pyTelegramBotAPI
pypi.org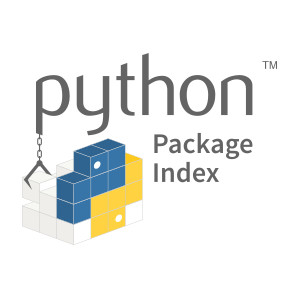
A Python implementation for the Telegram Bot API.
See https://core.telegram.org/bots/api
Creating a Telegram bot with the pyTelegramBotAPI
There are two ways to define a Telegram Bot with the pyTelegramBotAPI. ### The listener mechanism
- First, create a TeleBot instance.
import telebot TOKEN = '<token string>' bot = telebot.TeleBot(TOKEN)
- Then, define a listener function.
def echo_messages(*messages): """ Echoes all incoming messages of content_type 'text'. """ for m in messages: chatid = m.chat.id if m.content_type == 'text': text = m.text bot.send_message(chatid, text)
- Now, register your listener with the TeleBot instance and call TeleBot#polling()
bot.set_update_listener(echo_messages) bot.polling() while True: # Don't let the main Thread end. pass
- use Message’s content_type attribute to check the type of Message. Now Message supports content types:
- text
- audio
- document
- photo
- sticker
- video
- location
- contact
- new_chat_participant
- left_chat_participant
- new_chat_title
- new_chat_photo
- delete_chat_photo
- group_chat_created
- That’s it!
The decorator mechanism
- First, create a TeleBot instance.
import telebot TOKEN = '<token string>' bot = telebot.TeleBot(TOKEN)
- Next, define all of your so-called message handlers and decorate them with @bot.message_handler
# Handle /start and /help @bot.message_handler(commands=['start', 'help']) def command_help(message): bot.reply_to(message, "Hello, did someone call for help?") # Handles all messages which text matches the regex regexp. # See https://en.wikipedia.org/wiki/Regular_expression # This regex matches all sent url's. @bot.message_handler(regexp='((https?):((//)|(\\\\))+([\w\d:#@%/;$()~_?\+-=\\\.&](#!)?)*)') def command_url(message): bot.reply_to(message, "I shouldn't open that url, should I?") # Handle all sent documents of type 'text/plain'. @bot.message_handler(func=lambda message: message.document.mime_type == 'text/plain', content_types=['document']) def command_handle_document(message): bot.reply_to(message, "Document received, sir!") # Default command handler. A lambda expression which always returns True is used for this purpose. @bot.message_handler(func=lambda message: True, content_types=['audio', 'video', 'document', 'text', 'location', 'contact', 'sticker']) def default_command(message): bot.reply_to(message, "This is the default command handler.")
- And finally, call bot.polling()
bot.polling() while True: # Don't end the main thread. pass
Use whichever mechanism fits your purpose! It is even possible to mix and match.
Source pypi.org