Backpage Jax Ts
💣 👉🏻👉🏻👉🏻 ALL INFORMATION CLICK HERE 👈🏻👈🏻👈🏻
Sign up or log in to customize your list.
Join Stack Overflow to learn, share knowledge, and build your career.
Sign up with email Sign up Sign up with Google Sign up with GitHub Sign up with Facebook
Connect and share knowledge within a single location that is structured and easy to search.
I'm learning JAX-RS (aka, JSR-311) using Jersey. I've successfuly created a Root Resource and am playing around with parameters:
This works great, and handles any format in the current locale which is understood by the Date(String) constructor (like YYYY/mm/dd and mm/dd/YYYY). But if I supply a value which is invalid or not understood, I get a 404 response.
How can I customize this behavior? Maybe a different response code (probably "400 Bad Request")? What about logging an error? Maybe add a description of the problem ("bad date format") in a custom header to aid troubleshooting? Or return a whole Error response with details, along with a 5xx status code?
51k1717 gold badges124124 silver badges176176 bronze badges
29.4k1818 gold badges8989 silver badges119119 bronze badges
There are several approaches to customize the error handling behavior with JAX-RS. Here are three of the easier ways.
The first approach is to create an Exception class that extends WebApplicationException.
And to throw this newly create Exception you simply:
Notice, you don't need to declare the exception in a throws clause because WebApplicationException is a runtime Exception. This will return a 401 response to the client.
The second and easier approach is to simply construct an instance of the WebApplicationException directly in your code. This approach works as long as you don't have to implement your own application Exceptions.
This code too returns a 401 to the client.
Of course, this is just a simple example. You can make the Exception much more complex if necessary, and you can generate what ever http response code you need to.
One other approach is to wrap an existing Exception, perhaps an ObjectNotFoundException with an small wrapper class that implements the ExceptionMapper interface annotated with a @Provider annotation. This tells the JAX-RS runtime, that if the wrapped Exception is raised, return the response code defined in the ExceptionMapper.
2,98611 gold badge1818 silver badges2828 bronze badges
3,60511 gold badge1414 silver badges1515 bronze badges
In your example, the call to super() should be slightly different: super(Response.status(Status.UNAUTHORIZED). entity(message).type("text/plain").build()); Thanks for the insight though. – Jon Onstott Jan 22 '10 at 22:12
In the scenario mentioned in the question, you will not get a chance to throw an exception, as Jersey will raise exception as it will not be able to create instance of Date object from the input value. Is there a way to intercept Jersey exception? There is one ExceptionMapper interface, however that also intercepts the exceptions thrown by the method (get in this case). – Rejeev Divakaran Jun 2 '10 at 11:19
How do you avoid the exception to appear in your server logs if the 404 is a valid case and not an error (i.e. every time you query for a resource, just to see if it already exists, with your approach a stacktrace appears in the server logs). – Guido Nov 24 '11 at 9:34
Worth mentioning that Jersey 2.x defines exceptions for some of the commonest HTTP error codes. So instead of defining your own subclasses of WebApplication, you can use built-in ones like BadRequestException and NotAuthorizedException. Look at the subclasses of javax.ws.rs.ClientErrorException for example. Also note you can supply a details string to the constructors. For example: throw new BadRequestException("Start date must precede end date"); – Bampfer Jul 31 '15 at 20:02
you forgot to mention yet another approach: implementing the ExceptionMapper interface (which is a better approach then extending). See more here vvirlan.wordpress.com/2015/10/19/… – ACV Oct 20 '15 at 20:04
Create above class. This will handle 404 (NotFoundException) and here in toResponse method you can give your custom response. Similarly there are ParamException etc. which you would need to map to provide customized responses.
2,15133 gold badges3030 silver badges5555 bronze badges
99811 gold badge99 silver badges1212 bronze badges
You can use implements ExceptionMapper as well for generic exceptions – Saurabh Oct 26 '15 at 10:03
This would handle WebApplicationExceptions thrown by JAX-RS Client too, hiding the error origin. Better have a custom Exception (not derived from WebApplicationException) or throw WebApplications with complete Response. WebApplicationExceptions thrown by JAX-RS Client should be handled directly at the call, else the response of another service is passed through as response of your service although it is an unhandled internal server error. – Markus Kull Aug 25 '16 at 8:31
Jersey throws an com.sun.jersey.api.ParamException when it fails to unmarshall the parameters so one solution is to create an ExceptionMapper that handles these types of exceptions:
9,46499 gold badges6060 silver badges9898 bronze badges
2,6752323 silver badges2020 bronze badges
where should I create this mapper specifically for Jersey to register it? – Patricio May 30 '13 at 17:55
All you have to do is to add the @Provider annotation, see here for more details: stackoverflow.com/questions/15185299/… – Jan Kronquist Jun 5 '13 at 11:19
You could also write a reusable class for QueryParam-annotated variables
Although the error handling is trivial in this case (throwing a 400 response), using this class allows you to factor-out parameter handling in general which might include logging etc.
49444 silver badges99 bronze badges
I am trying to add a custom query param handler in Jersey (migrating from CXF) this looks remarkably similar to what I am doing but I don't know how to install/create a new provider. Your above class doesn't show me this. I am using JodaTime DateTime objects for QueryParam and don't have a provider to decode them. Is it just as easy as subclassing it, giving it a String constructor and handling that? – Christian Bongiorno Jul 6 '12 at 1:35
Just create a class like the DateParam one above that wraps a org.joda.time.DateTime instead of java.util.Calendar. You use that with @QueryParam rather than DateTime itself. – Charlie Brooking Jul 6 '12 at 6:31
If you are using Joda DateTime, jersey comes with DateTimeParam for you to use directly. No need to write your own. See github.com/dropwizard/dropwizard/blob/master/dropwizard-jersey/… – Srikanth Apr 29 '15 at 18:25
I am going to add this because its super useful, but only if you are using Jackson with Jersey. Jackson 2.x has a JodaModule that can be registered with the ObjectMapper registerModules method. It can handle all of the joda type conversions. com.fasterxml.jackson.datatype.joda.JodaModule – j_walker_dev Aug 25 '15 at 6:53
One obvious solution: take in a String, convert to Date yourself. That way you can define format you want, catch exceptions and either re-throw or customize error being sent. For parsing, SimpleDateFormat should work fine.
