Delphi Public Private
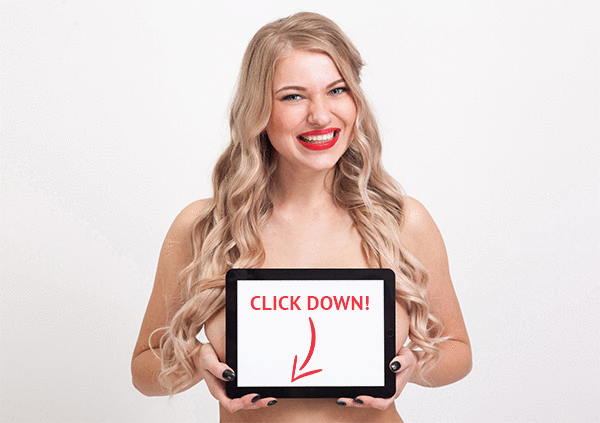
🛑 👉🏻👉🏻👉🏻 INFORMATION AVAILABLE CLICK HERE👈🏻👈🏻👈🏻
Last Updated on Wed, 06 Jan 2021 | Mastering Delphi
For class-based encapsulation, the Object Pascal language has three access specifiers: private, protected, and public. A fourth, published, controls RTTI and design time information and will be discussed in more detail in Chapter 5. Here are the three classic access specifiers:
Generally, the fields of a class should be private; the methods are usually public. However, this is not always the case. Methods can be private or protected if they are needed only internally to perform some partial computation. Fields can be protected so that you can manipulate them in subclasses, but only if you are fairly sure that their type definition is not going to change. Access specifiers only restrict code outside your unit from accessing certain members of classes declared in the interface section of your unit. This means that if two classes are in the same unit, there is no protection for their private fields. Only by placing a class in the interface portion of a unit will you limit the visibility from classes and functions in other units to the public method and fields of the class.
As an example, consider this new version of the TDate class:
Month, Day, Year: Integer; public procedure SetValue (y, m, d: Integer); overload; procedure SetValue (NewDate: TDateTime); overload; function LeapYear: Boolean; function GetText: string; procedure Increase; end;
In this version, the fields are now declared to be private, and there are some new methods. The first, GetText, is a function that returns a string with the date. You might think of adding other functions, such as GetDay, GetMonth, and GetYear, which simply return the corresponding private data, but similar direct data-access functions are not always needed. Providing access functions for each and every field might reduce the encapsulation and make it harder to modify the internal implementation of a class. Access functions should be provided only if they are part of the logical interface of the class you are implementing.
Another new method is the Increase procedure, which increases the date by one day. This is far from simple, because you need to consider the different lengths of the various months as well as leap and non-leap years. What I'll do to make it easier to write the code is change the internal implementation of the class to Delphi's TDateTime type for the internal implementation. The class definition will change to (the complete code will be in the next example, DateProp):
type TDate = class private fDate: TDateTime; public procedure SetValue (y, m, d: Integer); overload; procedure SetValue (NewDate: TDateTime); overload; function LeapYear: Boolean; function GetText: string; procedure Increase; end;
Notice that because the only change is in the private portion of the class, you won't have to modify any of your existing programs that use it. This is the advantage of encapsulation!
The TDateTime type is actually a floating-point number. The integral portion of the number indicates the date since 12/30/1899, the same base date used by OLE Automation and Microsoft applications. (Use negative values to express previous years.) The decimal portion indicates the time as a fraction. For example, a value of 3.75 stands for the second of January 1900, at 6:00 a.m. (three-quarters of a day). To add or subtract dates, you can add or subtract the number of days, which is much simpler than adding days with a day/month/year representation.
19.01.2010, 19:06. Показов 23544. Ответов 7
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs;
type
TForm1 = class(TForm)
procedure FormCreate(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure TForm1.FormCreate(Sender: TObject);
begin
end;
end.
Помощь в написании контрольных, курсовых и дипломных работ здесь.
Разделы Public и Private
Здравствуйте, если у вас есть время, помогите пожалуйста... Возникла такая проблема: во время...
отключить Private и Public
private { Private declarations } public { Public declarations } end; Можно ли...
Public: и private:
Вопрос: данные модиф. доступа могут быть записаны только 1 раз каждый class sample1{ public:...
Public и Private
Доброго времени суток! Написал код и понял, что некоторые методы должны быть private, а не public....
private - раздел частных объявлений;
public - раздел общих объявлений;
Сообщение было отмечено как решение
Хотел бы узнать для чего нужны ключивые слова private и public , в инете толковых обьяснений не нашёл
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs;
type
TForm1 = class(TForm)
procedure FormCreate(Sender: TObject);
private
{ Private declarations }
procedure cls(s:string);
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure TForm1.FormCreate(Sender: TObject);
begin
end;
procedure TForm1.cls(s:string);
begin
showmessage(s);
close;
end;
end.
Сообщение было отмечено как решение
public - доступны всем
protected - потомкам и текущему модулю
private - текущему модулю
strict protected - потомкам
strict private - никому
вроде так... Последние два Delphi 7+
Тут такой вопрос возник, наверное, глупый, но нахрапом ответ в интернете не нашел:
"procedure FormCreate(Sender: TObject);" объявлена сразу после класса формы, ни в private, ни в public, ни под каким разделом, так значит она к какому разделу относится? вообще, что это за область полей между "TForm1 = class(TForm)" и "private"? Спасибо.
DIEsel_92, имхо private.
Просто упомянутые вами секции предназначены для свойств и методов, которые вы создаёте сами, ручками. А то что создаётся автоматом Дельфи помещает именно туда, куда вы и сказали.
