C#
K.K- Write a c# Sharp program to calculate root of quadratic equation
using System;
public class Exercise1
{
public static void Main()
{
int a, b, c;
double d, x1, x2;
Console.Write("\n\n");
Console.Write("Calculate root of Quadratic Equation :\n");
Console.Write("----------------------------------------");
Console.Write("\n\n");
Console.Write("Input the value of a : ");
a = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the value of b : ");
b = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the value of c : ");
c = Convert.ToInt32(Console.ReadLine());
d = b * b - 4 * a * c;
if (d == 0)
{
Console.Write("Both roots are equal.\n");
x1 = -b / (2.0 * a);
x2 = x1;
Console.Write("First Root Root1= {0}\n", x1);
Console.Write("Second Root Root2= {0}\n", x2);
}
else if (d > 0)
{
Console.Write("Both roots are real and diff-2\n");
x1 = (-b + Math.Sqrt(d)) / (2 * a);
x2 = (-b - Math.Sqrt(d)) / (2 * a);
Console.Write("First Root Root1= {0}\n", x1);
Console.Write("Second Root root2= {0}\n", x2);
Console.ReadLine();
}
else
Console.Write("Root are imeainary;\nNo Solution. \n\n");
Console.ReadLine();
}
}
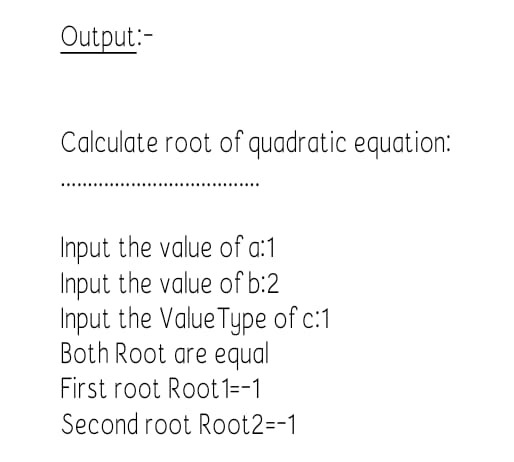
2. write a program to in c# sharp to count the frequency of each element of an array
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Frequency2
{
class Program
{
static void Main(string[] args)
{
int[] arr1 = new int[100];
int[] fr1 = new int[100];
int n, i, j, ctr;
Console.WriteLine("\n\n Count the Frequency of Each Each Element of an Array:\n");
Console.Write("--------------------------------------------------------------\n");
Console.Write("Input the number of Elements to be stored in the array:\n");
n = Convert.ToInt32(Console.ReadLine());
Console.Write("Input {0} element in the array:\n", n);
for (i = 0; i < n; i++)
{
Console.Write("Element-{0}-", i);
arr1[i] = Convert.ToInt32(Console.ReadLine());
fr1[i] = -1;
}
for (i = 0; i < n; i++)
{
ctr = 1;
for (j = i + 1; j < n; j++)
{
if (arr1[i] == arr1[j])
{
ctr++;
fr1[j] = 0;
}
}
if (fr1[i] != 0)
{
fr1[i] = ctr;
}
}
Console.Write("\n The Frequency of all element of the array:\n");
for (i = 0; i < n; i++)
{
if (fr1[i] != 0)
{
Console.WriteLine("{0} occurs {1} times\n", arr1[i], fr1[i]);
Console.ReadLine();
}
}
}
}
}
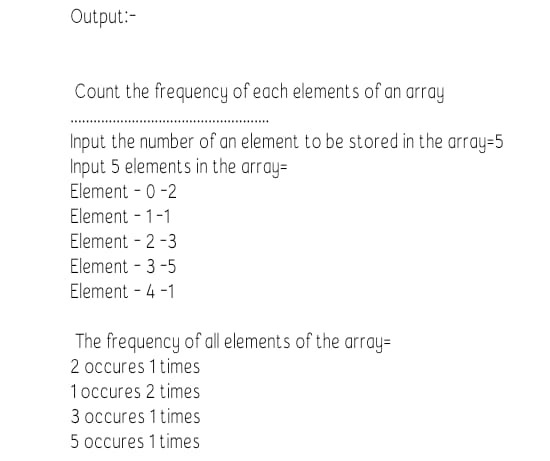
3. write a program to display the selected date in the calender