I am sure there are ways to hook handlers for data types too, but perhaps little bit of simple code is all you need in this case.
101k2828 gold badges186186 silver badges226226 bronze badges
I too like StaxMan would probably implement that QueryParam as a String, then handle the conversion, rethrowing as necessary.
If the locale specific behavior is the desired and expected behavior, you would use the following to return the 400 BAD REQUEST error:
throw new WebApplicationException(Response.Status.BAD_REQUEST);
See the JavaDoc for javax.ws.rs.core.Response.Status for more options.
2,10922 gold badges1414 silver badges1717 bronze badges
" The type T of the annotated parameter, field or property must either:
1) Be a primitive type
2) Have a constructor that accepts a single String argument
3) Have a static method named valueOf or fromString that accepts a single String argument (see, for example, Integer.valueOf(String))
4) Have a registered implementation of javax.ws.rs.ext.ParamConverterProvider JAX-RS extension SPI that returns a javax.ws.rs.ext.ParamConverter instance capable of a "from string" conversion for the type.
5) Be List, Set or SortedSet, where T satisfies 2, 3 or 4 above. The resulting collection is read-only. "
If you want to control what response goes to user when query parameter in String form can't be converted to your type T, you can throw WebApplicationException. Dropwizard comes with following *Param classes you can use for your needs.
If you need Joda DateTime, just use Dropwizard DateTimeParam.
If the above list does not suit your needs, define your own by extending AbstractParam. Override parse method. If you need control over error response body, override error method.
Date(String arg) constructor is deprecated. I would use Java 8 date classes if you are on Java 8. Otherwise joda date time is recommended.
8,86422 gold badges1717 silver badges2828 bronze badges
This is the correct behavior actually. Jersey will try to find a handler for your input and will try to construct an object from the provided input. In this case it will try to create a new Date object with the value X provided to the constructor. Since this is an invalid date, by convention Jersey will return 404.
What you can do is rewrite and put birth date as a String, then try to parse and if you don't get what you want, you're free to throw any exception you want by any of the exception mapping mechanisms (there are several).
7,80344 gold badges5656 silver badges7171 bronze badges
I wanted to catch all the errors at a central place and transform them.
Following is the code for how I handled it.
Create the following class which implements ExceptionMapper and add @Provider annotation on this class. This will handle all the exceptions.
Override toResponse method and return the Response object populated with customised data.
32711 silver badge1414 bronze badges
Approach 1: By extending WebApplicationException class
Create new exception by extending WebApplicationException
Now throw 'RestException' whenever required.
You can see complete application at this link.
Approach 2: Implement ExceptionMapper
Following mapper handles exception of type 'DataNotFoundException'
You can see complete application at this link.
2,5091919 silver badges3737 bronze badges
Just as an extension to @Steven Lavine answer in case you want to open the browser login window. I found it hard to properly return the Response (MDN HTTP Authentication) from the Filter in case that the user wasn't authenticated yet
This helped me to build the Response to force browser login, note the additional modification of the headers. This will set the status code to 401 and set the header that causes the browser to open the username/password dialog.
10433 silver badges99 bronze badges
Highly active question. Earn 10 reputation in order to answer this question. The reputation requirement helps protect this question from spam and non-answer activity.
Senior Software Engineers, Full Stack
Globavista Ltd (trading as BigOceanData)No office location
Juni Technology ABNo office location
Lead Frontend Developer / Vue.js (m/f/x)
Senior Fullstack (Node/React) Developer (Remote, EU and US Timezones)
Instructor (m/f/x) for PHP Web Development (freelance)
Senior Full Stack Developer (Cloud)
Senior Web Developer (Node/ReactJS)
To subscribe to this RSS feed, copy and paste this URL into your RSS reader.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.2.17.38594
IBM и Red Hat - новая глава открытых инноваций. Узнать больше
Этот сайт больше не обновляется и не поддерживается. Содержание предоставляется "как есть". Учитывая быстрое развитие технологий, некоторые материалы, код или иллюстрации могут к настоящему моменту устареть.
Навин Балани (Naveen Balani) и Раджив Хати (Rajeev Hathi)
Опубликовано 21.12.2009
В этом учебном руководстве мы спроектируем и разработаем приложение обработки заказов, отображающее свою функциональность в виде Web-сервисов, посредством которых различные клиенты могут размещать информацию о заказе независимым от используемой платформы способом.
После прочтения данного учебного руководства вы сможете применить концепции и полученные знания для разработки Web-сервисов в ваших приложениях, используя технологию JAX-WS.
Чтобы успешно освоить данное учебное руководство, вы должны понимать основы технологии Web-сервисов и иметь некоторый опыт программирования на языке Java.
Чтобы выполнять примеры данного руководства, вам необходимо установить Java Platform, Standard Edition (Java SE) 6.0.
JAX-WS - это технология, разработанная для упрощения создания Web-сервисов и клиентов Web-сервисов на языке Java. Она предоставляет полный стек Web-сервисов, облегчающий разработку и развертывание Web-сервисов. JAX-WS поддерживает WS-I Basic Profile 1.1. Это гарантирует, что Web-сервисы, разработанные с использованием стека JAX-WS, могут потребляться любыми клиентами, разработанными на любом языке программирования и удовлетворяющими стандарту WS-I Basic Profile. JAX-WS также включает в себя JAXB (Java Architecture for XML Binding) и SAAJ (SOAP with Attachments API for Java).