что это за область полей между "TForm1 = class(TForm)" и "private"?
Помощь в написании контрольных, курсовых и дипломных работ здесь.
Public/private
Подскажите, пожауйста, где можно почитать про Public и Private механизмы создания документов? А то...
Из private в public
Доброго времени суток. Подскажите, есть ли способ "в ходе выполнения кода" изменить статус...
Поменять public на private
#include <iostream> #include <vector> #include <fstream> #include <cstring> #include <cstdlib>...
private, protected, public
class test { public: test(); int getPrivate(); int vpublic; protected: int vprotected;...
КиберФорум - форум программистов, компьютерный форум, программирование
Powered by vBulletin® Version 3.8.9
Copyright ©2000 - 2021, vBulletin Solutions, Inc.
Mistress Spank Video
Mom And Boy Fucking
Mature Porn Vintage Video
My Girl Xxx
Naked Women Ass Up Gapping
Public and private declarations - Delphi Guide - Delphi Power
Cybern8.com » Delphi. Урок 21. Группы доступа
Private Protected and Public - Mastering Delphi - Delphi Power
Private - Деректива. Справочник - Основы Delphi
Delphi private protected public - IT Справочник
Delphi private protected public - modspintires.net
Public - Деректива. Справочник - Основы Delphi
Delphi Basics : Public command
Delphi Public Private
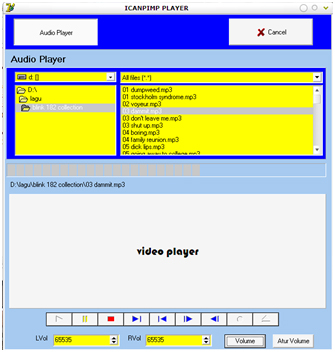

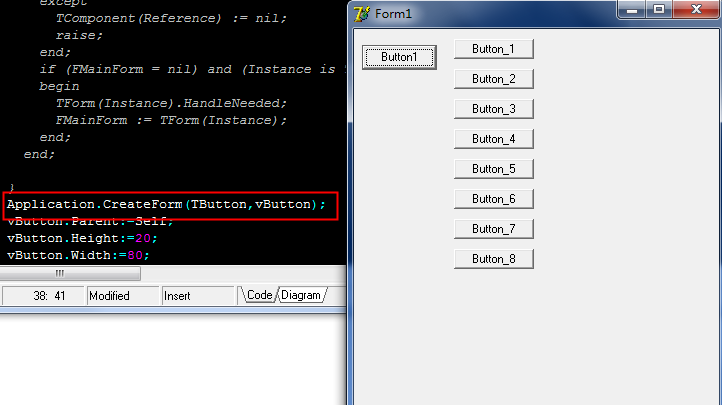


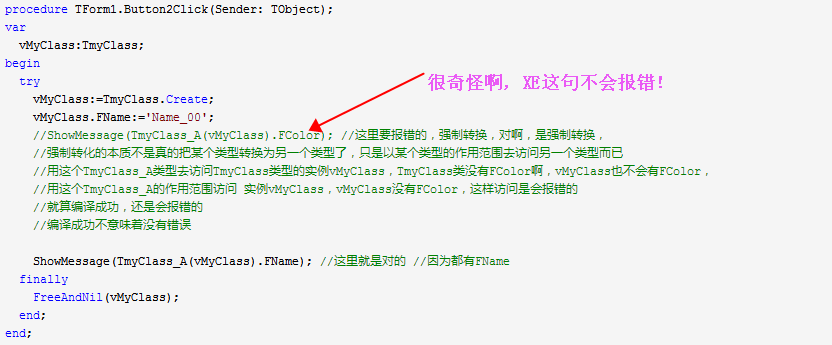
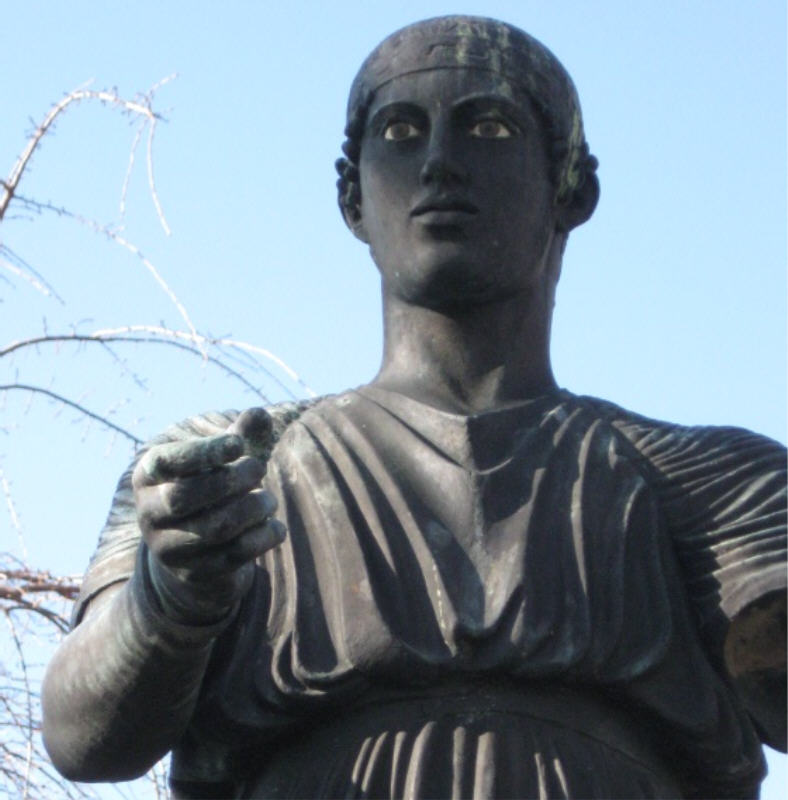
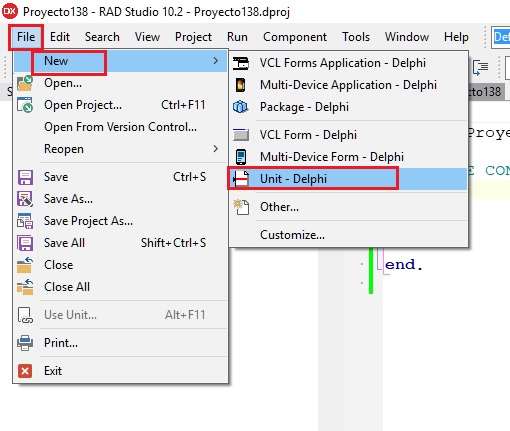

