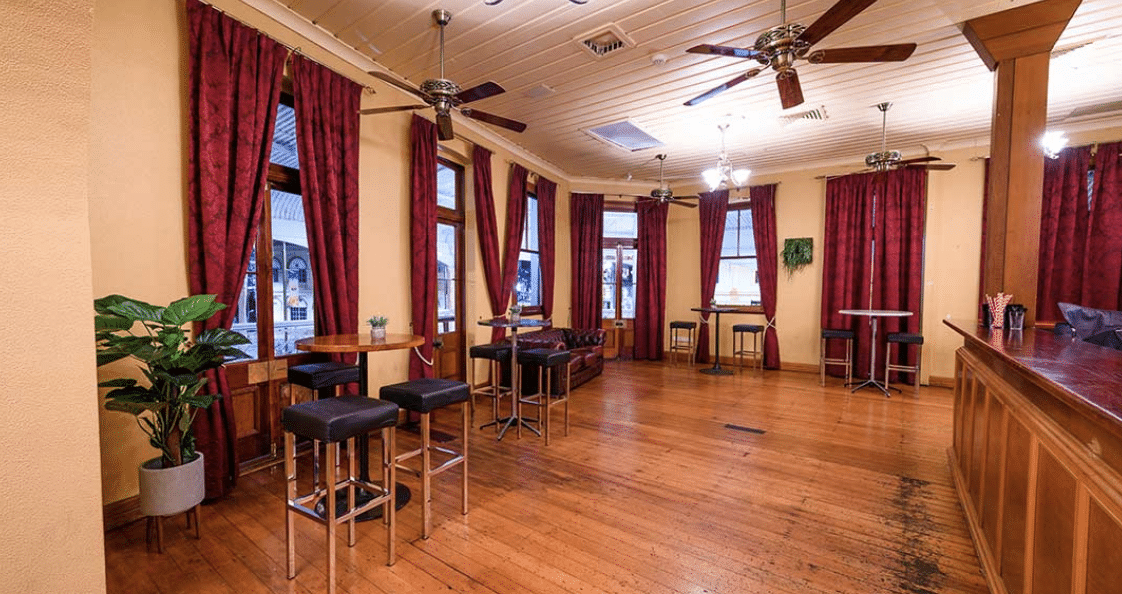
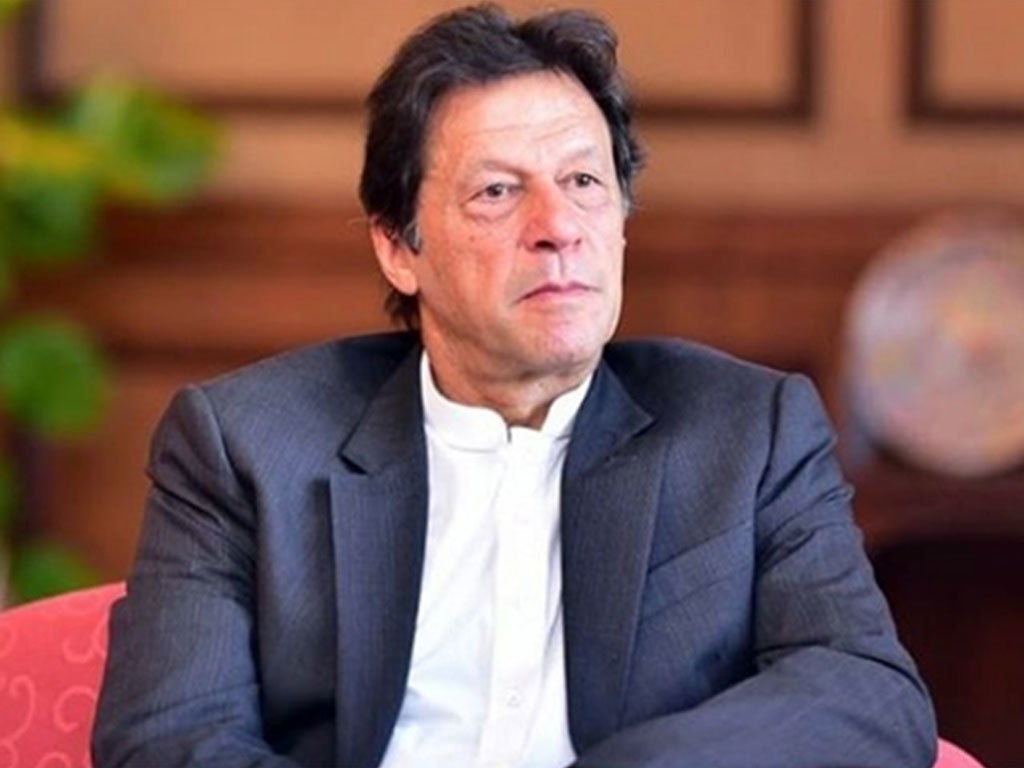
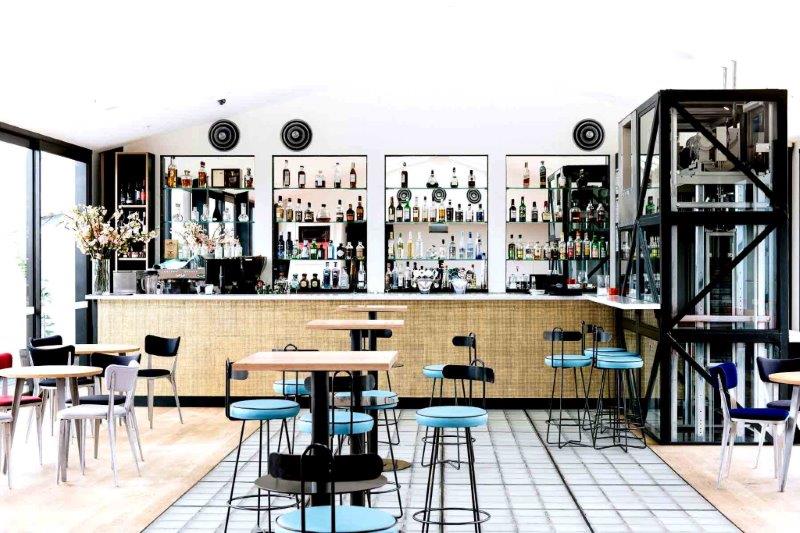
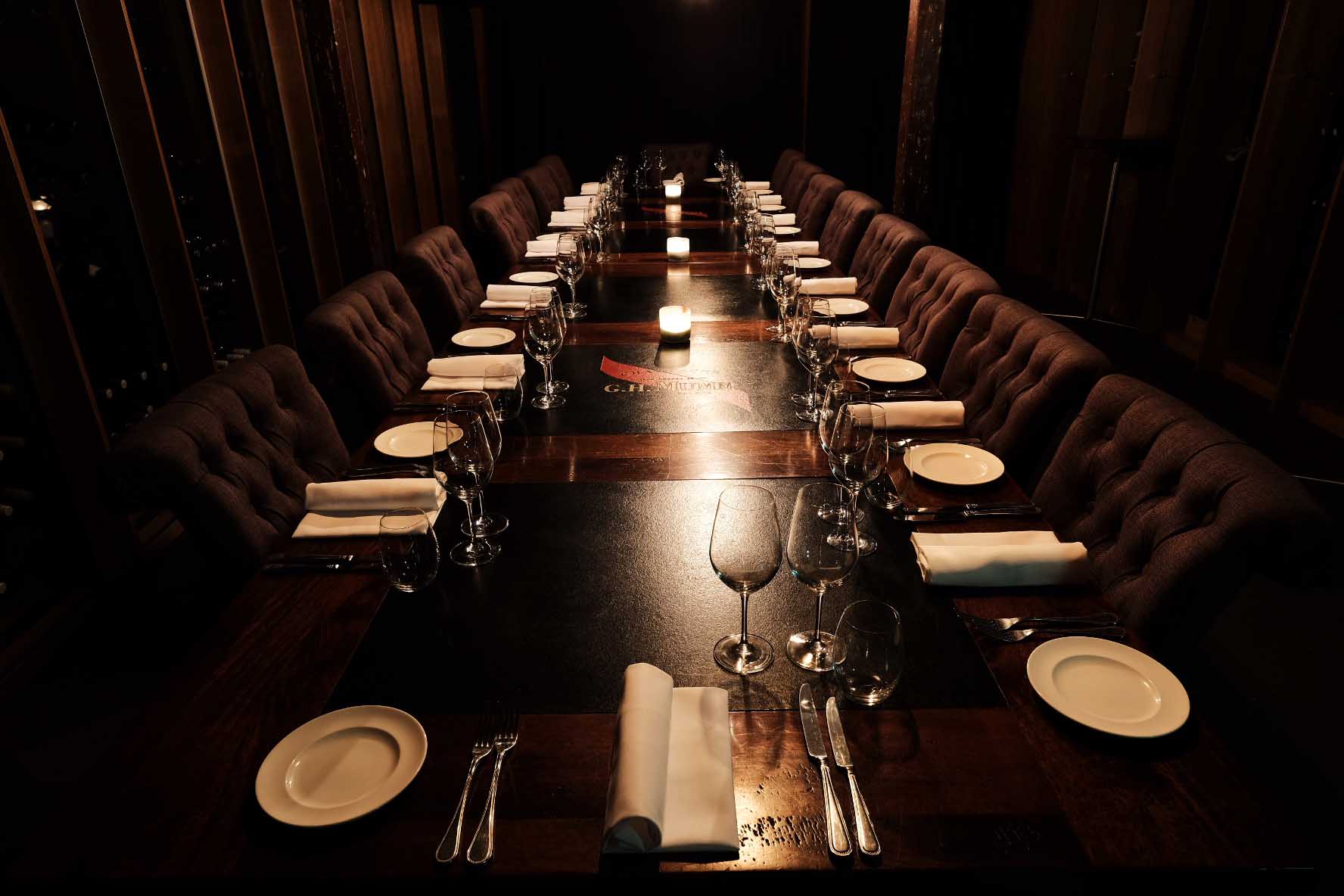