JAXB реализует возможности связывания данных, предоставляя удобный способ отобразить XML-схему на представление в Java-коде. JAXB скрывает преобразование сообщений XML-схемы в SOAP-сообщенияя от Java-кода, избавляя разработчика от необходимости глубокого понимания XML и SOAP-анализа. Спецификация JAXB определяет связывание между Java и XML-схемами. SAAJ обеспечивает стандартный способ работы с XML-вложениями, находящимися в SOAP-сообщении.
Более того, JAX-WS ускоряет разработку Web-сервисов, предоставляя библиотеку аннотаций для преобразования POJO-классов (plain old Java object – традиционные Java-объекты) в Web-сервисы. Она также определяет детализированное отображение сервисов, определенных на языке WSDL (Web Services Description Language), в Java-классы, реализующие эти сервисы. Все сложные типы, определенные в WSDL, отображаются в Java-классы согласно отображению, определенному спецификацией JAXB. JAX-WS ранее поставлялась с платформой Java Platform, Enterprise Edition (Java EE) 5. Спецификация JAX-WS 2.0 разрабатывается под эгидой JSR 224 Java Community Process (JCP).
Хорошим способом знакомства с JAX-WS является разработка Web-сервиса. Web-сервис можно разработать двумя способами:
Подход с первичностью WSDL требует хорошего понимания WSDL и XSD (XML Schema Definition) для определения форматов сообщений. Если вы являетесь новичком в Web-сервисах, сначала лучше придерживаться принципа первичности кода. Этот подход и будет использоваться в данном учебном руководстве при разработке Web-сервисов.
При использовании подхода первичности кода работа начинается с Java-класса или классов, реализующих функциональные возможности, которые вы хотите отобразить в виде сервисов. Такой подход особенно полезен, когда Java-реализации уже существуют и необходимо лишь отобразить их в виде сервисов.
Давайте начнем с создания Web-сервиса обработки заказов, принимающего информацию о заказе, месте доставки, заказываемых позициях и, в конечном итоге, генерирующего в качестве ответа идентификатор подтверждения (confirmation ID). Код этого сервиса представлен в листинге 1. Это макетная реализация, выводящая идентификатор клиента и количество заказываемых позиций на консоль, а затем возвращающая фиктивный идентификатор заказа A1234. (Исходный код полного приложения можно загрузить, используя ссылки, приведенные в разделе "Загрузка" данного руководства.) Извлеките исходный код на диск C в папку JAXWS-Tutorial. Эта папка содержит исходный код, показанный в листинге 1.
package com.ibm.jaxws.tutorial.service;
import com.ibm.jaxws.tutorial.service.bean.OrderBean;
//JWS-аннотация, указывающая, что имя portType Web-сервиса
//равно "OrderProcessPort", имя сервиса равно
//"OrderProcess", а targetNamespace, использующийся в сгенерированном
//WSDL, равен "http://jawxs.ibm.tutorial/jaxws/orderprocess".
@WebService(serviceName = "OrderProcess",
portName = "OrderProcessPort",
targetNamespace = "http://jawxs.ibm.tutorial/jaxws/orderprocess")
//JWS-аннотация, определяющая отображение сервиса на
//протокол SOAP-сообщений. В частности, она определяет, что SOAP-сообщения
@SOAPBinding(style=SOAPBinding.Style.DOCUMENT,use=SOAPBinding.Use.LITERAL,
parameterStyle=SOAPBinding.ParameterStyle.WRAPPED)
public OrderBean processOrder(OrderBean orderBean) {
System.out.println("processOrder called for customer"
+ orderBean.getCustomer().getCustomerId());
// Заказанными позициями являются
if (orderBean.getOrderItems() != null) {
System.out.println("Number of items is "
+ orderBean.getOrderItems().length);
//Установить идентификатор заказа.
orderBean.setOrderId("A1234");
OrderBean хранит информацию о заказе, как показано в листинге 2. В частности, он содержит ссылки на клиента, заказанные позиции и объект адреса доставки.
package com.ibm.jaxws.tutorial.service.bean;
private Address shippingAddress;
private OrderItem[] orderItems;
public Customer getCustomer() {
public void setCustomer(Customer customer) {
public void setOrderId(String orderId) {
public Address getShippingAddress() {
public void setShippingAddress(Address shippingAddress) {
this.shippingAddress = shippingAddress;
public OrderItem[] getOrderItems() {
public void setOrderItems(OrderItem[] orderItems) {
this.orderItems = orderItems;
Отправным пунктом разработки JAX-WS Web-сервиса является Java-класс, аннотируемый при помощи аннотации javax.jws.WebService. Используемые аннотации JAX-WS являются частью метаданных Web Services Metadata для спецификации Java Platform (JSR-181). Как вы, вероятно, заметили, OrderProcessService аннотируется при помощи аннотации WebService, которая определяет класс как оконечную точку Web-сервиса.
Класс OrderProcessService (это класс с аннотацией @javax.jws.WebService) неявно определяет интерфейс оконечной точки (service endpoint interface - SEI), объявляющий методы, которые клиент может активизировать в сервисе. Все public-методы, определенные в классе (если они не аннотированы аннотацией @WebMethod с элементом exclude, установленным в true), отображаются на WSDL-операции. Аннотация @WebMethod является необязательной и используется для настройки работы Web-сервиса. Помимо элемента exclude, аннотация javax.jws.WebMethod предоставляет название операции и элементы action, которые используются для настройки атрибута name и элемента SOAP action в WSDL-документе. Эти свойства не обязательны; если они не определены, из имени класса наследуются значения по умолчанию.
После реализации Web-сервиса необходимо сгенерировать все артефакты, требующиеся для развертывания сервиса, а затем спакетировать Web-сервис в развертываемый артефакт (обычно в WAR-файл) и развернуть WAR-файл на любом совместимом сервере, поддерживающем спецификацию JAX-WS 2.0. Обычно сгенерированные артефакты являются классами, обеспечивающими преобразование Java-объектов в XML, а WSDL-файл и XSD-схема основываются на интерфейсе сервиса.