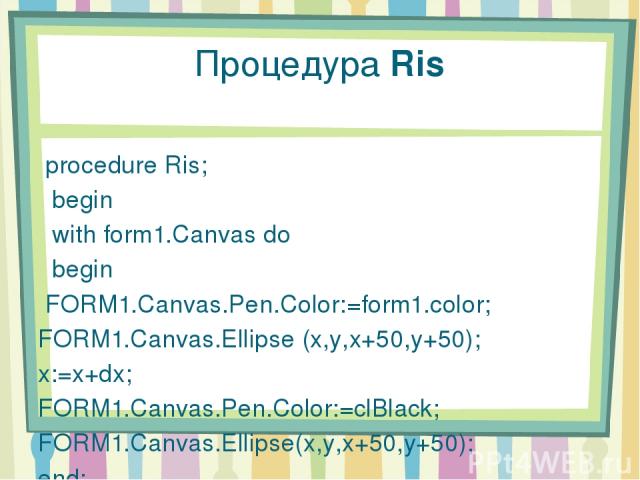
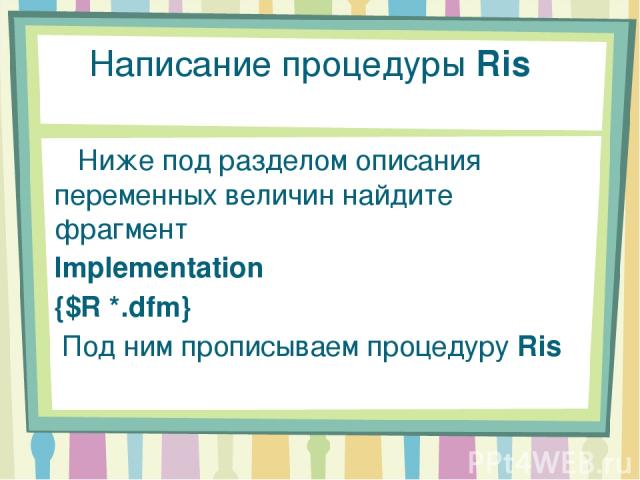
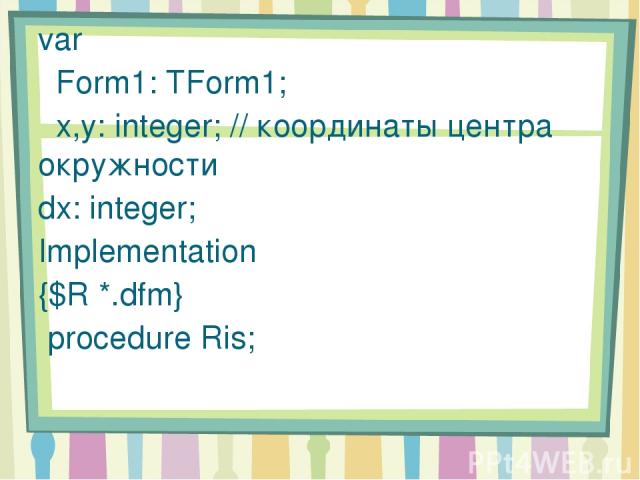

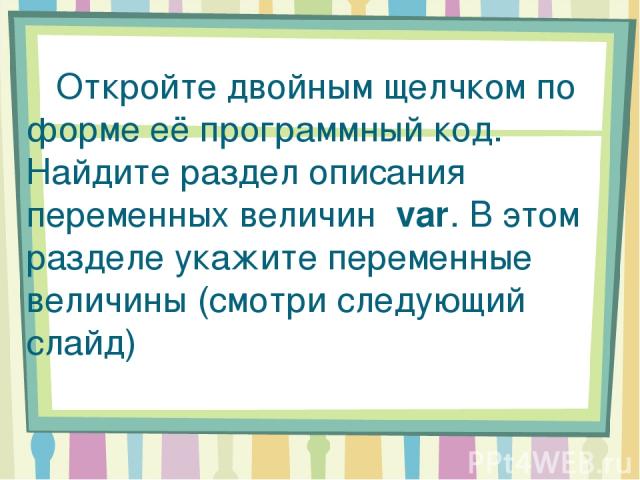
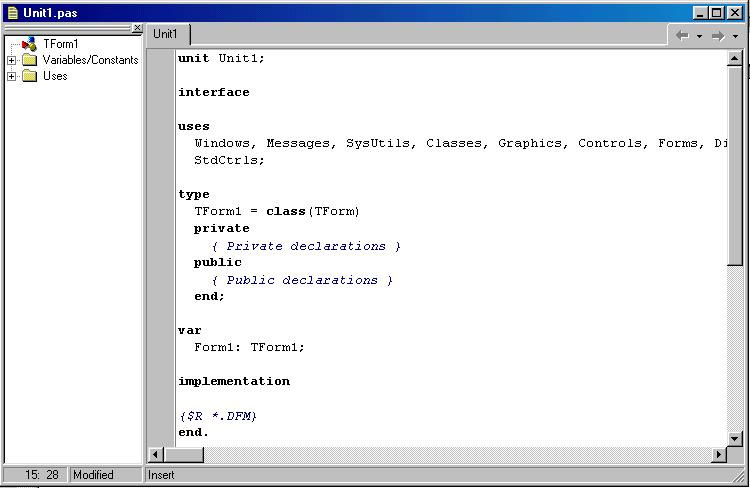
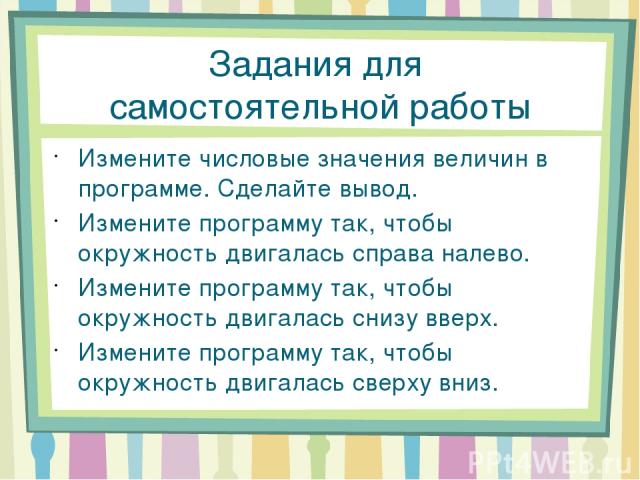

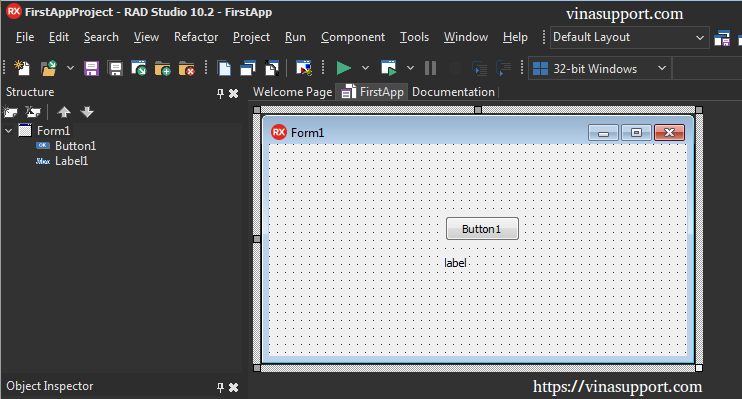
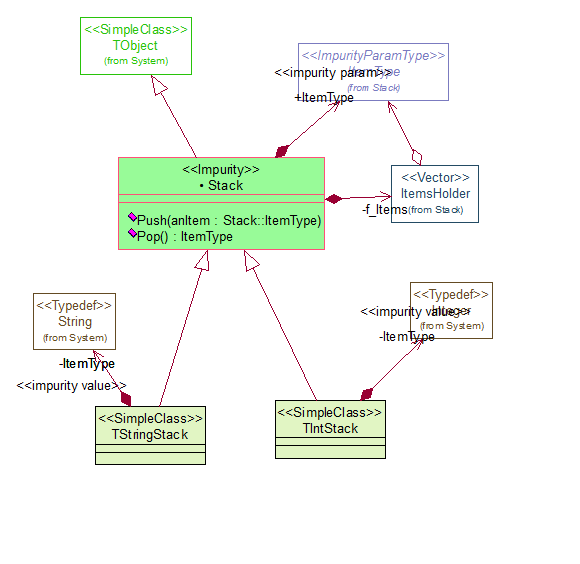
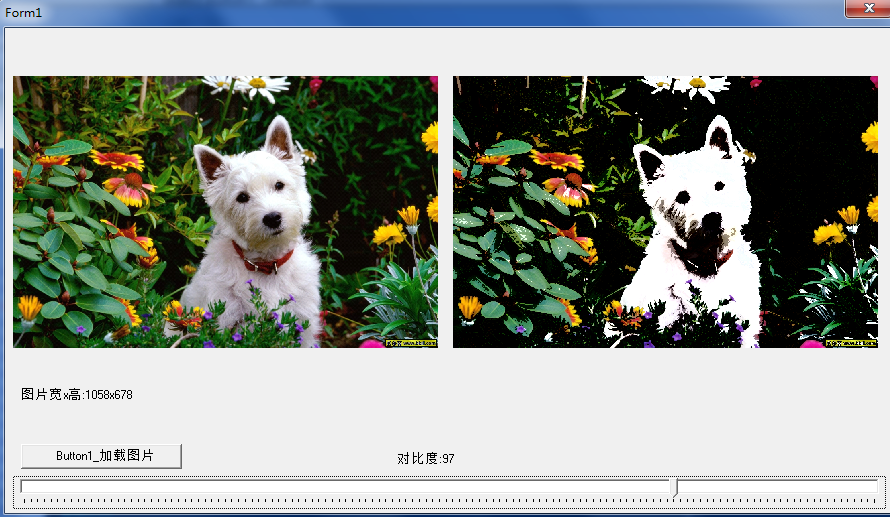