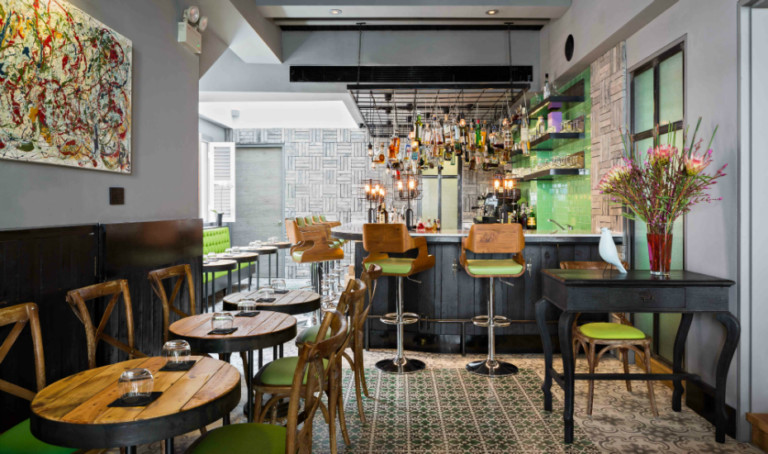
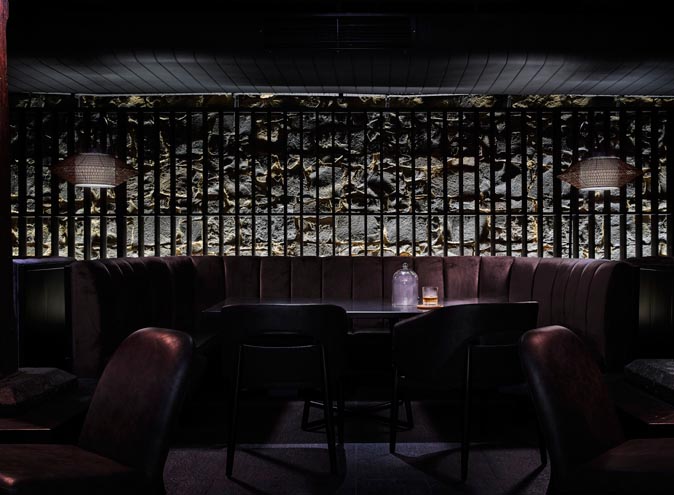
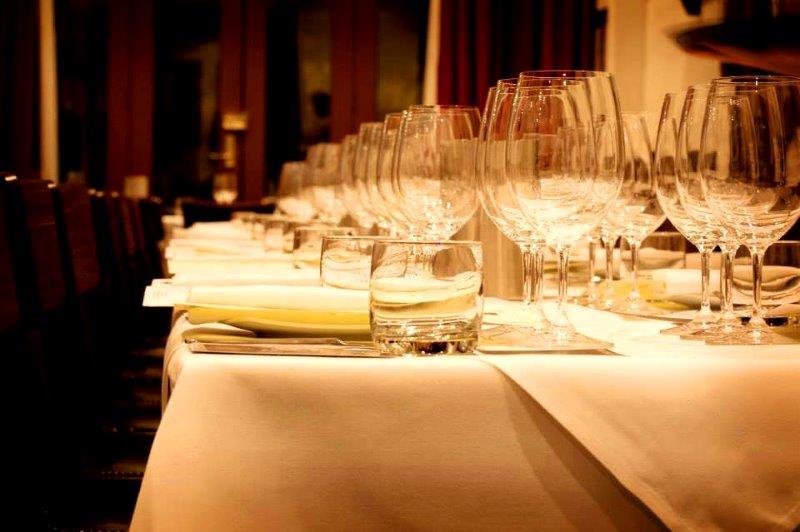