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace DateCalender3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void dateTimePicker1_ValueChanged(object sender, EventArgs e)
{
dateTimePicker1.Format = dateTimePicker1.Format;
dateTimePicker1.Value = DateTime.Today;
}
private void button1_Click(object sender, EventArgs e)
{
DateTime idate;
idate = dateTimePicker1.Value;
MessageBox.Show("selected date is" + idate);
}
}
}
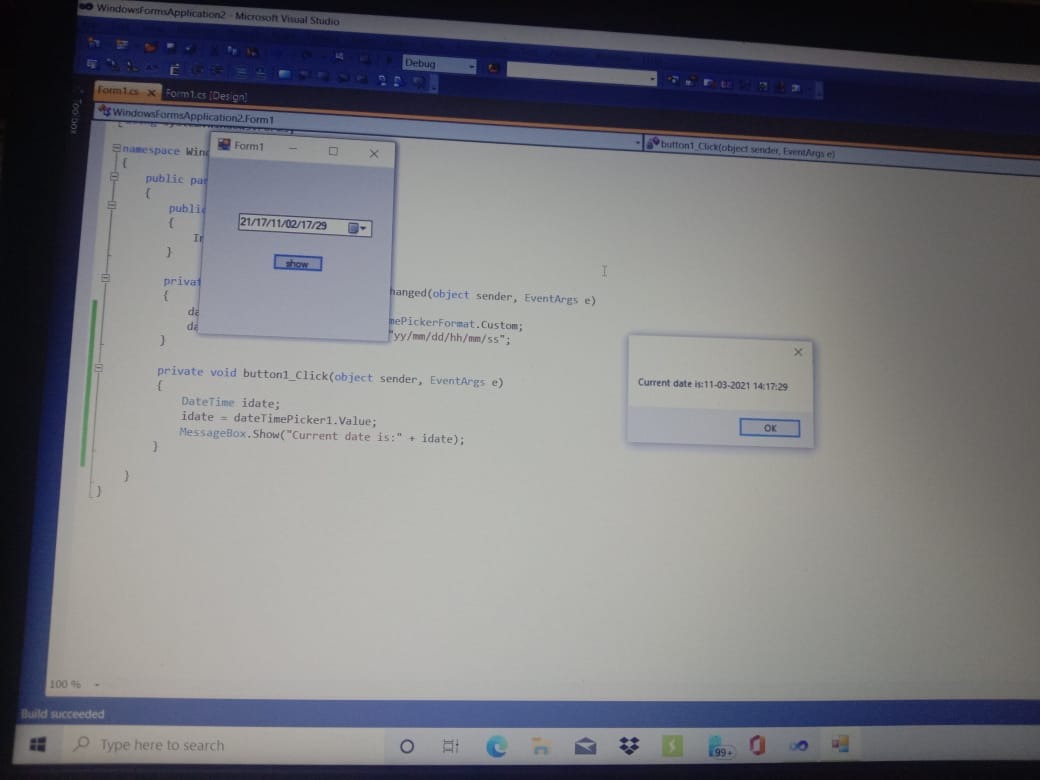
4.write a program to create a function to calculate the sum of the individual digits of a given number
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication3
{
class Program
{
static void Main(string[] args)
{
Console.Write("input a number(Only integer):-");
int n;
n = Convert.ToInt32(Console.ReadLine());
int sum = 0;
while (n != 0)
{
sum += n % 10;
n /= 10;
}
Console.WriteLine("sum of digit:" + sum);
Console.ReadLine();
}
}
}
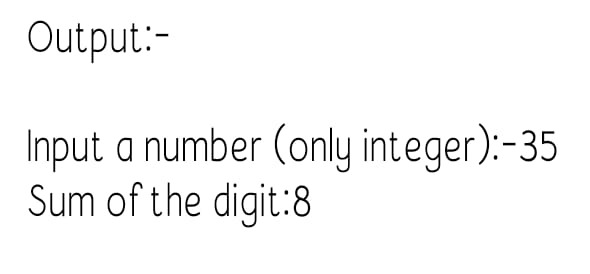
5.write a program to implement multilevel inheritance.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Inheritance5
{
class Program
{
public class A
{
public void getA()
{
Console.WriteLine("class A");
}
}
public class B : A
{
public void getB()
{
Console.WriteLine("class B");
}
}
public class C : B
{
public void getC()
{
Console.WriteLine("class C");
}
}
public static void Main(String[] args)
{
C c = new C();
c.getA();
c.getB();
c.getC();
Console.ReadLine();
}
}
}
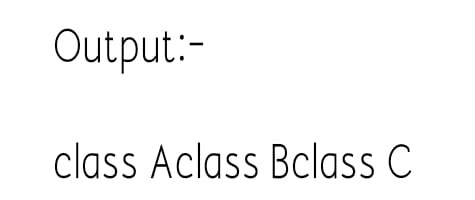
6. Create an application that allows the user to enter a number in the textbox named 'getnum'. check whether the number in the textbox 'getnum' is palindrome or not .print the message accordingly in the label control named lbldisplay when the user clicks on the button check.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace palindrome
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int rev = 0;
int num = Convert.ToInt32(getnum.Text);
int origenialnum = num;
while (num != 0)
{
int digit = num % 10;
rev = rev * 10 + digit;
num = num / 10;
}
if (rev == origenialnum)
{
lblshow.Text = "Number" + origenialnum + "is palindrome";
}
else
{
lblshow.Text = "number" + origenialnum + "is not palindrome";
}
}
private void getnum_TextChanged(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void lblshow_Click(object sender, EventArgs e)