Для тестирования в Java 6 включен облегченный Web-сервер, на котором можно публиковать Web-сервис путем активизации простого вызова API. Далее мы рассмотрим, как протестировать Web-сервисы, используя данный подход.
Переносимые JAX-WS-артефакты для Web-сервиса обработки заказов генерируются при помощи программы wsgen. Эта программа считывает класс Web SEI и генерирует все необходимые артефакты для развертывания и активизации Web-сервиса. Программа wsgen генерирует WSDL-файл и XSD-схему для Web-сервиса, который нужно опубликовать.
Для генерирован
Evolution of Jax's "Gotcha" Grab (1993-2019) - YouTube
java - JAX-RS / Jersey how to customize error handling? - Stack Overflow
Проектирование и разработка Web-сервисов JAX-WS 2.0
JAX Quickstart — JAX documentation
Примеры использования Java JAXB - чтение и запись в файл XML
St Pete Mature Escorts
Videochat Girls
Queeny Luv Porn
Backpage Jax Ts



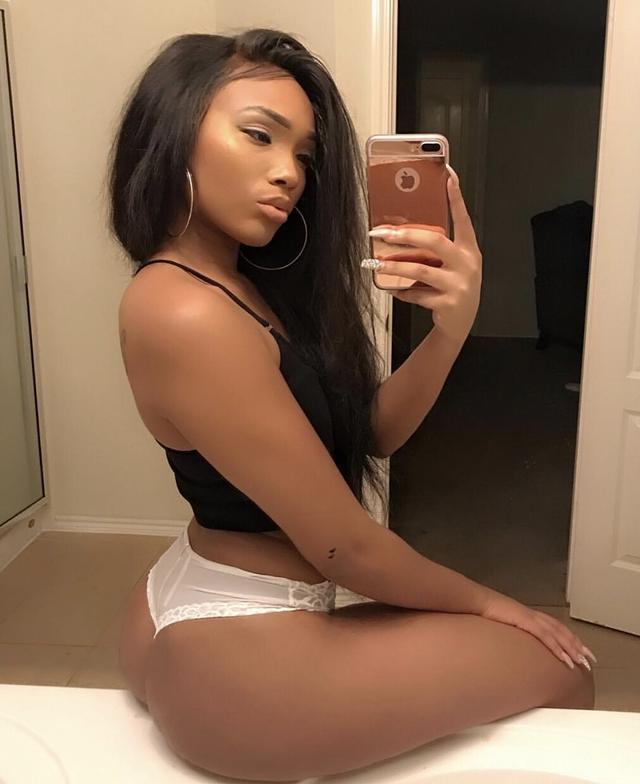
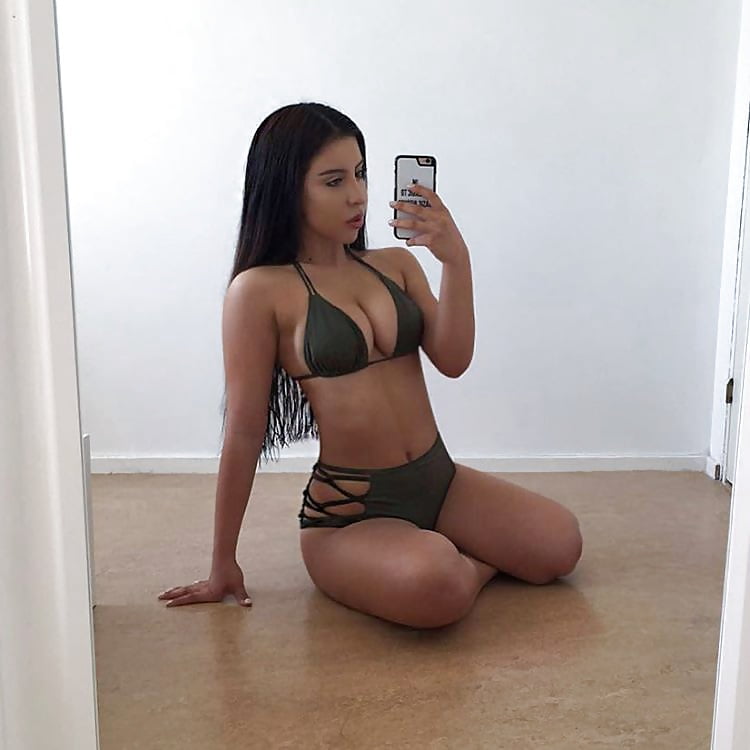



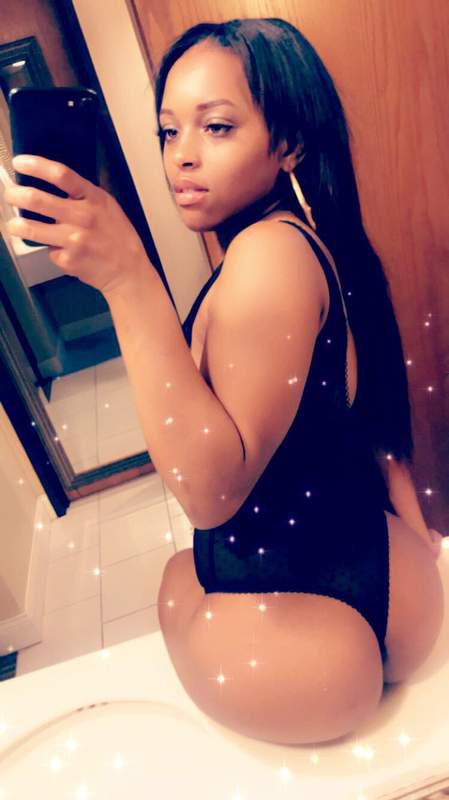
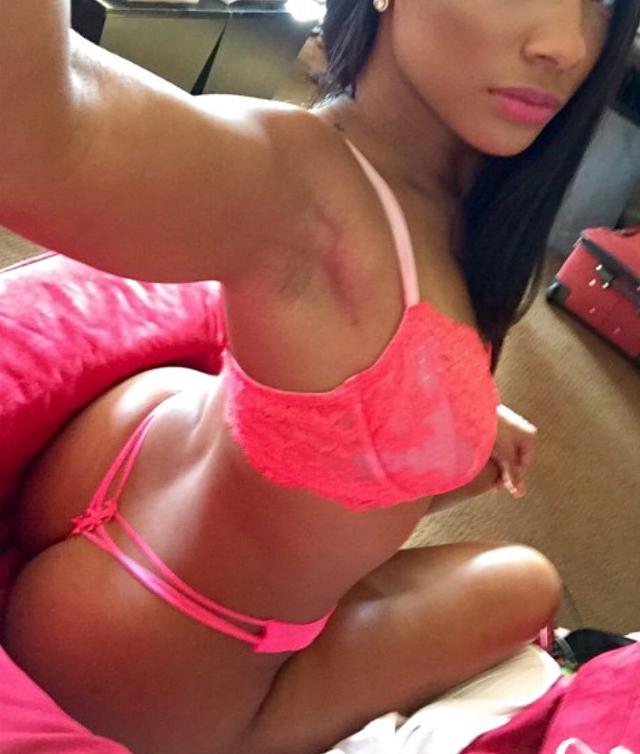





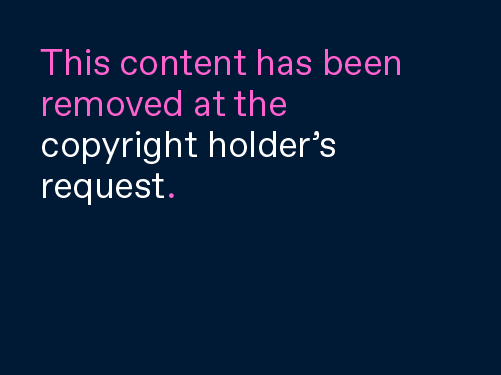
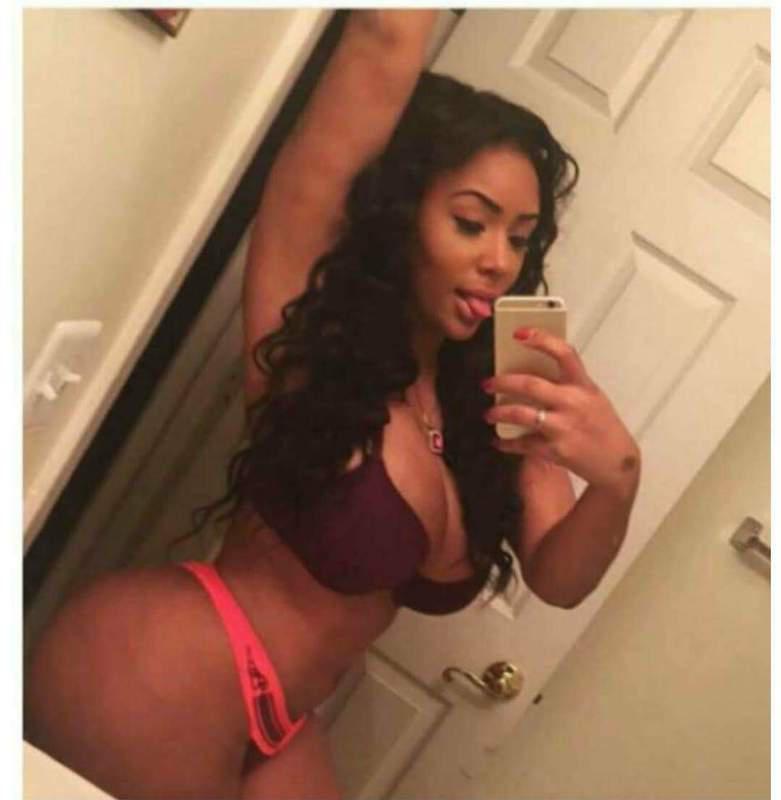
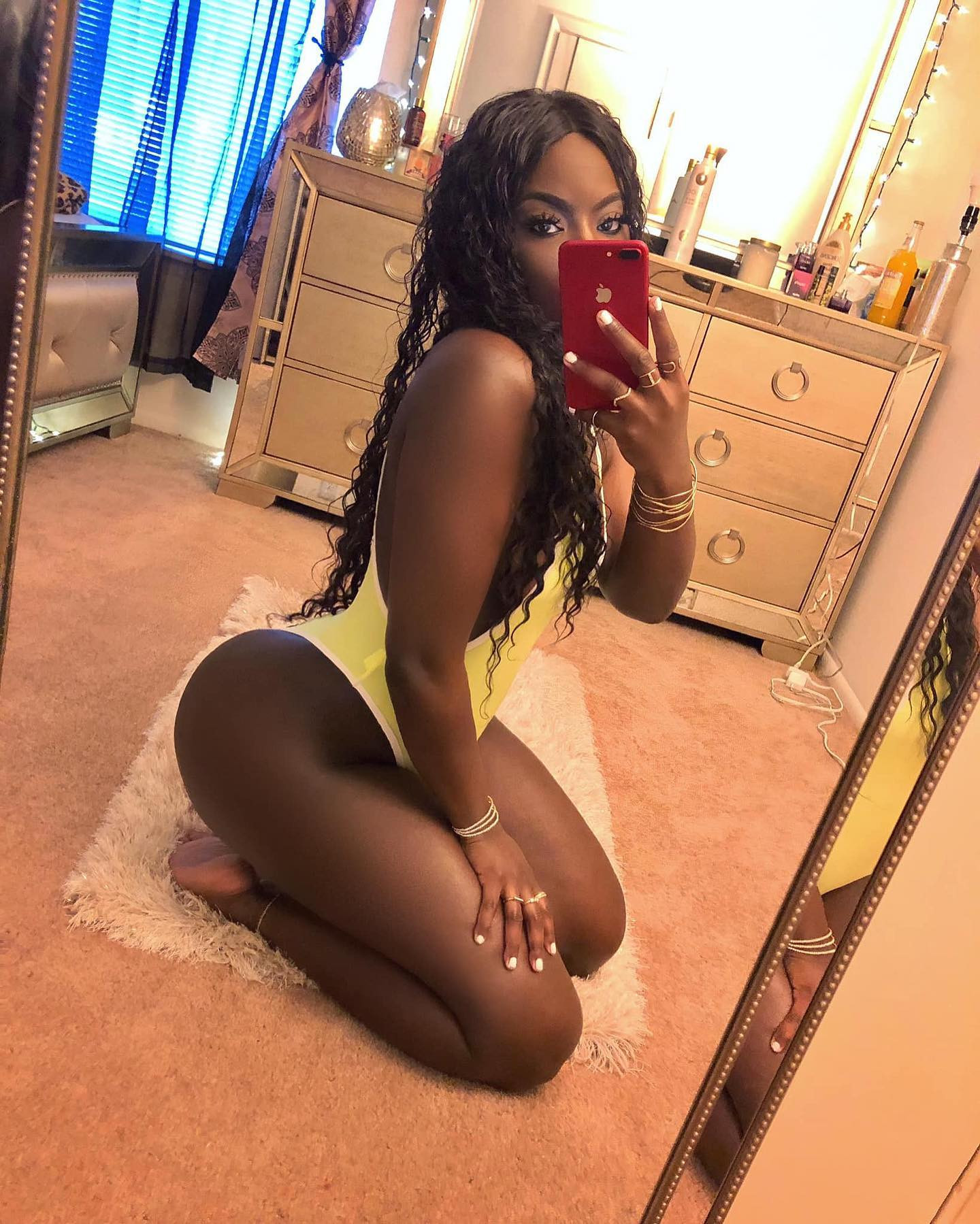