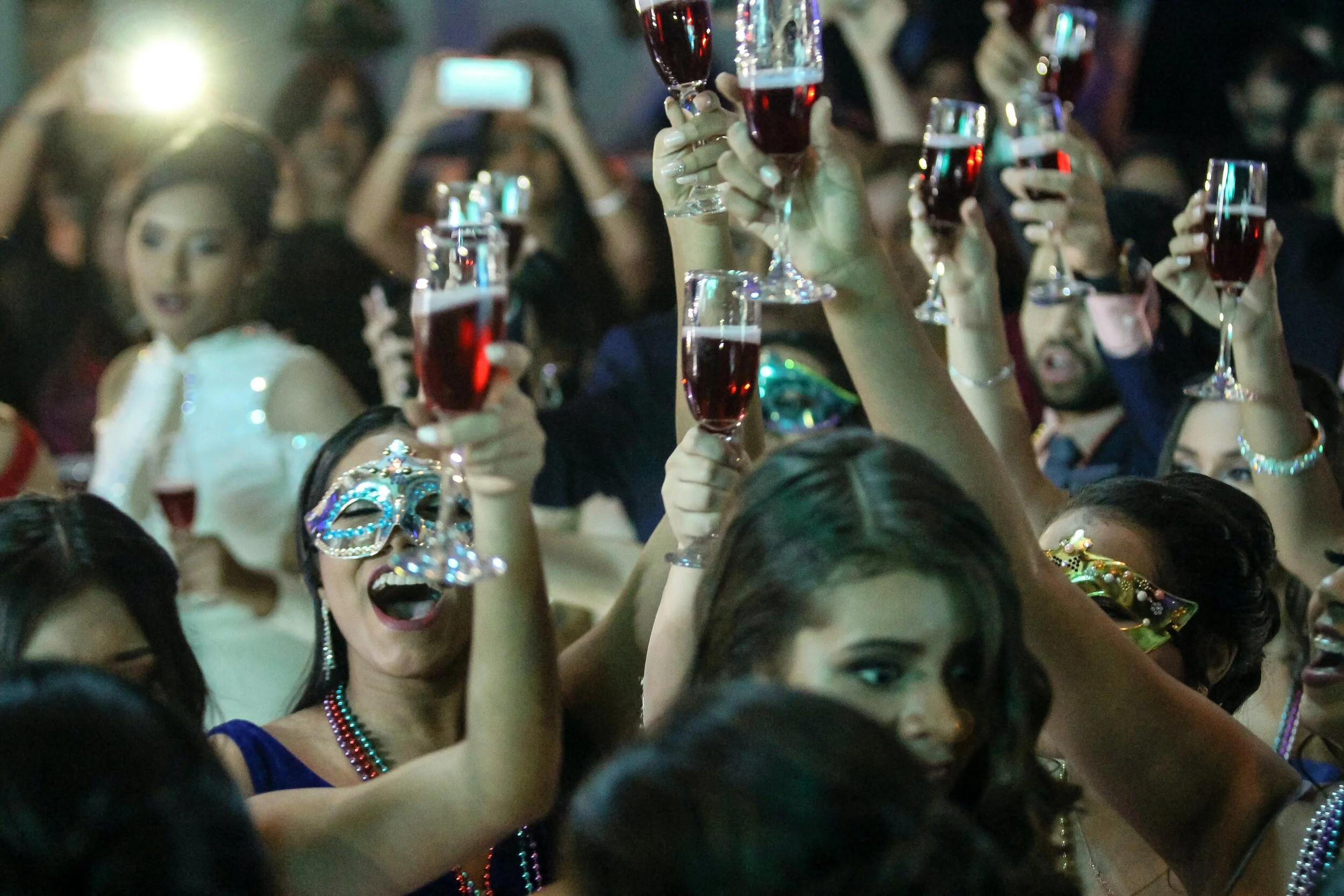



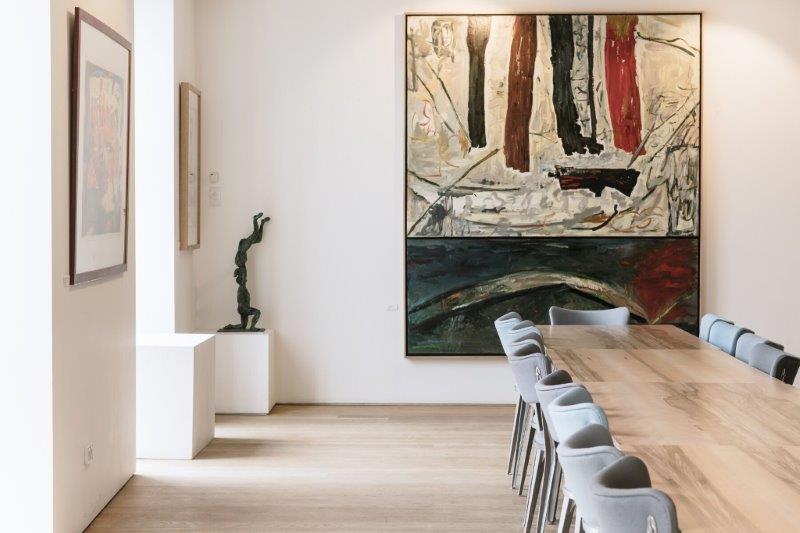



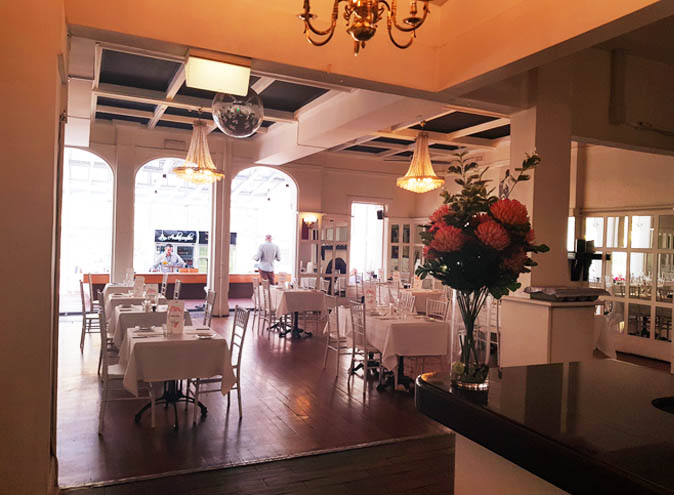
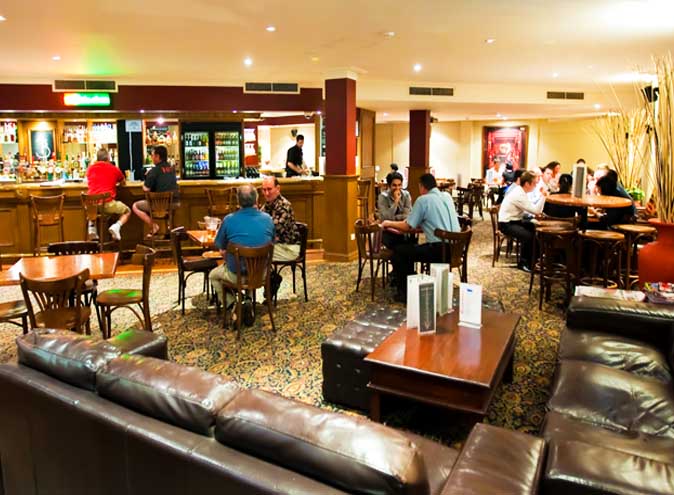
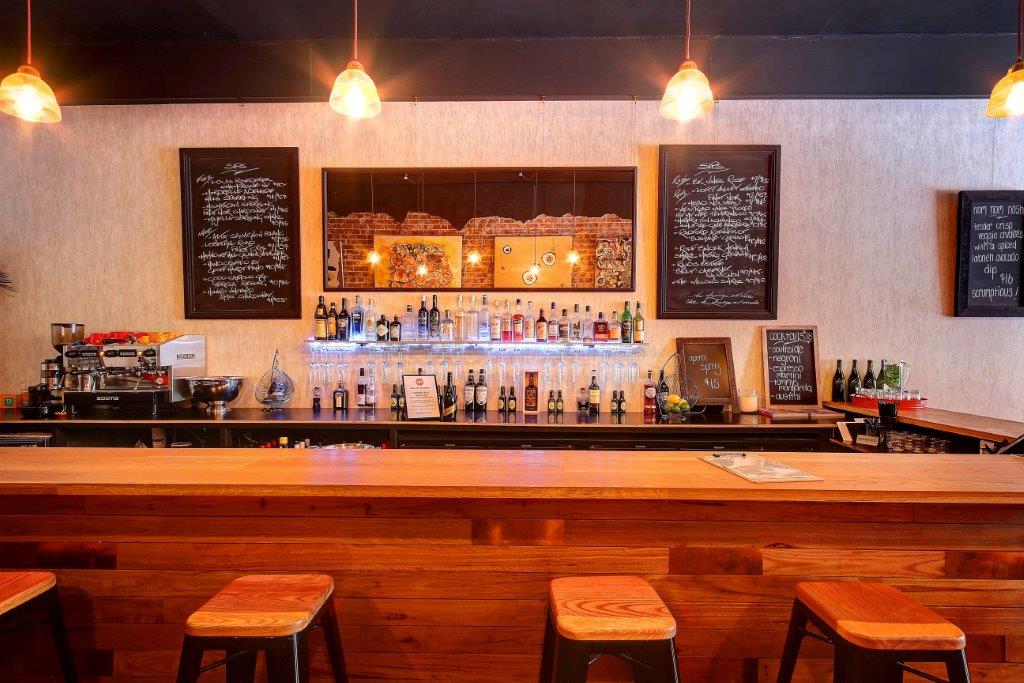