{
}
}
}
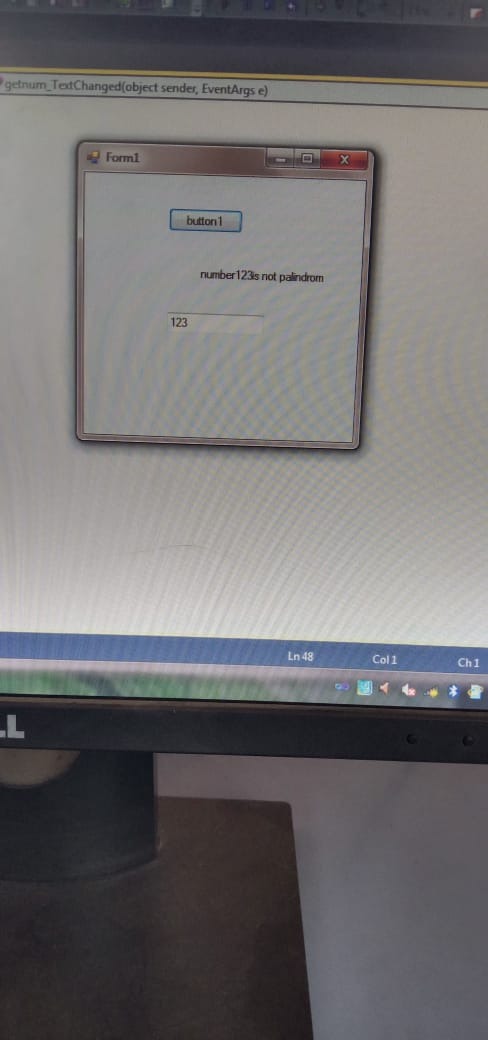
7. write a program to count a total number of alphabets, digits, special characters in a string.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace alphbet7
{
class Program
{
static void Main(string[] args)
{
String str;
int alp, digits, splch, i, l;
alp = digits = splch = i = 0;
Console.Write("\n\n count total number of alphabets,digits and special charater:\n");
Console.Write("-----------------\n");
Console.Write("Input the string:");
str = Console.ReadLine();
l = str.Length;
while (i < l)
{
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z'))
{
alp++;
}
else if (str[i] >= '0' && str[i] <= '9')
{
digits++;
}
else
{
splch++;
}
i++;
}
Console.Write("Number of alphabets in the String is :{0}\n", alp);
Console.Write("Number of digits in the string is :{0}\n", digits);
Console.Write("Number of Special Characters in the string is:{0}\n", splch);
Console.ReadLine();
}
}
}
8. C# program to demonstrate iList interface
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace lab10
{
class Program
{
static void Main(string[] args)
{
int[] a = new int[3];
a[0] = 1;
a[1] = 2;
a[2] = 3;
Display(a);
List<int> list = new List<int>();
list.Add(5);
list.Add(7);
list.Add(9);
Display(list);
Console.ReadLine();
}
static void Display(IList<int> list)
{
Console.WriteLine("count:{0}",list.Count);
foreach (int num in list)
{
Console.WriteLine(num);
Console.ReadLine();
}
}
}
}
9.write a program to display the contact number of an author using database.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
using System.Data.SqlClient;
namespace ShivarajK102
{
class Program
{
static void Main(string[] args)
{
string Dpath=@"Data Source=(LocalDB)\v11.0;AttachDbFilename=c:\users\admin26\documents\visual studio 2012\Projects\ShivarajK102\ShivarajK102\Database102.mdf;Integrated Security=True ";
SqlConnection con = new SqlConnection(Dpath);
con.Open();
Console.WriteLine("Enter the name of the Author");
string name= Console.ReadLine();
Console.WriteLine("Enter a Contact number of Author");
string Contact = Console.ReadLine();
string qry = "Insert into Info(AName,AContact) values('" + name + "','" + Contact + " ')";
SqlCommand cmd = new SqlCommand(qry,con);
cmd.ExecuteNonQuery();
con.Close();
Console.WriteLine("Data inserted Successfully");
Console.ReadLine();
}
}
}