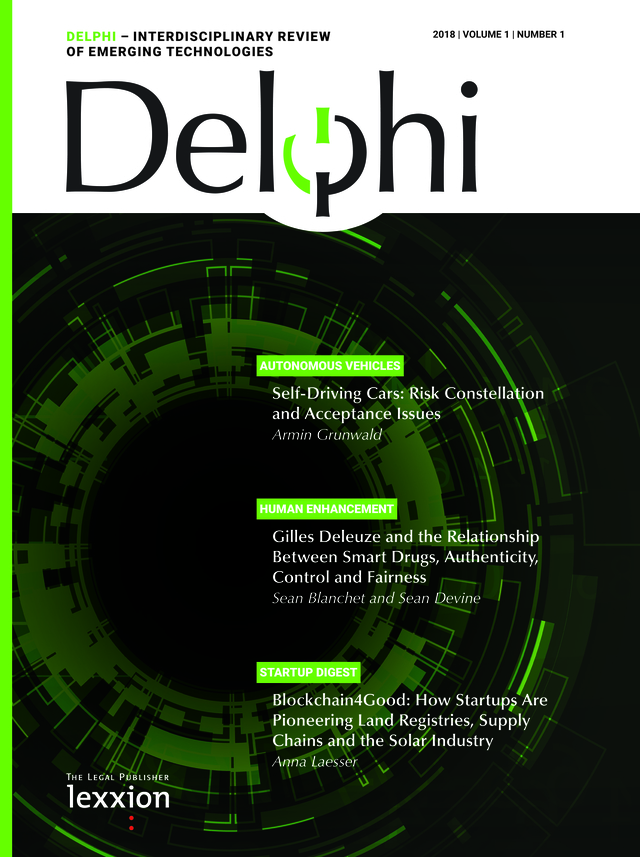

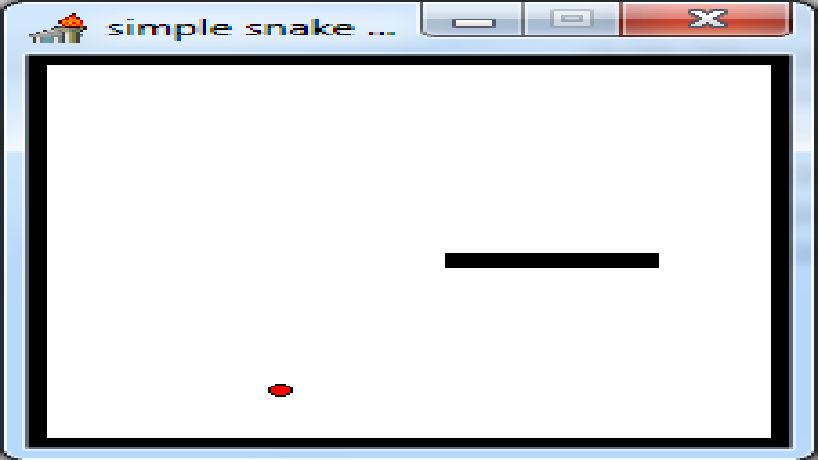

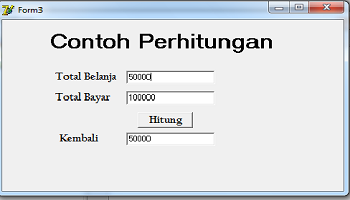
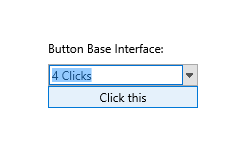
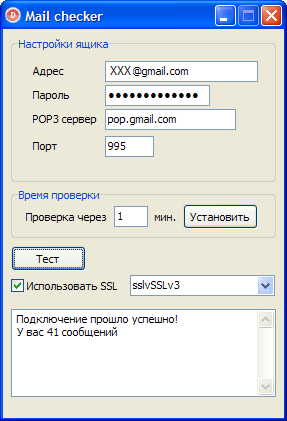
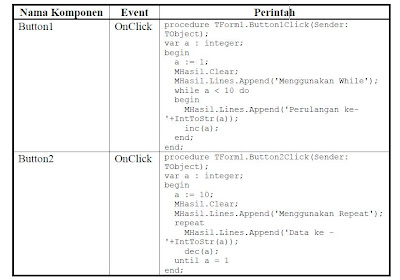



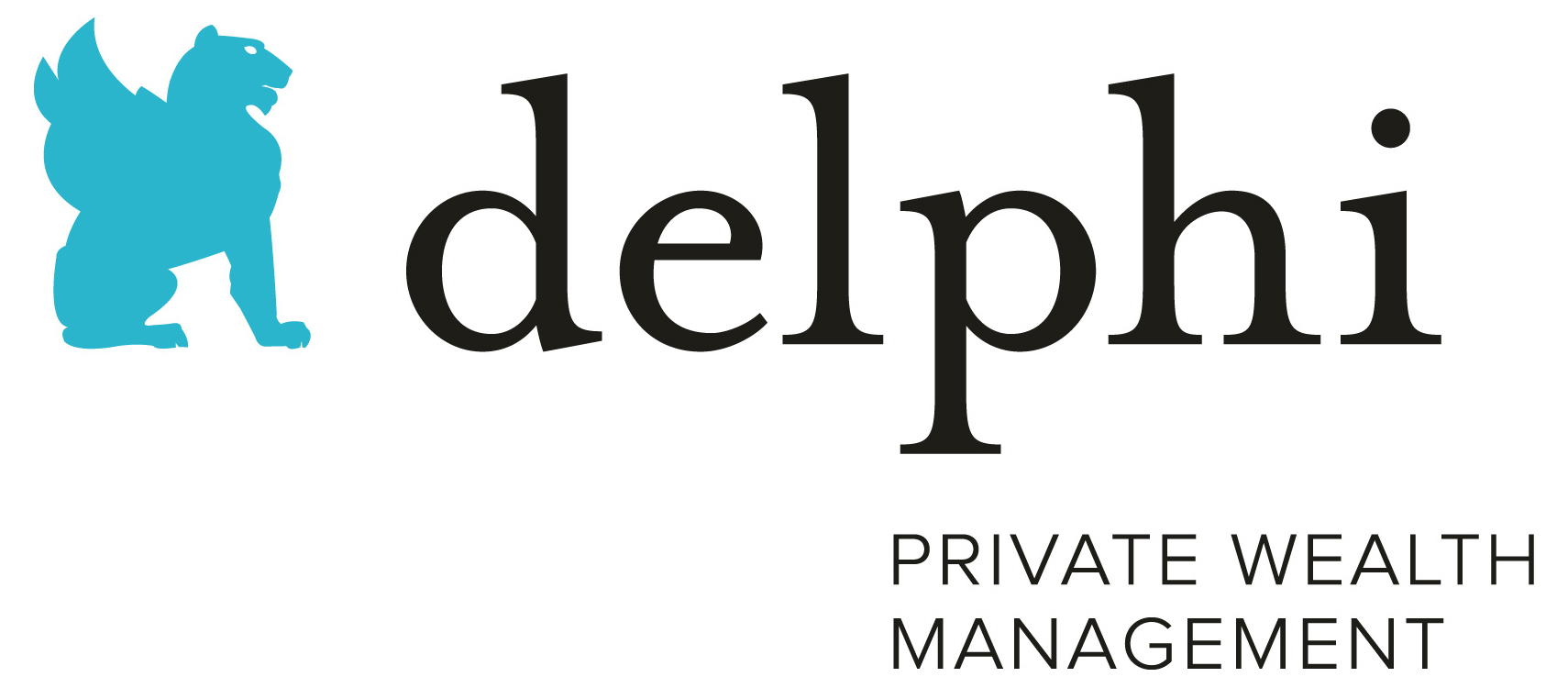


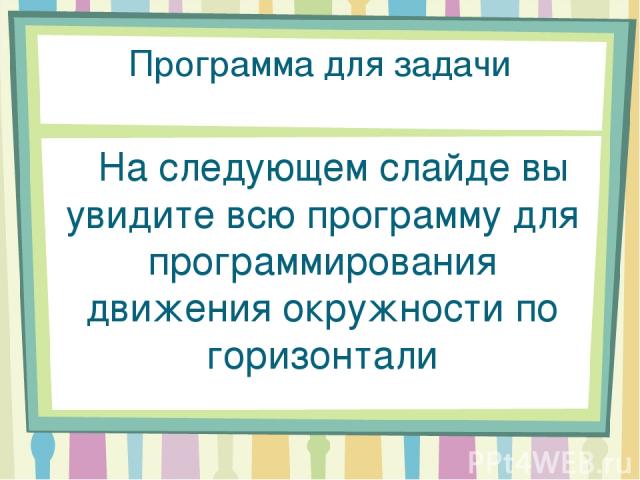

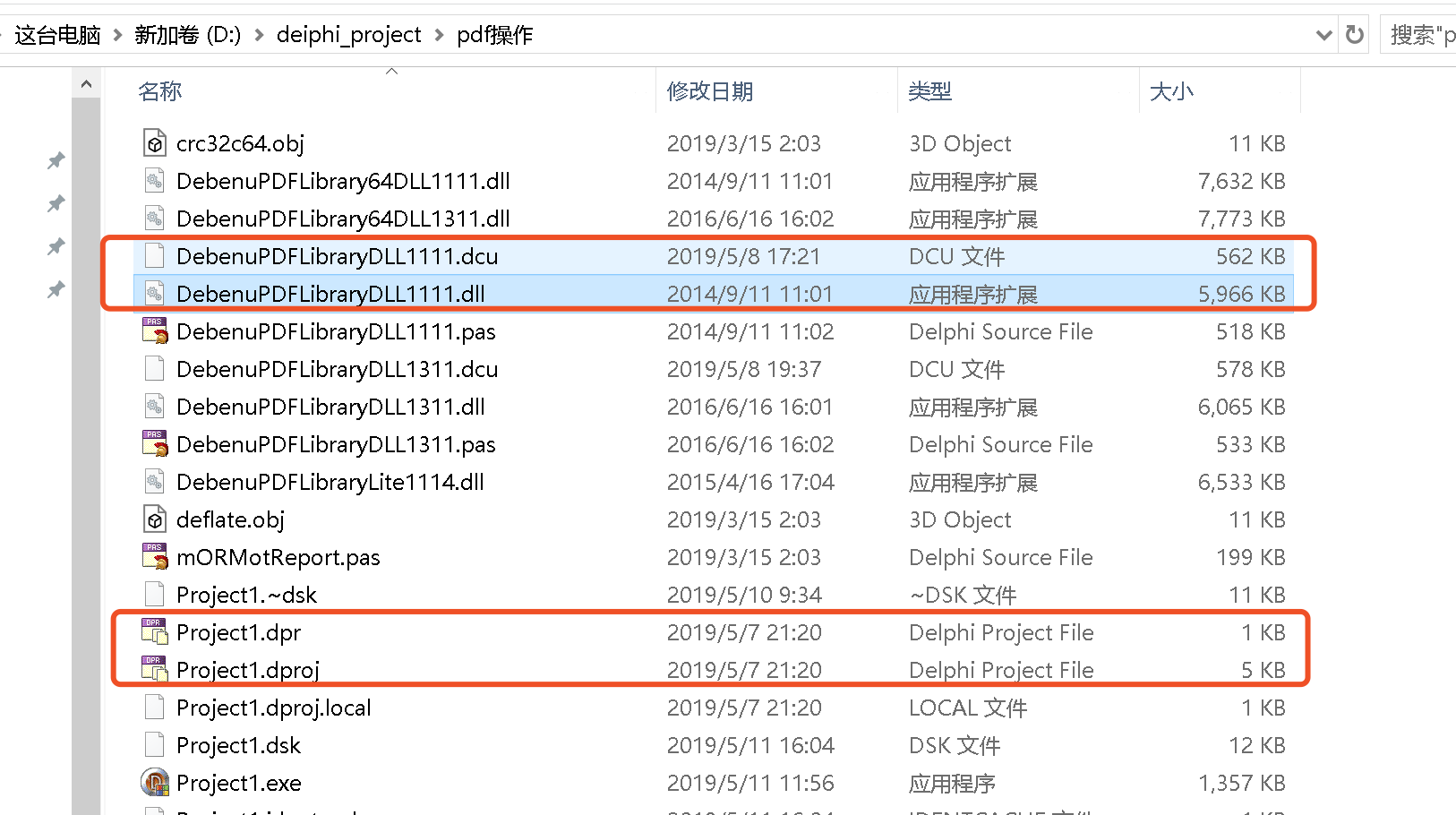