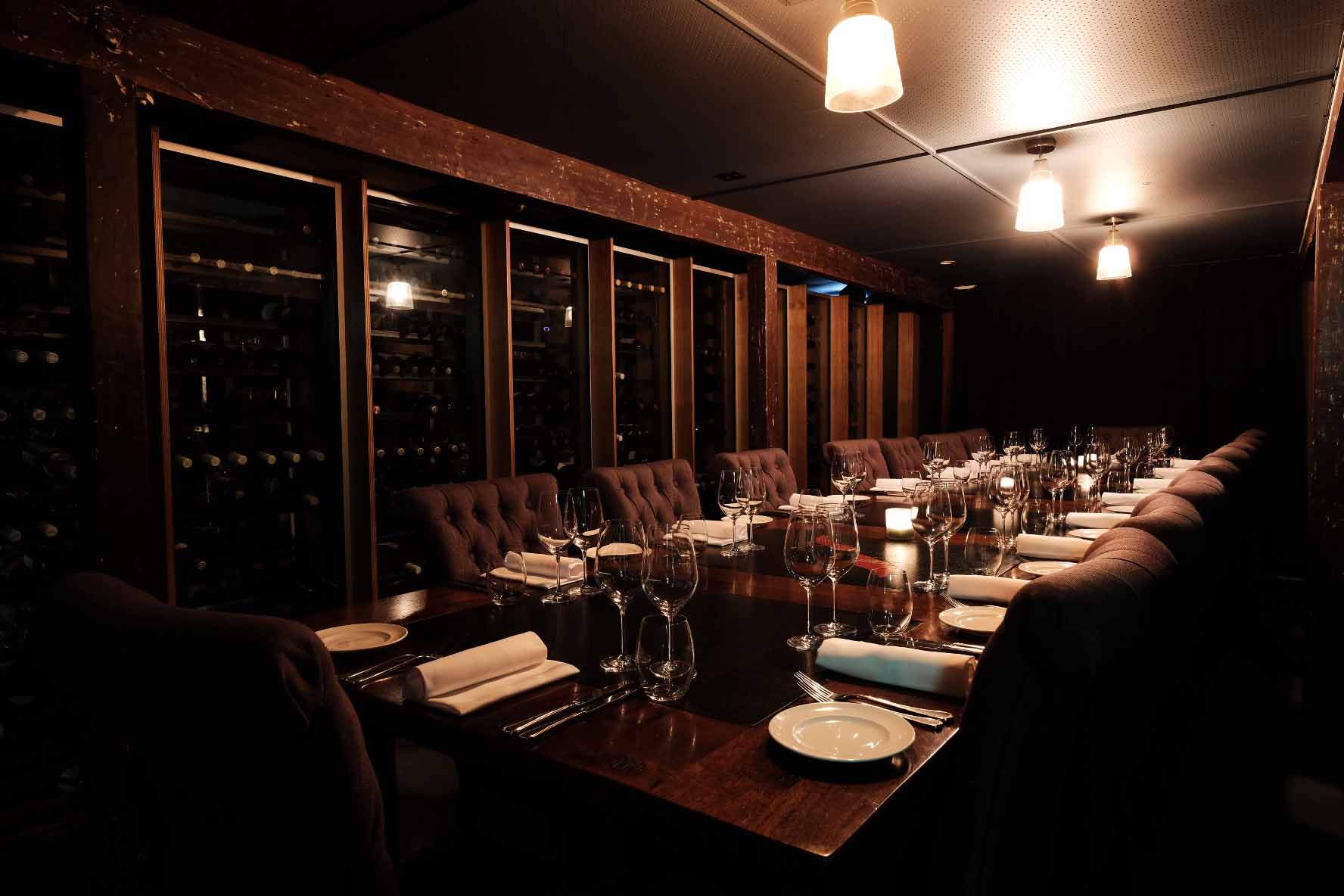
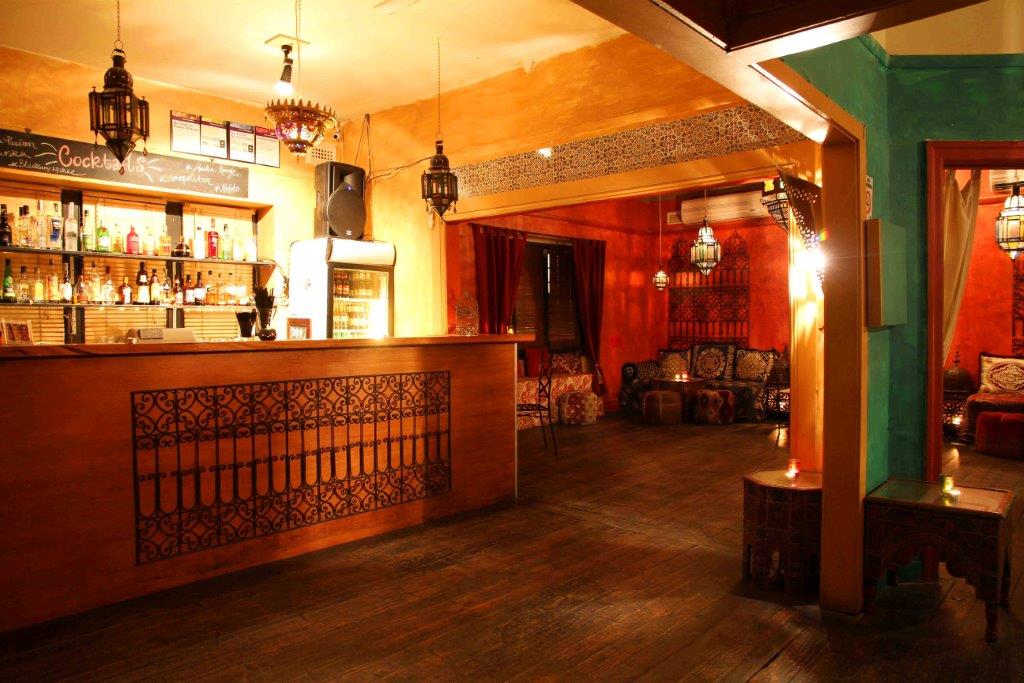
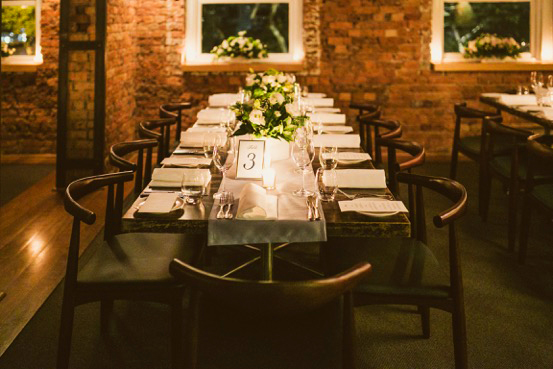
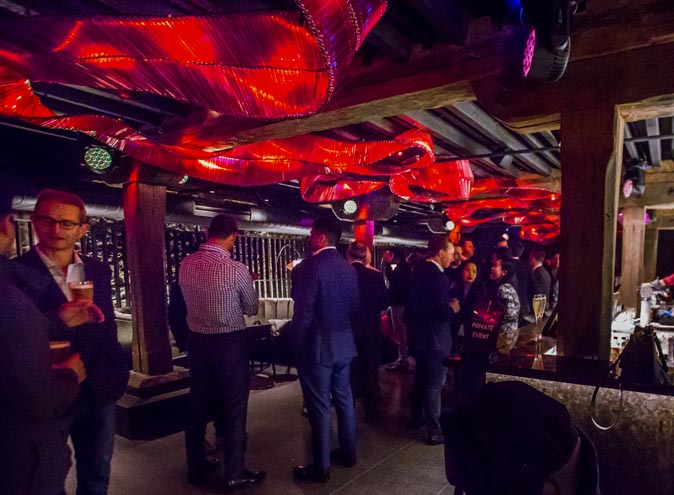

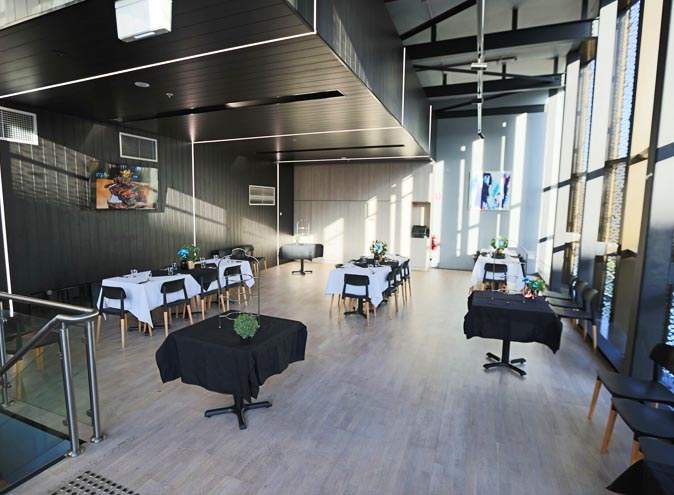
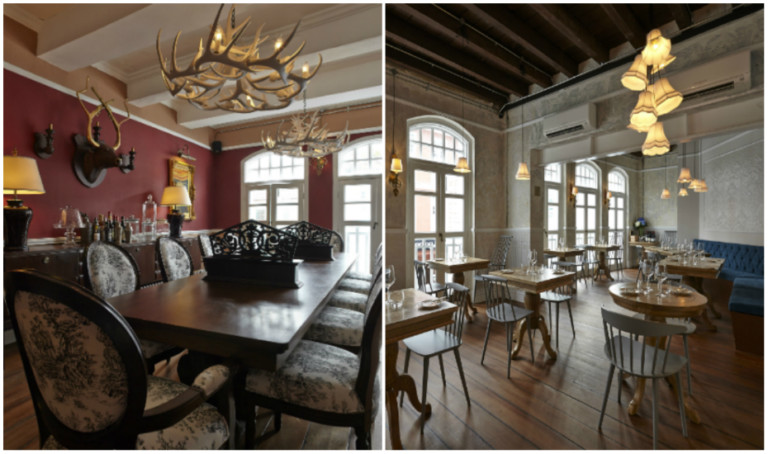
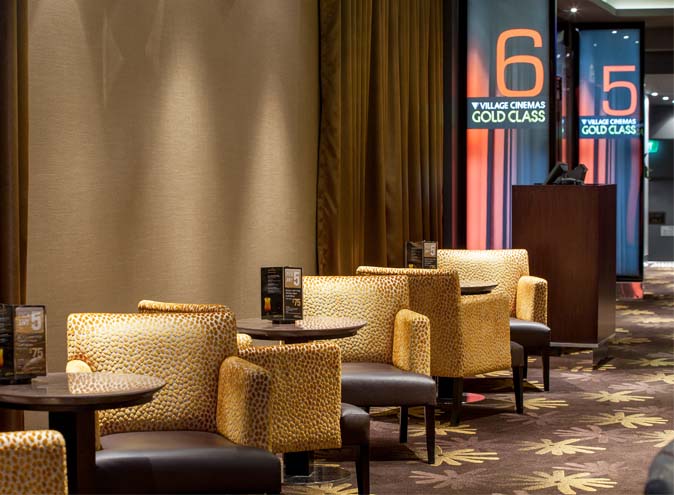
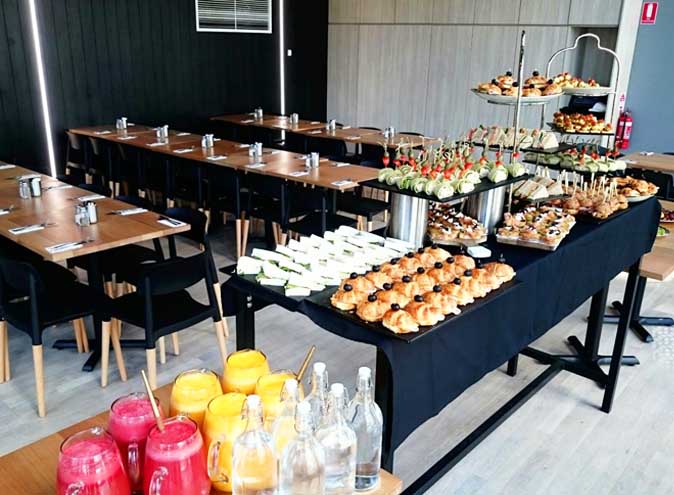