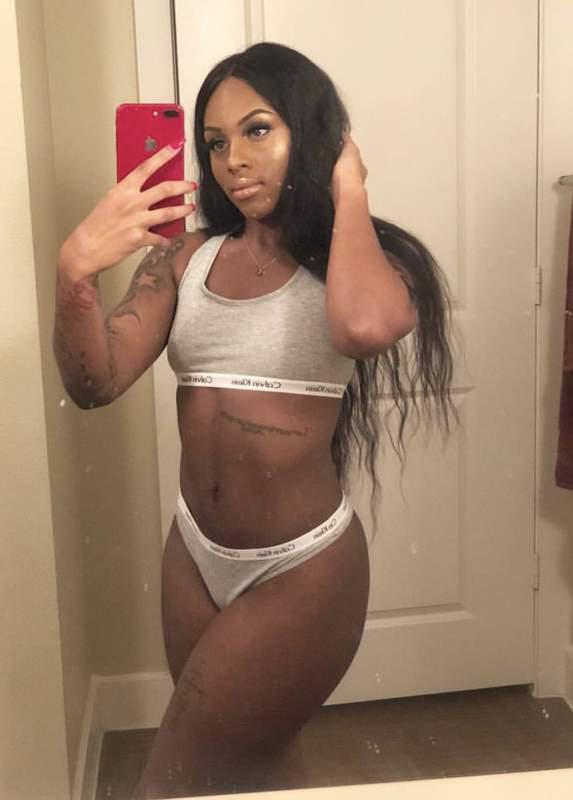

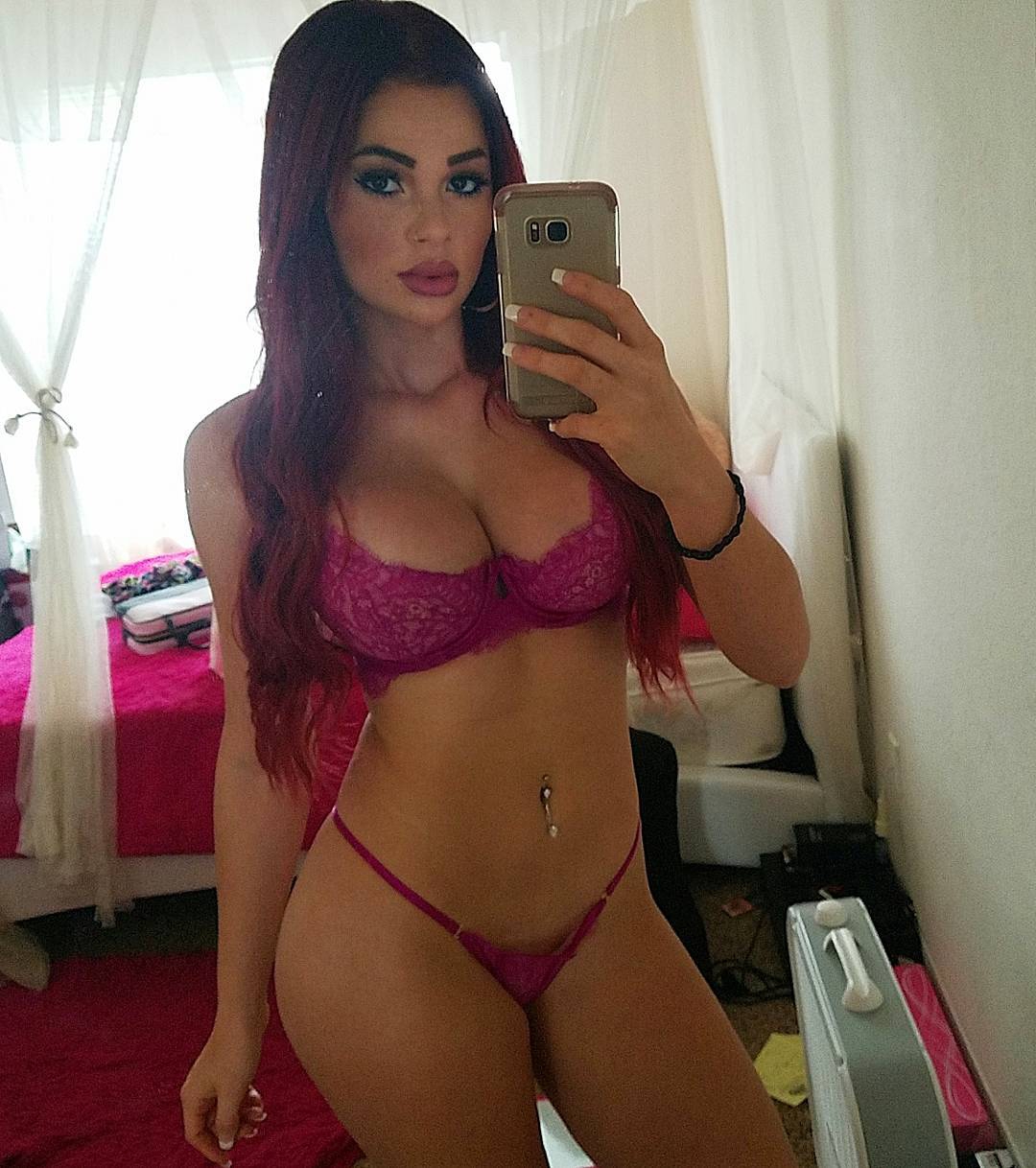



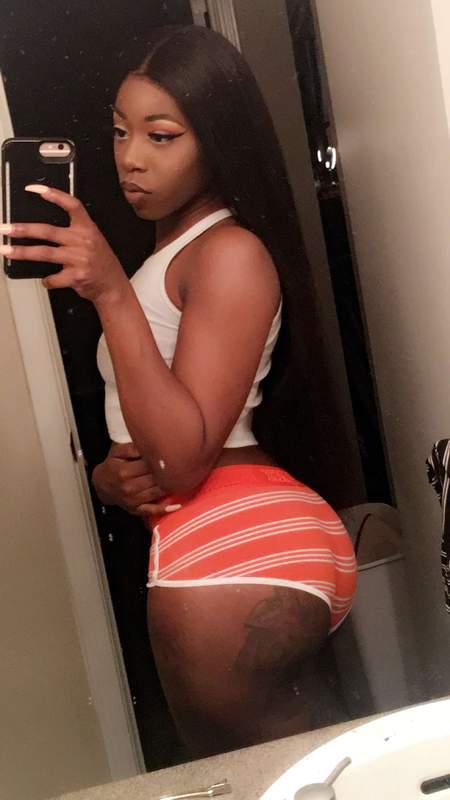



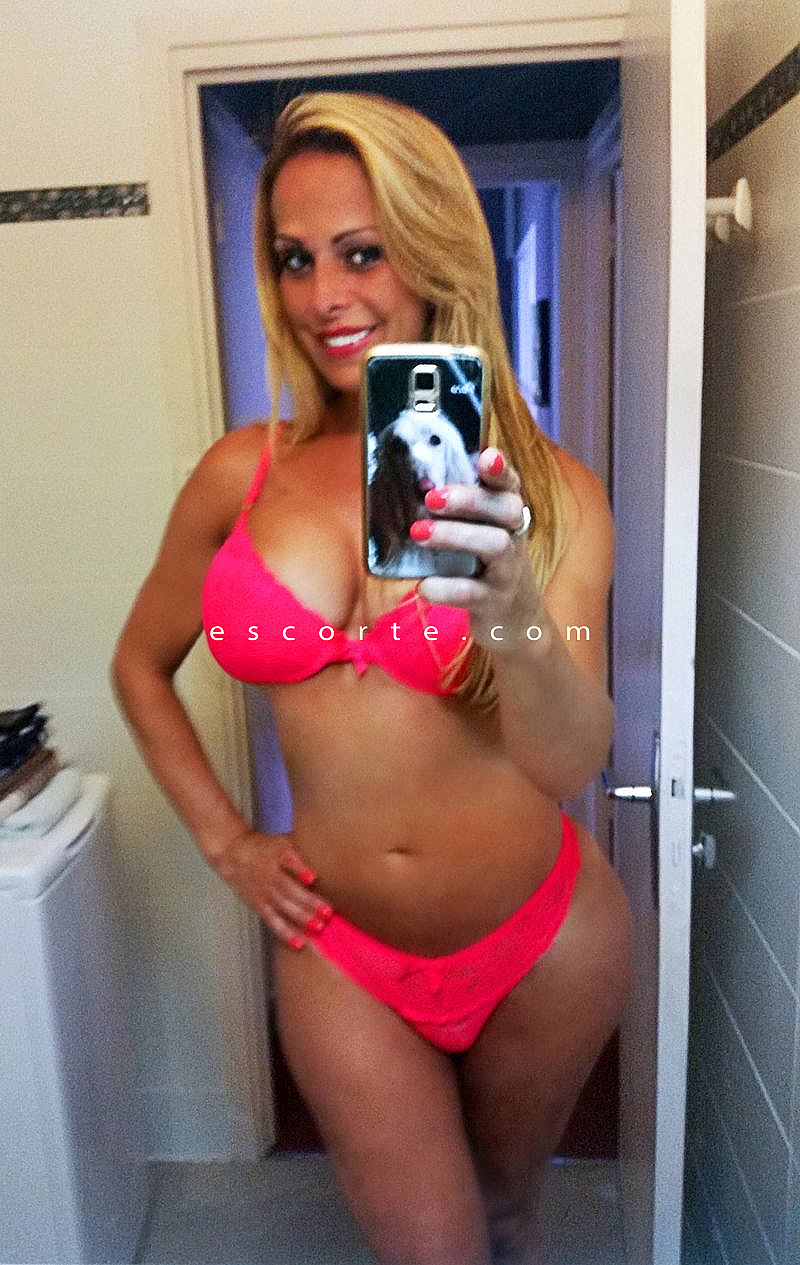
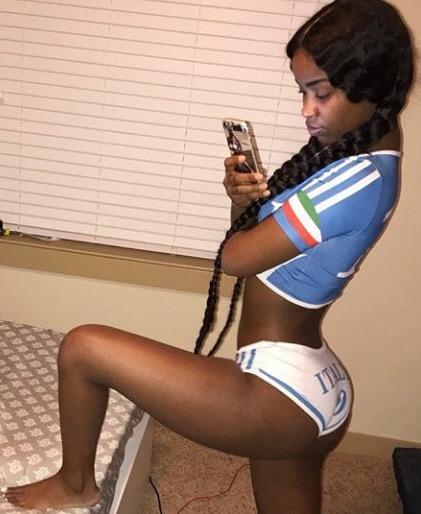
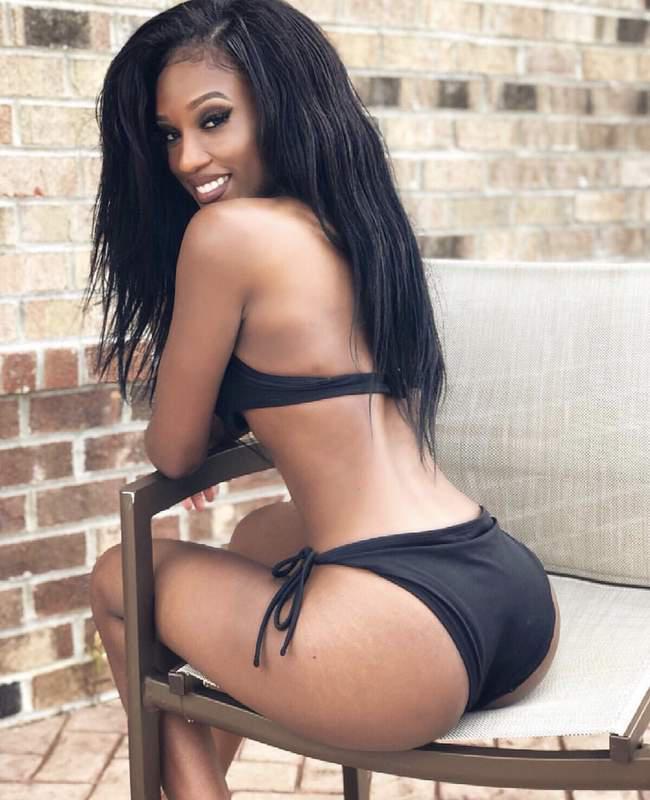



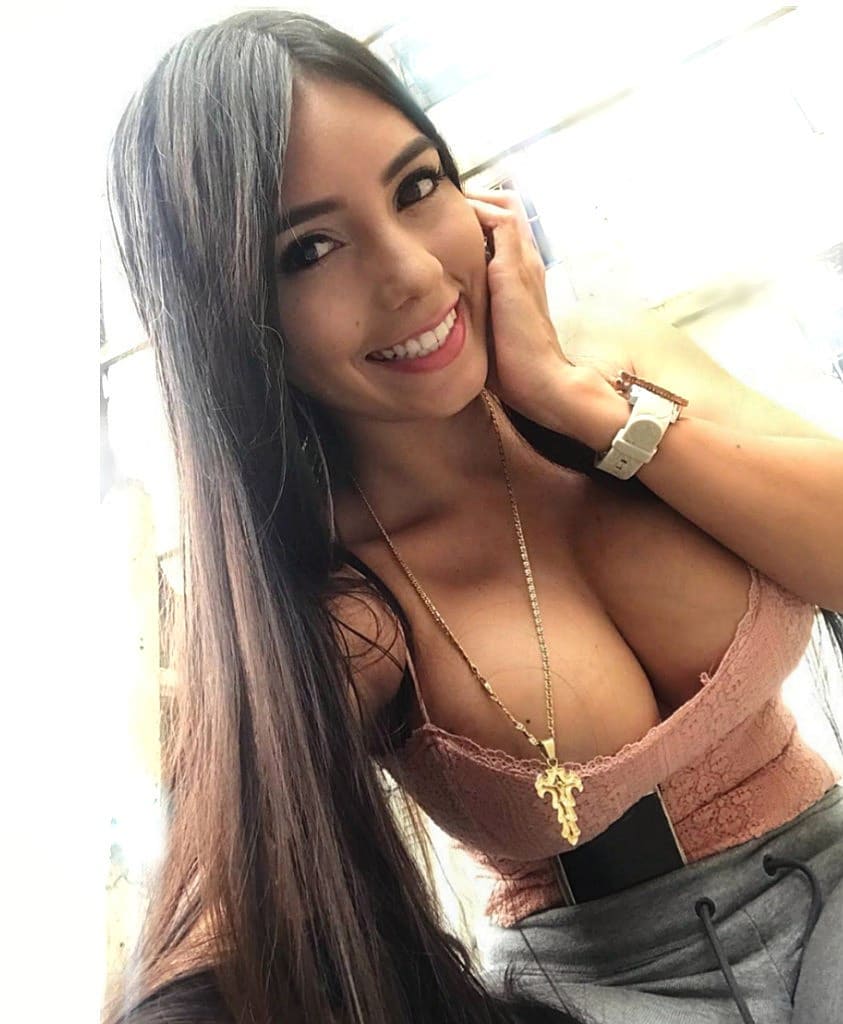