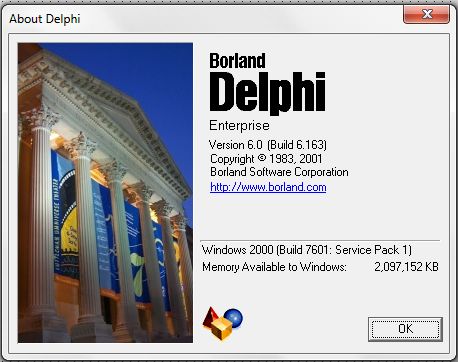
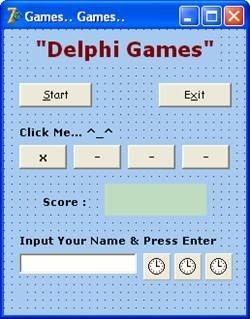
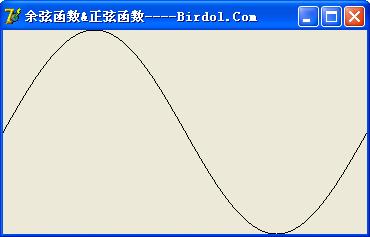
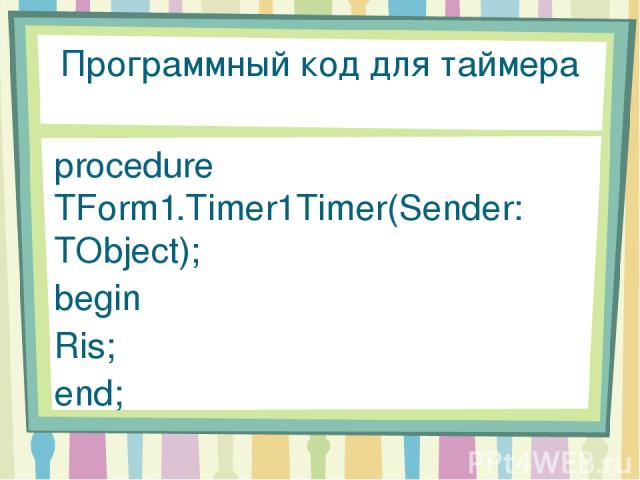
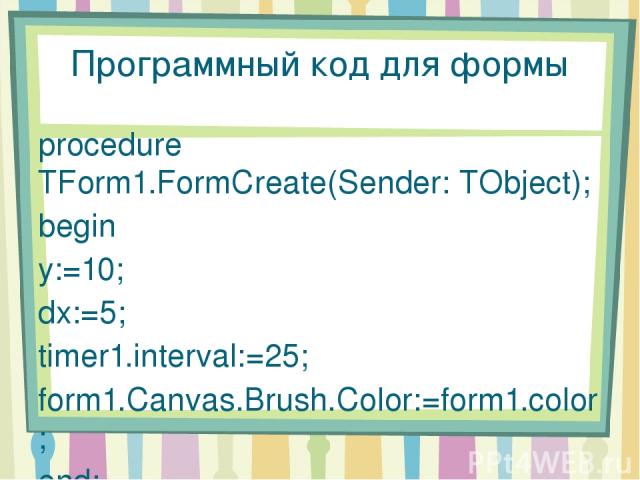
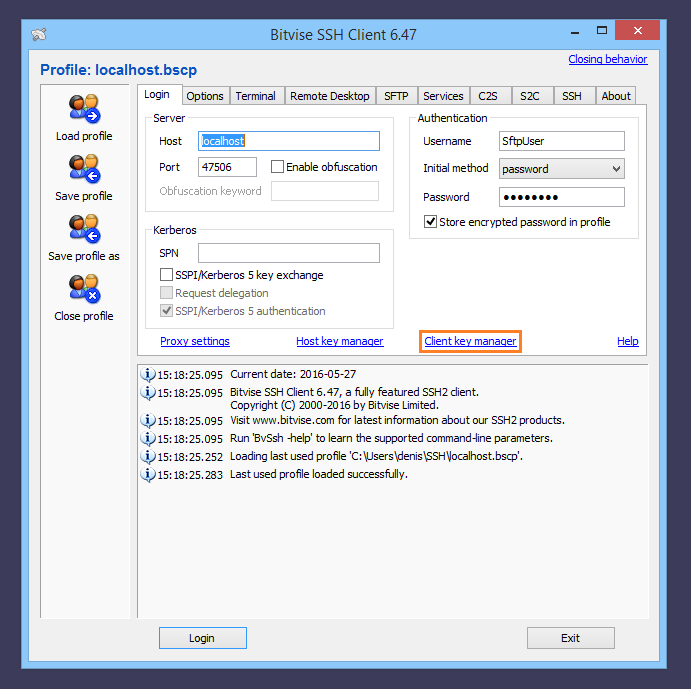

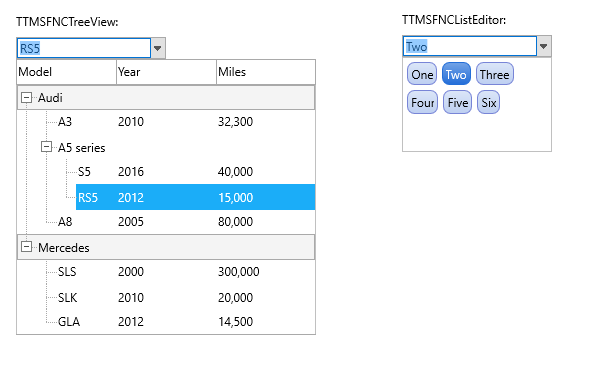
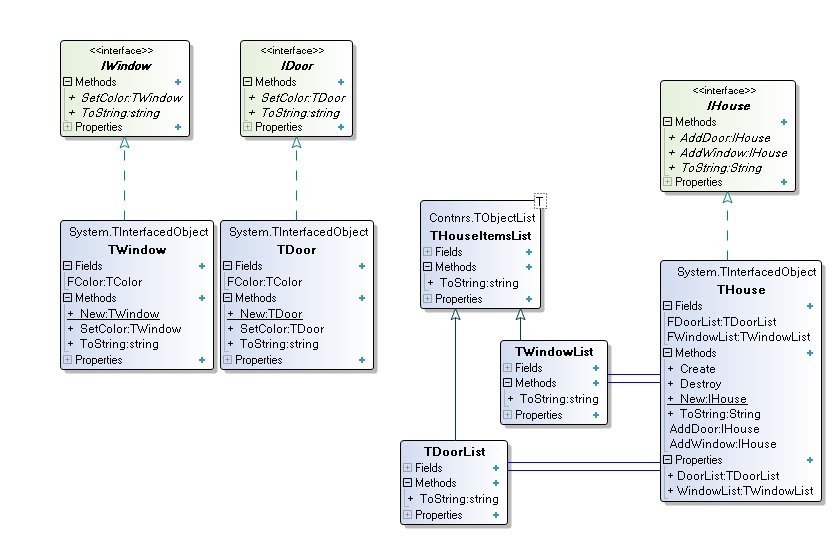

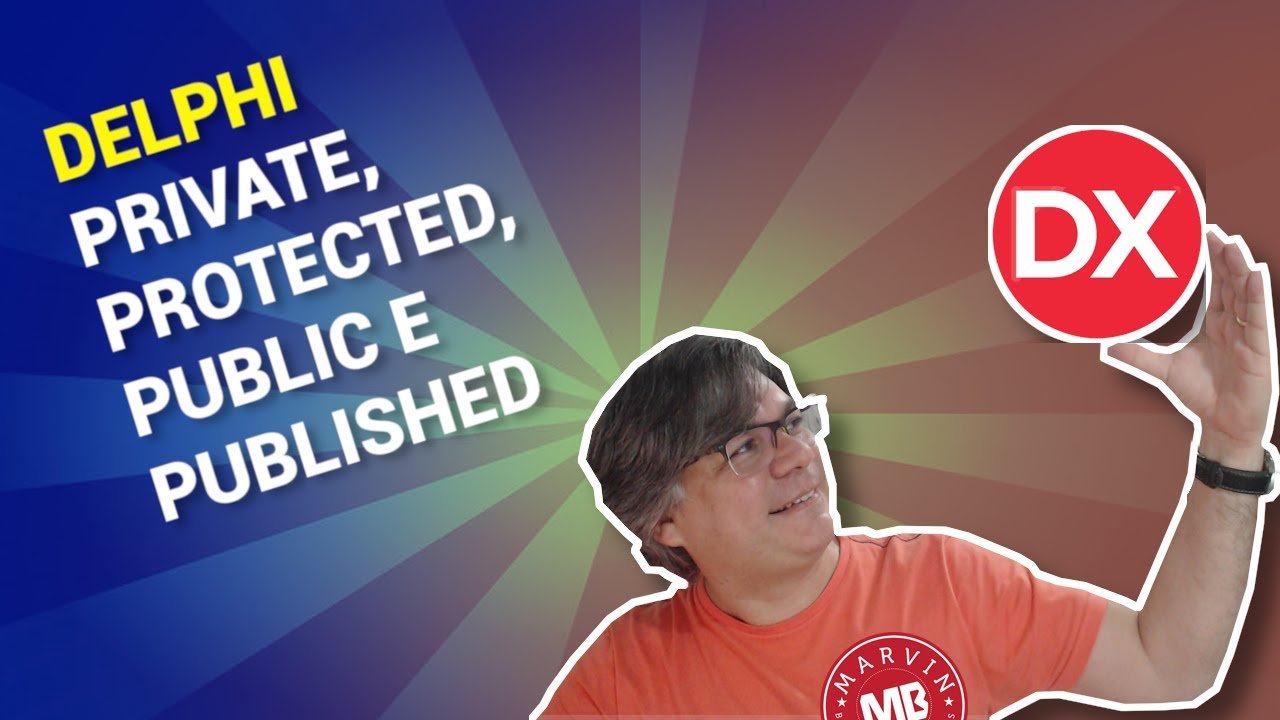