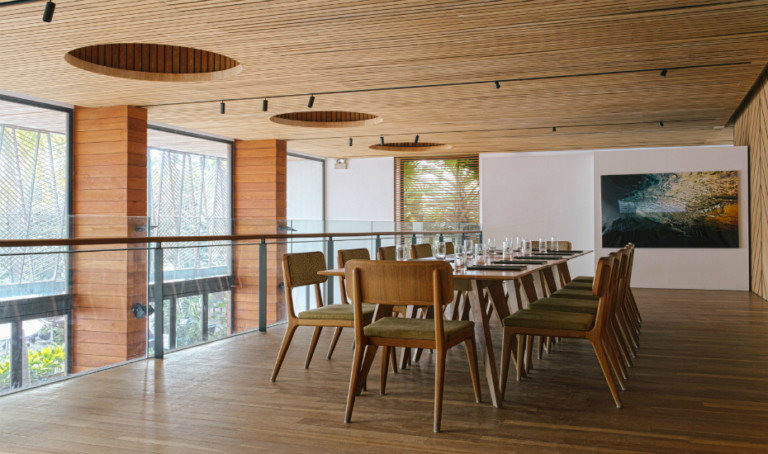

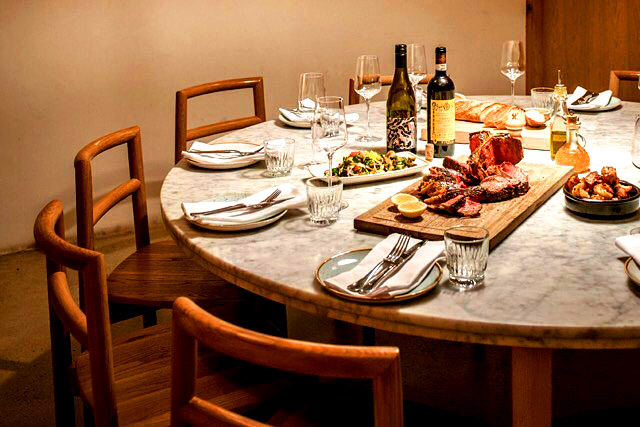

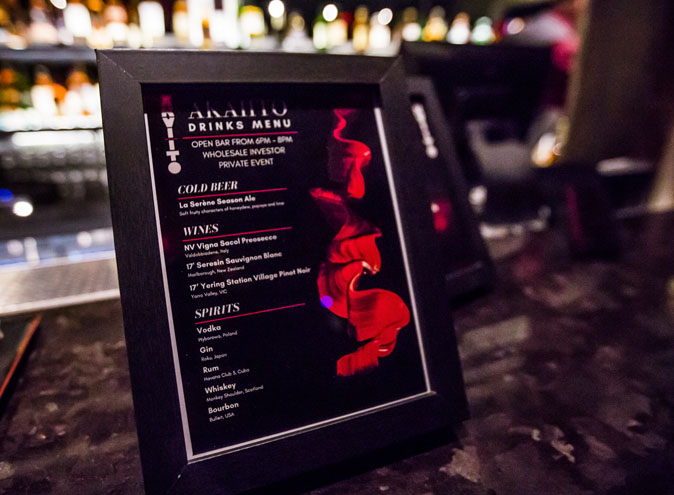

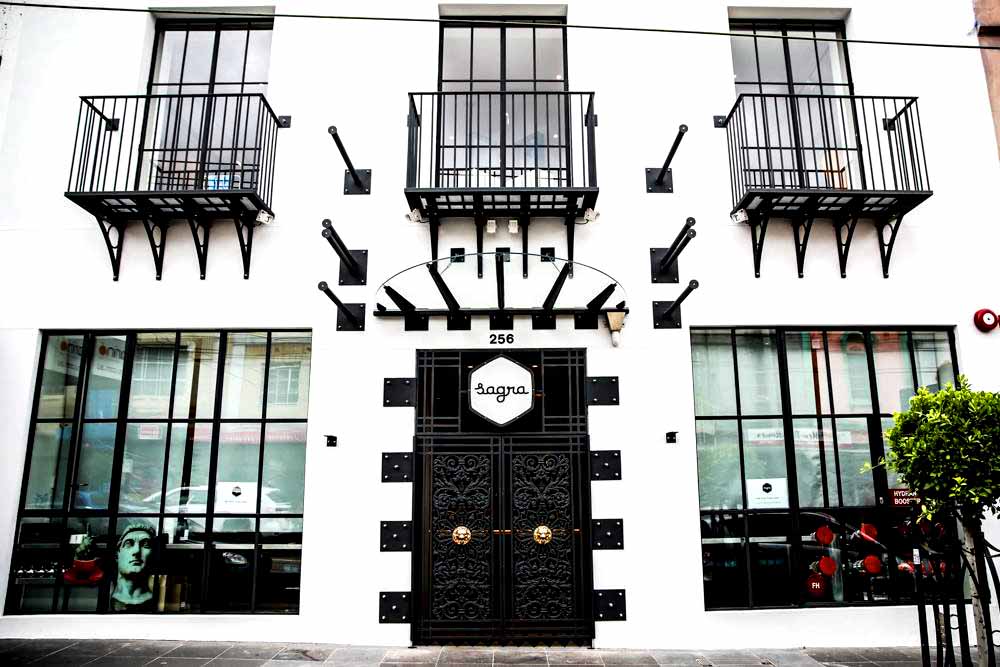


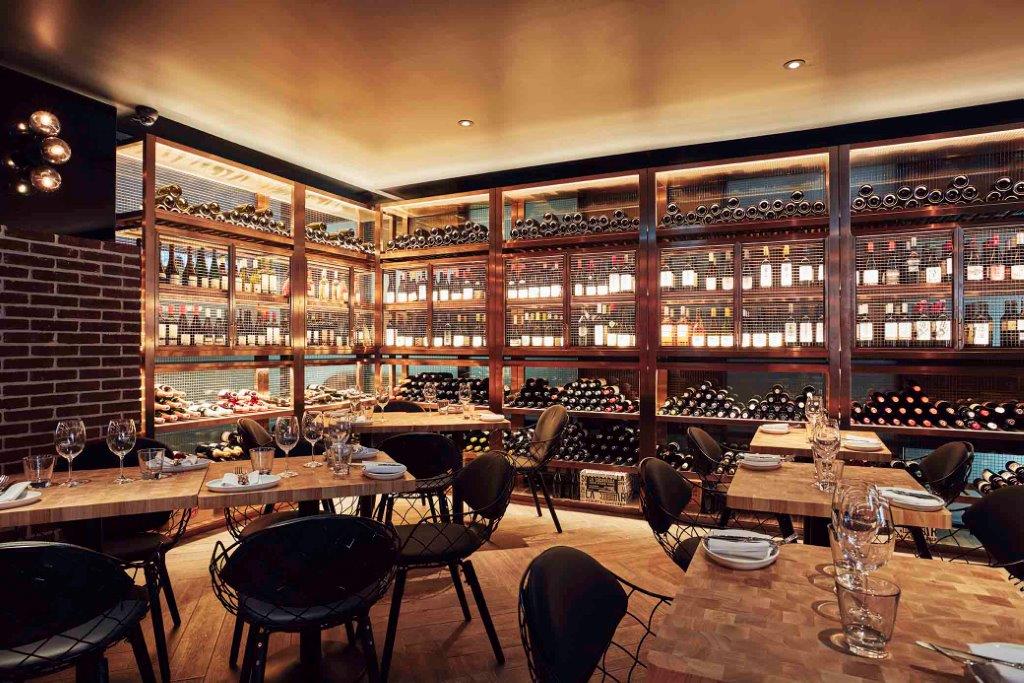
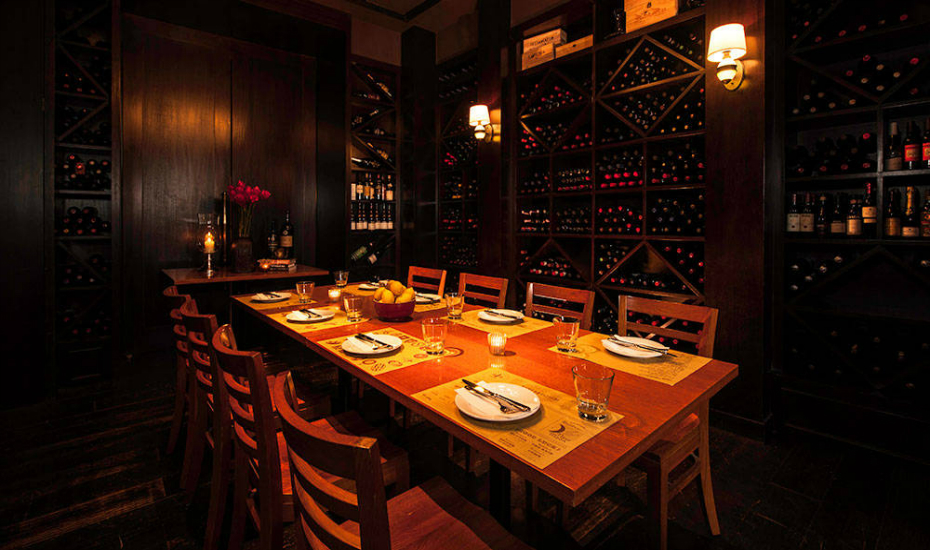

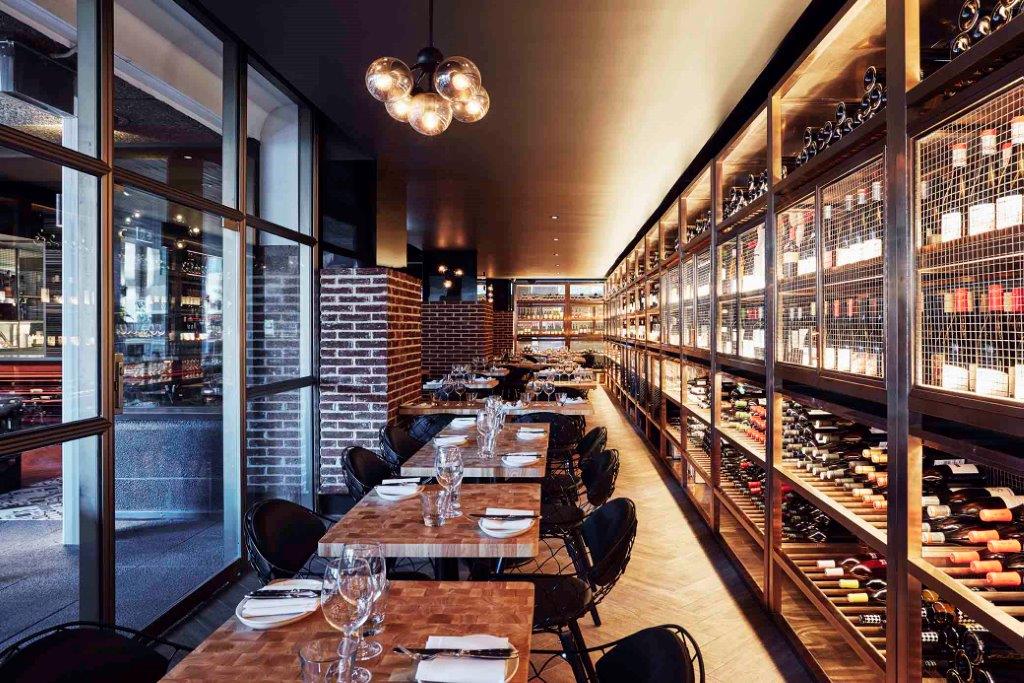
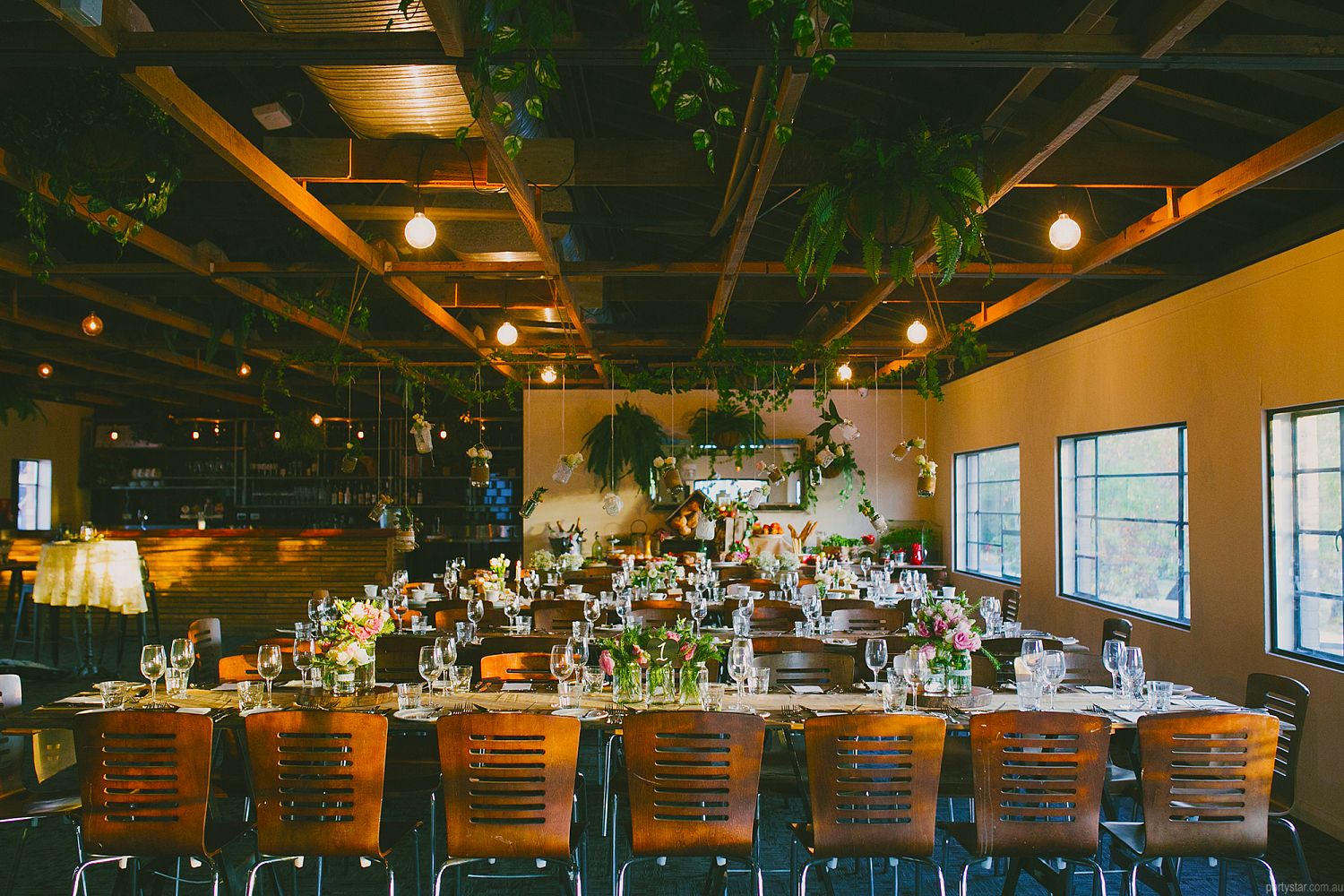
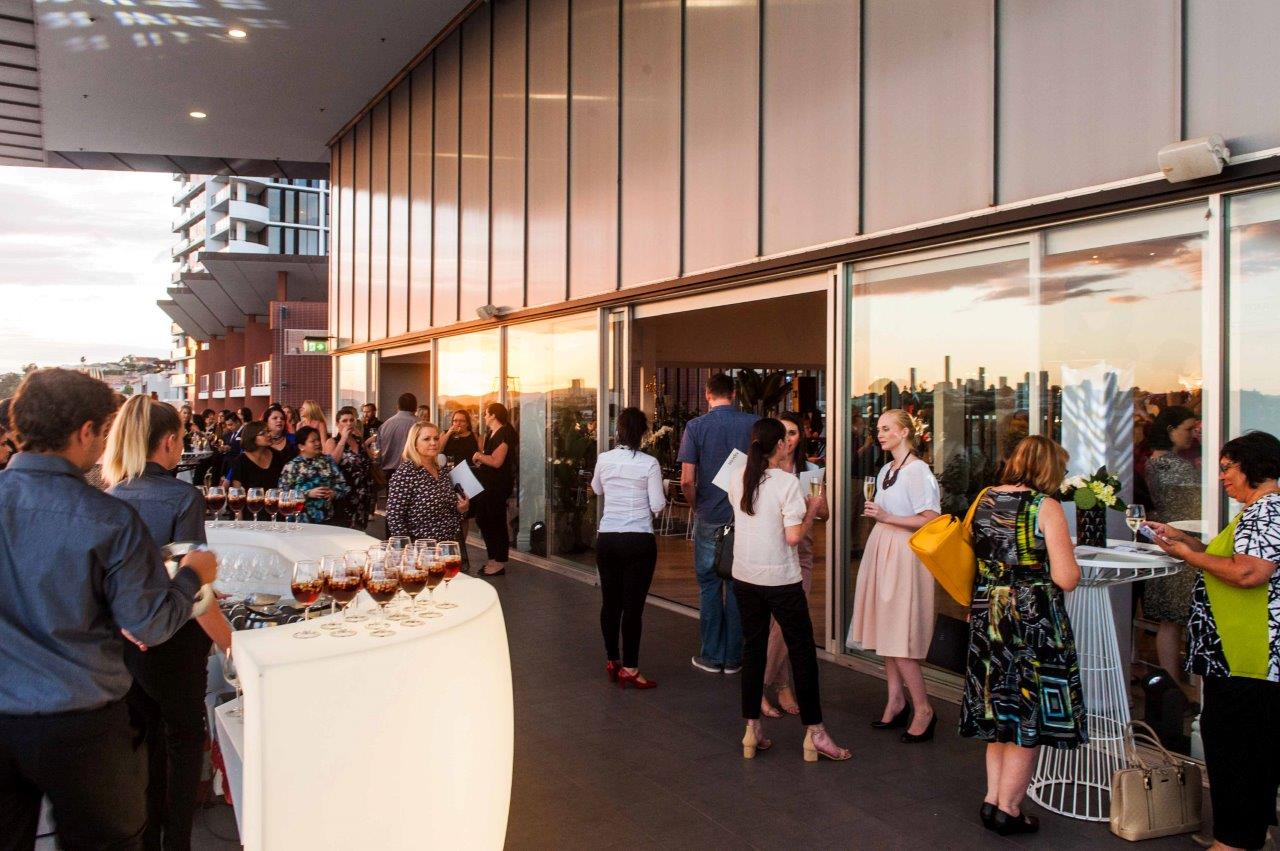

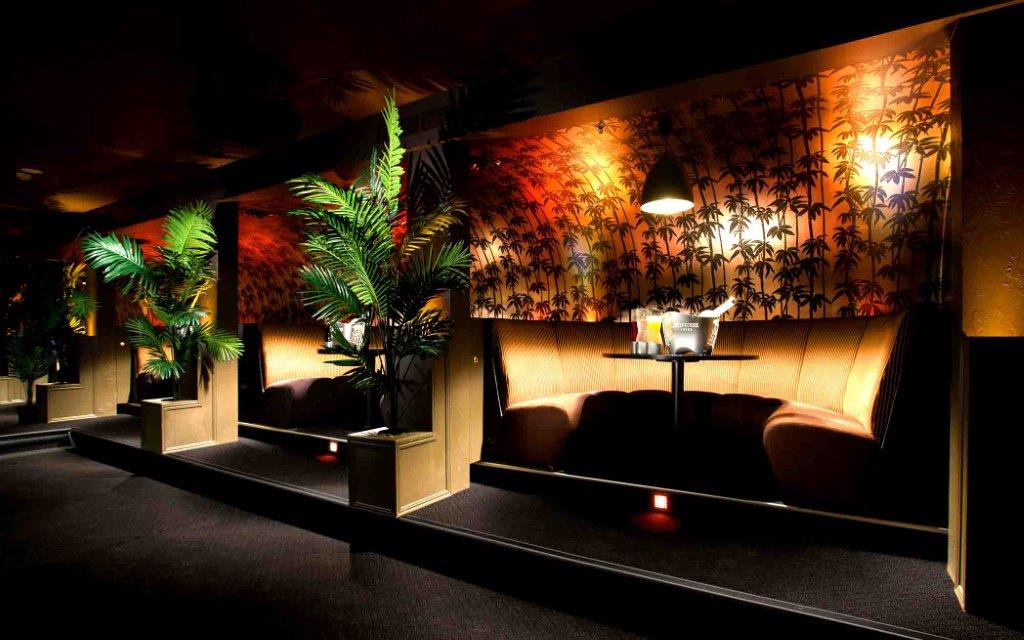
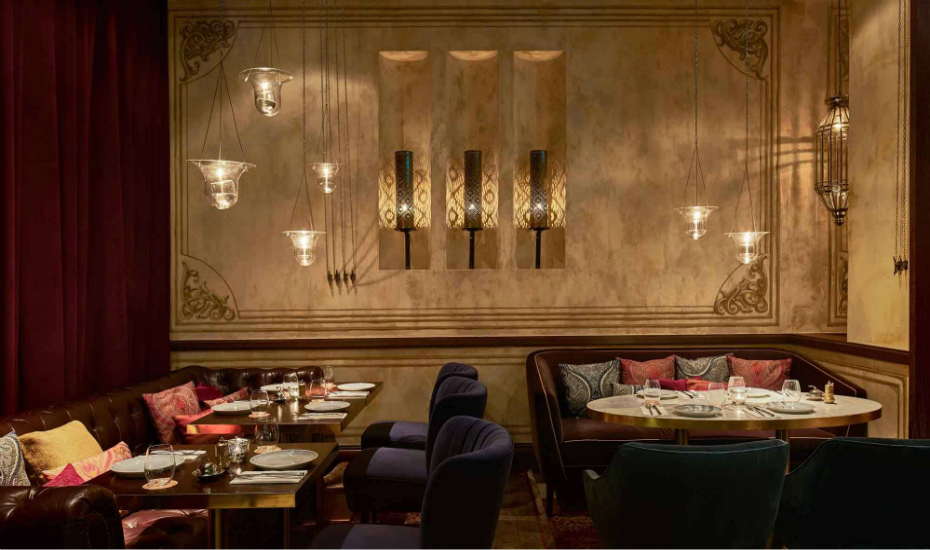
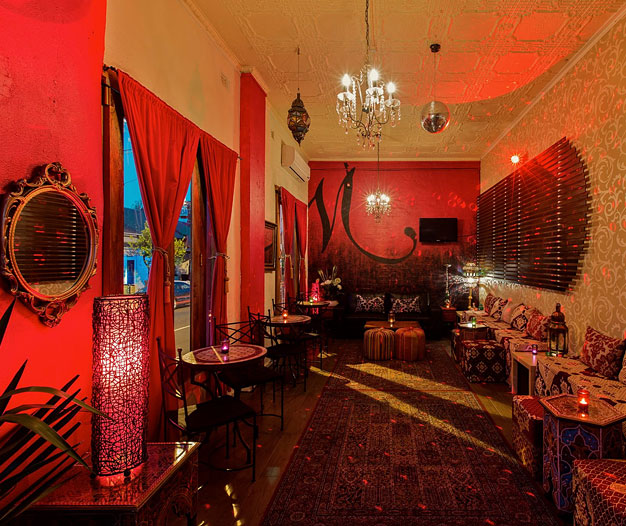
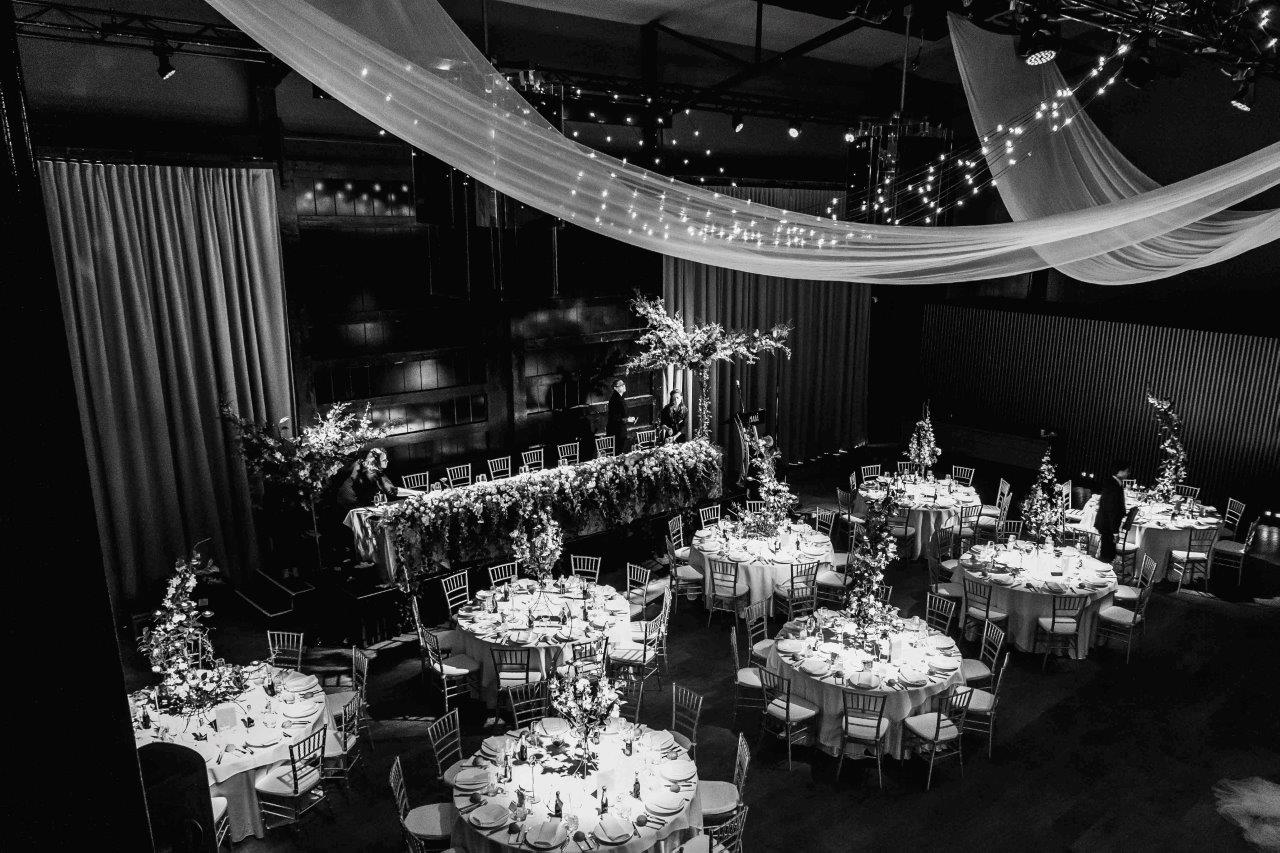
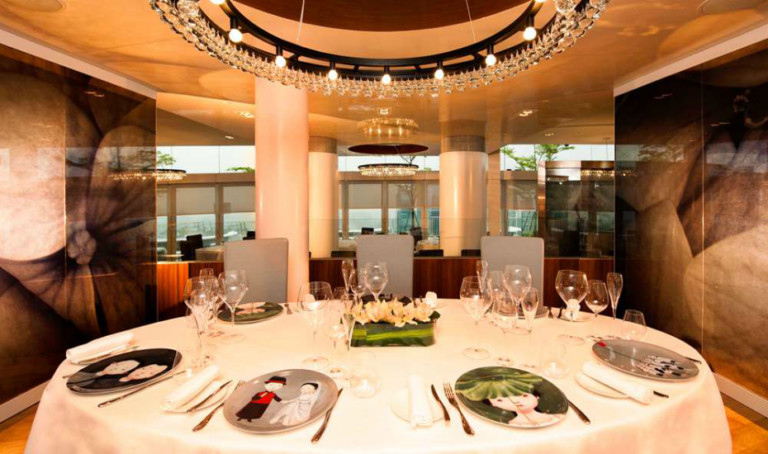

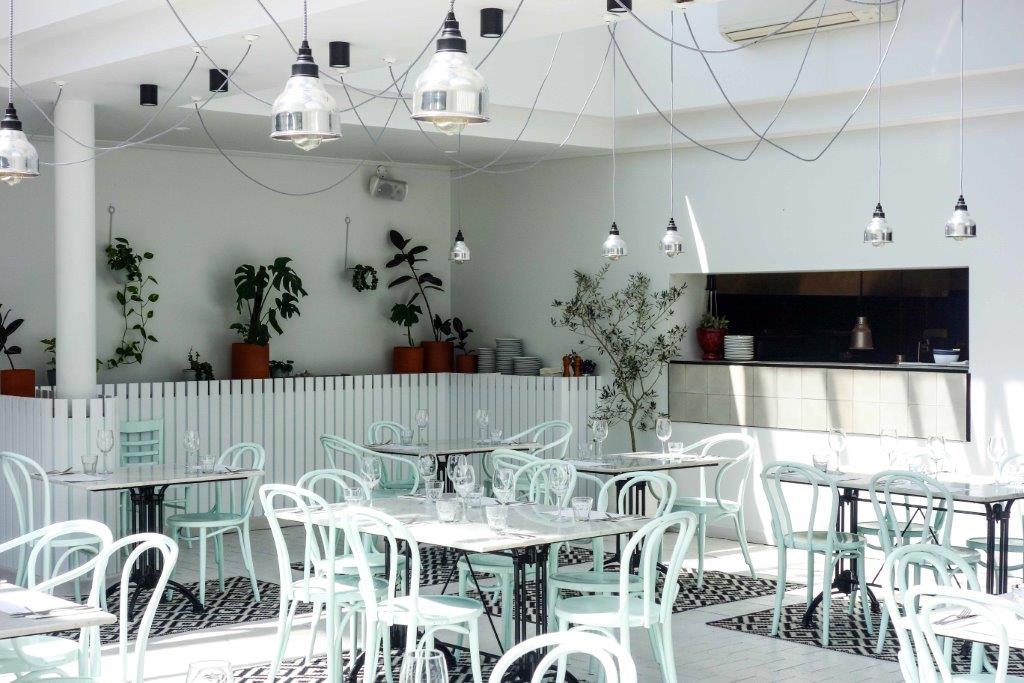


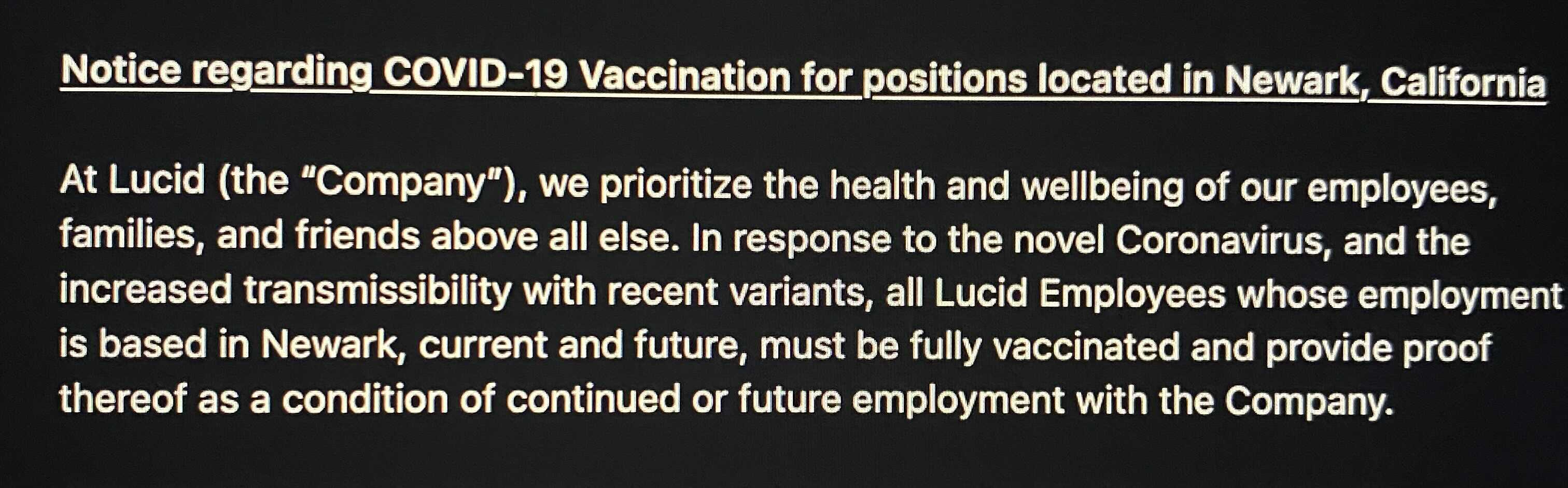





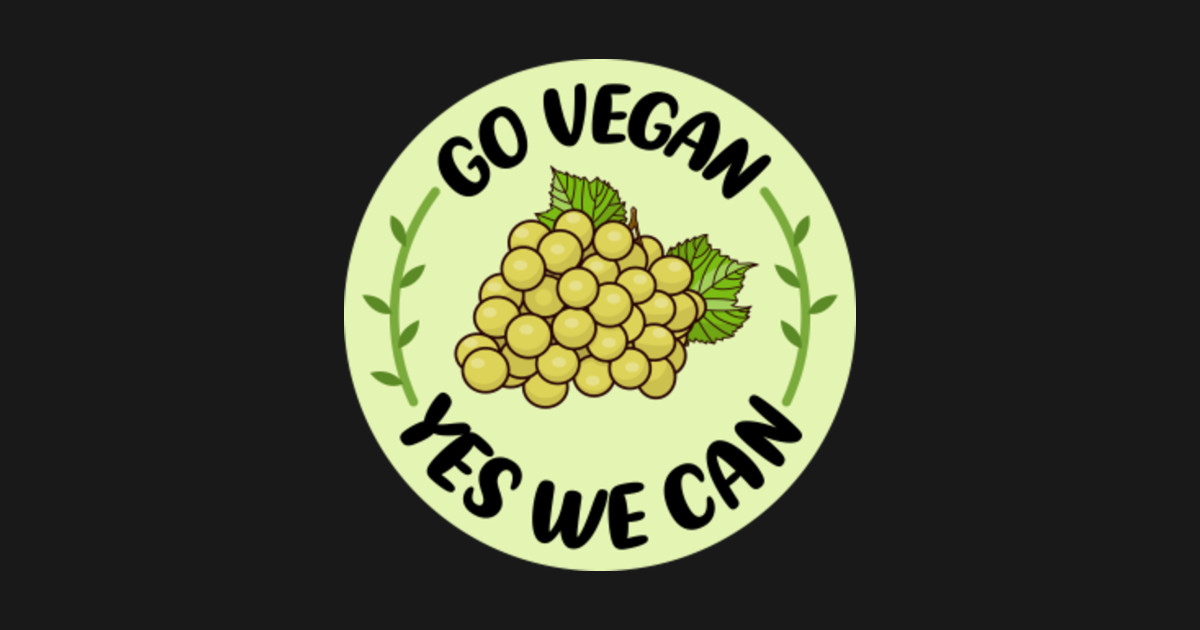

